vue3
ref、reactive
toRefs
setup + ref + reactive 实现了数据响应式,不能使用 ES6 解构,会消除响应特性。所以需要 toRefs 解构,使用时,需要先引入。
let me = reactive({
single:true,
want:"暖的像火炉的暖男"
})
//运行为
let me = proxy : { single: true, want:"暖的像火炉的暖男" }
const { single, want } = toRefs( me )
toRef
toRef作用:将对象某一个属性,作为引用返回。
let me = reactive({
single:true,
want:"暖的像火炉的暖男"
})
let lv = toRef( me, 'love' )
console.log('love',love);
//打印结果
ObjectRefImpl {
__v_isRef: true
_key: "love"
_object: Proxy {single: true, want: "暖的像火炉的暖男"}
value: undefined
[[Prototype]]: Object
}
toRef 是组件之间进行传值值,对可选参数进行处理,运行时,先查看 me中是否存在 love ,如果存在时就继承 me 中的 love ,如果不存在时就创建一个 love ,然后解构赋值给变量 lv。
methods
props、context 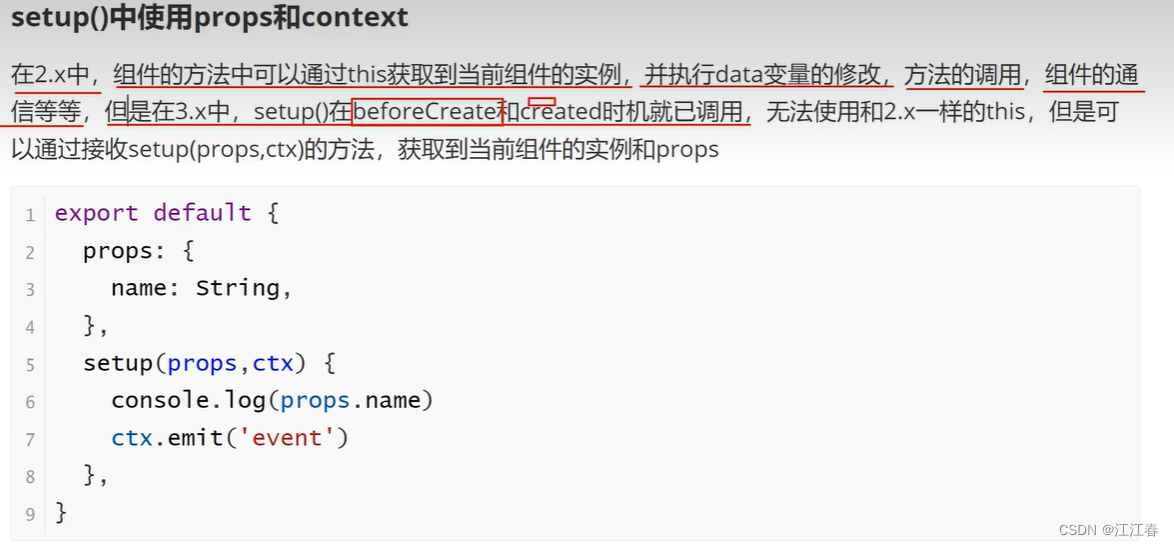
生命周期
script setup
- 无需return向外暴露,无需set up
<template> {{todoList}} </template> <script setup> let todoList = [ {todo:"我想看海",isCheck:false}, {todo:"我想浪漫",isCheck:true}, ] </script>
- 无需注册component,引用即可用
<template> <SetUp></SetUp> <set-up></set-up> </template> <script setup> import SetUp from "./SetUp.vue" </script>
使用 defineProps 方法来获取props,取代setup中的props
-
const props = defineProps({ a: String, b: String })
- 获取 attrs、slots 和 emits(setup( props, context )接收两个参数,context 上下文环境,其中包含了属性、插槽、自定义事件三部分。)
使用 script setup 语法糖时,
<script setup> import { useAttrs, useSlots } from 'vue' const slots = useSlots(); const attrs = useAttrs(); const emits = defineEmits(['getChild']); </script>
- useAttrs 方法 获取 attrs 属性
- useSlots 方法获取 slots 插槽
- defineEmits 方法获取 emit 自定义事件
- 对外暴露属性
子
<template>
{{msg}}
</template>
<script setup>
import { ref } from 'vue'
let msg = ref("Child Components");
// defineExpose无需导入,直接使用
defineExpose({
msg
});
</script>
父
<template>
<Child ref="child" />
</template>
<script setup>
import { ref, onMounted } from 'vue'
import Child from './components/Child.vue'
let child = ref(null);
onMounted(() => {
console.log(child.value.msg); // Child Components
console.log(child.value.num); // 123
})
</script>
Computed与watch
watch
import { watch } from "vue"
watch(
name ,
( curVal , preVal )=>{ //业务处理 },
options
)
共有三个参数,分别为:
- name :需要帧听的属性
- (curVal,preVal)=>{ //业务处理 } 箭头函数,是监听到的最新值和本次修改之前的值,此处进行逻辑处理。
- options :配置项,对监听器的配置,如:是否深度监听。
页面刚进入的时候并不会执行,值发生改变的时候,才会打印出当前最新值和修改之前的值。
Computed
import { ref, computed } from "vue"
export default{
setup(){
const num1 = ref(1)
const num2 = ref(1)
let sum = computed(()=>{
return num1.value + num2.value
})
}
}
mixin混入(vue2)
mixin 对象把一些组件公用的选项,如data内数据,方法、计算属性、生命周期钩子函数,单独提取出来,然后在组件内引入,就可以与组件本身的选项进行合并。
<script>
const myMixin = {
data(){
return {
num:520
}
},
mounted(){
console.log('mixin mounted');
}
}
export default {
mixins:[myMixin],
}
</script>
属性相同、同名方法
属性值相同时,优先继承实例内的值
命周期钩子函数执行顺序
生命周期函数会合并执行,优先执行 mixin 中的, 然后再执行实例中的