一、分页
1、HospPlusConfig中配置分页插件
1
/**
2
*
分页插件
3
*/
4
@Bean
5
public
PaginationInterceptor
paginationInterceptor
() {
6
return new
PaginationInterceptor();
7
}
2、分页Controller方法
HospitalSetController中添加分页方法
1
@ApiOperation
(value =
"
分页医院设置列表
"
)
2
@GetMapping
(
"{page}/{limit}"
)
3
public
R
pageList
(
4
@ApiParam
(name =
"page"
, value =
"
当前页码
"
, required =
true
)
5
@PathVariable
Long page,
6
7
@ApiParam
(name =
"limit"
, value =
"
每页记录数
"
, required =
true
)
8
@PathVariable
Long limit){
9
10
Page<HospitalSet> pageParam =
new
Page<>(page, limit);
11
12
hospitalSetService.page(pageParam,
null
);
13
List<HospitalSet> records = pageParam.getRecords();
14
long total = pageParam.getTotal();
15
16
return
R.ok().data(
"total"
, total).data(
"rows"
, records);
17
}
3、Swagger中测试
二、条件查询
根据医院名称,医院编号查询
1、创建查询对象
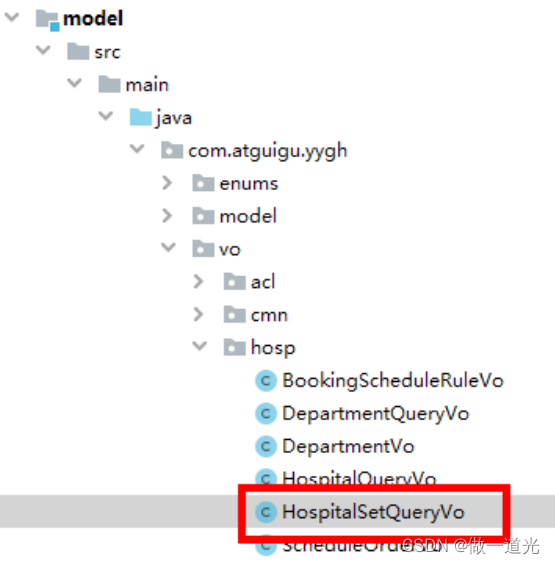
1
@Data
2
public class
HospitalSetQueryVo
{
3
4
@ApiModelProperty
(value =
"
医院名称
"
)
5
private
String hosname;
6
7
@ApiModelProperty
(value =
"
医院编号
"
)
8
private
String hoscode;
9
}
2、controller
1
@ApiOperation
(value =
"
分页条件医院设置列表
"
)
2
@PostMapping
(
"{page}/{limit}"
)
3
public
R
pageQuery
(
4
@ApiParam
(name =
"page"
, value =
"
当前页码
"
, required =
true
)
5
@PathVariable
Long page,
6
7
@ApiParam
(name =
"limit"
, value =
"
每页记录数
"
, required =
true
)
8
@PathVariable
Long limit,
9
10
@ApiParam
(name =
"hospitalSetQueryVo"
, value =
"
查询对象
"
, required =
false
)
11
@RequestBody
(required =
false
) HospitalSetQueryVo hospitalSetQueryVo){
12
13
Page<HospitalSet> pageParam =
new
Page<>(page, limit);
14
15
QueryWrapper<HospitalSet> queryWrapper =
new
QueryWrapper<>();
16
17
if
(hospitalSetQueryVo ==
null
){
18
hospitalSetService.page(pageParam, queryWrapper);
19
}
else
{
20
String hosname = hospitalSetQueryVo.getHosname();
21
String hoscode = hospitalSetQueryVo.getHoscode();
22
23
if
(!StringUtils.isEmpty(hosname)) {
24
queryWrapper.like(
"hosname"
, hosname);
25
}
26
27
if
(!StringUtils.isEmpty(hoscode) ) {
28
queryWrapper.eq(
"hoscode"
, hoscode);
29
}
30
hospitalSetService.page(pageParam, queryWrapper);
31
}
32
33
List<HospitalSet> records = pageParam.getRecords();
34
long total = pageParam.getTotal();
35
36
return
R.ok().data(
"total"
, total).data(
"rows"
, records);
37
}