一、题1
1.1 题目描述
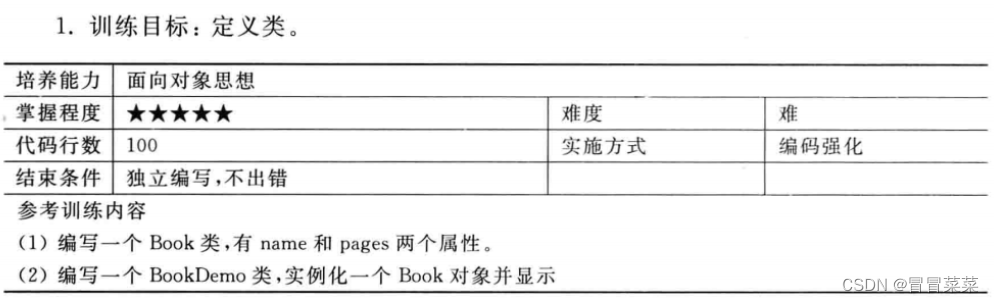
1.2 代码块
public class Book {
private String name;
private int pages;
public String getname()
{
return name;
}
public void setname(String name)
{
this.name=name;
}
public int getPages()
{
return pages;
}
public void setPages(int pages)
{
this.pages = pages;
}
public Book()
{}
public Book(String name,int pages)
{
this.name = name;
this.pages=pages;
}
}
public class BookDemo {
public static void main(String[] args)
{
Book book = new Book ("Java 8 基础应用与开发",339);
System.out.println("这本书的名字是:" + book.getname());
System.out.println("这本书的页码是:" + book.getPages());
}
}
1.3 运行截图
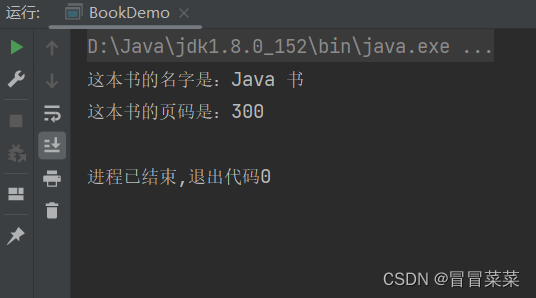
二、题2
2.1 题目描述
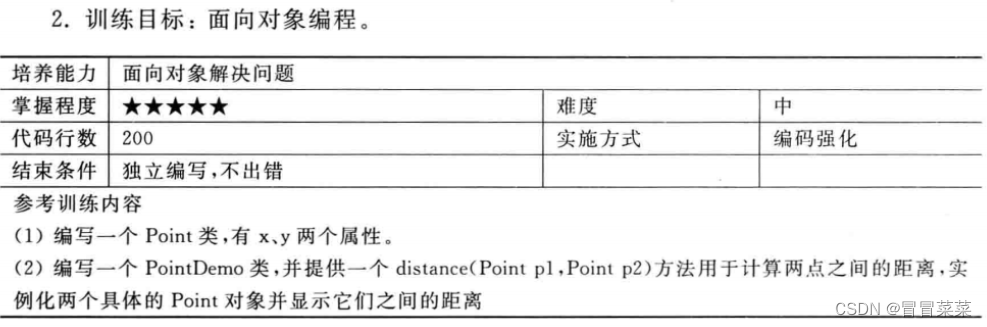
2.2 代码块
public class Point {
private double x;
private double y;
public double getX() {
return x;
}
public double getY() {
return y;
}
public void setX(double x) {
this.x = x;
}
public void setY(double y) {
this.y = y;
}
}
import java.util.Scanner;
import static java.lang.Math.*;
public class PointDemo {
public static void main(String[] args){
Scanner sc = new Scanner(System.in);
System.out.println("请输入第一个点:");
double x1 = sc.nextDouble();
double y1 = sc.nextDouble();
Point p1 = new Point();
p1.setX(x1);
p1.setY(y1);
System.out.println("请输入第二个点:");
double x2 = sc.nextDouble();
double y2 = sc.nextDouble();
Point p2 = new Point();
p1.setX(x2);
p1.setY(y2);
double ans1 = (Math.pow(p1.getX(),2)- Math.pow(p2.getX(),2))+(Math.pow(p1.getY(),2)- Math.pow(p2.getY(),2));
double ans2 = Math.sqrt(ans1);
double ans3 = Math.abs(ans2);
System.out.println("两点距离是:"+ans3);
}
}
2.3 运行截图
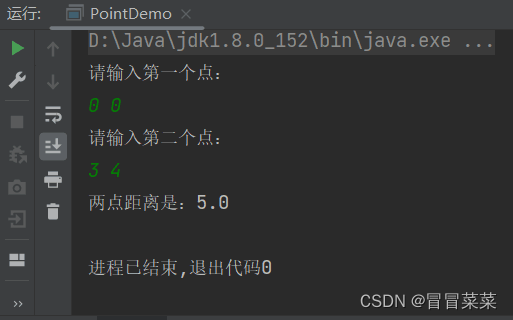