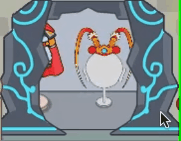
import MyBaseView from "./MyBaseView";
const { ccclass, property } = cc._decorator;
@ccclass
export default class ScrollCard extends MyBaseView {
@property({ tooltip: "是否自动展示" })
Move_zidong: boolean = true;
@property({ tooltip: "自动展示速度" })
Speed_zidong: number = 0.05;
@property({ tooltip: "是否纵方向" })
Direction_isHorizontal: boolean = true;
@property({ tooltip: "每个item的间隔" })
itemOffset: number = 0;
@property({ tooltip: "移动速度" })
speed: number = 500;
@property({ tooltip: "减速频率" })
rub: number = 1.0;
@property({ tooltip: "缩放最小值" })
scaleMin: number = 1.0;
@property({ tooltip: "缩放最大值" })
scaleMax: number = 1.0;
@property({ type: [cc.Node], tooltip: "滚动item" })
item: cc.Node[] = [];
_startTime = 0;
_moveSpeed = 0;
itemList = [];
_maxSize;
_screenRect;
onLoad() {
this._initItemPos();
this.updateScale();
}
public onTouchBegan(event: cc.Event.EventTouch): boolean {
this.clickNode = null;
if (this.getIsClickNode(this.node, event.getLocation())) {
this.clickNode = this.node;
this._moveSpeed = 0;
this._startTime = new Date().getTime();
return true;
}
return false;
}
public onTouchMoved(event: cc.Event.EventTouch): void {
if (this.clickNode) {
let movePos = event.getDelta();
this.itemMoveBy(movePos);
}
}
public onTouchEnd(event: cc.Event.EventTouch): void {
if (this.clickNode) {
this.clickNode = null;
let curpos = event.getLocation();
let startpos = event.getStartLocation();
let dis;
if (this.Direction_isHorizontal) {
dis = startpos.x - curpos.x;
} else {
dis = startpos.y - curpos.y;
}
let curTime = new Date().getTime();
let disTime = curTime - this._startTime;
this._moveSpeed = dis / disTime;
}
}
ontou
_initItemPos() {
this.node.anchorY = 0.5;
this.node.anchorX = 0.5;
this._maxSize = new cc.Size(0, 0);
for (let i = 0; i < this.item.length; i++) {
this._maxSize.width += this.item[i].width;
this._maxSize.height += this.item[i].height;
this._maxSize.width += this.itemOffset;
this._maxSize.height += this.itemOffset;
}
let startPos;
if (this.Direction_isHorizontal) {
startPos = cc.v2(-this._maxSize.width * this.node.anchorX, -this._maxSize.height * this.node.anchorY);
} else {
startPos = cc.v2(this._maxSize.width * this.node.anchorX, this._maxSize.height * this.node.anchorY);
}
this._screenRect = new cc.Rect(startPos.x, startPos.y, this._maxSize.width, this._maxSize.height);
this.itemList = [];
for (let i = 0; i < this.item.length; i++) {
let anchor = this.item[i].getAnchorPoint();
let itemSize = this.item[i].getContentSize();
if (this.Direction_isHorizontal) {
startPos.addSelf(cc.v2(itemSize.width * anchor.x, itemSize.height * anchor.y));
this.item[i].x = startPos.x;
this.item[i].y = 0;
startPos.addSelf(cc.v2(itemSize.width * anchor.x, itemSize.height * anchor.y));
startPos.addSelf(cc.v2(this.itemOffset, this.itemOffset));
} else {
startPos.subSelf(cc.v2(itemSize.width * anchor.x, itemSize.height * anchor.y));
this.item[i].x = 0;
this.item[i].y = startPos.y;
startPos.subSelf(cc.v2(itemSize.width * anchor.x, itemSize.height * anchor.y));
startPos.subSelf(cc.v2(this.itemOffset, this.itemOffset));
}
this.itemList[i] = this.item[i];
}
}
itemMoveBy(pos) {
for (let i = 0; i < this.item.length; i++) {
if (this.Direction_isHorizontal) {
this.item[i].x += pos.x;
} else {
this.item[i].y += pos.y;
}
}
this.updatePos();
}
updatePos() {
let startItem = this.itemList[0];
let endItem = this.itemList[this.itemList.length - 1];
let startout = false;
if (this.Direction_isHorizontal) {
if (startItem.x < -this._maxSize.width / 2) {
startout = true;
}
} else {
if (startItem.y > this._maxSize.width / 2) {
startout = true;
}
}
if (startout) {
let item = this.itemList.shift();
this.itemList.push(item);
if (this.Direction_isHorizontal) {
item.x = endItem.x + endItem.width + this.itemOffset;
} else {
item.y = endItem.y - endItem.height - this.itemOffset;
}
}
let endout = false;
if (this.Direction_isHorizontal) {
if (endItem.x > this._maxSize.width / 2) {
endout = true;
}
} else {
if (endItem.y < -this._maxSize.height / 2) {
endout = true;
}
}
if (endout) {
let item = this.itemList.pop();
this.itemList.unshift(item);
if (this.Direction_isHorizontal) {
item.x = startItem.x - startItem.width - this.itemOffset;
} else {
item.y = startItem.y + startItem.height + this.itemOffset;
}
}
this.updateScale();
}
updateScale() {
if (this.scaleMax < this.scaleMin || this.scaleMax == 0) {
return;
}
for (let i = 0; i < this.item.length; i++) {
let pre;
if (this.Direction_isHorizontal) {
let x = this.item[i].x + this._maxSize.width / 2;
if (this.item[i].x < 0) {
pre = x / this._maxSize.width;
}
else {
pre = 1 - x / this._maxSize.width;
}
} else {
let y = this.item[i].y + this._maxSize.height / 2;
if (this.item[i].y < 0) {
pre = y / this._maxSize.height;
}
else {
pre = 1 - y / this._maxSize.height;
}
}
pre *= 2;
let scaleTo = this.scaleMax - this.scaleMin;
scaleTo *= pre;
scaleTo += this.scaleMin;
scaleTo = Math.abs(scaleTo);
this.item[i].scaleX = scaleTo;
this.item[i].scaleY = scaleTo;
}
}
update(dt) {
if (this._moveSpeed == 0) {
if (this.Move_zidong && !this.clickNode) {
for (let i = 0; i < this.item.length; i++) {
if (this.Direction_isHorizontal) {
this.item[i].x -= this.Speed_zidong * dt * this.speed;
} else {
this.item[i].y -= this.Speed_zidong * dt * this.speed;
}
}
let moveTo = -this._moveSpeed * dt * this.speed;
this.itemMoveBy(cc.v2(moveTo, moveTo))
this.updatePos();
}
return;
}
for (let i = 0; i < this.item.length; i++) {
if (this.Direction_isHorizontal) {
this.item[i].x -= this._moveSpeed * dt * this.speed;
} else {
this.item[i].y -= this._moveSpeed * dt * this.speed;
}
}
if (this._moveSpeed > 0) {
this._moveSpeed -= dt * this.rub;
if (this._moveSpeed < 0) {
this._moveSpeed = 0;
}
} else {
this._moveSpeed += dt * this.rub;
if (this._moveSpeed > 0) {
this._moveSpeed = 0;
}
}
let moveTo = -this._moveSpeed * dt * this.speed;
this.itemMoveBy(cc.v2(moveTo, moveTo))
this.updatePos();
}
}