数据结构–二叉树的层遍历
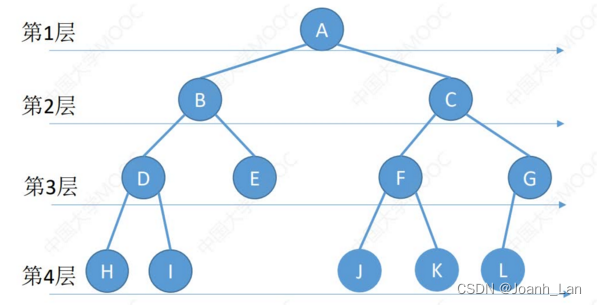

算法思想:
①初始化一个辅助
队列
\color{red}队列
队列
②根结点入队
③若队列非空,则队头结点出队,访问该结点,并将其左、右孩子插入队尾(如果有的话)
④重复③直至队列为空
代码实现
typedef struct BiTNode
{
ElemType data; //数据域
struct BiTNode *lchild, *rchild; //左、右孩子指针
struct BiTnode *parent; //父节点指针
}BiTNode, *BiTree;
typedef struct LinkNode
{
BiTNode *data;
struct LinkNode* next;
}LinkNode;
typedef struct
{
LinkNode *front, *rear;
}LinkQueue;
void initQueue(LinkQueue &Q)
{
Q.front = Q.rear = (LinkNode*)malloc(sizeof(LinkNode));
Q.front->next = NULL;
}
void EnQueue(LinkQueue &Q, BiTree T)
{
LinkNode* s = (LinkNode*)malloc(sizeof(LinkNode));
s->data = T;
s->next = NULL;
Q.rear->next = s;
Q.rear = s;
}
bool DeQueue(LinkQueue &Q, BiTree& T)
{
if (Q.front == Q.rear) return false;
LinkNode* p = Q.front->next;
T = p->data;
Q.front->next = p->next;
if (Q.rear == p)
Q.rear = Q.front;
free(p);
return true;
}
bool isEmpty(LinkQueue Q)
{
return Q.front == Q.rear;
}
void LevelOrder(BiTree T)
{
LinkQueue Q;
initQueue(Q);
BiTree p;
EnQueue(Q, T);
while(!isEmpty(Q))
{
DeQueue(Q, p);
visit(p); //访问出队元素
if (p->lchild != NULL)
EnQueue(Q, p->lchild);
if (p->rchild != NULL)
EnQueue(Q, p->rchild);
}
}
知识点回顾与重要考点
树的层次遍历算法思想:
①初始化一个辅助
队列
\color{red}队列
队列
②根结点入队
③若队列非空,则队头结点出队,访问该结点,并将其左、右孩子插入队尾(如果有的话)
④重复③直至队列为空