一、线程简介
多任务,看似同时在做,实际上同一时间只做一件事
多线程,相当于路上多加一条车道
普通方法vs多线程:
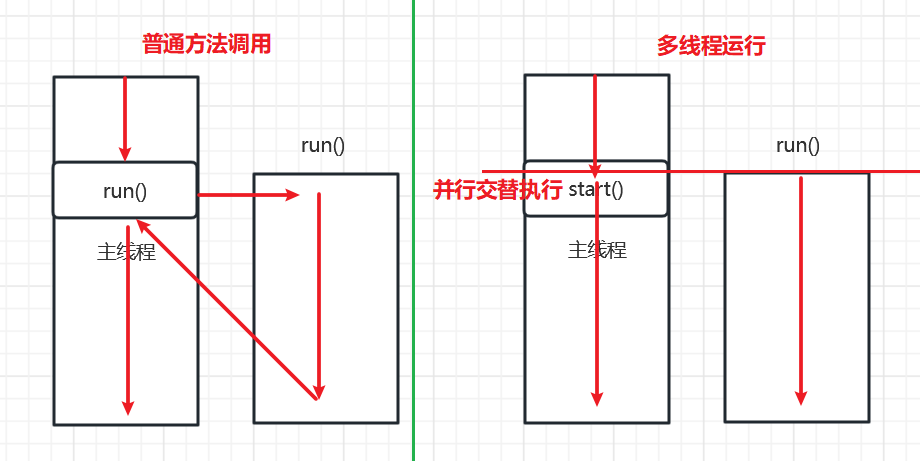
程序、进程、线程:
程序:静态的概念,程序和数据的有序集合
进程:操作系统中运行的程序就是一个个进程,如qq、播放器、浏览器等
是系统资源分配的一个个单位,程序的执行过程,是动态的概念
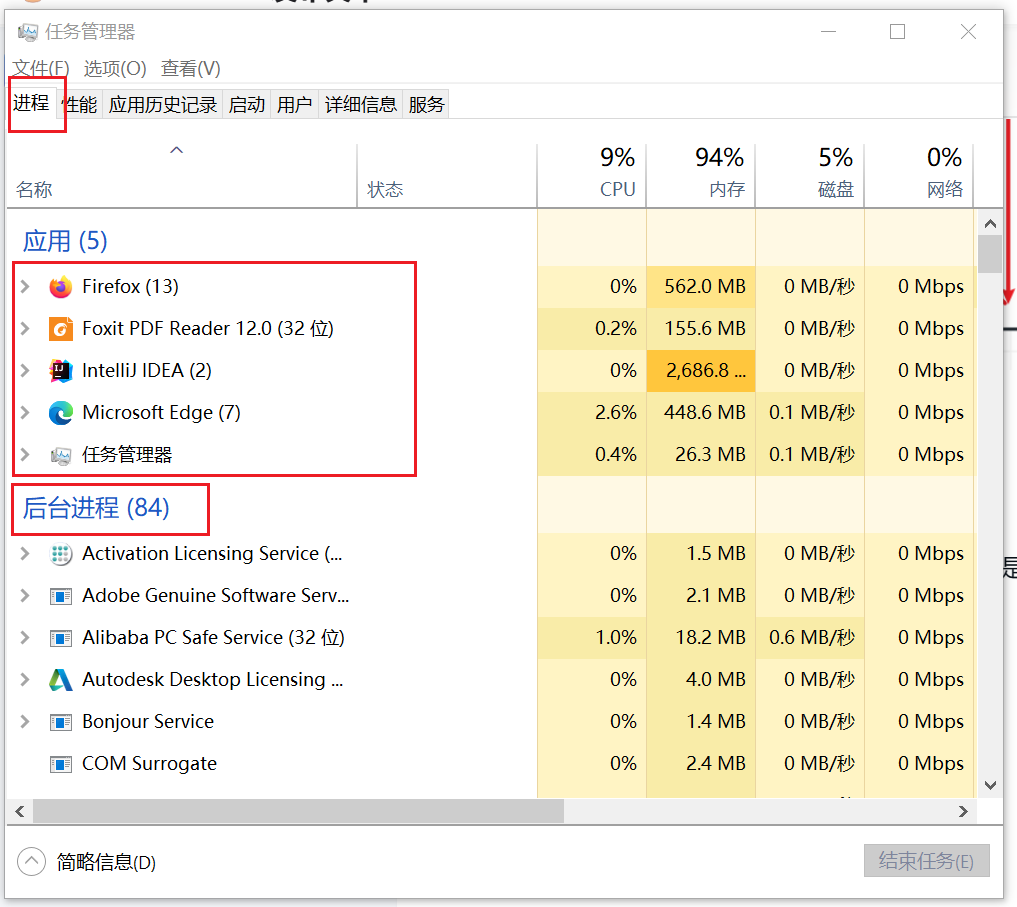
线程:一个进程里包括多个线程,如看B站时,图像、语音、弹幕多个线程组成了一个视频
是cpu的执行调度的单位,多线程是模拟出来的,多cpu才是真的多线程
二、线程实现(重点)
2.1 三种创建方式
Thread类(重点)
Runnable接口(重点)
Callable接口(了解)
2.2 Thread
StartThread1类
public class StartThread1 extends Thread{
// 重写线程入口点
@Override
public void run() {
// 线程体
System.out.println("正在执行线程1");
}
}
Test类(main方法在这)
public static void main(String[] args) {
// 创建线程对象
Thread th1 = new StartThread1();
Thread th2 = new StartThread1();
Thread th3 = new StartThread1();
/*
调用线程
线程执行是由CPU进行调度的
*/
th1.start();
th2.start();
th3.start();
}
2.3 Runnable
2.4 插播! 龟兔赛跑
Race线程,通过name判断线程执行的代码
注意,thread.sleep(1000)要 catch(InterruptedException e)
public class Race extends Thread{
private int miles = 35;
public int getMiles(){
return miles;
}
public void setMiles(int miles){
this.miles = miles;
}
public void run()
{
// 小兔子
if(getName() == "rabbit"){
// 记录剩余路线长度
int leftMiles = 0;
for (int i = 5; i <= 35; i+=5){
try {
if(i != 35){
// run
Thread.sleep(1000);
leftMiles = miles - i;
System.out.println("小兔子向前跑了5米!还剩" + leftMiles + "米");
// sleep
if( i % 10 == 0){
Thread.sleep(2000);
System.out.println("小兔子睡着啦");
}
}else{
// last run
Thread.sleep(1000);
System.out.println("小兔子向前跑了5米,跑到终点啦");
}
}catch (InterruptedException e){
e.printStackTrace();
}
}
}
// 小乌龟
if(getName() == "turtle"){
int leftMiles = miles;
// 记录剩余路线长度
for (int i = 3; i <= 35; i+=4){
try {
Thread.sleep(1000);
leftMiles = miles - i;
System.out.println("小乌龟向前爬了3米, 还剩" + leftMiles + "米");
}catch (InterruptedException e){
e.printStackTrace();
}
}
try {
Thread.sleep(1000);
System.out.println("小乌龟到终点了!");
}catch (InterruptedException e){
e.printStackTrace();
}
}
}
}
test类执行
public class Test {
public static void main(String[] args) {
// Thread中有name属性,Race继承了Thread,就不用单独在Race中写name了
Thread rabbit = new Race();
rabbit.setName("rabbit");
Thread turtle = new Race();
turtle.setName("turtle");
rabbit.start();
turtle.start();
}
}
2.5 callable 略
三、线程状态
3.1 进程状态
新建、就绪、阻塞、运行、结束
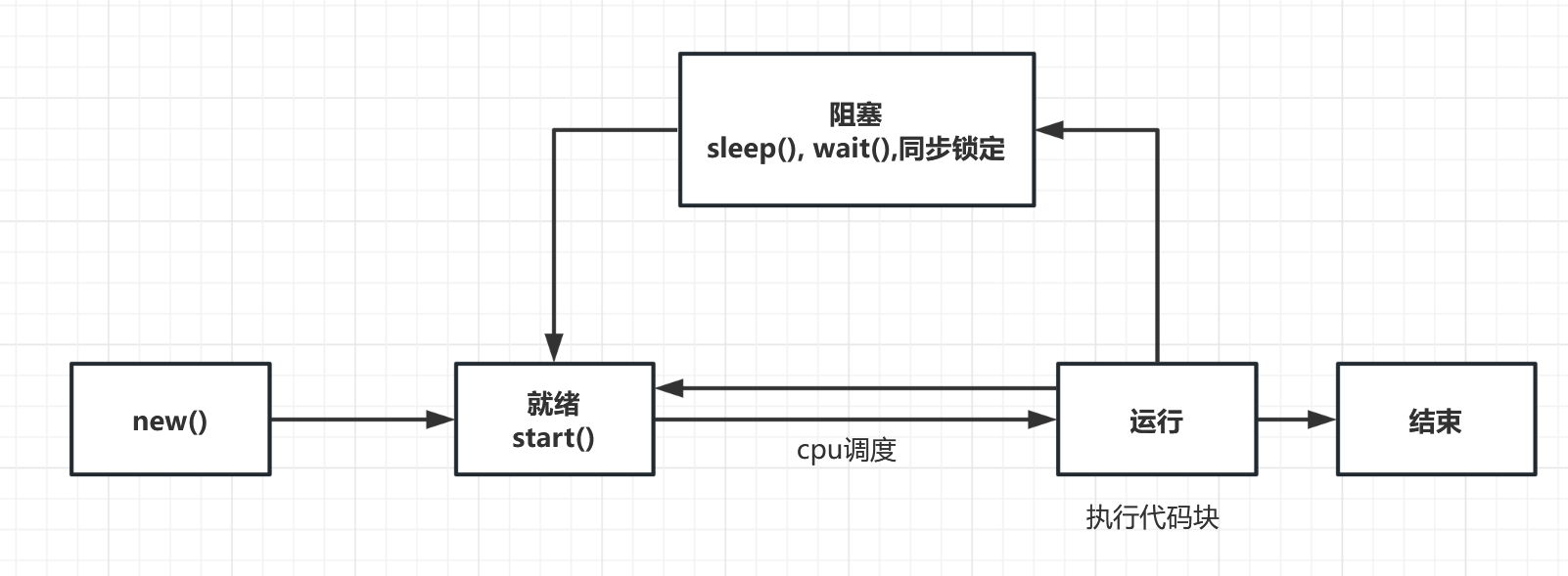