2.1.1、创建 .aidl 文件
// IImoocAidl.aidl
package com.test.server;
// Declare any non-default types here with import statements
interface IImoocAidl {
// 计算两个数的和
int add(int num1,int num2);
}
然后make project,会生成IImoocAidl.java文件
项目目录:
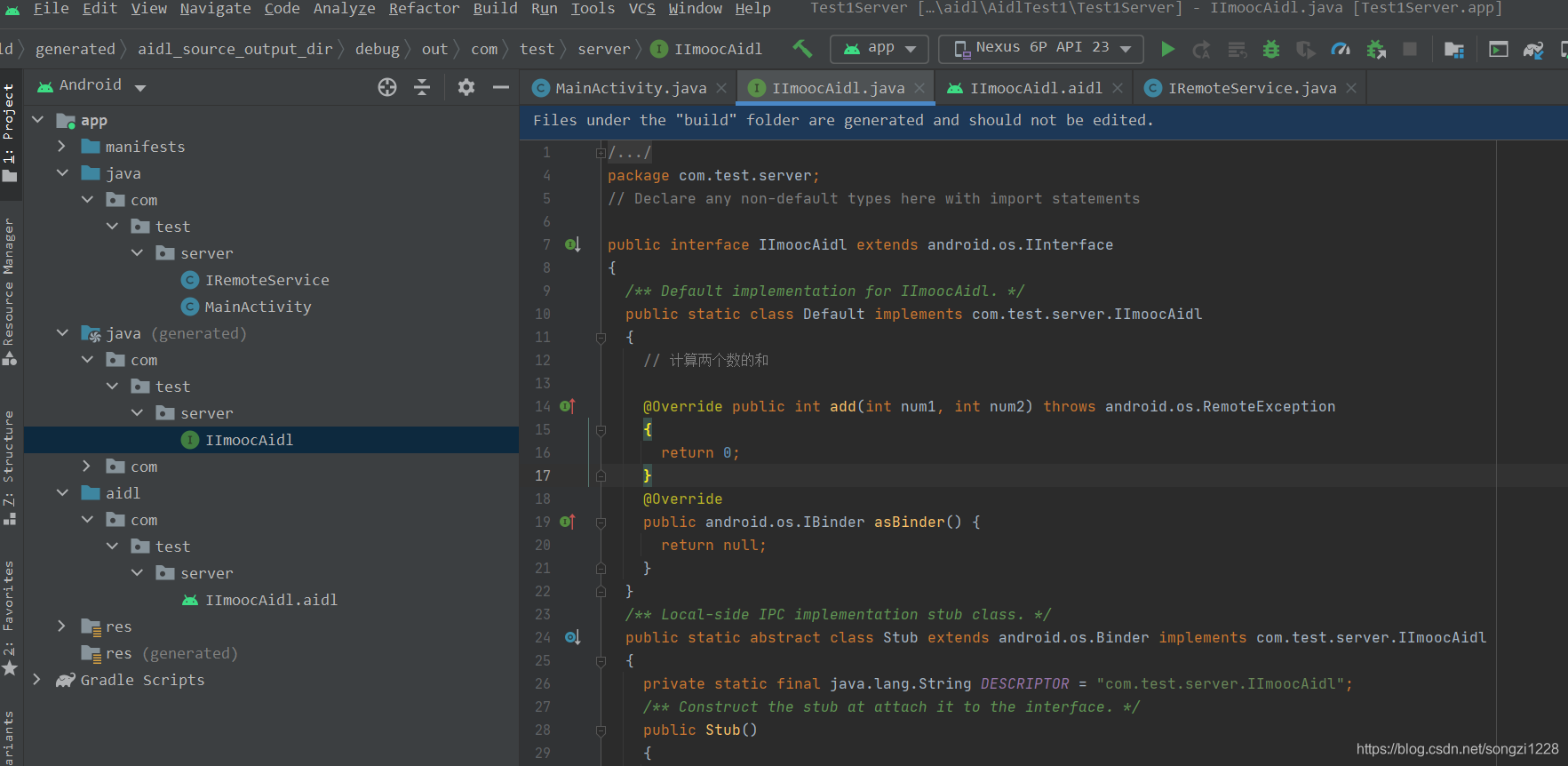
2.1.2、实现接口
public class IRemoteService extends Service {
private static final String TAG = “IRemoteService”;
@Nullable
@Override
public IBinder onBind(Intent intent) {
return iBinder;
}
private IBinder iBinder = new IImoocAidl.Stub() {
@Override
public int add(int num1, int num2) throws RemoteException {
Log.e(TAG, "收到了远程的请求,请求的参数是:num1 = " + num1 + "num2 = " + num2);
return num1 + num2;
}
};
}
2.1.3、向客户端公开接口
public class IRemoteService extends Service {
private static final String TAG = “IRemoteService”;
/**
-
当客户端绑定到该服务的时候,会执行此方法
-
@param intent
-
@return
*/
@Nullable
@Override
public IBinder onBind(Intent intent) {
return iBinder;
}
private IBinder iBinder = new IImoocAidl.Stub() {
@Override
public int add(int num1, int num2) throws RemoteException {
Log.e(TAG, "收到了远程的请求,请求的参数是:num1 = " + num1 + "num2 = " + num2);
return num1 + num2;
}
};
}
2.1.4、配置文件注册Service并启动Service
配置文件注册:
<service
android:name=“.IRemoteService”
android:exported=“true” />
启动Service:
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Intent intent = new Intent(this, IRemoteService.class);
startService(intent);
}
}
2.2、客户端
在同项目中新建一个名为client的module
2.2.1、创建客户端界面
ClientActivity
public class ClientActivity extends AppCompatActivity {
EditText etNum1, etNum2;
TextView tvRes;
Button btnAdd;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_client);
etNum1 = findViewById(R.id.et_num1);
etNum2 = findViewById(R.id.et_num2);
tvRes = findViewById(R.id.tv_res);
btnAdd = findViewById(R.id.btn_add);
btnAdd.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
}
});
}
}
布局文件:
<?xml version="1.0" encoding="utf-8"?><androidx.constraintlayout.widget.ConstraintLayout xmlns:android=“http://schemas.android.com/apk/res/android”
xmlns:app=“http://schemas.android.com/apk/res-auto”
xmlns:tools=“http://schemas.android.com/tools”
android:layout_width=“match_parent”
android:layout_height=“match_parent”
tools:context=“.ClientActivity”>
<EditText
android:id=“@+id/et_num1”
android:layout_width=“match_parent”
android:layout_height=“wrap_content”
android:hint=“输入第一个数字”
android:textSize=“22sp”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent”
app:layout_constraintTop_toTopOf=“parent” />
<TextView
android:id=“@+id/tv_add”
android:layout_width=“wrap_content”
android:layout_height=“wrap_content”
android:text=“+”
android:textSize=“100sp”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent”
app:layout_constraintTop_toBottomOf=“@+id/et_num1” />
<EditText
android:id=“@+id/et_num2”
android:layout_width=“match_parent”
android:layout_height=“wrap_content”
android:hint=“输入第二个数字”
android:textSize=“22sp”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent”
app:layout_constraintTop_toBottomOf=“@+id/tv_add” />
<TextView
android:id=“@+id/tv_res”
android:layout_width=“wrap_content”
android:layout_height=“wrap_content”
android:layout_marginTop=“10dp”
android:text=“计算结果”
android:textSize=“40sp”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent”
app:layout_constraintTop_toBottomOf=“@+id/et_num2” />
<Button
android:id=“@+id/btn_add”
android:layout_width=“match_parent”
android:layout_height=“wrap_content”
android:layout_marginTop=“10dp”
android:text=“计算”
android:textSize=“22sp”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent”
app:layout_constraintTop_toBottomOf=“@+id/tv_res” />
</androidx.constraintlayout.widget.ConstraintLayout>
2.2.2、把服务端的aidl文件拷贝到客户端
2.2.3、绑定服务并拿取数据
public class ClientActivity extends AppCompatActivity {
private static final String TAG = “ClientActivity”;
EditText etNum1, etNum2;
TextView tvRes;
Button btnAdd;
IImoocAidl iImoocAidl;
private ServiceConnection mConn = new ServiceConnection() {
@Override