使用文件IO 实现父进程向子进程发送信息,并总结中间可能出现的各种问题
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/wait.h>
#define MAX_SIZE 256
int main(int argc, const char *argv[])
{
int fd[2];
pid_t pid;
char buf[MAX_SIZE];
//创建管道
if(pipe(fd)==-1)
{
perror("pipe");
return -1;
}
pid=fork();
//父进程发送消息到管道,写之前关闭读端
if(pid>0)
{
close(fd[0]);//关闭读端
strcpy(buf,"hello my son");
write(fd[1],buf,strlen(buf)+1);//传数据到管道
close(fd[1]);//关闭写端
wait(NULL);//等待子进程结束
}else if(pid==0){//子进程从管道接收消息
close(fd[1]);//关闭写端
read(fd[0],buf,MAX_SIZE);
printf("子进程接收到的%s\n",buf);
close(fd[0]);//关闭读端
}else if(pid==-1){
perror("pid");
return -2;
}
return 0;
}
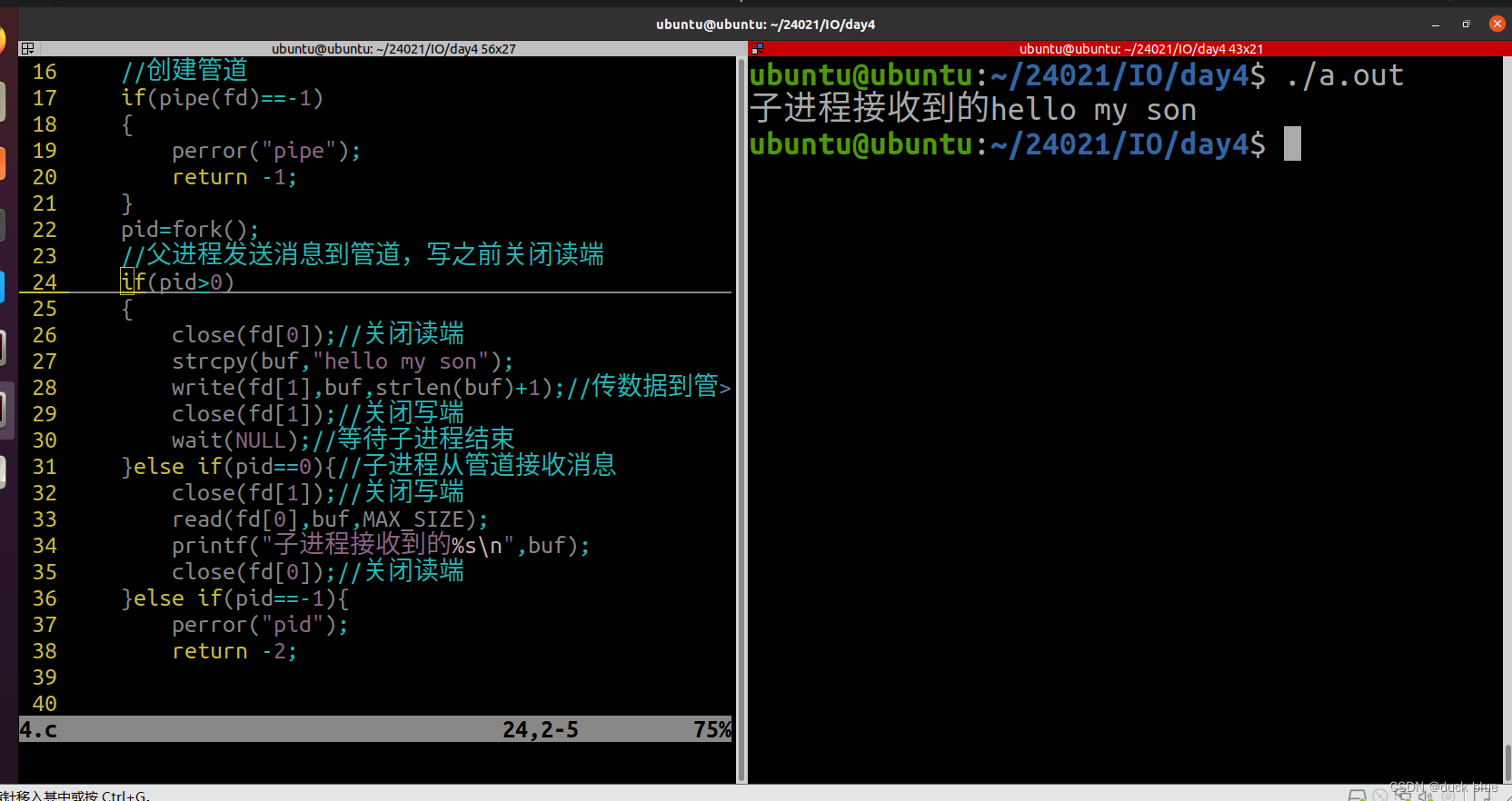