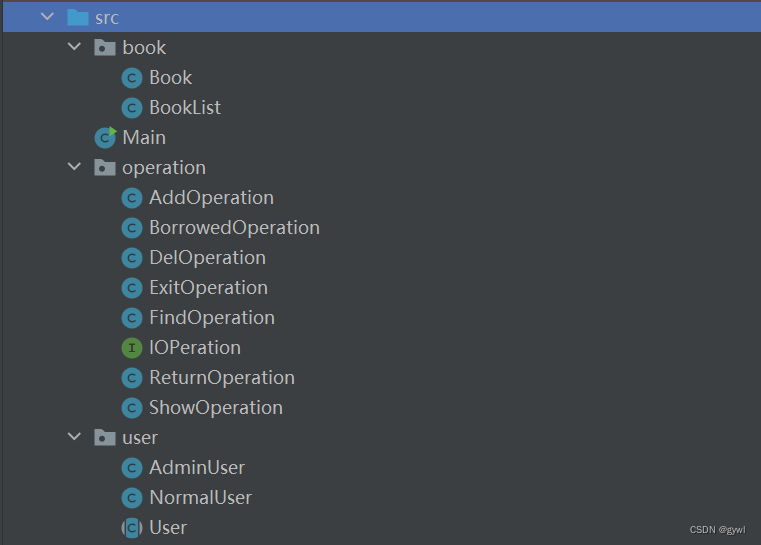
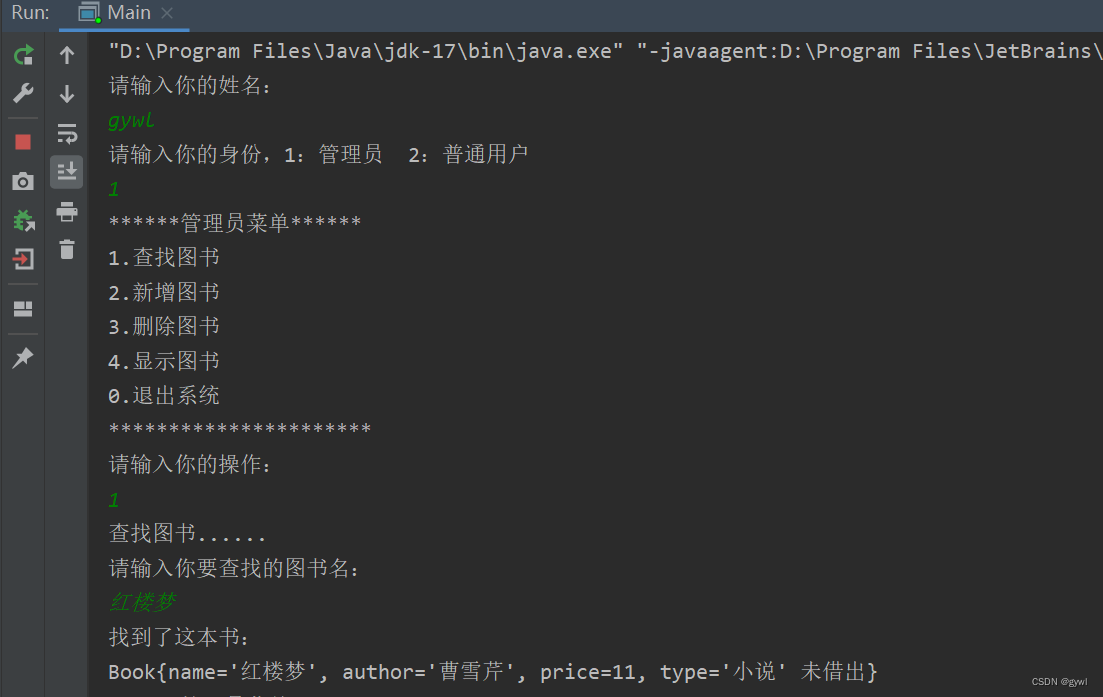
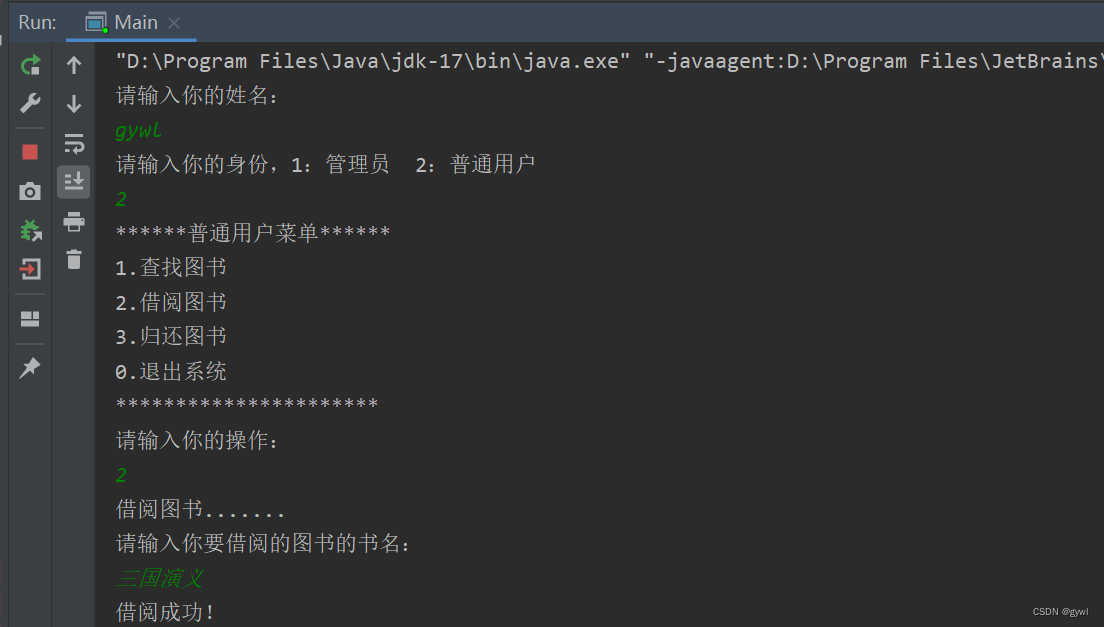
Main
import book.BookList;
import user.AdminUser;
import user.NormalUser;
import user.User;
import java.util.Scanner;
//程序入口函数
public class Main {
public static User login(){
Scanner scanner=new Scanner(System.in);
System.out.println("请输入你的姓名:");
String name=scanner.nextLine();
System.out.println("请输入你的身份,1:管理员 2:普通用户");
int choice=scanner.nextInt();
if(choice==1){
AdminUser adminUser=new AdminUser(name);
return adminUser;
}else{
NormalUser normalUser=new NormalUser(name);
return normalUser;
}
}
public static void main(String[] args) {
BookList bookList = new BookList();
//user有可能引用管理员用户 有可能引用普通用户
User user = login();
while (true) {
int choice = user.menu();
//伪代码 io[choice].work(书架);
user.doIoperations(choice, bookList);
}
}
}
book
Book
package book;
public class Book {
private String name;
private String author;
private int price;
private String type;
private boolean isLend;//是否被借出
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAuthor() {
return author;
}
public void setAuthor(String author) {
this.author = author;
}
public int getPrice() {
return price;
}
public void setPrice(int price) {
this.price = price;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public boolean isLend() {
return isLend;
}
public void setLend(boolean lend) {
isLend = lend;
}
public Book(String name, String author, int price, String type) {
this.name = name;
this.author = author;
this.price = price;
this.type = type;
//this.isLend = isLend;默认值false 未被借出
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
", author='" + author + '\'' +
", price=" + price +
", type='" + type + '\'' +
(isLend==true?" 已借出":" 未借出")+
//", isLend=" + isLend +
'}';
}
}
BookList
package book;
//书架
public class BookList {
private Book[] books = new Book[10];
private int usedSize;//用来记录当前存放了多少书
public BookList() {
books[0] = new Book("三国演义", "罗贯中", 10, "小说");
books[1] = new Book("西游记", "吴承恩", 12, "小说");
books[2] = new Book("红楼梦", "曹雪芹", 11, "小说");
this.usedSize = 3;
}
public Book getBook(int pos) {
return books[pos];
}
public void setBook(int pos,Book book){
books[pos]=book;
}
public int getUsedSize() {
return usedSize;
}
public void setUsedSize(int usedSize) {
this.usedSize = usedSize;
}
public boolean isFull() {
if (this.usedSize == books.length) {
return true;
}
else return false;
}
}
operation
AddOperation
package operation;
import book.BookList;
import book.Book;
import java.util.Scanner;
public class AddOperation implements IOPeration {
public void work(BookList bookList){
System.out.println("新增图书......");
if(bookList.isFull()){
System.out.println("书架满了 不能新增了!");
return;
}
System.out.println("请输入你要新增的图书的书名:");
Scanner scanner=new Scanner(System.in);
String bookName=scanner.nextLine();
System.out.println("请输入你要新增的图书的作者:");
String author=scanner.nextLine();
System.out.println("请输入你要新增图书的价格:");
int price=scanner.nextInt();
System.out.println("请输入你要新增的图书的类型:");
String type=scanner.nextLine();
Book book=new Book(bookName,author,price,type);
int currentSize=bookList.getUsedSize();
bookList.setBook(currentSize,book);
bookList.setUsedSize(currentSize+1);
System.out.println("新增图书成功!");
}
//IOPeration[]
}
BorrowedOperation
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class BorrowedOperation implements IOPeration{
public void work(BookList bookList){
System.out.println("借阅图书.......");
System.out.println("请输入你要借阅的图书的书名:");
Scanner scanner=new Scanner(System.in);
String bookName=scanner.nextLine();
int currentSize=bookList.getUsedSize();
for(int i=0;i<currentSize;i++) {
Book book = bookList.getBook(i);
if(book.getName().equals(bookName)){
book.setLend(true);
System.out.println("借阅成功!");
return;
}
}
System.out.println("借阅失败!");
}
}
DelOperation
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class DelOperation implements IOPeration{
public void work(BookList bookList){
System.out.println("删除图书......");
System.out.println("请输入你要删除的图书的书名:");
Scanner scanner=new Scanner(System.in);
String bookName=scanner.nextLine();
int currentSize=bookList.getUsedSize();
int pos=-1;
int i;
for(i=0;i<currentSize;i++) {
Book book = bookList.getBook(i);
if (book.getName().equals(bookName)) {
//找到这本书了 记录下标
pos=i;
break;
}
}
if(i>=currentSize){
System.out.println("没有你要找的书!");
return;
}
//开始删除
for (int j = pos; j <currentSize-1 ; j++) {
Book book=bookList.getBook(j+1);
bookList.setBook(j,book);
}
bookList.setUsedSize(currentSize-1);
bookList.setBook(currentSize-1,null);
System.out.println("删除成功!");
}
}
ExitOperation
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class DelOperation implements IOPeration{
public void work(BookList bookList){
System.out.println("删除图书......");
System.out.println("请输入你要删除的图书的书名:");
Scanner scanner=new Scanner(System.in);
String bookName=scanner.nextLine();
int currentSize=bookList.getUsedSize();
int pos=-1;
int i;
for(i=0;i<currentSize;i++) {
Book book = bookList.getBook(i);
if (book.getName().equals(bookName)) {
//找到这本书了 记录下标
pos=i;
break;
}
}
if(i>=currentSize){
System.out.println("没有你要找的书!");
return;
}
//开始删除
for (int j = pos; j <currentSize-1 ; j++) {
Book book=bookList.getBook(j+1);
bookList.setBook(j,book);
}
bookList.setUsedSize(currentSize-1);
bookList.setBook(currentSize-1,null);
System.out.println("删除成功!");
}
}
ReturnOperation
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class ReturnOperation implements IOPeration {
@Override
public void work(BookList bookList) {
System.out.println("归还图书......");
System.out.println("请输入你要归还的图书的书名:");
Scanner scanner = new Scanner(System.in);
String bookName = scanner.nextLine();
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
if (book.getName().equals(bookName)) {
book.setLend(false);
System.out.println("归还成功!");
return;
}
}
System.out.println("归还失败!");
}
}
ShowOperation
package operation;
import book.Book;
import book.BookList;
public class ShowOperation implements IOPeration {
public void work(BookList bookList) {
System.out.println("显示图书.......");
int currentSize = bookList.getUsedSize();
for (int i = 0; i < currentSize; i++) {
Book book = bookList.getBook(i);
System.out.println(book);
}
}
}
FindOperation
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
public class FindOperation implements IOPeration {
public void work(BookList bookList){
System.out.println("查找图书......");
System.out.println("请输入你要查找的图书名:");
Scanner scanner=new Scanner(System.in);
String bookName= scanner.nextLine();
int currentSize= bookList.getUsedSize();
for(int i=0;i<currentSize;i++){
Book book=bookList.getBook(i);
if(book.getName().equals(bookName)){
System.out.println("找到了这本书:");
System.out.println(book);
return;
}
}
System.out.println("没有你要找的书......");
}
}
IOPration
package operation;
import book.Book;
import book.BookList;
import java.util.Scanner;
package operation;
import book.BookList;
public interface IOPeration {
void work(BookList bookList);
}
user
AdminUser
package user;
import operation.*;
import java.util.Scanner;
public class AdminUser extends User{
public AdminUser(String name) {
super(name);
this.ioPerations=new IOPeration[]{
new ExitOperation(),
new FindOperation(),
new AddOperation(),
new DelOperation(),
new ShowOperation(),
};
}
public int menu(){
System.out.println("******管理员菜单******");
System.out.println("1.查找图书");
System.out.println("2.新增图书");
System.out.println("3.删除图书");
System.out.println("4.显示图书");
System.out.println("0.退出系统");
System.out.println("**********************");
System.out.println("请输入你的操作:");
Scanner scanner=new Scanner(System.in);
int choice= scanner.nextInt();
return choice;
}
}
NormalUser
package user;
import operation.*;
import java.util.Scanner;
public class NormalUser extends User{
public NormalUser(String name) {
super(name);
this.ioPerations=new IOPeration[]{
new ExitOperation(),
new FindOperation(),
new BorrowedOperation(),
new ReturnOperation(),
};
}
public int menu(){
System.out.println("******普通用户菜单******");
System.out.println("1.查找图书");
System.out.println("2.借阅图书");
System.out.println("3.归还图书");
System.out.println("0.退出系统");
System.out.println("**********************");
System.out.println("请输入你的操作:");
Scanner scanner=new Scanner(System.in);
int choice= scanner.nextInt();
return choice;
}
}
User
package user;
import book.BookList;
import operation.IOPeration;
import operation.IOPeration;
public abstract class User {
protected String name;
protected IOPeration[] ioPerations;
public User(String name){
this.name=name;
}
public abstract int menu();
public void doIoperations(int choice,BookList bookList){
this.ioPerations[choice].work(bookList);
}
}