#include "widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
{
//设置定时器
timer=new QTimer(this);
timeid=this->startTimer(1000);
connect(timer,&QTimer::timeout,this,&Widget::timeout_slot);
speecher=new QTextToSpeech(this);
//边框
this->setFixedSize(500,400);
this->setWindowTitle("时钟");
//时钟框
lab=new QLabel(this);
this->lab->resize(220,50);
this->lab->move(50,50);
//闹钟框
edit=new QLineEdit(this);
this->edit->setInputMask("00:00");
this->edit->resize(150,50);
this->edit->move(300,30);
//启动按钮
but1=new QPushButton("启动",this);
this->but1->resize(70,30);
this->but1->move(edit->x(),edit->y()+70);
//停止按钮
but2=new QPushButton("关闭",this);
this->but2->resize(70,30);
this->but2->move(but1->x()+90,but1->y());
//语音播报框
text=new QTextEdit(this);
this->text->setPlaceholderText("铃声");
this->text->resize(400,200);
this->text->move(50,150);
connect(but1,&QPushButton::clicked,this,&Widget::on_but1_clicked);
connect(but2,&QPushButton::clicked,this,&Widget::on_but2_clicked);
}
Widget::~Widget()
{
}
void Widget::timerEvent(QTimerEvent *event)
{
if(event->timerId()==timeid)
{
QDateTime sys_dt=QDateTime::currentDateTime();
//展示时间到ui界面
this->lab->setText(sys_dt.toString("hh:mm"));
this->lab->setAlignment(Qt::AlignCenter);//设置标签文本对齐方式
this->lab->setFont(QFont("宋体",15));
}
}
void Widget::on_but1_clicked()
{
timeid1=this->startTimer(5000);
but1->setEnabled(false);
text->setEnabled(false);
}
void Widget::on_but2_clicked()
{
int ret=QMessageBox::information(this,"提示","是否取消闹钟",QMessageBox::Yes|QMessageBox::No,QMessageBox::Yes);
if(QMessageBox::Yes==ret)
{
this->killTimer(timeid1);
but1->setEnabled(true);
text->setEnabled(true);
edit->clear();
}
}
void Widget::timeout_slot()//处理timeout信号对应的槽函数
{
if(lab->text()==edit->text())
{
QString lm=text->toPlainText();
speecher->say(lm);
}
}
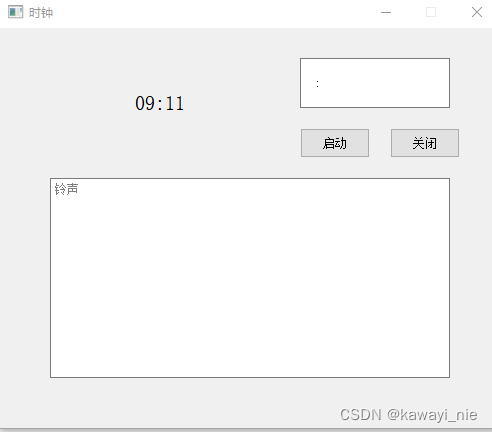