一、Xmind整理:
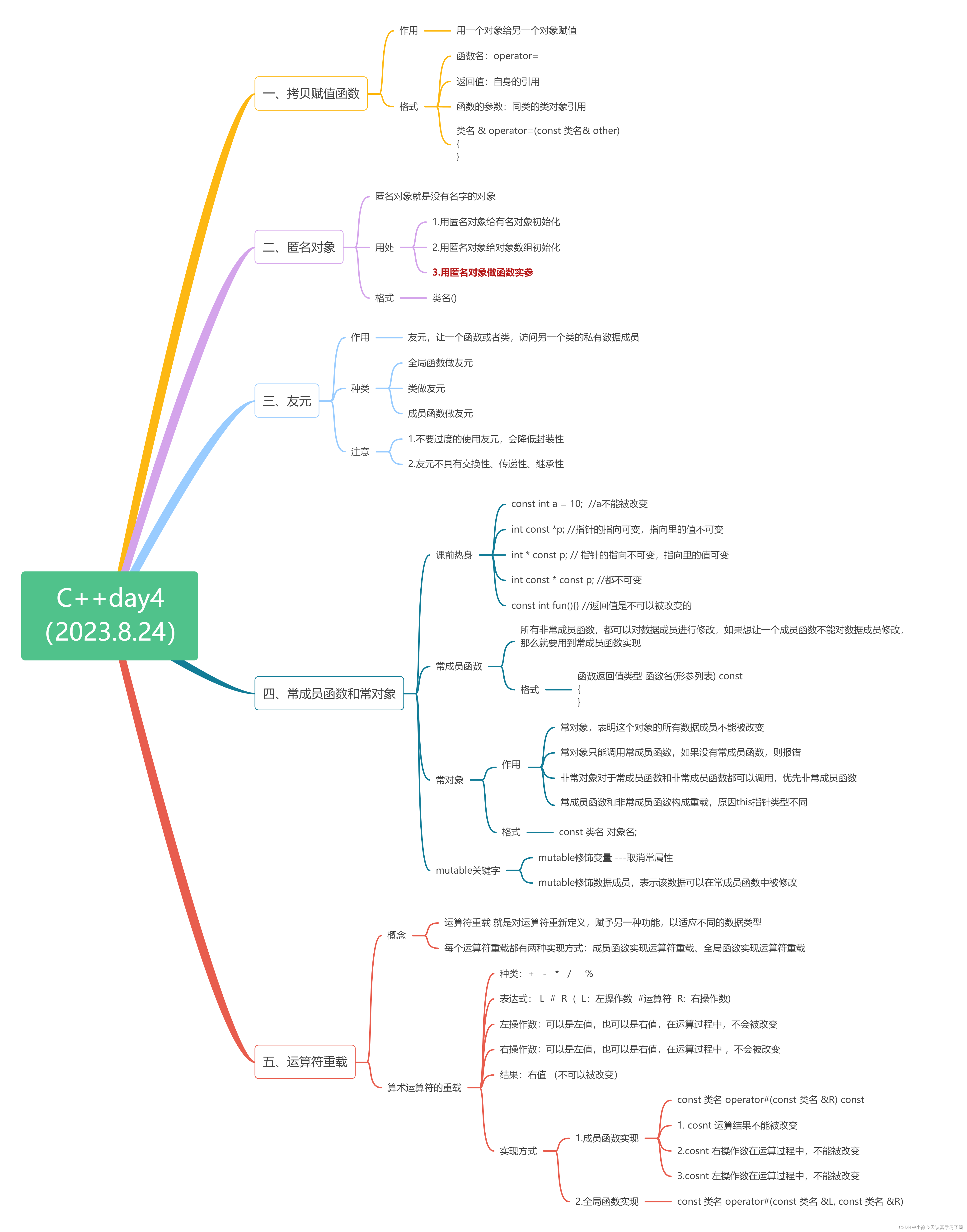
二、上课笔记整理:
1.day3作业订正:设计一个Per类,类中包含私有成员:姓名、年龄、指针成员身高...
#include <iostream>
using namespace std;
//封装人 类
class Person
{
private:
string name;
int age;
double *h; //身高
public:
//无参构造函数
Person() { cout << "Person::无参构造函数" << endl;}
//有参构造
Person(string name, int age , double h):name(name),age(age),h(new double(h))
//h = new double(h)
{cout << "Person::有参构造函数" << endl;}
//拷贝构造函数
Person(const Person& p):name(p.name),age(p.age),h(new double(*(p.h)))
{cout << "Person::拷贝构造函数" << endl; }
~Person()
{
cout << "Person::析构函数" << endl;
delete h;
}
};
//封装学生 类
class Stu
{
private:
int math;
Person p;
public:
//
Stu() {cout << "Stu::无参构造" << endl;}
//
Stu(string name, int age, double h, int math):math(math),p(name,age,h)
{cout << "Stu::有参构造" << endl;}
//拷贝构造函数
Stu(const Stu& s):math(s.math),p(s.p)
{
cout << "Stu::拷贝构造函数" << endl;
}
~Stu()
{
cout << "Stu::析构函数" << endl;
}
};
int main()
{
Stu s; //
Stu s1("张三",34,189,67); //
Stu s2 = s1; //
return 0;
}
2.封装学生的类,写出构造函数,析构函数、拷贝构造函数、拷贝赋值函数
#include <iostream>
using namespace std;
class Stu
{
private:
string name;
int age;
public:
Stu()
{
cout << "无参构造函数" << endl;
}
Stu(string name,int age):name(name),age(age)
{
cout << "有参构造函数" << endl;
}
Stu(const Stu &other):name(other.name),age(other.age)
{
cout << "拷贝构造函数" << endl;
}
Stu &operator=(const Stu &other)
{
if(this != &other)
{
name = other.name;
age = other.age;
cout << "拷贝赋值函数" << endl;
}
return *this;
}
~Stu()
{
cout << "析构函数" << endl;
}
void display()
{
cout << name << endl;
cout << age << endl;
}
};
int main()
{
Stu s1 ("zhangsan",20);
s1.display();
Stu s2 =s1;
s2.display();
Stu s3;
s3 = s2;
s3.display();
return 0;
}
3.匿名对象
#include <iostream>
using namespace std;
class Stu
{
private:
string name;
int age;
public:
Stu()
{
cout << "无参构造函数" <<endl;
}
Stu(string name,int age):name(name),age(age)
{
cout << "有参构造函数" << endl;
}
void show()
{
cout << name << endl;
cout << age << endl;
}
};
void fun(Stu s)
{
s.show();
}
int main()
{
Stu s1 = Stu("zhangsan",12);
s1.show();
Stu s[3] = {Stu("a",1),Stu("b",2),Stu("c",3)};
s[0].show();
s[1].show();
s[2].show();
fun(Stu("lisi",5));
return 0;
}
4.全局函数、类做友元
#include <iostream>
using namespace std;
class Room
{
friend void goodgay(Room &r);
friend class GoodGay;
private:
string mybedroom;
public:
string livingroom;
public:
Room()
{
mybedroom = "卧室";
livingroom = "客厅";
}
};
//类做友元
class GoodGay
{
public:
Room *r;
void visit()
{
cout << "好基友类正在访问" << r->mybedroom <<endl;
cout << "好基友类正在访问" << r->livingroom <<endl;
}
public:
GoodGay()
{
r = new Room;
}
};
//全局函数做友元
void goodgay(Room &r)
{
cout << "好基友函数正在访问" << r.mybedroom <<endl;
cout << "好基友函数正在访问" << r.livingroom <<endl;
}
int main()
{
Room r;
goodgay(r);
GoodGay g;
g.visit();
return 0;
}
5.成员函数做友元
#include <iostream>
using namespace std;
class Room;//声明有这样的类
//类做友元
class GoodGay
{
public:
Room *r;
void visit();//在类内声明
GoodGay();
};
//封装 房间 类
class Room
{
friend void GoodGay::visit(); //这个类的成员函数是本类的好朋友。可以访问本类所有成员
private:
string m_BedRoom; //卧室
public:
string m_SittingRoom; //客厅
public:
//无参
Room()
{
m_BedRoom = "卧室";
m_SittingRoom = "客厅";
}
};
void GoodGay::visit() //在类外定义成员函数
{
cout << "好基友类正在访问。。" << r->m_SittingRoom << endl;
cout << "好基友类正在访问。。" << r->m_BedRoom << endl;
}
GoodGay::GoodGay()
{
r = new Room;
}
int main()
{
GoodGay g;
g.visit();
return 0;
}
6.常成员函数
#include <iostream>
using namespace std;
class Stu
{
private:
string name;
int age;
public:
//无参构造函数
Stu()
{
}
//有参构造函数
Stu(string name, int age):name(name),age(age)
{
}
//常成员函数
void show()const
{
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
}
};
int main()
{
Stu s1("zhangsan",10);
s1.show();
return 0;
}
7.常对象+mutable关键字
#include <iostream>
using namespace std;
class Stu
{
private:
string name;
mutable int age;
public:
//无参构造函数
Stu()
{
}
//有参构造函数
Stu(string name, int age):name(name),age(age)
{
}
//常成员函数
void show()const
{
age = 19;
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
}
//非常成员函数
void show()
{
age = 16;
cout << "姓名:" << name << endl;
cout << "年龄:" << age << endl;
}
};
int main()
{
//非常对象
Stu s1("zhangsan",10);
s1.show();
//常对象
const Stu s2("lisi",15);
s2.show();
return 0;
}
8.实现+号运算符重载(成员函数、全局函数)
#include <iostream>
using namespace std;
class Person
{
// friend const Person operator+(const Person &L, const Person &R);
private:
int a;
int b;
public:
//无参构造函数
Person()
{
}
//有参构造函数
Person(int a, int b):a(a),b(b)
{
}
//成员函数实现 + 号运算符重载
const Person operator+(const Person &R)const
{
Person temp;
temp.a = a + R.a;
temp.b = b + R.b;
return temp;
}
void show()
{
cout << "a = " << a << endl;
cout << "b = " << b << endl;
}
};
//全局函数实现 + 号运算符重载
//const Person operator+(const Person &L, const Person &R)
//{
// Person temp;
// temp.a = L.a + R.a;
// temp.b = L.b + R.b;
// return temp;
//}
int main()
{
Person p1(10,10);
Person p2(10,10);
Person p3 = p1 + p2;
p3.show();
return 0;
}