目录
引言
一、string类对象的修改操作
1.1 push_back()
1.2 append()
1.3 operator+=()
1.4 c_str()
1.5 substr()
1.6 find()
1.7 rfind()
二、string类非成员函数
2.1 operator+()
2.2 operator<<()
2.3 operator>>()
2.4 getline()
2.5 relational operators编辑
结语
引言
🔍在本博客中,我们将一起踏入
string
类的奥秘世界,探索它的对象修改操作。我们将学会如何添加、删除、替换甚至是重塑字符串,就像在迷宫中开辟新的通路一样。而为了更好地解谜,我们还将了解一些非成员函数,这些函数就像是迷宫中的暗门,为我们提供了额外的洞察力和力量。
无论是解决实际问题,还是纯粹的编程乐趣,string
类都是你的得力助手。让我们一起踏上这段关于C++魔法宝剑的探险之旅吧!🚀📜
一、string类对象的修改操作
1.1 push_back()
在 C++ 中,std::string
类具有一个名为 push_back()
的成员函数,用于向字符串的末尾添加一个字符。
以下是使用 push_back()
函数的示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
std::cout << "Before push_back: " << str << std::endl;
str.push_back('!'); // 向字符串末尾添加 '!'
std::cout << "After push_back: " << str << std::endl;
return 0;
}
在上面的示例中,我们首先创建了一个字符串 str
("Hello"),然后使用 push_back()
函数将字符'!' 添加到字符串的末尾,使其变为 "Hello!"。
需要注意的是,push_back()
函数只能添加一个字符到字符串末尾。
1.2 append()
在 C++ 中,std::string
类具有名为 append()
的成员函数,它用于将一个字符串、子串或字符序列添加到另一个字符串的末尾。
下面对主要的版本进行解释
string& append (const string& str);
这个重载版本接受一个std::string
对象作为参数,它将参数中的字符串内容追加到当前字符串的末尾。
string& append (const string& str, size_t subpos, size_t sublen);
这个版本允许你从参数字符串的指定位置subpos
开始,追加长度为sublen
的子串到当前字符串末尾。
string& append (const char* s);
该版本接受一个 C 风格的字符串指针作为参数,将参数字符串内容追加到当前字符串的末尾。
string& append (const char* s, size_t n);
这个版本与上一个版本类似,但是你可以指定要追加的字符数n
,而不是追加整个 C 字符串。
string& append (size_t n, char c);
这个版本在当前字符串的末尾添加n
个字符c
,用于填充指定数量的字符。
以下是使用 append()
函数的示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
std::cout << "Before append: " << str << std::endl;
str.append(", world!"); // 将子串 ", world!" 添加到字符串末尾
std::cout << "After append: " << str << std::endl;
return 0;
}
在上述示例中,我们首先创建了一个字符串 str
("Hello"),然后使用 append()
函数将子串 ", world!" 添加到字符串的末尾,使其变为 "Hello, world!"。
append()
函数可以接受多种不同类型的参数,包括 C 字符串、std::string
对象、字符序列等。这使得你能够很方便地将不同类型的文本内容追加到一个字符串中。
总之,append()
函数是用于向 std::string
的末尾添加字符串、子串或字符序列的成员函数,它可以实现字符串的动态拼接。
1.3 operator+=()
在C++中,std::string
类支持 operator+=
运算符重载,允许你将一个字符串或字符序列追加到另一个字符串的末尾。这是一种便捷的方式来修改字符串内容。
对各个版本进行解释
string& operator+= (const string& str);
这个重载版本接受一个std::string
对象作为参数,它将参数中的字符串内容追加到当前字符串的末尾。
string& operator+= (const char* s);
该版本接受一个 C 风格的字符串指针作为参数,将参数字符串内容追加到当前字符串的末尾。
string& operator+= (char c);
这个版本接受一个字符c
作为参数,将这个字符追加到当前字符串的末尾。
以下是使用 operator+=
运算符的示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello";
std::cout << "Before operator+=: " << str << std::endl;
str += ", world!"; // 使用 operator+= 将 ", world!" 追加到字符串末尾
std::cout << "After operator+=: " << str << std::endl;
return 0;
}
在上述示例中,我们首先创建了一个字符串 str
("Hello"),然后使用 operator+=
运算符将 ", world!" 追加到字符串的末尾,使其变为 "Hello, world!"。
你可以将任何能够隐式转换为 std::string
对象的类型与 operator+=
运算符一起使用,这包括字符串、字符、C 字符串等。
1.4 c_str()
在 C++ 中,std::string
类的成员函数 c_str()
用于获取一个指向以 null 结尾的 C 字符串(即 C 风格字符串)的指针,该字符串与 std::string
对象的内容相同。这个函数通常在需要将 std::string
转换为 C 字符串的场景中很有用。
以下是如何使用 c_str()
函数的示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
const char *cstr = str.c_str(); // 获取与字符串内容相同的 C 字符串
std::cout << "C-style string: " << cstr << std::endl;
return 0;
}
在上述示例中,我们使用 c_str()
函数获取了与字符串 str
的内容相同的 C 字符串,并将其指针赋值给 cstr
。然后,我们通过 cout
输出了这个 C 字符串。
需要注意的是,c_str()
函数返回的指针指向的 C 字符串是一个常量,不能通过这个指针修改字符串的内容。
总之,c_str()
函数是一个方便的方法,可以将 std::string
转换为 C 字符串,适用于需要与 C 风格函数或库进行交互的情况。
1.5 substr()
substr()
函数接受两个参数:起始索引位置和子串的长度。它返回一个新的 std::string
对象,其中包含了从起始索引开始的指定长度的子串。
以下是 substr()
函数的用法示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::string sub = str.substr(7, 5); // 从索引位置 7 开始,提取长度为 5 的子串
std::cout << "Substring: " << sub << std::endl;
return 0;
}
在上面的示例中,我们首先创建了一个字符串 str
("Hello, world!"),然后使用 substr()
函数从索引位置 7 开始,提取长度为 5 的子串,即 "world"。
需要注意的是,substr()
函数不会修改原始字符串,而是返回一个新的字符串。如果不提供长度参数,substr()
函数将提取从指定索引到字符串末尾的所有字符。
1.6 find()
在 C++ 的标准库中,std::string
类提供了 find()
成员函数,用于在字符串中查找子串或字符,并返回找到的第一个匹配位置的索引。如果未找到,则返回一个特定的无效位置。
解释一下重载版本
size_t find (const string& str, size_t pos = 0) const;
这个重载版本接受一个std::string
对象作为参数,以及一个可选的起始搜索位置pos
。它在字符串中从指定位置开始查找给定的子串,并返回找到的第一个匹配位置的索引。
size_t find (const char* s, size_t pos = 0) const;
该版本接受一个 C 风格的字符串指针作为参数,以及一个可选的起始搜索位置pos
。它在字符串中从指定位置开始查找给定的 C 字符串,并返回找到的第一个匹配位置的索引。
size_t find (const char* s, size_t pos, size_t n) const;
这个版本接受一个 C 风格的字符串指针和一个长度参数n
,以及一个起始搜索位置pos
。它在字符串中从指定位置开始查找给定长度的字符数组,并返回找到的第一个匹配位置的索引。
size_t find (char c, size_t pos = 0) const;
最后一个版本接受一个字符c
作为参数,以及一个可选的起始搜索位置pos
。它在字符串中从指定位置开始查找给定字符,并返回找到的第一个匹配位置的索引。
以下是 find()
函数的基本用法示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
size_t found = str.find("world"); // 在字符串中查找 "world"
if (found != std::string::npos) {
std::cout << "Substring found at position: " << found << std::endl;
} else {
std::cout << "Substring not found" << std::endl;
}
return 0;
}
在上面的示例中,我们使用 find()
函数在字符串 str
中查找子串 "world"。如果找到了子串,find()
函数会返回子串的第一个字符在原字符串中的索引位置。如果未找到,它会返回一个特定的常量值 std::string::npos
。
解释一下std::string::npos
std::string::npos
是 std::string
类中的一个静态成员变量,它表示字符串中的无效位置或未找到的位置。当在字符串中执行查找操作但未找到目标子串或字符时,查找函数会返回这个特定的值作为索引。
具体来说,std::string::npos
是一个 size_t
类型的常量,通常是一个最大可能的值,用来表示无效的位置。在 C++ 标准库中,它的值是 std::numeric_limits<std::streamsize>::max()
,但在大多数情况下,你只需要将它视为一个用于表示无效位置的特殊值。
1.7 rfind()
std::string
类提供了 rfind()
成员函数,用于在字符串中从后往前查找指定的子串或字符,并返回找到的最后一个匹配位置的索引。如果未找到,它会返回特定的无效位置。
解释一下重载版本
size_t rfind (const string& str, size_t pos = npos) const;
这个重载版本接受一个std::string
对象作为参数,以及一个可选的起始搜索位置pos
。它从后往前在字符串中查找给定的子串,并返回找到的最后一个匹配位置的索引。
size_t rfind (const char* s, size_t pos = npos) const;
该版本接受一个 C 风格的字符串指针作为参数,以及一个可选的起始搜索位置pos
。它从后往前在字符串中查找给定的 C 字符串,并返回找到的最后一个匹配位置的索引。
size_t rfind (const char* s, size_t pos, size_t n) const;
这个版本接受一个 C 风格的字符串指针和一个长度参数n
,以及一个起始搜索位置pos
。它从后往前在字符串中查找给定长度的字符数组,并返回找到的最后一个匹配位置的索引。
size_t rfind (char c, size_t pos = npos) const;
最后一个版本接受一个字符c
作为参数,以及一个可选的起始搜索位置pos
。它从后往前在字符串中查找给定字符,并返回找到的最后一个匹配位置的索引。
这些 rfind()
函数的不同重载版本提供了多种方式来从后往前在 std::string
中查找指定的子串、字符或字符数组,返回找到的最后一个位置的索引,或者返回 std::string::npos
,如果未找到匹配项。
以下是 rfind()
函数的使用示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world! Hello!";
size_t found = str.rfind("Hello"); // 从后往前查找子串 "Hello"
if (found != std::string::npos) {
std::cout << "Substring found at position: " << found << std::endl;
} else {
std::cout << "Substring not found" << std::endl;
}
return 0;
}
在这个示例中,我们使用 rfind()
函数在字符串 str
中从后往前查找子串 "Hello"。如果找到了子串,rfind()
函数会返回最后一个匹配的子串的第一个字符在原字符串中的索引位置。如果未找到,它会返回一个特定的常量值 std::string::npos
。
类似于 find()
函数,rfind()
函数也有多个重载版本,可以用于查找字符、C 字符串以及指定起始位置的情况。
二、string类非成员函数
2.1 operator+()
operator+
是 C++ 中的加法运算符,可以用于执行不同类型的加法操作。对于 std::string
类型,operator+
也被重载,用于连接(拼接)两个字符串。
重载函数解释
string operator+ (const string& lhs, const string& rhs);
这个重载版本接受两个std::string
对象作为参数,并返回一个新的字符串,其中包含两个操作数的内容连接在一起。
string operator+ (const string& lhs, const char* rhs);
string operator+ (const char* lhs, const string& rhs);
这两个版本接受一个std::string
对象和一个 C 字符串指针作为参数,或者一个 C 字符串指针和一个std::string
对象作为参数。它们返回一个新的字符串,其中包含两个操作数的内容连接在一起。
string operator+ (const string& lhs, char rhs);
string operator+ (char lhs, const string& rhs);
这两个版本接受一个std::string
对象和一个字符,或者一个字符和一个std::string
对象作为参数。它们返回一个新的字符串,其中包含操作数的内容连接在一起。
以下是使用这些 operator+
运算符的示例:
#include <iostream>
#include <string>
int main() {
std::string str1 = "Hello, ";
std::string str2 = "world!";
std::string result1 = str1 + str2; // 连接两个字符串
std::string result2 = str1 + "C++"; // 连接一个字符串和一个 C 字符串
std::string result3 = "Welcome " + str2; // 连接一个 C 字符串和一个字符串
std::string result4 = str1 + '!'; // 连接一个字符串和一个字符
std::cout << "Result 1: " << result1 << std::endl;
std::cout << "Result 2: " << result2 << std::endl;
std::cout << "Result 3: " << result3 << std::endl;
std::cout << "Result 4: " << result4 << std::endl;
return 0;
}
通过使用这些重载的 operator+
运算符,可以方便地连接不同类型的字符串和字符,得到一个新的字符串。
2.2 operator<<()
operator<<
是 C++ 中的输出流运算符,通常用于将数据输出到流(如标准输出流 cout
)中。对于 std::string
类型,operator<<
也被重载,用于将字符串内容输出到流中。
以下是 std::string
类中 operator<<
运算符的使用示例:
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, world!";
std::cout << "String: " << str << std::endl;
return 0;
}
在上面的示例中,我们使用 operator<<
运算符将字符串 str
的内容输出到标准输出流 cout
中。输出结果为:
需要注意的是,operator<<
运算符用于将字符串内容输出到流中,而不是将字符串对象本身作为字符串输出。也就是说,它不会输出字符串的长度或其他信息,而只会输出字符串的内容。
2.3 operator>>()
operator>>
是 C++ 中的输入流运算符,通常用于从流(如标准输入流 cin
)中读取数据。对于 std::string
类型,operator>>
也被重载,用于从流中读取字符串数据。
以下是 std::string
类中 operator>>
运算符的使用示例:
#include <iostream>
#include <string>
int main() {
std::string str;
std::cout << "Enter a string: ";
std::cin >> str;
std::cout << "You entered: " << str << std::endl;
return 0;
}
在上面的示例中,我们使用 operator>>
运算符从标准输入流 cin
中读取用户输入的字符串,并将其存储在字符串变量 str
中。然后,我们使用 operator<<
运算符将用户输入的字符串输出到标准输出流中。
需要注意的是,operator>>
运算符默认以空格为分隔符,所以它会读取并存储用户输入的一个单词,直到遇到空格或换行符为止。如果你需要读取整行输入,可以使用 std::getline()
函数。
2.4 getline()
std::getline()
是 C++ 标准库中的一个函数,用于从输入流中读取一行文本,并将其存储到一个字符串对象中。这个函数可以读取包括空格在内的整行内容,直到遇到换行符为止。
以下是 std::getline()
函数的使用示例:
#include <iostream>
#include <string>
int main() {
std::string line;
std::cout << "Enter a line of text: ";
std::getline(std::cin, line); // 从标准输入读取一行文本
std::cout << "You entered: " << line << std::endl;
return 0;
}
需要注意的是,std::getline()
函数接受两个参数:第一个参数是输入流(如 std::cin
),第二个参数是一个字符串对象,用于存储读取的内容。它会读取整行文本,包括空格,但不会将换行符放入结果字符串中。
重载版本解释
is
: 输入流对象,如std::cin
。str
: 要存储读取内容的std::string
对象。delim
: 指定的分隔符字符。默认情况下,分隔符为换行符\n
如果你希望使用除换行符之外的其他分隔符,你可以在函数调用中提供第三个参数,例如:
在这个示例中,std::getline()
函数会读取输入流中的一行文本,直到遇到分号为止,并将读取的内容存储在字符串对象 line
中。
2.5 relational operators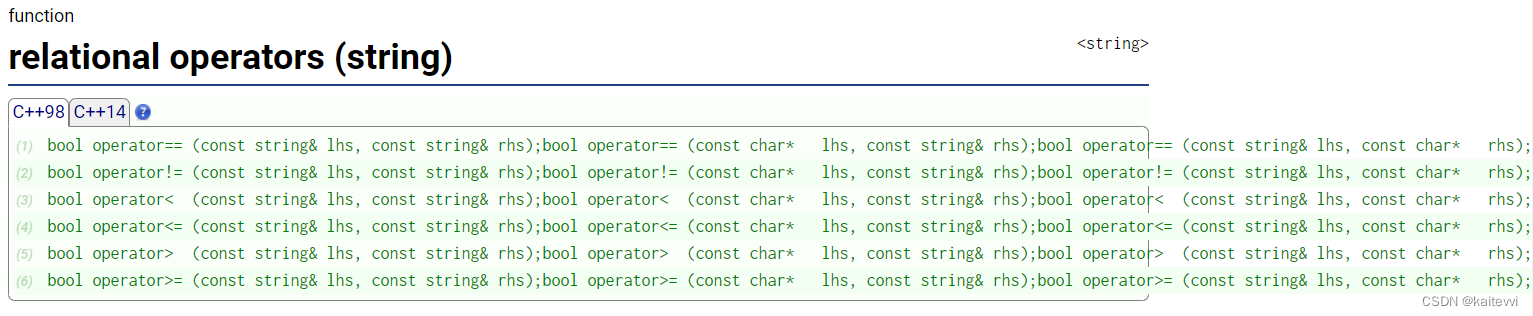
详细解释
①bool operator== (const string& lhs, const string& rhs);
bool operator== (const char* lhs, const string& rhs);
bool operator== (const string& lhs, const char* rhs);
以上三个版本的 operator==
运算符用于检查左边的字符串和右边的字符串(或C字符串)是否相等。
②bool operator!= (const string& lhs, const string& rhs);
bool operator!= (const char* lhs, const string& rhs);
bool operator!= (const string& lhs, const char* rhs);
以上三个版本的 operator!=
运算符用于检查左边的字符串和右边的字符串(或C字符串)是否不相等。
③bool operator< (const string& lhs, const string& rhs);
bool operator< (const char* lhs, const string& rhs);
bool operator< (const string& lhs, const char* rhs);
以上三个版本的 operator<
运算符用于检查左边的字符串是否小于右边的字符串(或C字符串)。
④bool operator<= (const string& lhs, const string& rhs);
bool operator<= (const char* lhs, const string& rhs);
bool operator<= (const string& lhs, const char* rhs);
以上三个版本的 operator<=
运算符用于检查左边的字符串是否小于等于右边的字符串(或C字符串)。
⑤bool operator> (const string& lhs, const string& rhs);
bool operator> (const char* lhs, const string& rhs);
bool operator> (const string& lhs, const char* rhs);
以上三个版本的 operator>
运算符用于检查左边的字符串是否大于右边的字符串(或C字符串)。
⑥bool operator>= (const string& lhs, const string& rhs);
bool operator>= (const char* lhs, const string& rhs);
bool operator>= (const string& lhs, const char* rhs);
以上三个版本的 operator>=
运算符用于检查左边的字符串是否大于等于右边的字符串(或C字符串)。
结语
终于,我们来到了string详解系列的终点。通过这一系列的内容,相信你已经对C++中的string类有了更深入的了解。从基本用法到各种成员函数,我们探索了string类在处理字符串时的强大功能。🎉💡
但是故事并没有结束,更多精彩内容还在后头!接下来,我将为大家呈现模拟实现string以及深入解析string底层原理的文章。通过这些内容,你将更加深入地理解string的背后机制,为你的C++编程之路增添新的见解。🔍🛠️
所以,请继续关注,敬请期待即将到来的内容!如果在写作过程中有任何疑问或需要帮助,欢迎随时向我提问。让我们一同探索C++字符串的奥秘,创作出精彩的学习资源吧!🚀📚