目录
1.MyBatis 是什么?
MyBatis官网:mybatis – MyBatis 3 | 简介
2.为什么要学习 MyBatis?
3.怎么学MyBatis?
4.第⼀个MyBatis查询
4.1 创建数据库和表
4.2 添加MyBatis框架⽀持
4.2.1 ⽼项⽬添加MyBatis
4.2.2 新项⽬添加MyBatis
4.3 配置连接字符串和MyBatis
4.3.1 配置连接字符串
4.3.2 配置 MyBatis 中的 XML 路径
4.4 添加业务代码
4.4.1 添加实体类
4.4.2 添加 mapper 接⼝
4.4.3 添加UserMapper.xml
4.4.4 添加 Service
4.4.5 添加 Controller
5.增、删、改操作
5.1 增加用户操作
5.2 修改用户操作
5.3 删除用户操作
6.查询操作
6.1 单表查询
6.1.1 参数占位符 #{} 和 ${}
SQL 注入问题
6.1.2 ${} 优点
6.1.3 SQL 注入问题
6.1.4 like 查询
1.MyBatis 是什么?
MyBatis官网:mybatis – MyBatis 3 | 简介
2.为什么要学习 MyBatis?
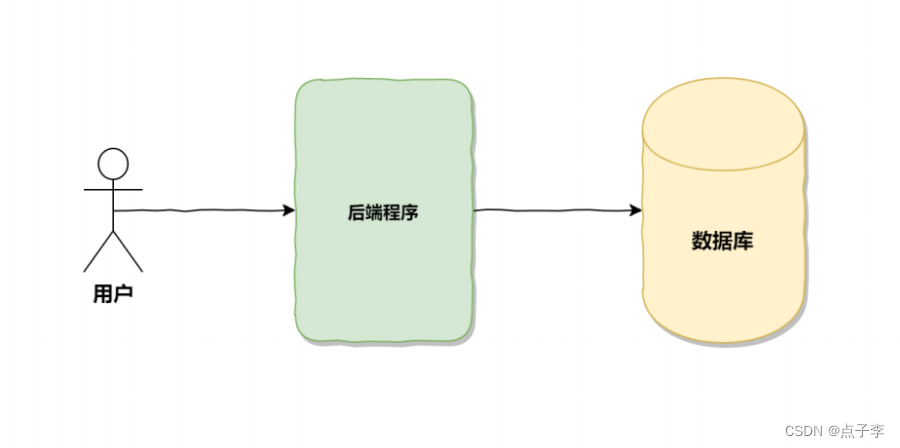
- 1. 创建数据库连接池 DataSource
- 2. 通过 DataSource 获取数据库连接 Connection
- 3. 编写要执⾏带 ? 占位符的 SQL 语句
- 4. 通过 Connection 及 SQL 创建操作命令对象 Statement
- 5. 替换占位符:指定要替换的数据库字段类型,占位符索引及要替换的值
- 6. 使⽤ Statement 执⾏ SQL 语句
- 7. 查询操作:返回结果集 ResultSet,更新操作:返回更新的数量
- 8. 处理结果集
- 9. 释放资源
JDBC 操作示例回顾
-- 创建数据库
create database if not exists `library` default character set utf8mb4;
-- 使⽤数据库
use library;
-- 创建表
create table if not exists `soft_bookrack` (
`book_name` varchar(32) NOT NULL,
`book_author` varchar(32) NOT NULL,
`book_isbn` varchar(32) NOT NULL primary key
) ;
以下是JDBC操作的具体实现代码
import javax.sql.DataSource;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
public class SimpleJdbcOperation {
private final DataSource dataSource;
public SimpleJdbcOperation(DataSource dataSource) {
this.dataSource = dataSource;
}
/**
* 添加⼀本书
*/
public void addBook() {
Connection connection = null;
PreparedStatement stmt = null;
try {
//获取数据库连接
connection = dataSource.getConnection();
//创建语句
stmt = connection.prepareStatement(
"insert into soft_bookrack (book_name, book_author, b
ook_isbn) values (?,?,?);"
);
//参数绑定
stmt.setString(1, "Spring in Action");
stmt.setString(2, "Craig Walls");
stmt.setString(3, "9787115417305");
//执⾏语句
stmt.execute();
} catch (SQLException e) {
//处理异常信息
} finally {
//清理资源
try {
if (stmt != null) {
stmt.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
//
}
}
}
/**
* 更新⼀本书
*/
public void updateBook() {
Connection connection = null;
PreparedStatement stmt = null;
try {
//获取数据库连接
connection = dataSource.getConnection();
//创建语句
stmt = connection.prepareStatement(
"update soft_bookrack set book_author=? where book_is
bn=?;"
);
//参数绑定
stmt.setString(1, "张卫滨");
stmt.setString(2, "9787115417305");
//执⾏语句
stmt.execute();
} catch (SQLException e) {
//处理异常信息
} finally {
//清理资源
try {
if (stmt != null) {
stmt.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
//
}
}
}
/**
* 查询⼀本书
*/
public void queryBook() {
Connection connection = null;
PreparedStatement stmt = null;
ResultSet rs = null;
Book book = null;
try {
//获取数据库连接
connection = dataSource.getConnection();
//创建语句
stmt = connection.prepareStatement(
"select book_name, book_author, book_isbn from soft_b
ookrack where book_isbn =?"
);
//参数绑定
stmt.setString(1, "9787115417305");
//执⾏语句
rs = stmt.executeQuery();
if (rs.next()) {
book = new Book();
book.setName(rs.getString("book_name"));
book.setAuthor(rs.getString("book_author"));
book.setIsbn(rs.getString("book_isbn"));
}
System.out.println(book);
} catch (SQLException e) {
//处理异常信息
} finally {
//清理资源
try {
if (rs != null) {
rs.close();
}
if (stmt != null) {
stmt.close();
}
if (connection != null) {
connection.close();
}
} catch (SQLException e) {
//
}
}
}
public static class Book {
private String name;
private String author;
private String isbn;
//省略 setter getter ⽅法
}
}
3.怎么学MyBatis?
4.第⼀个MyBatis查询
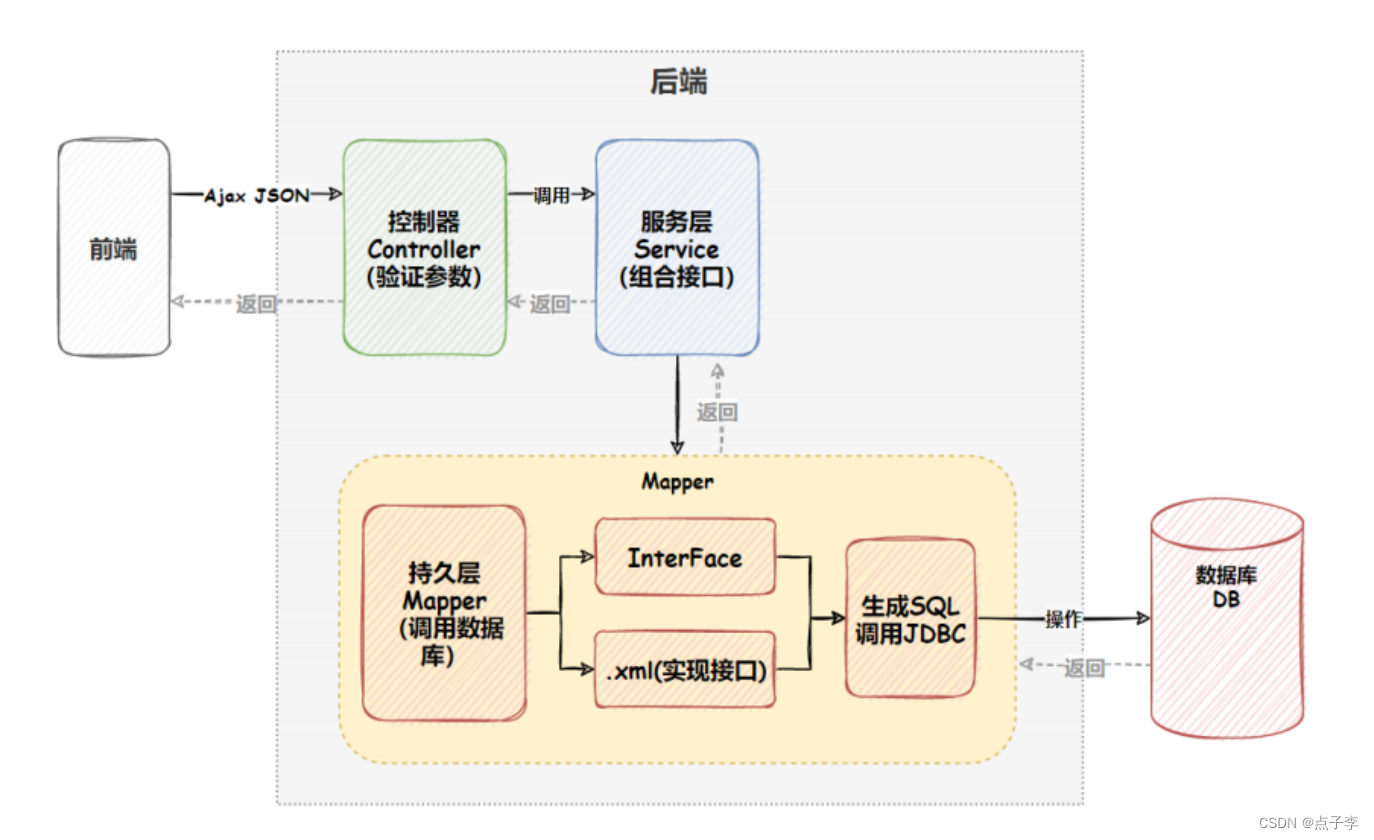
1. 将输⼊数据(即传⼊对象)+SQL 映射成原⽣ SQL2. 将结果集映射为返回对象,即输出对象
ORM 把数据库映射为对象:
4.1 创建数据库和表
-- 创建数据库
drop database if exists mycnblog;
create database mycnblog DEFAULT CHARACTER SET utf8mb4;
-- 使⽤数据数据
use mycnblog;
-- 创建表[⽤户表]
drop table if exists userinfo;
create table userinfo(
id int primary key auto_increment,
username varchar(100) not null,
password varchar(32) not null,
photo varchar(500) default '',
createtime datetime default now(),
updatetime datetime default now(),
`state` int default 1
) default charset 'utf8mb4';
-- 创建⽂章表
drop table if exists articleinfo;
create table articleinfo(
id int primary key auto_increment,
title varchar(100) not null,
content text not null,
createtime datetime default now(),
updatetime datetime default now(),
uid int not null,
rcount int not null default 1,
`state` int default 1
)default charset 'utf8mb4';
-- 创建视频表
drop table if exists videoinfo;
create table videoinfo(
vid int primary key,
`title` varchar(250),
`url` varchar(1000),
createtime datetime default now(),
updatetime datetime default now(),
uid int
)default charset 'utf8mb4';
-- 添加⼀个⽤户信息
INSERT INTO `mycnblog`.`userinfo` (`id`, `username`, `password`, `photo`,
`createtime`, `updatetime`, `state`) VALUES
(1, 'admin', 'admin', '', '2021-12-06 17:10:48', '2021-12-06 17:10:48', 1)
;
-- ⽂章添加测试数据
insert into articleinfo(title,content,uid)
values('Java','Java正⽂',1);
-- 添加视频
insert into videoinfo(vid,title,url,uid) values(1,'java title','http://ww
w.baidu.com',1);
4.2 添加MyBatis框架⽀持
4.2.1 ⽼项⽬添加MyBatis
<!-- 添加 MyBatis 框架 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.1.4</version>
</dependency>
<!-- 添加 MySQL 驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.38</version>
<scope>runtime</scope>
</dependency>
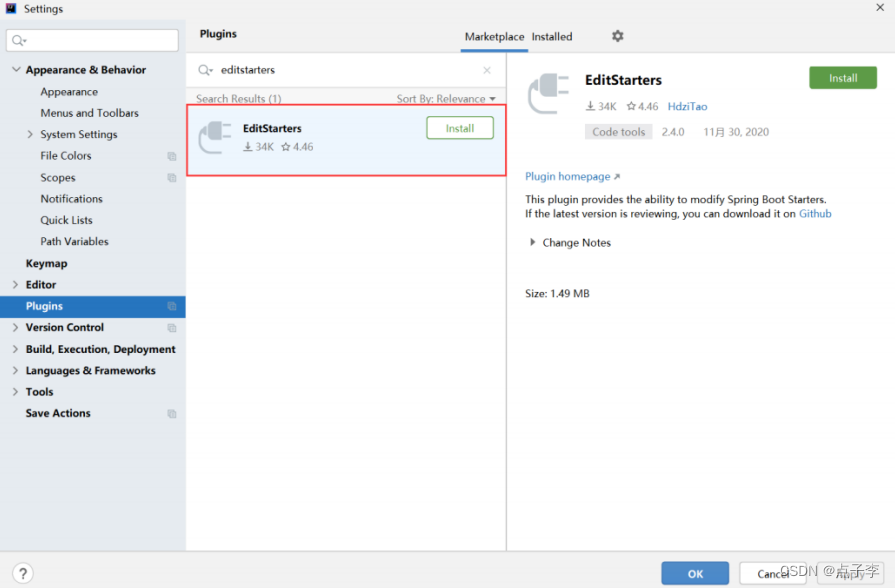
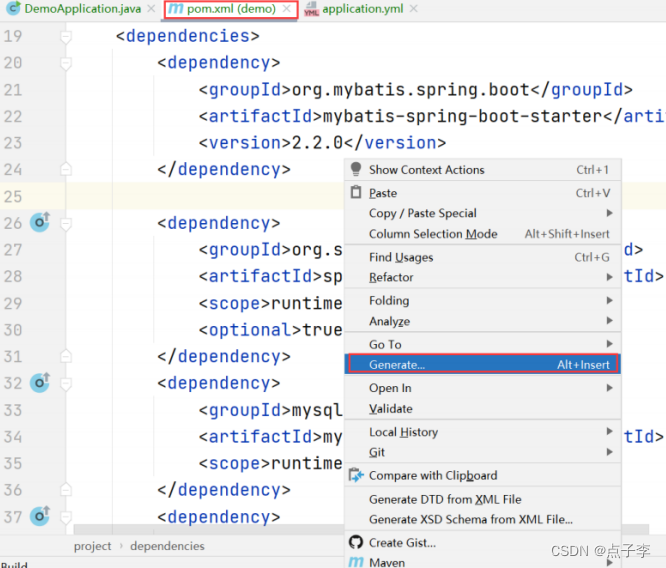
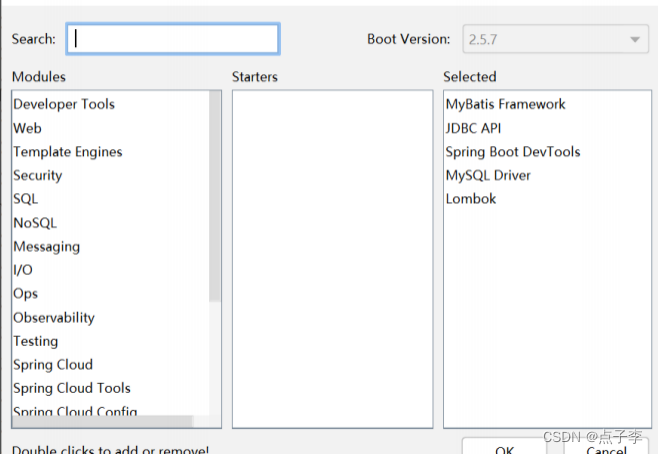
4.2.2 新项⽬添加MyBatis
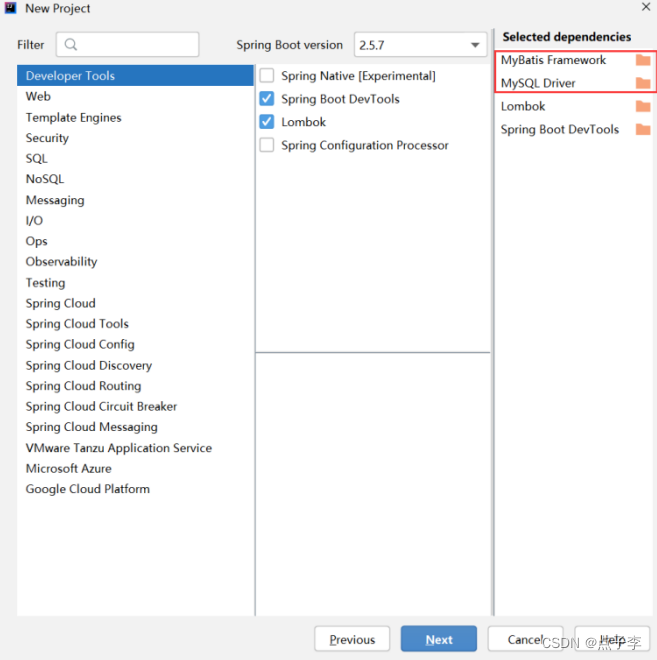
4.3 配置连接字符串和MyBatis
4.3.1 配置连接字符串
如果是application.yml添加如下内容
# 数据库连接配置
spring:
datasource:
url: jdbc:mysql://localhost:3306/mycnblog?characterEncoding=utf8&useSSL
=false
username: root
password: 123456
driver-class-name: com.mysql.cj.jdbc.Driver
如果使⽤ mysql-connector-java 是 5.x 之前的使⽤的是“ com.mysql.jdbc.Driver ” ,如果是⼤于 5.x 使⽤的是“ com.mysql.cj.jdbc.Driver ” 。
4.3.2 配置 MyBatis 中的 XML 路径
# 配置 mybatis xml 的⽂件路径,在 resources/mapper 创建所有表的 xml ⽂件
mybatis:
mapper-locations: classpath:mapper/**Mapper.xml
4.4 添加业务代码
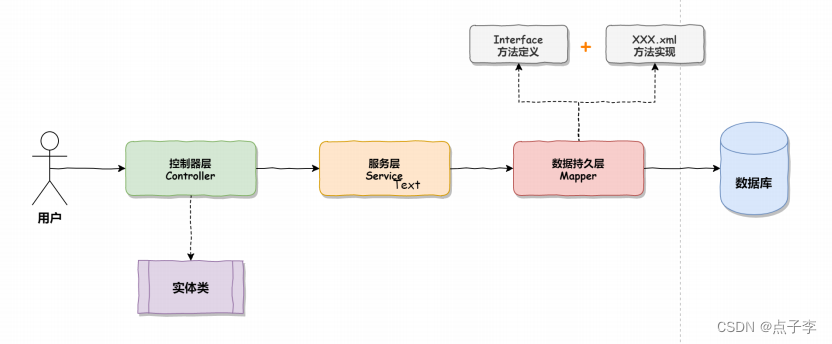
4.4.1 添加实体类
先添加用户的实体类:
import lombok.Data;
import java.util.Date;
@Data
public class User {
private Integer id;
private String username;
private String password;
private String photo;
private Date createTime;
private Date updateTime;
}
4.4.2 添加 mapper 接⼝
import org.apache.ibatis.annotations.Mapper;
import java.util.List;
@Mapper
public interface UserMapper {
public List<User> getAll();
}
此处引用ibatis和mybatis的区别博客http://t.csdn.cn/oVVj5
4.4.3 添加UserMapper.xml
数据持久层的实现,mybatis的固定xml格式:
<?xml version = "1.0" encoding = "UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybati
s.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
</mapper>
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybati
s.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.demo.mapper.UserMapper">
<select id="getAll" resultType="com.example.demo.model.User">
select * from userinfo
</select>
</mapper>
4.4.4 添加 Service
import org.springframework.stereotype.Service;
import javax.annotation.Resource;
import java.util.List;
@Service
public class UserService {
@Resource
private UserMapper userMapper;
public List<User> getAll() {
return userMapper.getAll();
}
}
4.4.5 添加 Controller
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.annotation.Resource;
import java.util.List;
@RestController
@RequestMapping("/u")
public class UserController {
@Resource
private UserService userService;
@RequestMapping("/getall")
public List<User> getAll(){
return userService.getAll();
}
}
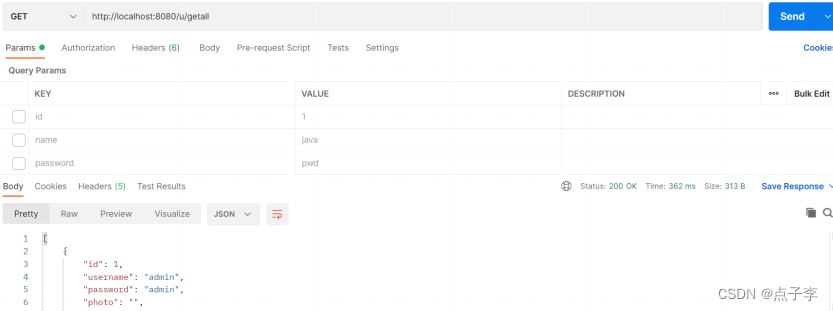
5.增、删、改操作
- <insert>标签:插⼊语句
- <update>标签:修改语句
- <delete>标签:删除语句
5.1 增加用户操作
controller 实现代码:
@RequestMapping(value = "/add",method = RequestMethod.POST)
public Integer add(@RequestBody User user){
return userService.getAdd(user);
}
Integer add(User user);
<insert id="add">
insert into userinfo(username,password,photo,state)
values(#{username},#{password},#{photo},1)
</insert>
Postman添加访问:
{"username":"mysql","password":"mysql","photo":"img.png"}
@RequestMapping(value = "/add2", method = RequestMethod.POST)
public Integer add2(@RequestBody User user) {
userService.getAdd2(user);
return user.getId();
}
@Mapper
public interface UserMapper {
// 添加,返回⾃增id
void add2(User user);
}
<!-- 返回⾃增id -->
<insert id="add2" useGeneratedKeys="true" keyProperty="id">
insert into userinfo(username,password,photo,state)
values(#{username},#{password},#{photo},1)
</insert>
- useGeneratedKeys:这会令 MyBatis 使⽤ JDBC 的 getGeneratedKeys ⽅法来取出由数据 库内部⽣成的主键(⽐如:像 MySQL 和 SQL Server 这样的关系型数据库管理系统的⾃动 递增字段),默认值:false。
- keyColumn:设置⽣成键值在表中的列名,在某些数据库(像 PostgreSQL)中,当主键列 不是表中的第⼀列的时候,是必须设置的。如果⽣成列不⽌⼀个,可以⽤逗号分隔多个属性 名称。
- keyProperty:指定能够唯⼀识别对象的属性,MyBatis 会使⽤ getGeneratedKeys 的返回 值或 insert 语句的 selectKey ⼦元素设置它的值,默认值:未设置(unset)。如果⽣成列 不⽌⼀个,可以⽤逗号分隔多个属性名称。
postman 返回结果:
5.2 修改用户操作
controller:
/** 修改操作
*
* @param id
* @param name
* @return
*/
@RequestMapping("/update")
public Integer update(Integer id, String name) {
return userService.update(id, name);
}
<update id="update">
update userinfo set username=#{name} where id=#{id}
</update>
5.3 删除用户操作
<delete id="delById" parameterType="java.lang.Integer">
delete from userinfo where id=#{id}
</delete>
6.查询操作
6.1 单表查询
@RequestMapping("/getuser")
public User getUserById(Integer id) {
return userService.getUserById(id);
}
<select id="getUserById" resultType="com.example.demo.model.User">
select * from userinfo where id=#{id}
</select>
6.1.1 参数占位符 #{} 和 ${}
- #{}:预编译处理。
- ${}:字符直接替换。
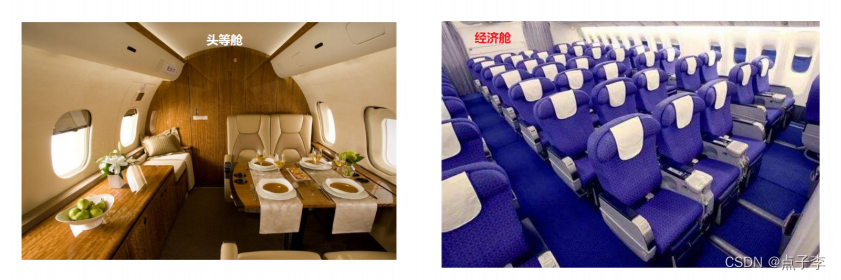
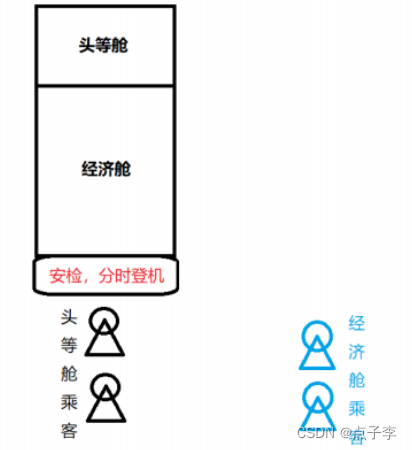
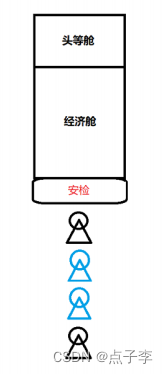
SQL 注入问题
6.1.2 ${} 优点
<select id="getAllBySort" parameterType="java.lang.String" resultType="com.
example.demo.model.User">
select * from userinfo order by id ${sort}
</select>
6.1.3 SQL 注入问题
<select id="isLogin" resultType="com.example.demo.model.User">
select * from userinfo where username='${name}' and password='${pwd}'
</select>
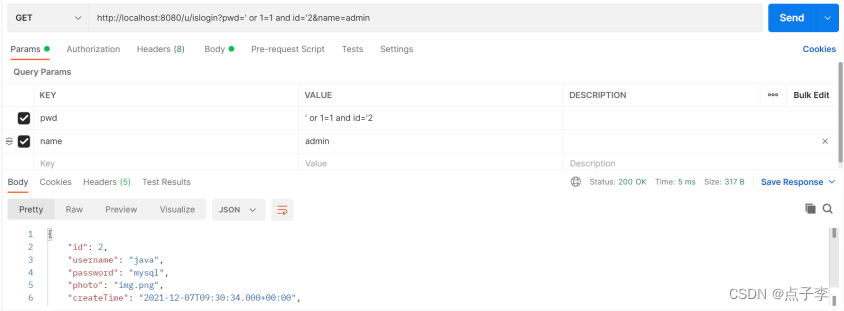
结论:⽤于查询的字段,尽量使⽤ #{} 预查询的⽅式。
6.1.4 like 查询
<select id="findUserByName2" resultType="com.example.demo.model.User">
select * from userinfo where username like '%#{username}%';
</select>
<select id="findUserByName3" resultType="com.example.demo.model.User">
select * from userinfo where username like concat('%',#{usernam
e},'%');
</select>