文章目录
- ⭐前言
- ⭐python re库
- 💖 re.match函数
- 💖 re.search函数
- 💖 re.compile 函数
- ⭐正则获取天气预报
- 💖 正则实现页面内容提取
- 💖 echarts的天气折现图
- ⭐结束
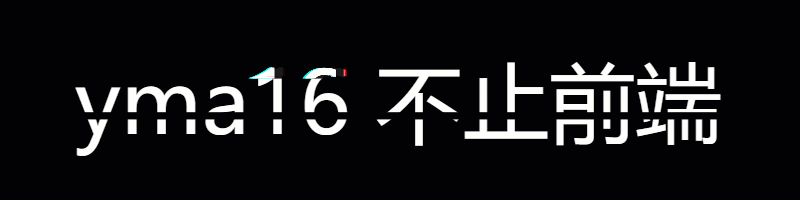
⭐前言
大家好,我是yma16,本文分享python的正则表达式并可视化展示抓取的天气预报数据。
该系列文章:
python爬虫_基本数据类型
python爬虫_函数的使用
python爬虫_requests的使用
python爬虫_selenuim可视化质量分
python爬虫_django+vue3可视化csdn用户质量分
⭐python re库
正则表达式(Regular expressions,也叫 REs、 regexs 或 regex patterns),是一个特殊的字符序列。
Python 自1.5版本起增加了re 模块,它提供 Perl 风格的正则表达式模式。
re 模块使 Python 语言拥有全部的正则表达式功能。
compile 函数根据一个模式字符串和可选的标志参数生成一个正则表达式对象。该对象拥有一系列方法用于正则表达式匹配和替换。
re 模块也提供了与这些方法功能完全一致的函数,这些函数使用一个模式字符串做为它们的第一个参数。
💖 re.match函数
re.match 尝试从字符串的起始位置匹配一个模式,如果不是起始位置匹配成功的话,match() 就返回 none。
函数语法:
re.match(pattern, string, flags=0)
参数 | 描述 |
---|---|
pattern | 匹配的正则表达式 |
string | 要匹配的字符串。 |
flags | 标志位,用于控制正则表达式的匹配方式,如:是否区分大小写,多行匹配等等。参见:正则表达式修饰符 - 可选标志 |
匹配成功 re.match 方法返回一个匹配的对象,否则返回 None。
import re
print(re.match('yma16', 'yma16 in csdn'))
print(re.match('yma16', 'yma16 in csdn').span())
print(re.match('csdn', 'yma16 in csdn'))
运行结果入下:
$ <re.Match object; span=(0, 5), match='yma16'>
$ (0, 5)
$ None
使用 group(num) 或 groups() 匹配对象函数来获取匹配表达式。
import re
line = "yma16 is a csdn player"
matchObj = re.match(r'(.*) is (.*?) .*', line, re.M | re.I)
if matchObj:
print("matchObj.group() : ", matchObj.group())
print("matchObj.group(1) : ", matchObj.group(1))
print("matchObj.group(2) : ", matchObj.group(2))
else:
print("No match!!")
运行结果:
matchObj.group() : yma16 is a csdn player
matchObj.group(1) : yma16
matchObj.group(2) : a
💖 re.search函数
re.search 扫描整个字符串并返回第一个成功的匹配。
re.search(pattern, string, flags=0)
示例:
import re
print(re.search('yma16', 'yma16 in csdn'))
print(re.search('yma16', 'yma16 in csdn').span())
print(re.search('csdn', 'yma16 in csdn'))
运行结果:
<re.Match object; span=(0, 5), match=‘yma16’>
(0, 5)
<re.Match object; span=(9, 13), match=‘csdn’>
re.match与re.search的区别
re.match只匹配字符串的开始,如果字符串开始不符合正则表达式,则匹配失败,函数返回None;而re.search匹配整个字符串,直到找到一个匹配。
💖 re.compile 函数
compile 函数用于编译正则表达式,生成一个正则表达式( Pattern )对象,供 match() 和 search() 这两个函数使用。
语法格式为:
re.compile(pattern[, flags])
示例:
import re
pattern = re.compile(r'([a-z]+) ([a-z]+)', re.I) # re.I 表示忽略大小写
m = pattern.match('csdn hello 2023')
print(m)
if m:
print(m.group(0))
运行结果如下:
<re.Match object; span=(0, 10), match=‘csdn hello’>
csdn hello
⭐正则获取天气预报
天气预报的网站:http://www.weather.com.cn/html/weather/101280601.shtml
深圳的天气预报前缀101280601
💖 正则实现页面内容提取
页面元素分析:
- wea 天气
- tem 温度
- win 风向
python获取代码如下:
import re
from urllib import request
def urllib_request():
url = 'http://www.weather.com.cn/html/weather/101280601.shtml'
req = request.Request(url)
resp = request.urlopen(req)
htmlText = resp.read().decode('utf-8')
return complie_Work(htmlText)
def complie_Work(htmlText):# 正则表达式处理html文本
findStyle = re.compile(r'<ul class="t clearfix">(.*?)</ul>', re.S) # re.S忽略换行(天气的那一行)
styles = re.findall(findStyle, htmlText) # 天气
styles = re.sub('<br(\s+)?/>(\s+)?', '', styles[0])
findDay = re.compile(r"<h1>(.*?)</h1>", re.S)
day = re.findall(findDay, styles) # 日期
findDiscribe=re.compile(r'<p title="(.*?)" class="wea">',re.S)
discribe=re.findall(findDiscribe,styles)#天气描述
findTem = re.compile(r"<span>(.*?)</span>(.*?)<i>(.*?)</i>", re.S)
tem = re.findall(findTem, styles)
length=len(tem)
title_data=day
temhigh_data=[]
temlow_data=[]
findEm = re.compile(r"<em>\s<span title=\"(.*?)\"", re.S)
em = re.findall(findEm, styles)#风向
length = len(day)
findWindlevel = re.compile(r"</em>\s<i><(.*?)</i>\s</p>", re.S)
wL = re.findall(findWindlevel, styles)#风的等级
if length<7:
# 获取晚上的温度
findtoday=re.compile(r"<p class=\"tem\">\n<i>(.*?)</i>",re.S)
today=re.findall(findtoday,styles)
s=today[0]
t=re.findall(r"\d+\.?\d*", s)
temhigh_data.append(t[0])
temlow_data.append(t[0])
else:
print('morning')
for i in tem:
temhigh_data.append(re.findall(r"\d+\.?\d*", i[0])[0])
temlow_data.append(re.findall(r"\d+\.?\d*", i[2])[0])
data={
'title':title_data,
'high':temhigh_data,
'low':temlow_data,
'discribe':discribe,
'windem':em,
'windwl': wL,
}
return data
print(urllib_request())
运行成功如下图所示:
inscode链接如下:
💖 echarts的天气折现图
html引入vue2的cdn渲染echarts
代码如下:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>echarts 滚动事件</title>
<!-- vue2 生产环境版本,优化了尺寸和速度 -->
<script src="https://cdn.jsdelivr.net/npm/vue@2"></script>
<script src="./echarts.js"></script>
</head>
<style>
#app {
position: absolute;
height: 100vh;
width: 100vw;
}
</style>
<body>
<div id="app">
<div>
<div id="second" style="width: 900px;height:400px;"></div>
</div>
</div>
<script type="text/javascript">
const weatherData={'title': ['4日(今天)', '5日(明天)', '6日(后天)', '7日(周五)', '8日(周六)', '9日(周日)', '10日(周一)'], 'high': ['32', '33', '33', '33', '33', '33'], 'low': ['27', '27', '28', '28', '28', '27'], 'discribe': ['多云', '阵雨转多云', '多云', '多云', '多云', '多云', '多云'], 'windem': ['无持续风向', '无持续风向', '西南风', '西南风', '无持续风向', '无持续风向', '西南风'], 'windwl': ['3级', '3级转3-4级', '3级', '3级转3-4级']}
const instanceVue = {
el: '#app',
name: 'ecahrts',
data() {
return {
secondChart: null,
maxNum:100,
};
},
mounted() {
this.initSecondData()
},
methods: {
initSecondData() {
// 基于准备好的dom,初始化echarts实例
const myChart = echarts.init(document.getElementById('second'));
const option = {
title: {
text: 'line'
},
tooltip: {
trigger: 'axis'
},
legend: {},
toolbox: {
show: true,
feature: {
dataZoom: {
yAxisIndex: 'none'
},
dataView: {
readOnly: false
},
magicType: {
type: ['line', 'bar']
},
restore: {},
saveAsImage: {}
}
},
xAxis: {
type: 'category',
boundaryGap: false,
data: weatherData.title
},
yAxis: {
type: 'value',
axisLabel: {
formatter: '{value} °C'
}
},
series: [{
name: 'Highest',
type: 'line',
data: weatherData.high,
markPoint: {
data: [{
type: 'max',
name: 'Max'
},
{
type: 'min',
name: 'Min'
}
]
},
markLine: {
data: [{
type: 'average',
name: 'Avg'
}]
}
},
{
name: 'Lowest',
type: 'line',
data: weatherData.low,
markPoint: {
data: [{
name: '周最低',
value: -2,
xAxis: 1,
yAxis: -1.5
}]
},
markLine: {
data: [{
type: 'average',
name: 'Avg'
},{
type: 'max',
name: 'Max'
},
{
type: 'min',
name: 'Min'
}
]
}
}
]
};
myChart.setOption(option);
this.secondChart = myChart;
}
}
}
// 实例化
new Vue(instanceVue);
</script>
</body>
</html>
运行结果如下:
inscode如下:
⭐结束
本文分享到这结束,如有错误或者不足之处欢迎指出!
👍 点赞,是我创作的动力!
⭐️ 收藏,是我努力的方向!
✏️ 评论,是我进步的财富!
💖 感谢你的阅读!