文章目录
- 1.迭代器(正向遍历)
- begin有两个版本
- 2.反向迭代器(反向遍历)
- rbegin由两个版本
- 3. at
- 4. insert ——头插
- 在pos位置前插入一个字符串
- 在pos位置前插入n个字符
- 在迭代器前插入一个字符
- 5. erase
- 从pos位置开始删除len个字符
- 从迭代器位置开始删除
- 6. replace——替换
- 从pos位置开始的n个字符替换成想要的字符
- 7. find ——查找
- 例题 替换空格
- 8. swap ——交换
- 9. c_str
- 10. substr
- 11. getline
1.迭代器(正向遍历)
#include<iostream>
#include<string>
using namespace std;
//迭代器
int main()
{
string s("hello world");
string::iterator it = s.begin();//遍历字符串
while (it != s.end())
{
cout << *it << " ";
it++;
}
return 0;
}
- s.begin()返回指向第一个位置的指针,s.end()返回指向最后一个位置的下一个的指针
begin有两个版本
#include<iostream>
#include<string>
using namespace std;
void func(const string& s1)
{
string::iterator it = s1.begin();//会报错
while (it != s1.end())
{
cout << *it << endl;
it++;
}
}
int main()
{
string s("hello world");
func(s);
return 0;
}
当我们想要在一个函数中实现迭代器,发现会报错是因为begin一共有两个版本
- 当函数的参数为const时,需要返回const的迭代器
#include<iostream>
#include<string>
using namespace std;
void func(const string& s1)
{
string::const_iterator it = s1.begin();//会报错
while (it != s1.end())
{
//*it = 2; //由于it可以看作指针,由const修饰后,it指向的内容不能被修改
cout << *it << endl;
it++;
}
}
int main()
{
string s("hello world");
func(s);
return 0;
}
- it可以看作指针,由const修饰后,it指向的内容不能被修改
- 只能遍历和读容器的数据,不能写
2.反向迭代器(反向遍历)
#include<iostream>
#include<string>
using namespace std;
int main()
{
//F反向迭代器
string s("hello world");
string::reverse_iterator rit = s.rbegin();
while (rit != s.rend())
{
cout << *rit <<" "; //d l r o w o l l e h
rit++;
}
return 0;
}
- s.rbegin()返回指向最后一个位置的指针,s.rend()返回指向第一个位置前一个的指针
rbegin由两个版本
- 同样反向迭代器的rbegin也存在两个版本,一个不用const和一个用const修饰的
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("hello");
string::const_reverse_iterator rit = s.rbegin();
while (rit != s.rend())
{
//*rit = 2; 会报错
cout << *rit << endl;
*rit++;
}
return 0;
}
- rit可以看作指针,由const修饰后,rit指向的内容不能被修改
- 与it的const版本特性一致,只能遍历和读容器的数据,不能写
3. at
- 返回pos位置的字符
- 如果访问越界,opertaor[ ]会直接报错
- 访问越界,at会抛异常
4. insert ——头插
在pos位置前插入一个字符串
- string& insert (size_t pos, const string& str);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("world");
s.insert(0, "hello");//0位置前插入字符串
cout << s << endl;//helloworld
return 0;
}
在pos位置前插入n个字符
- string& insert (size_t pos, size_t n, char c);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("world");
s.insert(0,1,'!');//pos位置插入1个字符!
cout << s << endl;
return 0;
}
在迭代器前插入一个字符
- iterator insert (iterator p, char c);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("world1");
s.insert(s.begin()+5, '!');
cout << s << endl;//world!1
return 0;
}
s.begin()代表开头的位置,s.begin()+5代表1的位置,在1之前插入字符!
5. erase
从pos位置开始删除len个字符
- string& erase (size_t pos = 0, size_t len = npos);
npos代表缺省值,即整数最大值
若len长度大于字符pos位置后的长度或者不给len值自动使用npos, 则pos位置之后全部删除
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("hello world");
s.erase(0, 5);
s.erase(2);//2位置后全部删除
cout << s << endl;// world
}
- 0位置开始,删除5个字符
从迭代器位置开始删除
- iterator erase (iterator p);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("hello world");
s.erase(s.begin()+1);
cout << s << endl;//hllo world
}
从迭代器位置开始删除一个字符
6. replace——替换
从pos位置开始的n个字符替换成想要的字符
- string& replace (size_t pos, size_t len, const char* s);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("hello world");
s.replace(5, 1, "%%d");
cout << s << endl;
}
从下标为5的位置开始的1个字符 替换成%%d
7. find ——查找
- size_t find (char c, size_t pos = 0) const;
- 查找字符,找到了返回当前pos位置的下标,没有找到就返回npos(整形最大值)
例题 替换空格
将空格替换成%20
#include<iostream>
#include<string>
using namespace std;
//替换空格问题
int main()
{
string s("i love you");
int i = 0;
int sum = 0;
for (i = 0; i < s.size(); i++)
{
sum++;
}
s.reserve(s.size() + 2 * sum);//提前开空间,避免replace扩容
size_t pos = s.find(' ');
while (pos != string::npos)
{
s.replace(pos, 1, "%20");
pos = s.find(' ', pos + 3);//跳过上一次已经替换过的空格
}
cout << s << endl;
return 0;
}
- 通过使用reserve提前扩容,以及跳过上一次已经替换的空格进行效率提升
8. swap ——交换
- void swap (string& str);
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s1("abc");
string s2("wer");
s1.swap(s2);//string类中swap
cout << s1 << endl;
cout << s2 << endl;
swap(s1, s2);//类模板中的swap
cout << s1 << endl;
cout << s2 << endl;
return 0;
}
string类中swap与类模板中的swap功能相同,
但string类的swap只能针对string完成交换,而类模板的swap,可以对任意类型完成交换
string类的swap更高效一点,直接修改两者指针的指向
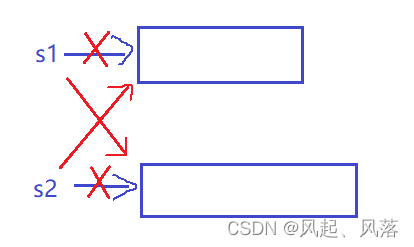
- 修改s1指针指向原来s2指向的空间,修改s2指针指向原来s1指针指向的空间
9. c_str
- const char*类型的指针
#include<iostream>
#include<string>
using namespace std;
int main()
{
string s("hello world");
cout << s << endl;//自定义类型 运算符重载<<
cout << s.c_str() << endl;//返回一个const char*指针,按照字符串打印 遇见'\0'结束
return 0;
}
10. substr
- 从pos位置取len个字符的子串
- string substr (size_t pos = 0, size_t len = npos) const;
- 从后往前查找字符
#include<iostream>
#include<string>
using namespace std;
int main()
{
string file("test.zip.c");
size_t pos = file.rfind('.');//倒着查找字符.
if (pos != string::npos)
{
string suffix = file.substr(pos);
cout << suffix << endl;
}
return 0;
}
有可能存在多个.存在,所以从后往前找后缀名
11. getline
- 用来解决cin遇见空格停止的情况
- 流提取默认使用空格/换行是多个值之间的分隔符 getline遇到换行结束
- stream& getline (istream& is, string& str);
#include<iostream>
#include<string>
using namespace std;
int main()
{
//cin>>s//输入 hello world 错误写法
getline(cin,s);//正确写法
return 0;
}
若输入hello world,cin会当成两个值,若想把hello world当成一个整体使用getline