目录
牛客.数字游戏编辑
牛客.体操队形(暴力搜索)
牛客.二叉树最大路径和编辑
牛客.排序子序列
牛客.数字游戏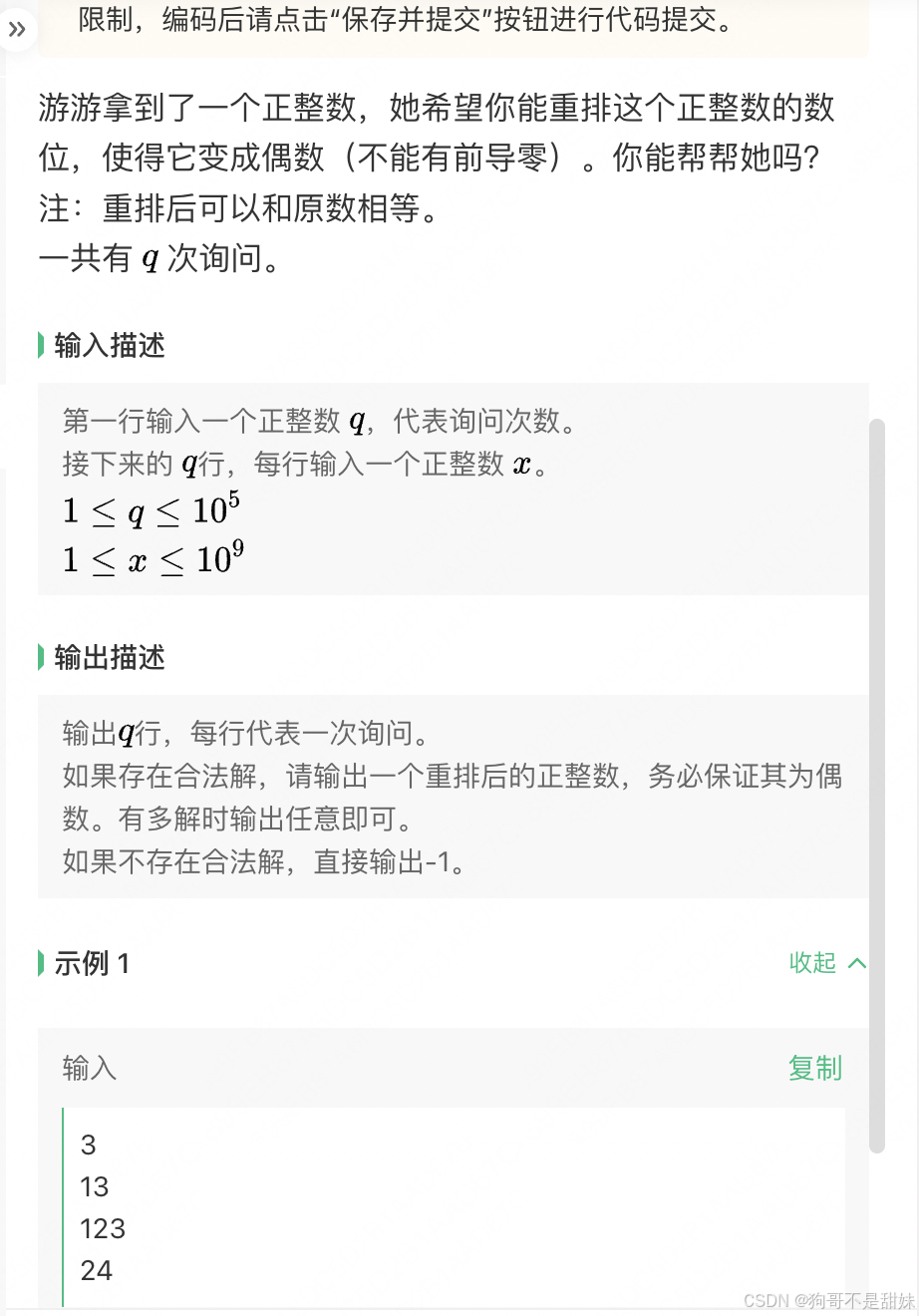
难度不大,但是要注意,他这个快速输入与输出
import java.util.*;
import java.io.*;
import java.util.StringTokenizer;
// 注意类名必须为 Main, 不要有任何 package xxx 信息
public class Main {
public static PrintWriter out=new PrintWriter(new BufferedWriter(new OutputStreamWriter(System.out)));
public static Read in=new Read();
public static void main(String[] args) throws IOException {
int q = in.nextInt();
int k = 0;
int[] ret = new int[q];
for (int i = 0; i < q; i++) {
String a = in.next();
k = Integer.parseInt(a);
if (k % 2 != 0) {
char[] b = a.toCharArray();
int p = 0;
for (int j = 0; j < b.length; j++) {
if (b[j] != 0 && b[j] % 2 == 0) {
char t = b[j];
b[j] = b[b.length - 1];
b[b.length - 1] = t;
p = 1;
break;
}
}
if (p == 1) {
for (int j = 0; j < b.length; j++) {
out.print(b[j]);
}
} else {
out.print(-1);
}
out.println("");
} else {
out.println(k);
}
}
out.close();
}
}
class Read{
StringTokenizer st=new StringTokenizer("");
BufferedReader bf=new BufferedReader(new InputStreamReader(System.in));
String next() throws IOException{
while(!st.hasMoreTokens()){
st=new StringTokenizer(bf.readLine());
}
return st.nextToken();
}
int nextInt()throws IOException{
return Integer.parseInt(next());
}
}
牛客.体操队形(暴力搜索)
递归/dfs
画出决策树
import java.util.*;
public class Main{
public static int n;
//这个数组是用来标记放的数字,不然需要new 一个数组来存储
public static boolean[]vis=new boolean[15];
public static int[]a=new int[15];
public static int count=0;
public static void dfs(int ret){
//已经放到最后一个位置了,找到了合法情况
if(ret==n+1)
{
count++;
return;
}
for(int i=1;i<=n;i++){
if(vis[i]==true)continue; //第i号队员已经放过了,即 11_
if(vis[a[i]]) return; //不合法情况12_ _ 直接剪枝
vis[i]=true; //相当于已经放上了i号队员
dfs(ret+1);
vis[i]=false;
}
}
public static void main(String[]args){
//如果数据很小的情况下,大概率是用递归的想法
Scanner in=new Scanner(System.in);
n=in.nextInt();
for(int i=1;i<=n;i++){
a[i]=in.nextInt();
}
dfs(1);
System.out.print(count);
}
}
牛客.二叉树最大路径和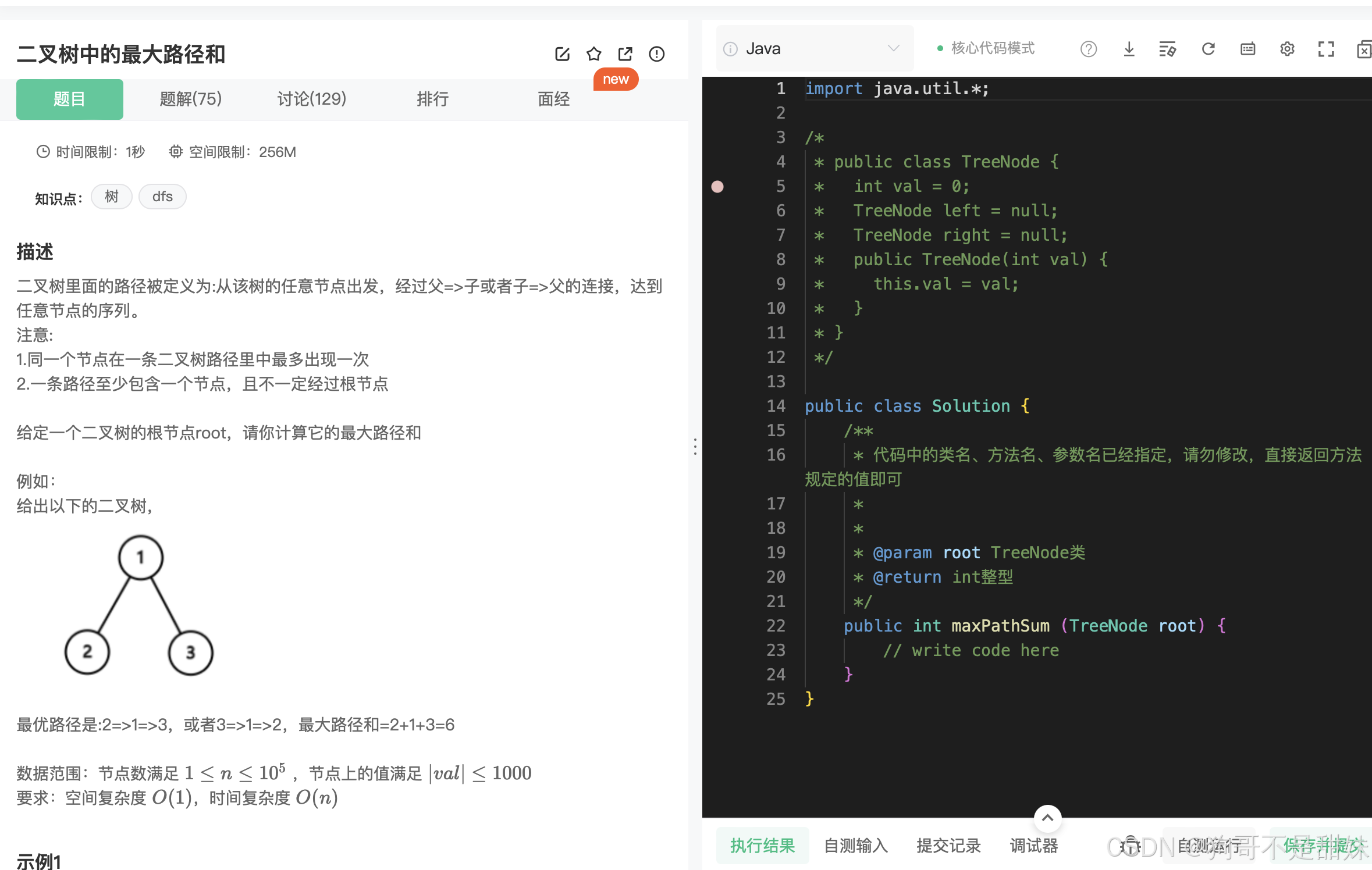
二叉树不是dfs/就是bfs/树形dp(不明显 如同dp[root],假如是堆就成了,树形遍历主要是后序遍历,)
import java.util.*;
/*
* public class TreeNode {
* int val = 0;
* TreeNode left = null;
* TreeNode right = null;
* public TreeNode(int val) {
* this.val = val;
* }
* }
*/
public class Solution {
/**
* 代码中的类名、方法名、参数名已经指定,请勿修改,直接返回方法规定的值即可
*
*
* @param root TreeNode类
* @return int整型
*/
//最后的返回结果是ret
int ret=-1010;
public int maxPathSum (TreeNode root) {
dfs(root);
return ret;
}
public int dfs (TreeNode root){
if(root==null){
return 0;
}
int l=Math.max(0,dfs(root.left));
int r=Math.max(0,dfs(root.right));
//ret值,ret是其中的左树单链和+右树单链和。
ret=Math.max(ret,root.val+l+r);
//但是这个函数返回的确是最长单链和。 返回值不一定是必须结果
return root.val+Math.max(l,r); //以我为根的最大单链和
}
}
牛客.排序子序列
看题半天没看懂啥意思,看了便题解,发现不是我想的那个意思,他的意思就是分段
然后我们需要找上升趋势的(一直取,直到它下降),(下降趋势也是一直取,直到它上升),平稳的往下一个走,看下一个什么情况
import java.util.Scanner; // 注意类名必须为 Main, 不要有任何 package xxx 信息 public class Main { public static void main(String[] args) { Scanner in = new Scanner(System.in); // 注意 hasNext 和 hasNextLine 的区别 int n=in.nextInt(); int[]a=new int[n]; for(int i=0;i<n;i++){ a[i]=in.nextInt(); } int count=0; //采用指针 int i=0; while(i<n){ //防止前面都ok。1 2 2 1 这个情况,i会剩到最后一个,这个时候,她单独一个节点需要++,否则就慢慢挪动指针, if(i==n-1){ count++; break; } if(a[i+1]>a[i]){ // 1 2 3 4 1 他跳出循环会count++; //假如发现了上升趋势,那么假如一直上升就一直++ while(i+1<n&&a[i]<=a[i+1])i++; count++; } //下降趋势 else if(a[i]>a[i+1]){ while(i+1<n&&a[i]>=a[i+1])i++; count++; } //2 2 2 1 这种情况就一直遍历 else{ while(i+1<n&&a[i]==a[i+1])i++; } i++; } System.out.print(count); } }