效果
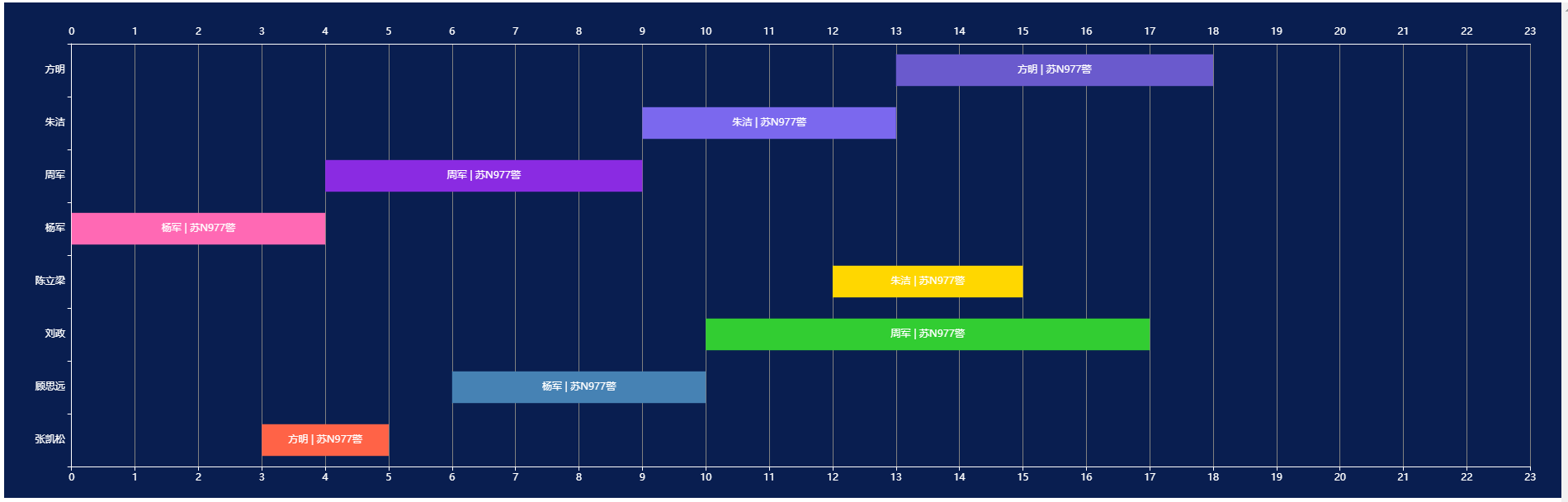
代码
<template>
<div class="index">
<div ref="scheduleChart" style="width: 100%; height: 600px"></div>
</div>
</template>
<script>
import * as echarts from "echarts";
export default {
name: "ScheduleChart",
data() {
return {
scheduleData: [
{
name: "张凯松",
shifts: [{ start: 3, end: 5, label: "方明 | 苏N977警" }],
},
{
name: "顾思远",
shifts: [{ start: 6, end: 10, label: "杨军 | 苏N977警" }],
},
{
name: "刘政",
shifts: [{ start: 10, end: 17, label: "周军 | 苏N977警" }],
},
{
name: "陈立梁",
shifts: [{ start: 12, end: 15, label: "朱洁 | 苏N977警" }],
},
{
name: "杨军",
shifts: [{ start: 0, end: 4, label: "杨军 | 苏N977警" }],
},
{
name: "周军",
shifts: [{ start: 4, end: 9, label: "周军 | 苏N977警" }],
},
{
name: "朱洁",
shifts: [{ start: 9, end: 13, label: "朱洁 | 苏N977警" }],
},
{
name: "方明",
shifts: [{ start: 13, end: 18, label: "方明 | 苏N977警" }],
},
],
XData: [
0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19,
20, 21, 22, 23,
],
};
},
mounted() {
this.initChart();
},
methods: {
initChart() {
this.chart = echarts.init(this.$refs.scheduleChart);
const yAxisData = this.scheduleData.map((item) => item.name);
const seriesData = this.scheduleData.map((item, index) => ({
name: item.name,
type: "custom",
renderItem: (params, api) => {
const categoryIndex = api.value(0);
const start = api.coord([api.value(1), categoryIndex]);
const end = api.coord([api.value(2), categoryIndex]);
const height = api.size([0, 1])[1] * 0.6;
return {
type: "group",
children: [
{
type: "rect",
shape: {
x: start[0],
y: start[1] - height / 2,
width: end[0] - start[0],
height: height,
},
style: api.style(),
},
{
type: "text",
style: {
text: api.value(3),
x: (start[0] + end[0]) / 2,
y: start[1],
fill: "white",
textAlign: "center",
textVerticalAlign: "middle",
fontSize: 12,
},
},
],
};
},
data: item.shifts.map((shift) => [
index,
shift.start,
shift.end,
shift.label,
]),
itemStyle: {
color: this.getColor(item.name),
},
}));
const option = {
tooltip: {
trigger: "item",
formatter: (params) => {
return `${params.value[3]}<br/>${params.value[1]}:00 - ${params.value[2]}:00`;
},
},
grid: {
left: "2%",
right: "2%",
bottom: "3%",
top: "5%",
containLabel: true,
},
xAxis: [
{
type: "category",
data: this.XData,
axisLabel: {
formatter: "{value}",
color: "white",
interval: 0,
},
axisLine: {
show: true,
lineStyle: {
color: "white",
},
},
axisTick: {
alignWithLabel: true,
},
splitLine: {
show: true,
lineStyle: {
color: "gray",
},
},
boundaryGap: false,
},
{
type: "category",
data: this.XData,
axisLabel: {
formatter: "{value}",
color: "white",
interval: 0,
},
axisLine: {
show: true,
lineStyle: {
color: "white",
},
},
axisTick: {
show: false,
alignWithLabel: true,
},
position: "top",
boundaryGap: false,
},
],
yAxis: {
type: "category",
data: yAxisData,
axisLabel: {
color: "white",
},
axisLine: {
show: true,
lineStyle: {
color: "white",
},
},
axisTick: {
show: true,
},
},
series: seriesData,
};
this.chart.setOption(option);
},
getColor(name) {
const colors = [
"#FF6347",
"#4682B4",
"#32CD32",
"#FFD700",
"#FF69B4",
"#8A2BE2",
"#7B68EE",
"#6A5ACD",
];
const index = this.scheduleData.findIndex((item) => item.name === name);
return colors[index % colors.length];
},
},
};
</script>
<style scoped>
.index {
background: #091e50;
}
</style>