sql 测试json表
CREATE TABLE `testjson` (
`id` int NOT NULL AUTO_INCREMENT,
`json_obj` json DEFAULT NULL,
`json_arr` json DEFAULT NULL,
`json_str` varchar(100) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_0900_ai_ci;
INSERT INTO test2.testjson
(id, json_obj, json_arr, json_str)
VALUES(1, '{"age": "1", "sex": "123"}', '[1, 2, 4]', '12');
INSERT INTO test2.testjson
(id, json_obj, json_arr, json_str)
VALUES(2, '{"age": "1", "sex": "123"}', '[1, 2, 4]', '12');
INSERT INTO test2.testjson
(id, json_obj, json_arr, json_str)
VALUES(3, '{"age": "1", "sex": "123"}', '[1, 2, 4]', '12');
INSERT INTO test2.testjson
(id, json_obj, json_arr, json_str)
VALUES(4, '{"age": "1", "sex": "123"}', '[1, 2, 4]', '12');
INSERT INTO test2.testjson
(id, json_obj, json_arr, json_str)
VALUES(5, '{"age": "1", "sex": "123"}', '[1, 2, 4]', '12');
后台springboot 文件pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.6.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>springboot-test</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>demo</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.3.0</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.11</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
application.yml
spring:
dataSource:
driver-class-name: com.mysql.cj.jdbc.Driver
password: root
url: jdbc:mysql://localhost:3307/test2?characterEncoding=utf8&useSSL=false&serverTimezone=UTC&rewriteBatchedStatements=true
username: root
实体类User.java
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import com.baomidou.mybatisplus.extension.handlers.JacksonTypeHandler;
import lombok.Data;
import java.io.Serializable;
@Data
@TableName(value = "testjson", autoResultMap=true)
public class User implements Serializable {
private static final long serialVersionUID = 1L;
@TableId(value = "id", type = IdType.AUTO)
private Long id;
@TableField(typeHandler = JacksonTypeHandler.class)
private JsonObj jsonObj;
private String jsonArr;
private String jsonStr;
}
JsonObj,java
@Data
public class JsonObj {
private String sex;
private Integer age;
}
UserMapper.java
@Mapper
public interface UserMapper extends BaseMapper<User> {
User selectById(Long id);
User selectByLike(String sex);
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.springboot.mapper.UserMapper">
<resultMap id="BaseResultMap" type="com.springboot.entity.User">
<id column="id" property="id"/>
<result column="json_obj" property="jsonObj"
typeHandler="com.baomidou.mybatisplus.extension.handlers.JacksonTypeHandler"/>
<result column="json_arr" property="jsonArr"/>
<result column="json_str" property="jsonStr"/>
</resultMap>
<select id="selectById" resultMap="BaseResultMap">
select *from testjson where `id`=#{id}
</select>
<select id="selectByLike" resultMap="BaseResultMap">
select * from testjson where json_obj->'$.sex' like '%'+#{sex}'+%'
</select>
</mapper>
import com.springboot.mapper.UserMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class TestController {
@Autowired
UserMapper userMapper;
@GetMapping("/testJson")
public String testJson(){
return "User===:"+userMapper.selectById(1L);
}
@GetMapping("/testJsonLike")
public String testJsonLike(){
return "User===:"+userMapper.selectByLike("123");
}
}
备注:常用JSON函数
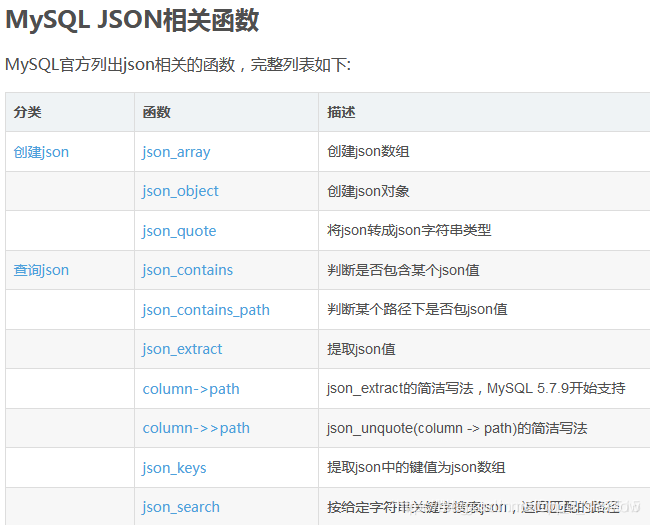
//插入和更新
JSON_SET和JSON_INSERT区别:set key存在会覆盖value,insert只会插入新的key,value
UPDATE testjson SET json_obj = JSON_SET(json_obj,'$.age','localhost','$.url','www.muscleape.com') WHERE id = 2;
UPDATE testjson SET json_obj = JSON_INSERT(json_obj,'$.url','www.test.com') WHERE id = 3;
//remove元素
UPDATE testjson SET json_obj = json_remove(json_obj, '$.age') WHERE id = 5;
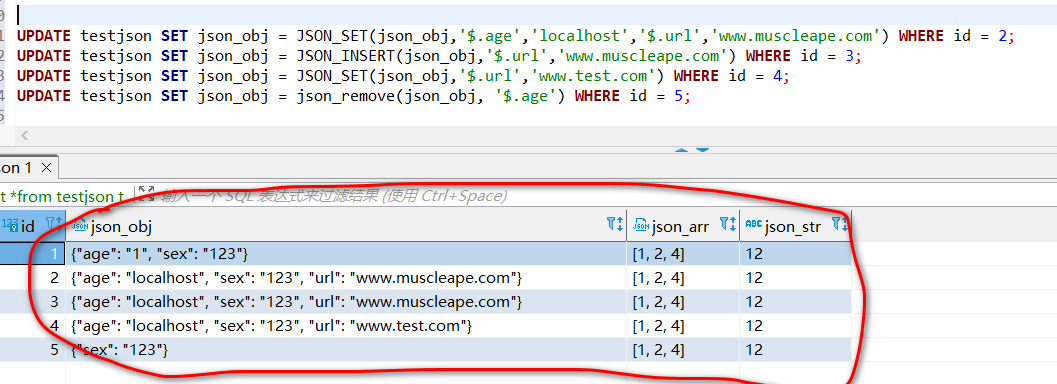
//模糊查询
select * from testjson where json_obj->'$.url' like '%muscleape%'
