(1)Java和C++在变量类型命名和使用
基本数据类型
对象类型与引用类型 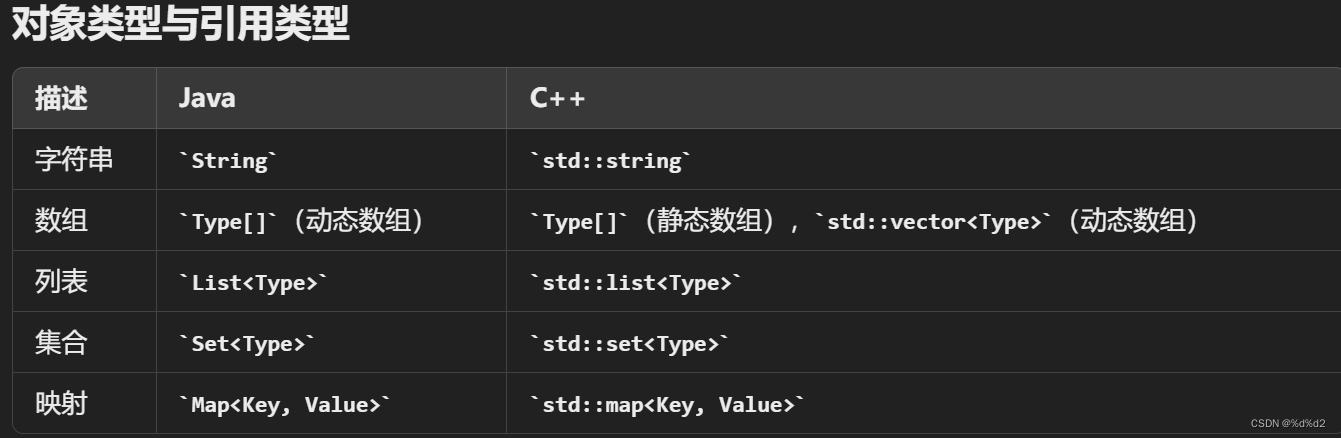
特殊类型
关键字和修饰符 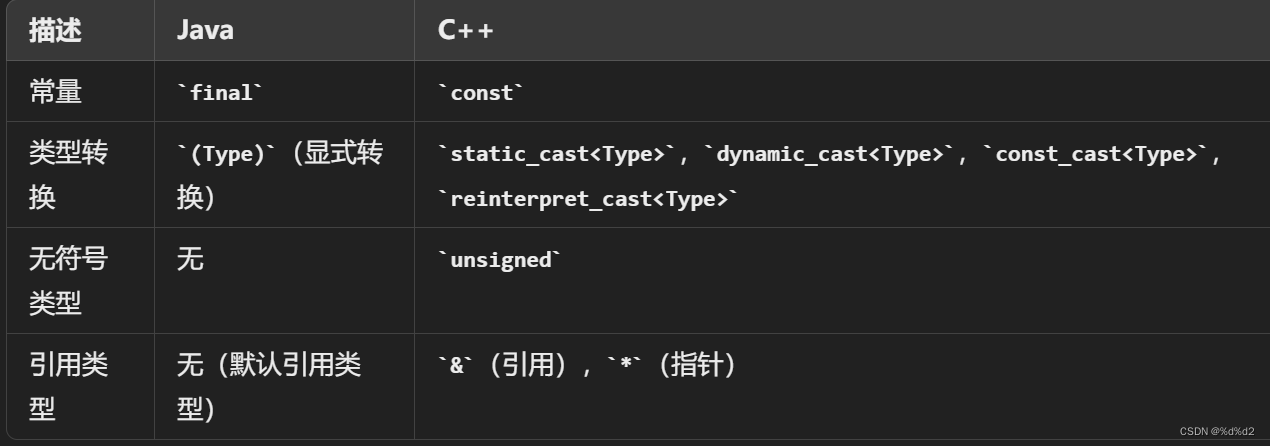
(2)快照图:
-
IDE调试工具:
- 许多IDE(如Eclipse、IntelliJ IDEA)提供了调试功能,可以在调试过程中查看程序的堆栈和堆的状态,帮助理解快照图。
示例代码:
public class SnapshotExample {
public static void main(String[] args) {
int a = 10;
int b = 20;
int c = a + b;
String s1 = "Hello";
String s2 = s1 + " World";
Person p = new Person("Alice", 30);
p.setAge(31);
}
}
class Person {
String name;
int age;
Person(String name, int age) {
this.name = name;
this.age = age;
}
void setAge(int age) {
this.age = age;
}
}
快照图:
Stack (栈) Heap (堆)
+-------------------+ +------------------------+
| main() | | (String@1) "Hello" |
|-------------------| +------------------------+
| a -> 10 | | (String@2) "Hello World"|
| b -> 20 | +------------------------+
| c -> 30 | | (Person@3) |
| s1 -> (String@1) | | name -> "Alice" |
| s2 -> (String@2) | | age -> 31 |
| p -> (Person@3) | +------------------------+
+-------------------+
(3)Java集合框架(Collections Framework)
import java.util.*;
public class CollectionOperations {
public static void main(String[] args) {
// 列表操作
List<String> list = new ArrayList<>(Arrays.asList("苹果", "香蕉", "橙子"));
list.remove("香蕉");
list.forEach(item -> System.out.println("列表项: " + item));
// 集合操作
Set<String> set = new HashSet<>(Arrays.asList("猫", "狗", "鸟"));
set.remove("狗");
set.forEach(item -> System.out.println("集合项: " + item));
// 映射操作
Map<String, Integer> map = new HashMap<>();
map.put("约翰", 25);
map.put("简", 30);
map.forEach((key, value) -> System.out.println(key + "的年龄是: " + value));
}
}