一、具体要求
编写
python
代码,扫描指定的目录下的所有文件,将这些扫描的文本内容邮 件发送到指定邮箱(
自己的
qq
邮箱
)
示例如下:
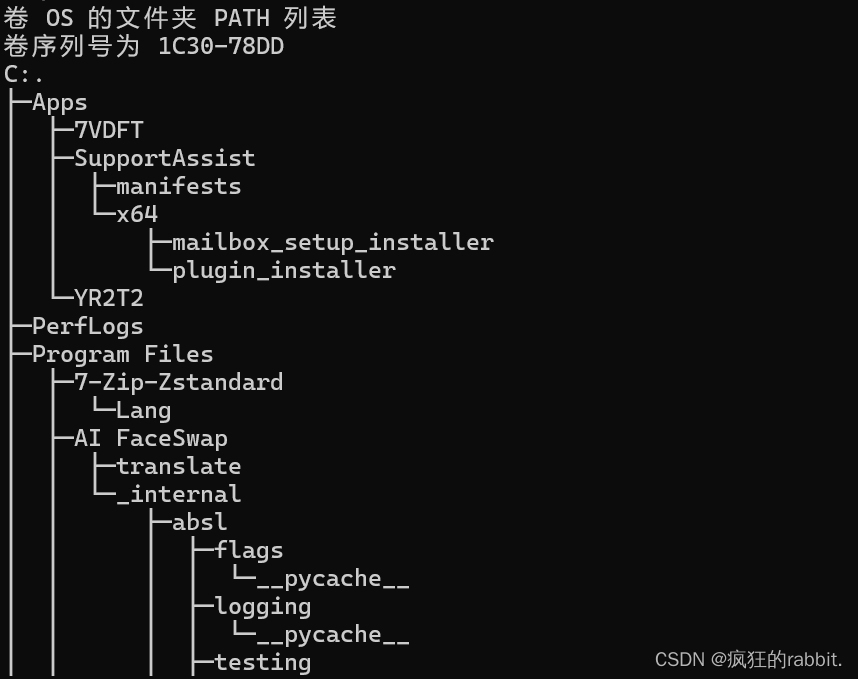
使用QQ邮箱SMTP服务器需要获取QQ邮箱授权码,获取方式可参考以下链接:
电子邮件
二、相关代码
import os
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
def list_files_recursively(path, depth=0):
"""扫描文件并打印树形结构"""
indent = " " * depth
files = sorted(os.listdir(path))
file_tree = ""
for f in files:
fullpath = os.path.join(path, f)
if os.path.isdir(fullpath):
file_tree += f"{indent}├── {f}/\n"
file_tree += list_files_recursively(fullpath, depth + 1)
if depth == 0 and f < files[-1]:
file_tree += f"{indent}│ \n"
elif os.path.isfile(fullpath):
file_tree += f"{indent}├── {f}\n"
if depth == 0 and f < files[-1]:
file_tree += f"{indent}│ \n"
return file_tree
def send_email(subject, body, to_email, from_email, password, smtp_server, smtp_port):
# 创建邮件对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain', 'utf-8'))
#SMTP 服务器
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(from_email, password)
server.sendmail(from_email, to_email, msg.as_string())
server.quit()
if __name__ == '__main__':
# 已经的一个文件夹路径
folder_path = "D:\\Typora"
tree_string = list_files_recursively(folder_path)
send_email(
"文件夹树形结构",
tree_string,
"**********@qq.com", # 收件人邮箱
"**********@qq.com", # 发件人邮箱(确保这个邮箱与你的SMTP账户一致)
"***********", # 此处填写你的QQ邮箱SMTP授权码
"smtp.qq.com",
587
)