new关键字
- 对象实例化
实例化对象的过程可以分为两部分:
(1) 声明对象:Cat one
(2) 实例化对象:new Cat()
- JVM可以被理解为Java程序与操作系统之间的桥梁
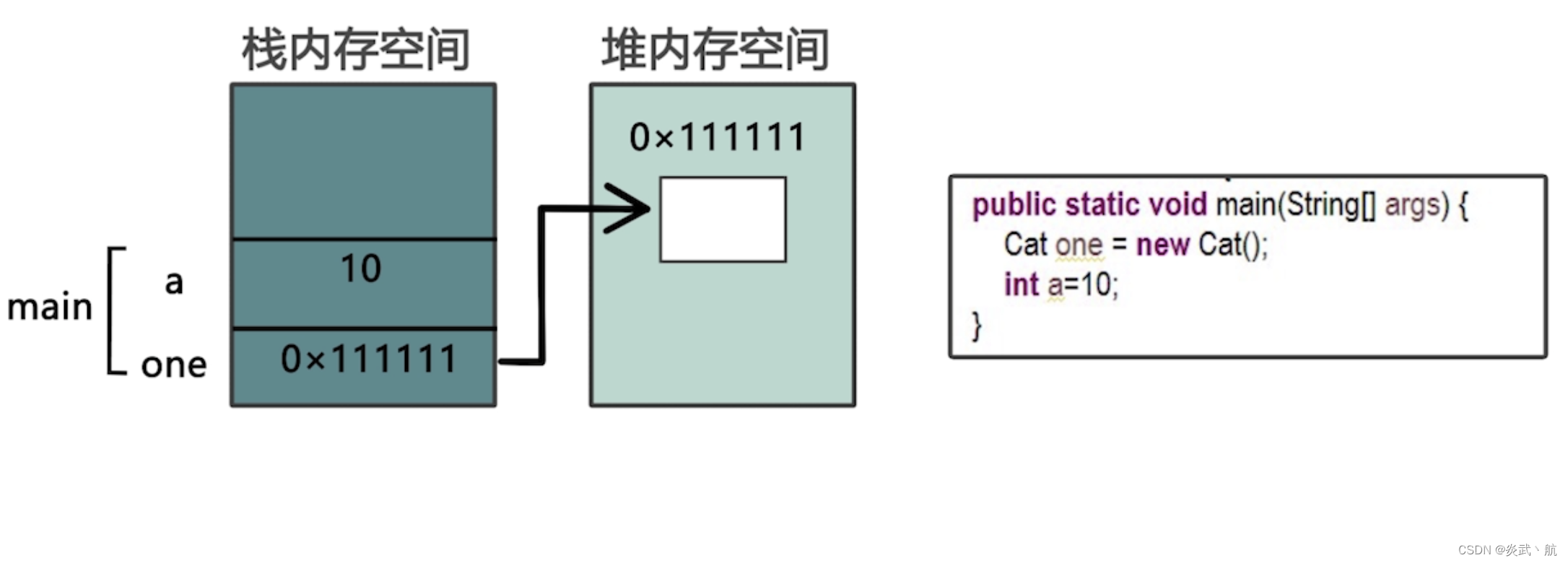
- 每次使用new关键字,就相当于在内存中开辟了一块新的存储空间。
package com.study.animal;
//单一职责原则
public class CatTest {
public static void main(String[] args) {
// 对象实例化
Cat one = new Cat();
Cat two = new Cat();
// 测试
// one.run();
one.name = "喵喵";
one.month = 2;
one.weight = 1000;
one.species = "英国短毛猫";
// two.run();
two.name = "卫卫";
two.month = 5;
two.weight = 800;
two.species = "英国短毛猫";
System.out.println("昵称:" + one.name);
System.out.println("年龄:" + one.month);
System.out.println("体重:" + one.weight);
System.out.println("品种:" + one.species);
System.out.println("昵称:" + two.name);
System.out.println("年龄:" + two.month);
System.out.println("体重:" + two.weight);
System.out.println("品种:" + two.species);
// one.run(one.name);
// one.Cat();
}
}
运行结果:
昵称:喵喵
年龄:2
体重:1000.0
品种:英国短毛猫
昵称:卫卫
年龄:5
体重:800.0
品种:英国短毛猫
注:修改two的信息是不会对one产生影响的。
4. 将one赋值给two
package com.study.animal;
//单一职责原则
public class CatTest {
public static void main(String[] args) {
// 对象实例化
Cat one = new Cat();
Cat two = one;
// 测试
// one.run();
one.name = "喵喵";
one.month = 2;
one.weight = 1000;
one.species = "英国短毛猫";
// two.run();
two.name = "卫卫";
two.month = 5;
two.weight = 800;
two.species = "英国短毛猫";
System.out.println("昵称:" + one.name);
System.out.println("年龄:" + one.month);
System.out.println("体重:" + one.weight);
System.out.println("品种:" + one.species);
System.out.println("昵称:" + two.name);
System.out.println("年龄:" + two.month);
System.out.println("体重:" + two.weight);
System.out.println("品种:" + two.species);
// one.run(one.name);
// one.Cat();
}
}
运行结果:
昵称:卫卫
年龄:5
体重:800.0
品种:英国短毛猫
昵称:卫卫
年龄:5
体重:800.0
品种:英国短毛猫
注:将one赋值给two,相当于,one和two拥有同一个家的钥匙,因此,one和two对这个家的任何改变都会影响家的布局。
5. 几点注意事项
(1) 需要多次访问同一对象时,必须进行声明;
注:可以匿名对象进行方法调用
new Cat().run();
(2) 同一作用范围内,必能定义同名对象;
例如:
Cat one = new Cat();
Cat one;
则会产生异常:Duplicate local variable one,即局部变量信息已经被定义过了,不能够进行重复定义。
(3) 可以同时声明多个引用,用逗号分隔
例如:
Cat one,two;
one = new Cat();
two = new Cat();
Cat Three = new Cat(), four = new Cat();