使用Unity提供的JsonUtility 简单封装了一个将数据以json格式存储到本地,方便数据读写的案例;
一共三个脚本:
MyJsonRW : 提供SaveJsonData和LoadWithJson,实现数据与json的转换和读写;
TestData : 测试数据类,列举了部分常用的简单数据类型(不过有坑,后面会说);
MyJsonExample : 例子实践,随便弄的UI;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System;
using System.IO;
public class MyJsonRW
{
public static void SaveJsonData<T>(string path, T data)
{
if (string.IsNullOrEmpty(path))
{
Debug.LogError("存储路径不存在");
return;
}
string json = JsonUtility.ToJson(data, true);
File.WriteAllText(path, json);
Debug.Log("json存储完成,路径:" + path);
}
public static T LoadWithJson<T>(string path)
{
if (string.IsNullOrEmpty(path))
{
Debug.LogError("读取路径不存在");
return default(T);
}
string text = File.ReadAllText(path);
T info = JsonUtility.FromJson<T>(text);
Debug.Log("读取 " + path + " 完成");
return info;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[System.Serializable]
public class TestData
{
public string str = "abc123测试";
public int num = 1123456;
public float f = 66589.451f;
public bool flag = true;
public List<string> list = new List<string>();
public void InitData()
{
list = new List<string>();
for (int i = 0; i < 5; i++)
{
string s = "list_data_" + i;
list.Add(s);
}
}
public void InitData(TestData data)
{
Debug.Log("读取数据==>");
str = data.str;
Debug.Log("str =" + str);
num = data.num;
Debug.Log("num =" + num);
f = data.f;
Debug.Log("f =" + f);
flag = data.flag;
Debug.Log("flag =" + (flag ? "true" : "false"));
list = data.list;
for (int i = 0; i < list.Count; i++)
{
Debug.Log(list[i]);
}
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MyJsonExample : MonoBehaviour
{
TestData data = new TestData();
string path = @"C:\Users\PC_20201126\Desktop\MyJson.json";
// Start is called before the first frame update
void Start()
{
data.InitData();
}
public void SaveTest()
{
MyJsonRW.SaveJsonData<TestData>(path, data);
}
public void LoadTest()
{
TestData L_data = MyJsonRW.LoadWithJson<TestData>(path);
L_data.InitData(L_data);
}
}
UI长这样😁
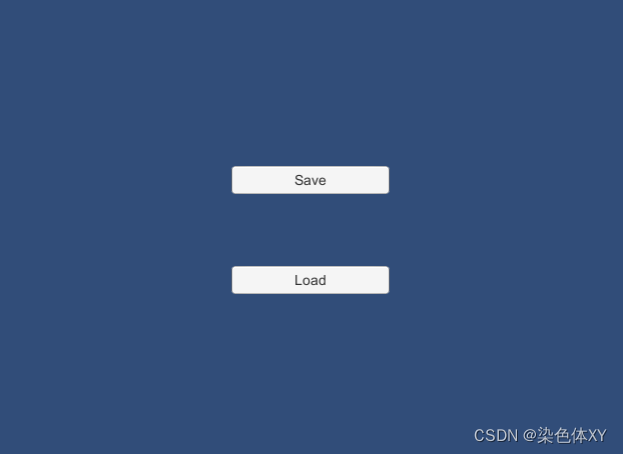
运行后的Log如下图:
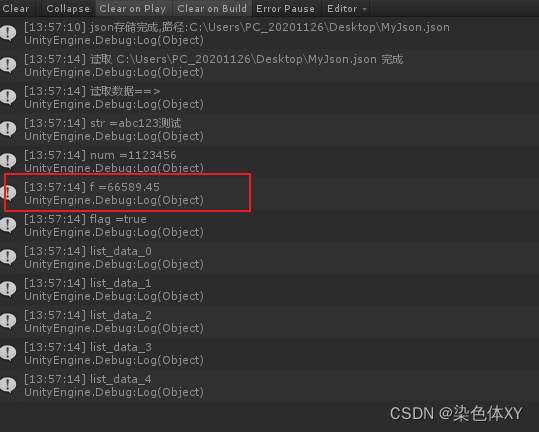
生成的json文件内容如下:
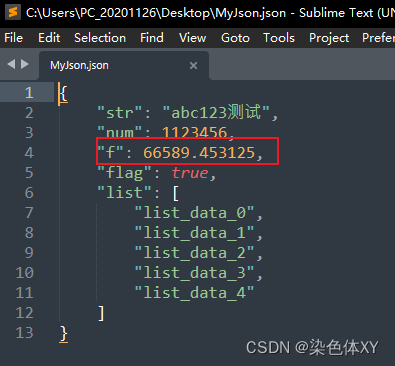
机智的小伙伴已经看到我红框里的内容了,是的浮点数有问题,代码里是 public float f = 66589.451f;
存储的,读取的,都不是这个数😂. 其他帖子给出的办法都是浮点数以string格式读写(就是存储的时候先转string,读取的时候再转回来);
还有遇到本地json里的内容是{},没有其他东西,但是读写数据都正常; 数据类成员变量没有公有化,就是要写成public ;
至于其他问题,如不支持枚举,只接受一个json对象,对泛型的支持不够友好等,网上基本都有解决方案,不在这里做过多赘述;第三方的json工具也不少,不一定非要用这个.