运算符重载
代码
#include <iostream>
using namespace std;
class Num
{
private:
int num1; //实部
int num2; //虚部
public:
Num(){}; //无参构造
Num(int n1,int n2):num1(n1),num2(n2){}; //有参构造
~Num(){}; //析构函数
const Num operator+(const Num &other)const //加号重载
{
Num a; //定义临时类对象
a.num1 = num1 + other.num1; //实部相加
a.num2 = num2 + other.num2; //虚部相加
return a;
}
bool operator==(const Num &other)const //相等重载
{
//判断实部虚部是否分别相等
if(num1 == other.num1 && num2 == other.num2)
{
return true;
}
else
{
return false;
}
}
Num &operator+=(const Num &other) //加等重载
{
//实部虚部分别加等于
num1 += other.num1;
num2 += other.num2;
return *this;
}
Num &operator++() //重载前置++
{
++num1;
++num2;
return *this;
}
Num &operator++(int) //重载后置++
{
num1++;
num2++;
return *this;
}
Num operator-()const //重载-
{
Num temp;
temp.num1 = -num1;
temp.num2 = -num2;
return temp;
}
void init(int n1,int n2) //修改类中的值
{
num1 = n1;
num2 = n2;
}
void show() //展示
{
if(num2 < 0)
{
cout << num1 << num2 << "j" << endl;
}
else
{
cout << num1 << "+" << num2 << "j" << endl;
}
}
};
int main()
{
Num test0; //调用无参构造
Num test1(2,3); //调用有参构造
test0.show(); //调用展示函数
cout << "*********************" << endl;
test0.init(3,2); //调用修改函数
test0.show();
cout << "*********************" << endl;
test0++;
test0.show();
cout << "*********************" << endl;
++test0;
test0.show();
cout << "*********************" << endl;
if(test0 == test1) // 调用重载的相等函数
{
cout << "true" << endl;
}
else
{
cout << "false" << endl;
}
cout << "*********************" << endl;
test0 += test1; //调用加等于重载函数
test0.show();
test1 = -test0; //调用重载-
cout << "*********************" << endl;
test1.show();
return 0;
}
运行结果
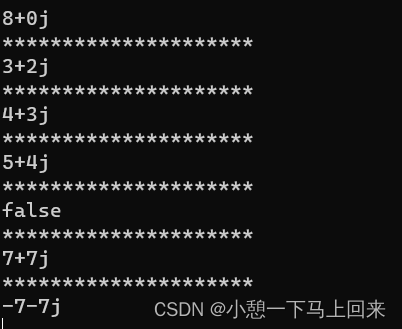