一、运算符重载
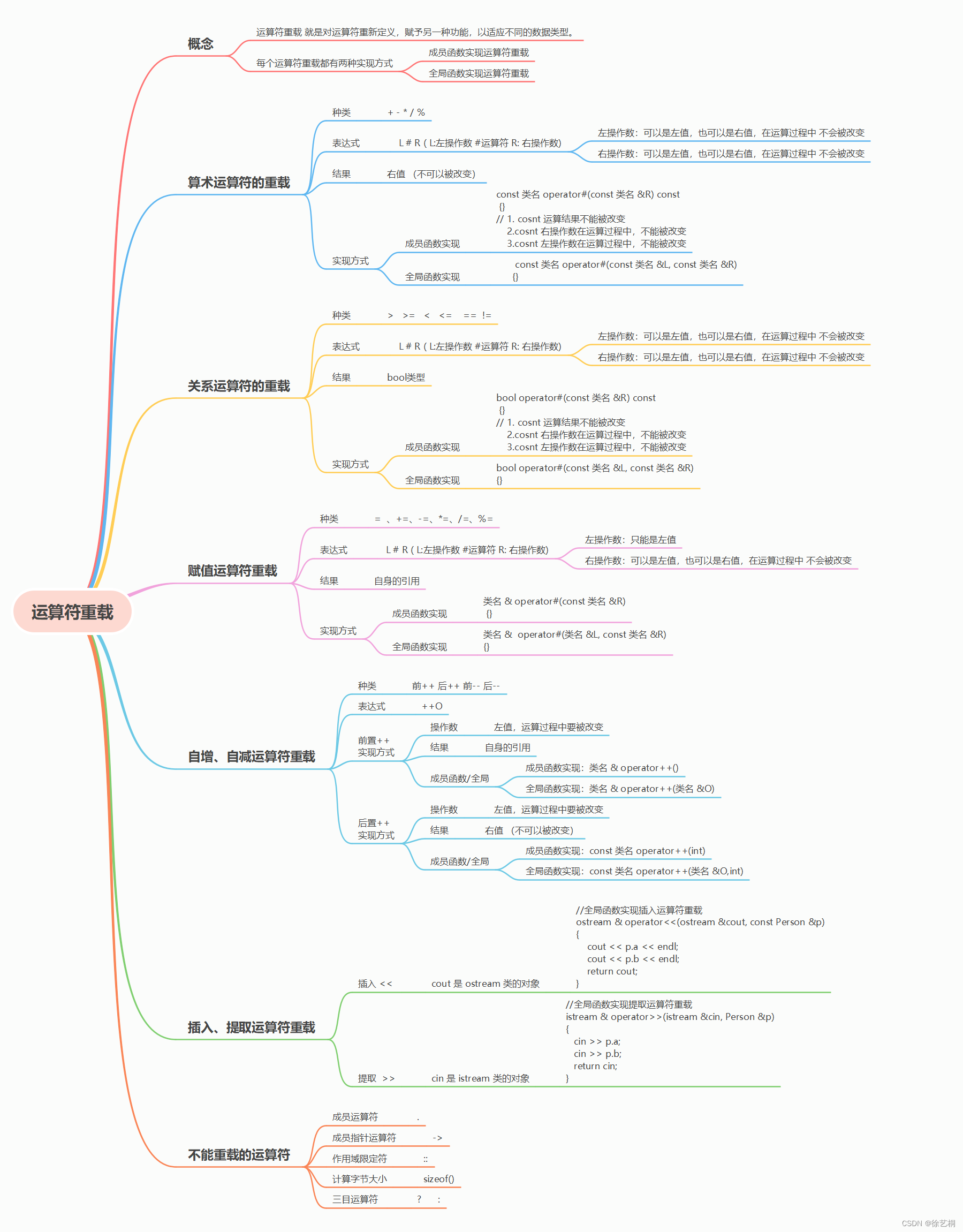
实例:(赋值运算符、自增自减运算符、插入提取运算符)
#include <iostream>
using namespace std;
class Person
{
friend Person & operator+=(Person &L,const Person &R);
friend Person & operator++(Person &O);
friend const Person operator++(Person &O,int);
friend ostream & operator<<(ostream & cout,const Person &p);
friend istream & operator>>(istream &cin,Person &p);
int a;
int b;
public:
//无参构造函数
Person() {}
//有参构造函数
Person(int a,int b):a(a),b(b)
{}
//成员函数实现+=运算符重载
// Person & operator+=(const Person &R)
// {
// a += R.a;
// b += R.b;
// return *this;
// }
//成员函数实现前++运算符重载
// Person & operator++()
// {
// ++a;
// ++b;
// return *this;
// }
//成员函数实现后++运算符重载
// const Person operator++(int)
// {
// Person temp;
// temp.a = a++;
// temp.b = b++;
// return temp;
// }
void show()
{
cout << "a = " << a << endl;
cout << "b = " << b << endl;
}
};
//全局函数实现+=运算符重载
Person & operator+=(Person &L,const Person &R)
{
L.a += R.a;
L.b += R.b;
return L;
}
//全局函数实现前++运算符重载
Person & operator++(Person &O)
{
++O.a;
++O.b;
return O;
}
//全局函数实现后++运算符重载
const Person operator++(Person &O,int)
{
Person temp;
temp.a = O.a++;
temp.b = O.b++;
return temp;
}
//全局函数实现插入
ostream & operator<<(ostream &cout,const Person &p)
{
cout << p.a << endl;
cout << p.b << endl;
return cout;
}
//全局函数实现提取
istream & operator>>(istream &cin,Person &p)
{
cin >> p.a;
cin >> p.b;
return cin;
}
int main()
{
Person p1(10,10);
Person p2(20,20);
cout << "--------- 实现+= ----------" << endl;
//+=
p1 += p2;
p1.show();
cout << "-------- 实现 前++ ---------" << endl;
//前++
Person p3(10,10);
p1 = ++p3;
p3.show();
p1.show();
cout << "-------- 实现 后++ ----------" << endl;
//后++
Person p4(10,10);
p4 = p2++;
p2.show();
p4.show();
cout << "-------- 实现cout ----------" << endl;
cout << p1;
cout << "-------- 实现cin ----------" << endl;
cin >> p2;
cout << p2;
return 0;
}
二、静态成员
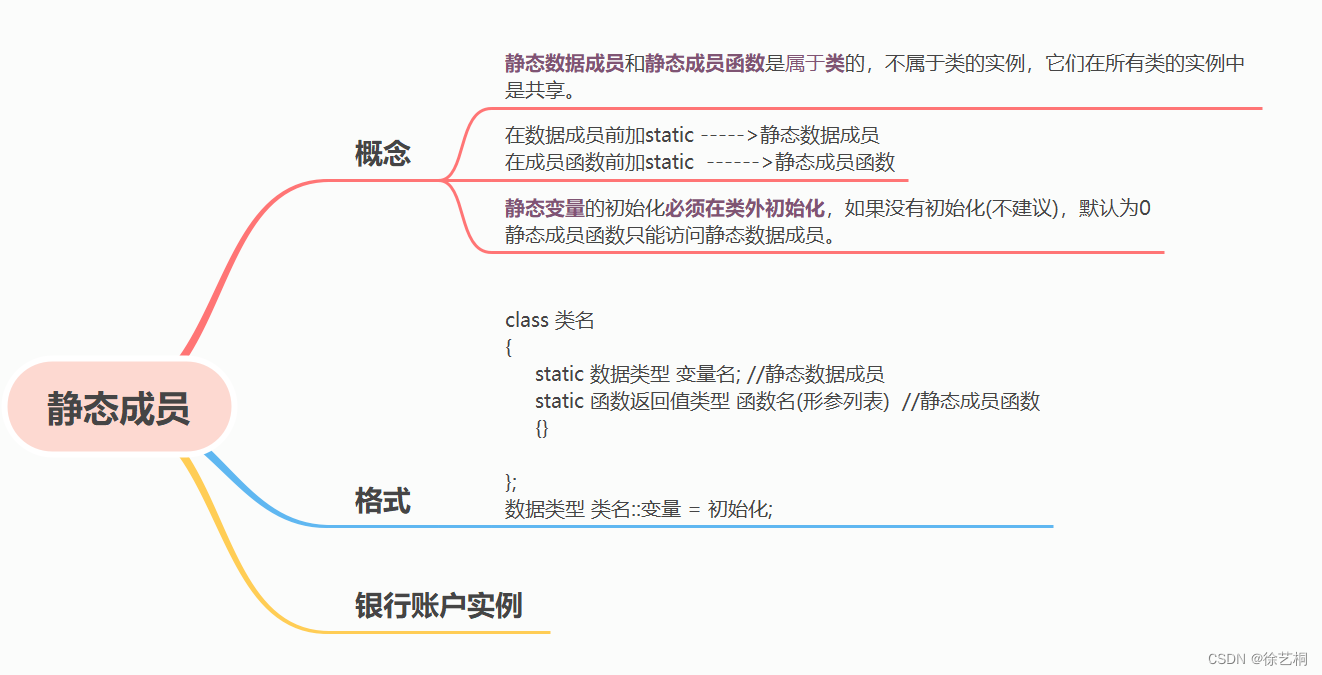
银行账户实例
#include <iostream>
using namespace std;
//封装银行账户 类
class BankAccount
{
private:
double balance; //余额
static double interest_rate; //利率 静态数据成员 属于类的
public:
//无参构造
BankAccount() {}
//有参构造函数
BankAccount(double b):balance(b)
{}
//静态成员函数 获取当前的利率
static double getInterestRate()
{
return interest_rate;
}
//静态成员函数,设置当前利率
static void setInterestRate(double rate)
{
interest_rate = rate;
}
//静态成员函数 获取连本带利的余额
static double getLastMoney(BankAccount &account)
{
return account.balance*(1+interest_rate);
}
};
double BankAccount::interest_rate = 0.05; //在类外初始化 静态数据成员
int main()
{
cout << "当前利率:" << BankAccount::getInterestRate() << endl;
BankAccount::setInterestRate(0.03);
cout << "当前利率:" << BankAccount::getInterestRate() << endl;
BankAccount account1(1000.0);
BankAccount account2(2000.0);
cout << "第一个人连本带利的余额:" << BankAccount::getLastMoney(account1) << endl;
return 0;
}
三、类的继承
实例
#include <iostream>
using namespace std;
class Person //父类
{
private:
string name;
int age;
public:
//无参构造函数
Person()
{
cout << "父类的无参构造函数" << endl;
}
//有参构造函数
Person(string name, int age):name(name),age(age)
{
cout << "父类的有参构造函数" << endl;
}
//拷贝构造函数
Person(Person const &other):name(other.name),age(other.age)
{
cout << "父类的拷贝构造函数" << endl;
}
//拷贝赋值函数
Person & operator=(const Person &p)
{
name = p.name;
age = p.age;
cout << "父类的拷贝赋值函数" << endl;
return *this;
}
void show()
{
cout << "父类的show函数" << endl;
}
~Person()
{
cout << "父类的析构函数" << endl;
}
};
class Stu:public Person // 子类/派生类
{
private:
int id;
int math;
public:
//无参构造函数
Stu()
{
cout << "子类的无参构造函数" << endl;
}
//有参构造函数
Stu(string name, int age, int id, int math):Person(name,age),id(id),math(math)
{
cout << "子类的有参构造函数" << endl;
}
//子类的拷贝构造函数
Stu(Stu const &p):id(p.id),math(p.math),Person(p)
{
cout << "子类的拷贝构造函数" << endl;
}
//子类的拷贝赋值函数
Stu & operator=(const Stu &p)
{
Person::operator=(p);
id = p.id;
math = p.math;
cout << "子类的拷贝构造函数" << endl;
return *this;
}
void show()
{
cout << "子类的show函数" << endl;
}
~Stu()
{
cout << "子类的析构函数" << endl;
}
};
int main()
{
// cout << "----------------------无参构造函数-------" << endl;
// Stu p;
// cout << "----------------------有参构造函数-------" << endl;
// Stu p1("张三",8,1,99);
// cout << "----------------------拷贝构造函数-------" << endl;
// Stu p2=p1;
// cout << "----------------------show函数-------" << endl;
// p2.show();
// p2.Person::show();
Stu p1("张三",8,1,99);
cout << "----------------------拷贝赋值函数-------" << endl;
Stu p2;
p2 = p1;
return 0;
}
四、多继承
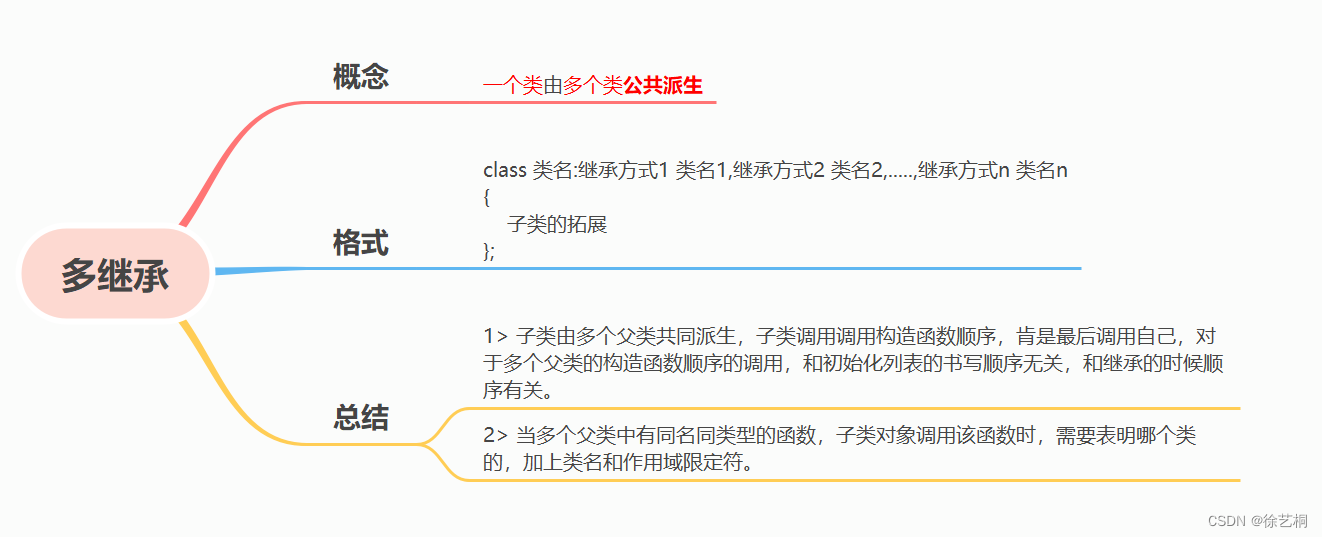
实例
#include <iostream>
using namespace std;
class Sofa
{
private:
string sitting;
public:
//无参构造函数
Sofa()
{
cout<< "Sofa的无参构造函数" << endl;
}
//有参构造函数
Sofa(string sitting):sitting(sitting)
{
cout<< "Sofa的有参构造函数" << endl;
}
void show()
{
cout << sitting << endl;
}
};
class Bed
{
private:
string sleep;
public:
//无参构造函数
Bed()
{
cout<< "Sofa的无参构造函数" << endl;
}
//有参构造函数
Bed(string sleep):sleep(sleep)
{
cout<< "Sofa的有参构造函数" << endl;
}
void show()
{
cout << sleep << endl;
}
};
class Sofa_Bed:public Sofa,public Bed
{
private:
int w;
public:
//无参构造函数
Sofa_Bed()
{
cout<< "沙发床的无参构造函数" << endl;
}
//有参构造函数
Sofa_Bed(string sit,string sleep,int w):Sofa(sit),Bed(sleep),w(w)
{
cout<< "沙发床的有参构造函数" << endl;
}
};
int main()
{
//Sofa_Bed s;
Sofa_Bed s1("可坐","可睡",5);
s1.Sofa::show();
s1.Bed::show();
return 0;
}