效果图:
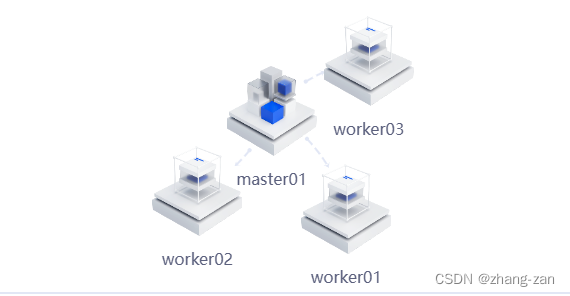
代码:
<template>
<div id="serveGraph" style="height: 100%; width: 100%; z-index: 88;"></div>
</template>
<script>
import { defineComponent,reactive,toRefs,onMounted,watch } from 'vue'
import * as echarts from 'echarts'
export default defineComponent({
name:'drawGraph',
props:{
graphData:{
type:Object,
default:{
data:[],
link:[],
}
},
},
emits:[],
components:{},
setup(props,ctx) {
const state = reactive({
data:[],
links:[],
paramsData:[],
myChart: null,
})
onMounted(() => {})
// 初始化数据
const init = function() {
state.data = [];
state.links = [];
let dataList = props.graphData.data;
for(var i = 0; i < dataList.length; i++) {
var obj = {
"name": dataList[i].name,
"type":'1',
symbol: dataList[i].master == true ? 'image://static/images/serve-1.png' : 'image://static/images/serve-2.png',
"symbolSize":[90,90],
"dragable":false,
"itemStyle":{
},
"dataDetail":dataList[i],
"category":1,
"lineStyle":{
width:1,
},
"label":{
show:true, // 是否显示标签
offset:[0,70],
fontSize:16,
color:'#5B607D'
},
}
state.data.push(obj)
}
draw();
}
// 绘制图表
const draw = function() {
var option = {
// 图表标题
title:{
show: false, // 显示策略,默认值true, 可选为: true(显示) | false (隐藏)
text:'服务器分布', // 主标题文本, '\n'指定换行
x:'center', // 水平安放位置,默认为左对齐,可选为:'center','left','right',{number}(x坐标,单位px)
y:'center', // // 垂直安放位置,默认为全图顶端,可选为:
// 'top' ¦ 'bottom' ¦ 'center'¦ {number}(y坐标,单位px)
//textAlign: null // 水平对齐方式,默认根据x设置自动调整
//backgroundColor: 'rgba(0,0,0,0)',
//borderColor: '#ccc', // 标题边框颜色
//borderWidth: 0, // 标题边框线宽,单位px,默认为0(无边框)
//padding: 5, // 标题内边距,单位px,默认各方向内边距为5,
// 接受数组分别设定上右下左边距,同css
itemGap:40, //主副标题纵向间隔, 单位为px,默认为10
textStyle: {
fontSize: 50,
fontWeight: 'bolder',
color: '#fff' // 主标题文字颜色
},
subtextStyle: {
color: '#aaa' // 副标题文字颜色
},
},
xAxis: {
show: false,
type: "value"
},
yAxis: {
show: false,
type: "value"
},
tooltip: {
trigger: 'item',
textStyle: { fontSize: '100%'},
axisPointer:{},
position: 'top',
show:true,
borderColor: 'red',
backgroundColor:'#fff',
textStyle:{
color:'#5B607D'
},
shadowColor: 'none',
borderWidth: '0', //边框宽度设置1
borderColor: 'transparent', //设置边框颜色
enterable:true,
formatter: params => {
let string = ``;
string += `<div style="min-width:200px;max-height:200px;background:#fafafa">
<div style="padding:10px;background:#fafafa">服务器信息详情</div>
<div style="padding:10px;background:#fafafa">
<div style="height:30px"><span style="display:inline-block;width:80px">名称:</span> <span>${params.data.name}</span></div>
</div>
</div>
`
return string;
},
},
// backgroundColor:'rgba(255, 255, 255, 0.5)',
// animationDurationUpdate: function (idx) {
// // 越往后的数据延迟越大
// return idx * 1000;
// },
// animationEasingUpdate: 'bounceIn',
series: [
{
type: 'graph',
name: "服务器详情信息",
layout: 'force',
force: {
// initLayout:5 , // 力引导的初始化布局,默认使用xy轴的标点
repulsion: 1300, // 节点之间的斥力因子。支持数组表达斥力范围,值越大斥力越大。
gravity: 0.4, // 节点受到的向中心的引力因子。该值越大节点越往中心点靠拢。
edgeLength: 100, // 边的两个节点之间的距离,这个距离也会受 repulsion影响 。值越大则长度越长
layoutAnimation: true // 因为力引导布局会在多次迭代后才会稳定,这个参数决定是否显示布局的迭代动画
// 在浏览器端节点数据较多(>100)的时候不建议关闭,布局过程会造成浏览器假死。
},
lineStyle: { // ========关系边的公用线条样式。
color: '#e2e7f5',
width: 2, //线的粗细
type: 'dashed', // 线的类型 'solid'(实线)'dashed'(虚线)'dotted'(点线)
globalCoord: false,
curveness: 0.2, // 线条的曲线程度,从0到1
opacity: 1// 图形透明度。支持从 0 到 1 的数字,为 0 时不绘制该图形。默认0.5
},
edgeSymbol: ['circle', 'arrow'],
edgeSymbolSize: 4,
edgeLabel: { show: false, textStyle: { fontSize: 10,color:'#fff ' }, formatter: "{c}" },
roam: true,
label: {
show: true,
color: "#fff"
},
data: state.data,
links: props.graphData.link,
draggable:true,
zoom:1,
},
]
}
state.myChart = echarts.init(document.getElementById('serveGraph'));
window.addEventListener('resize',() => {
state.myChart.resize();
})
state.myChart.setOption(option);
}
watch(()=>props.graphData,(val) => {
init();
},{
// immediate:true,
deep:true,
})
return {
...toRefs(state),
}
}
})
</script>
<style lang="scss" scoped>
</style>