剑指 Offer 第二版
文章目录
- 剑指 Offer 第二版
- [剑指 Offer 06. 从尾到头打印链表](https://www.nowcoder.com/practice/d0267f7f55b3412ba93bd35cfa8e8035?tpId=13&tqId=23278&ru=/exam/oj/ta&qru=/ta/coding-interviews/question-ranking&sourceUrl=%2Fexam%2Foj%2Fta%3Fpage%3D1%26tpId%3D13%26type%3D13)
剑指 Offer 06. 从尾到头打印链表
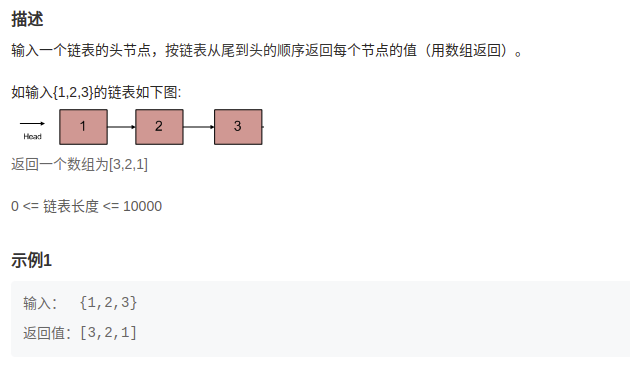
方法一:递归
看到这题,因为是从尾到头打印输出,首先想到的方式就是递归
class Solution {
public:
vector<int>res;
vector<int> reversePrint(ListNode* head) {
if(head==nullptr)
{
return {};
}
reversePrint(head->next);
res.push_back(head->val);
return res;
}
};
因为要遍历N个节点,因此时间复杂度为:O(N);
递归的实现方式调用函数栈,遍历N个节点,因此栈的大小至少为N,那么空间复杂度为:O(N)。
方法二:辅助栈
#include <stack>
#include <vector>
class Solution {
public:
vector<int> printListFromTailToHead(ListNode* head) {
stack<int>sta;
vector<int>res;
while (head!=nullptr) {
sta.push(head->val);
head=head->next;
}
while (!sta.empty()) {
res.push_back(sta.top());
sta.pop();
}
return res;
}
};
方法三:翻转数组
class Solution {
public:
vector<int> printListFromTailToHead(ListNode* head) {
vector<int> res;
while (head != nullptr) {
res.push_back(head->val);
head = head->next;
}
reverse(res.begin(), res.end());
return res;
}
};