题目描述
法一)计算链表长度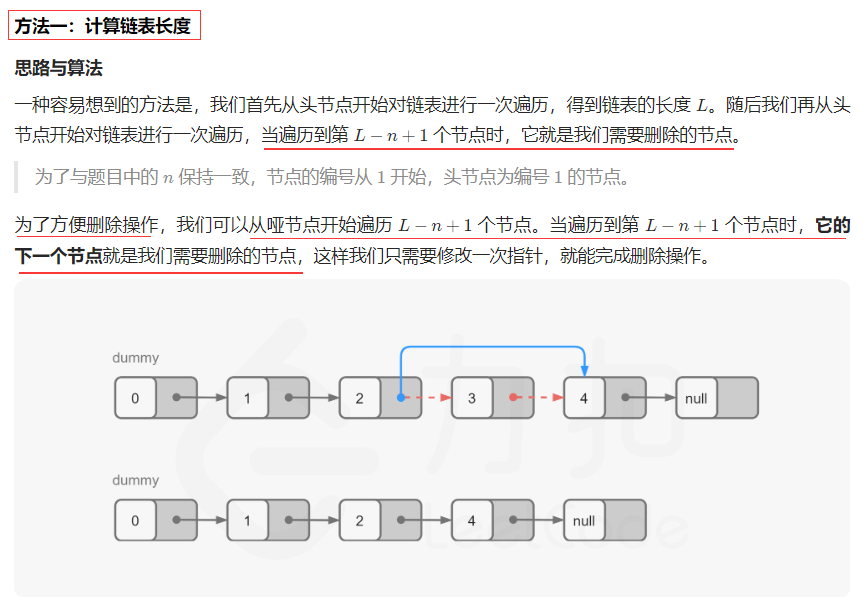
class Solution {
public:
int getLength(ListNode* head){
int len=0;
while(head){
++len;
head =head->next;
}
return len;
}
ListNode* removeNthFromEnd(ListNode* head, int n) {
int len = getLength(head);
ListNode* dummy = new ListNode (0, head); //0表示结点值,head表示下一个结点
ListNode* cur = dummy;
for(int i=1;i<len-n+1;i++){
cur = cur->next;
}
cur->next = cur->next->next;
ListNode* ans = dummy->next;
delete dummy;
return ans;
}
};
法二)栈
class Solution{
public:
ListNode* removeNthFromEnd(ListNode* head, int n){
stack<ListNode*> stk;
ListNode* dummy = new ListNode(0, head);
ListNode* cur = dummy;
while(cur){
stk.push(cur);
cur = cur->next;
}
for(int i=0;i<n;i++){
stk.pop();
}
ListNode* prev = stk.top();
prev->next = prev->next->next;
ListNode* ans = dummy->next;
delete dummy;
return ans;
}
};
法三)双指针