一、SSM框架优化的方向
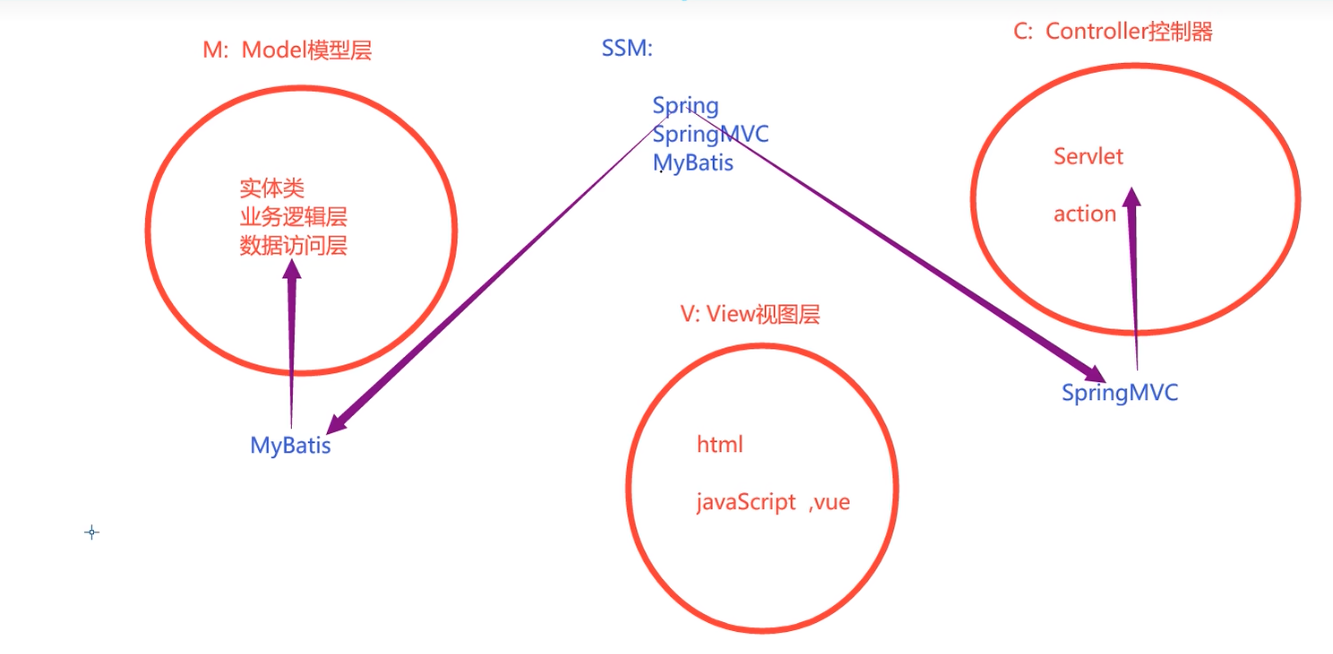
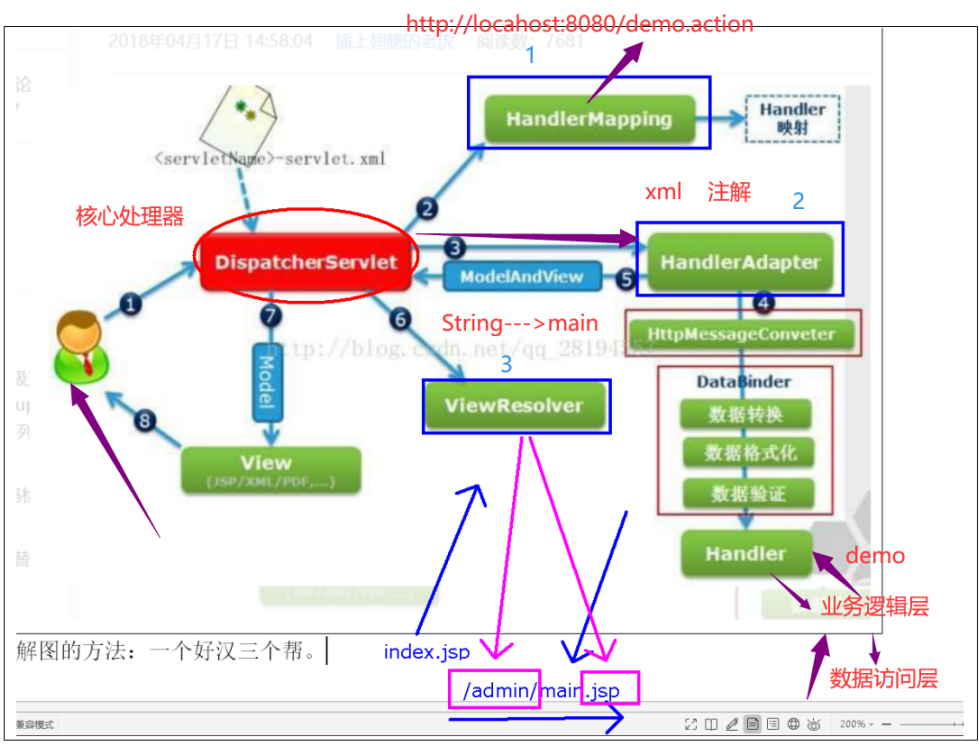
目录结构:
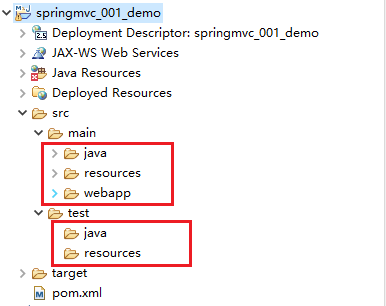
二、前期文件配置
pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.itheima</groupId>
<artifactId>springmvc_001_demo</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>war</packaging>
<dependencies>
<!--springmvc依赖-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!--servlet依赖-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>maven.plugins</finalName>
<pluginManagement>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.1</version>
</plugin>
</plugins>
</pluginManagement>
<resources>
<resource>
<directory>${basedir}/src/main/java</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
<resource>
<directory>${basedir}/src/main/resources</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</build>
</project>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context-3.0.xsd">
<context:component-scan base-package="com.itheima.controller" />
<!--配置spring创建容器时要扫描的包-->
<context:component-scan base-package="com.itheima.controller"></context:component-scan>
<!-- 处理映射器
<bean class="org.springframework.web.servlet.handler.BeanNameUrlHandlerMapping"/>
处理器适配器
<bean class="org.springframework.web.servlet.mvc.SimpleControllerHandlerAdapter"/>
-->
<!--配置视图解析器-->
<bean id="viewResolver" class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<!-- 配置前缀 -->
<property name="prefix" value="/WEB-INF/admin/"/>
<!-- 配置后缀 -->
<property name="suffix" value=".jsp"/>
</bean>
<!--配置spring开启注解mvc的支持 默认就是开启的 ,要想让其他组件(不包含映射器、适配器、处理器)生效就必须需要配置了-->
</beans>
(很重要)为什么要注册DispatcherServlet

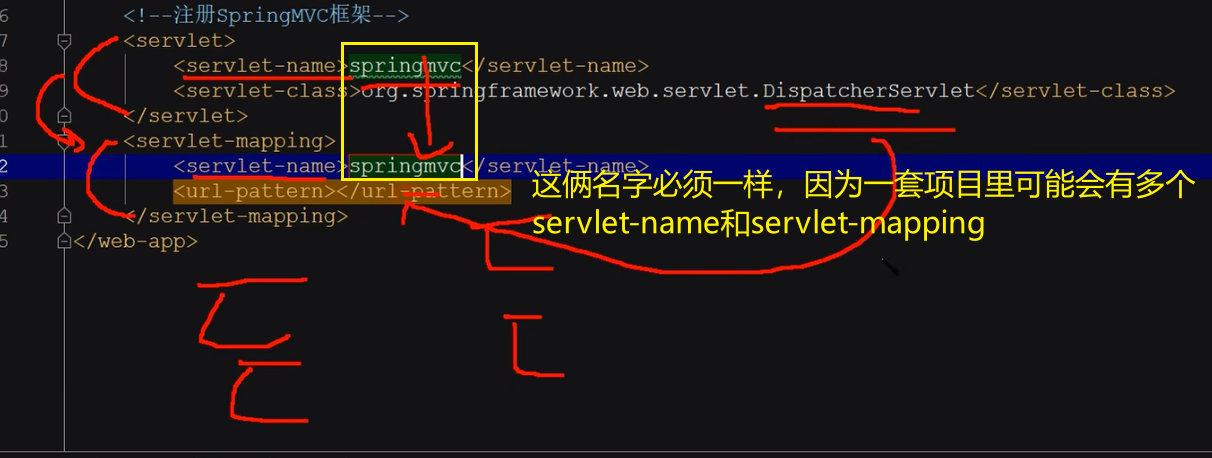
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!-- 注册SpringMVC框架 -->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.action</url-pattern>
</servlet-mapping>
</web-app>
三、项目发布
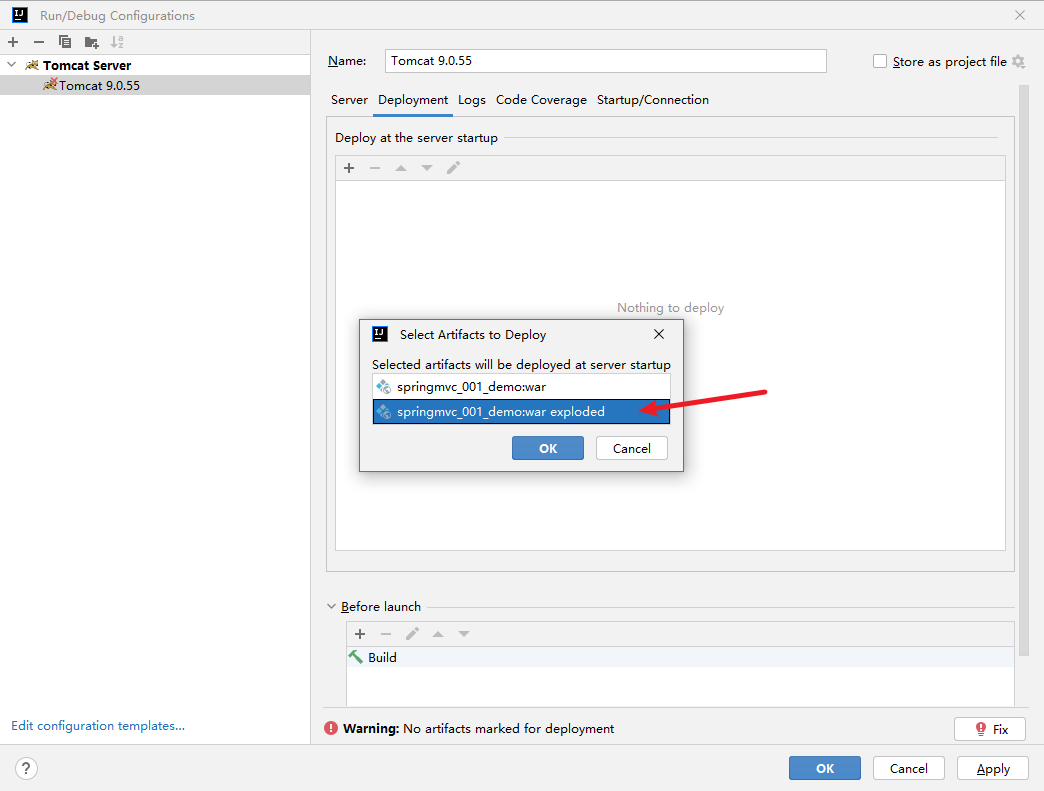
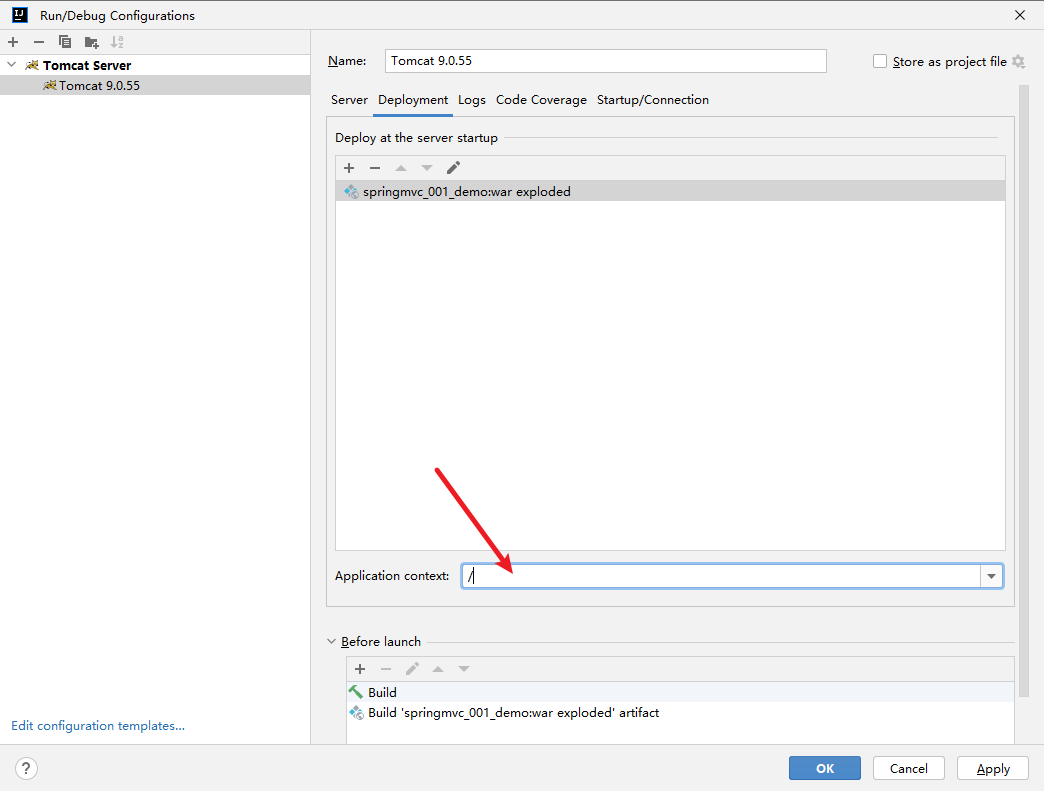
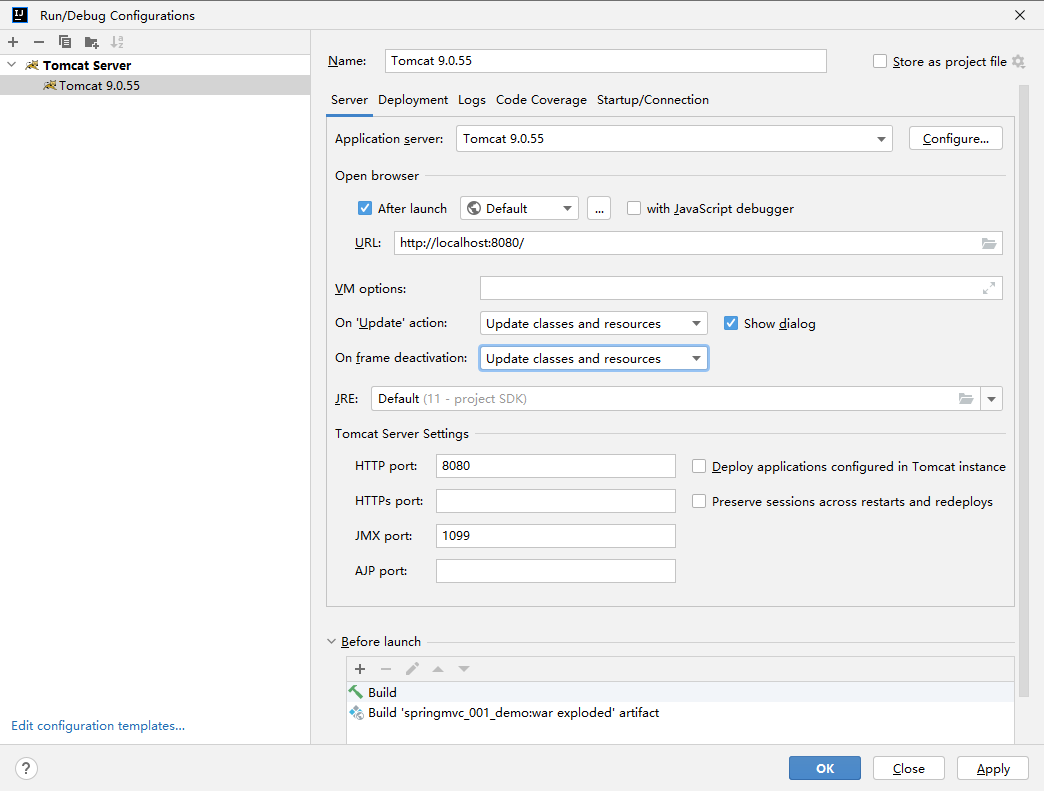
index.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%--${pageContext.request.contextPath}的意思是/项目名称,比如这个项目:/springmvc_001_demo,这个/表示http://localhost:8080/--%>
<a href="${pageContext.request.contextPath}/demo.action">访问服务器</a>
<a>${pageContext.request.contextPath}</a>
</body>
</html>
main.jsp
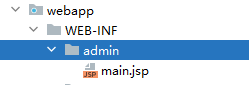
controller1.java
package com.itheima.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class controller1 {
@RequestMapping("/demo")
public String demo() {
System.out.println("服务器访问到了");
return "main";
}
}
这里加上springmvc之后

跳转之后:
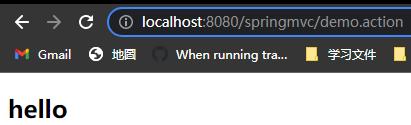
四、springmvc项目搭建流程再来一遍
创建javaweb工程
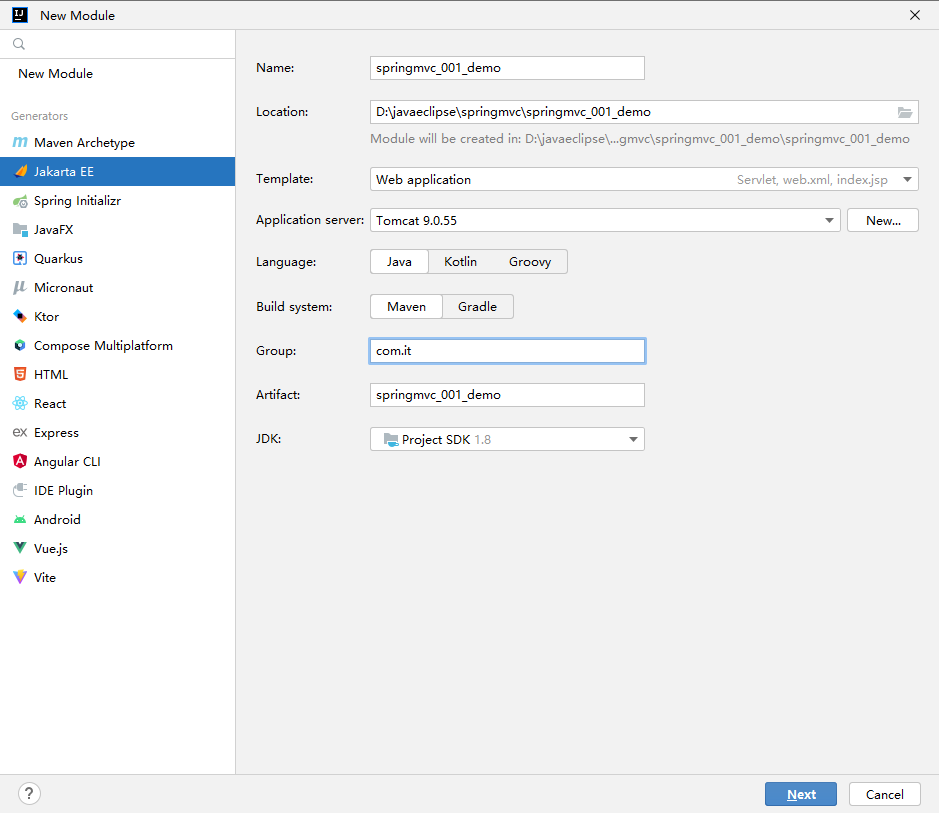
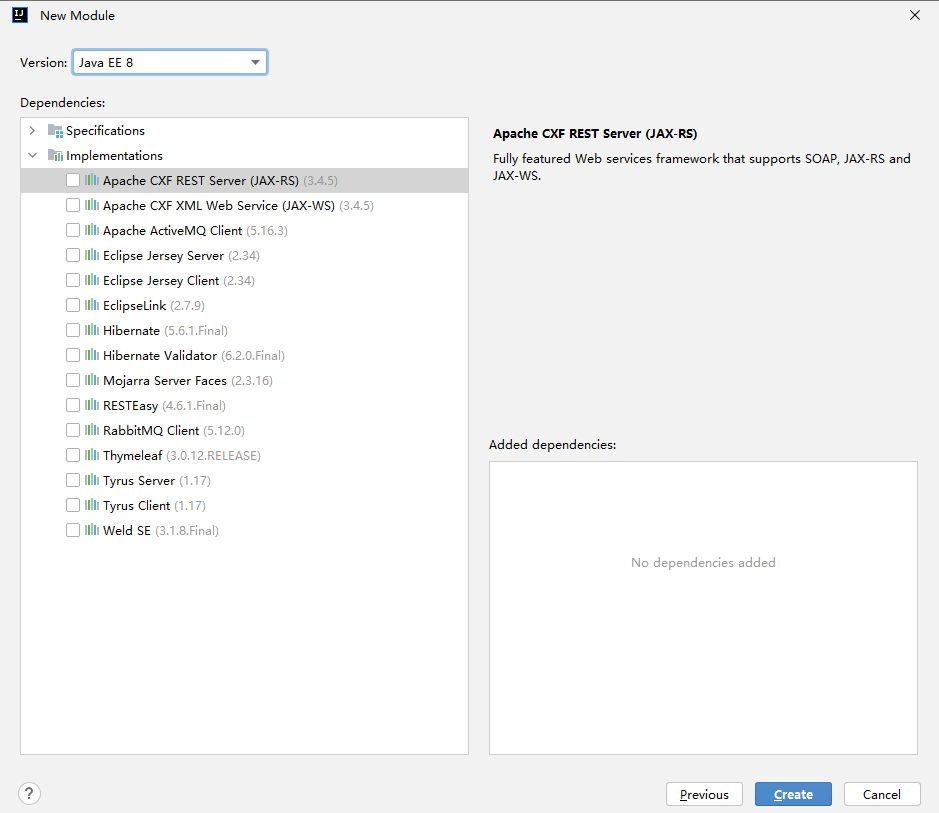
或者建Maven项目也可以,总之,下面这个目录结构不能错
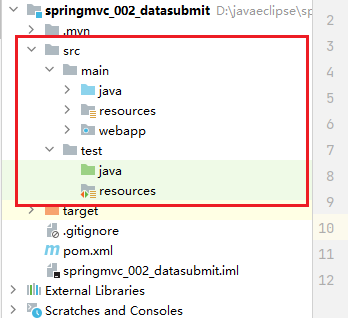
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.itheima</groupId>
<artifactId>springmvc_002_datasubmit</artifactId>
<version>1.0-SNAPSHOT</version>
<name>springmvc_002_datasubmit</name>
<packaging>war</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.source>1.8</maven.compiler.source>
<junit.version>5.9.1</junit.version>
</properties>
<dependencies>
<!--servlet依赖-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
<scope>provided</scope>
</dependency>
<!--springmvc依赖-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency> </dependencies>
<build>
<finalName>maven.plugins</finalName>
<pluginManagement>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.1</version>
</plugin>
</plugins>
</pluginManagement>
<resources>
<resource>
<directory>${basedir}/src/main/java</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
<resource>
<directory>${basedir}/src/main/resources</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</build>
</project>
resources/springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!--包扫描-->
<context:component-scan base-package="com.itheima.controller"></context:component-scan>
<!--视图解析器-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/admin/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
</beans>
webapp/WEB-INF/web.xml 注册springmvc框架
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--springmvc注册-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping><!--根据请求的路由,查询是否有处理请求的方法(根据@RequestMapping)-->
<servlet-name>springmvc</servlet-name>
<url-pattern>*.action</url-pattern>
</servlet-mapping>
<!--springmvc注册-->
</web-app>
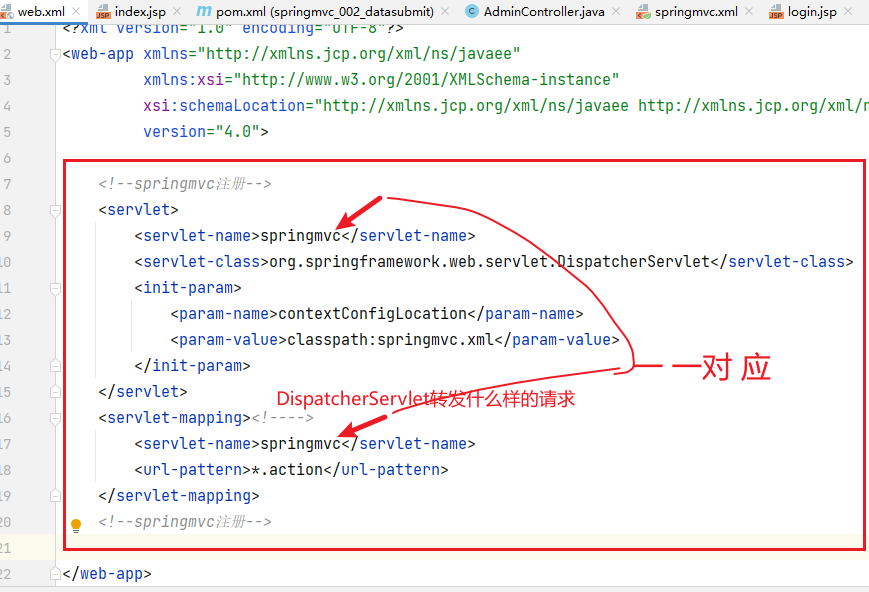
五、数据提交方式
1、form表单提交
DataController.java
package com.itheima.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
/**
* Description:
* date: 2023/6/8 21:40
* 参数名与jsp传入的变量名要一致
* @since JDK 11
*/
@Controller
public class DataController {
@RequestMapping(value = "/one")
public String dataSubmit(String name, String pw){
System.out.printf(name+" "+pw);
return "login";
}
}
index.jsp
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<br/>
<form action="${pageContext.request.contextPath}/one.action">
name:<input type="text" name="name"><br/>
password:<input type="password" name="pw"><br/>
<input type="submit" value="提交">
</form>
</body>
</html>
login.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/6/7
Time: 22:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h2>hello</h2>
</body>
</html>
2、对象封装提交数据
index.jsp
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<br/>
<form action="${pageContext.request.contextPath}/one.action">
name:<input type="text" name="name"><br/>
password:<input type="password" name="pw"><br/>
<input type="submit" value="提交">
</form>
<h3>封装成实体类进行数据的提交</h3>
<%--要求name的属性值与实体类对象的成员变量的值相同--%>
<form action="${pageContext.request.contextPath}/two.action" method="get">
name:<input type="text" name="name"><br/>
age:<input type="text" name="age"><br/>
<input type="submit" value="提交">
</form>
</body>
</html>
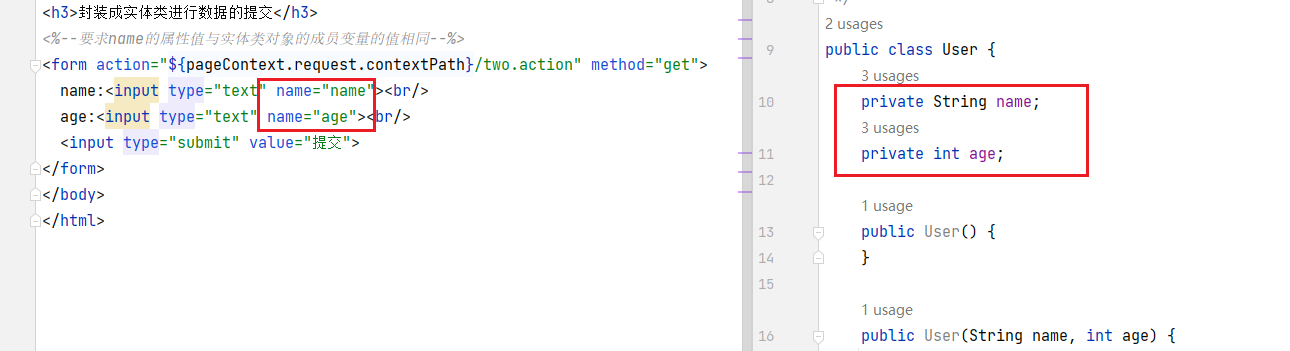
必须一致
实体类:User.java,需要有get和set方法
package com.itheima.domain;
/**
* Description:
* date: 2023/6/8 22:02
*
* @since JDK 11
*/
public class User {
private String name;
private int age;
public User() {
}
public User(String name, int age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
DataController.java
package com.itheima.controller;
import com.itheima.domain.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.RequestMapping;
/**
* Description:
* date: 2023/6/8 21:40
* 参数名与jsp传入的变量名要一致
* @since JDK 11
*/
@Controller
public class DataController {
@RequestMapping(value = "/one")
public String dataSubmit(String name, String pw){
System.out.printf(name+" "+pw);
return "login";
}
@RequestMapping(value = "/two")
public String dataSubmit2(User user){
System.out.printf(user.getName()+","+user.getAge());
return "login";
}
}
login.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/6/7
Time: 22:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h2>hello</h2>
</body>
</html>
3、动态占位符提交
1/1值,1/1{}
index.jsp
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<h3>3、动态占位符提交</h3>
<%--1个/1个值,1/一个{}--%>
<a href="${pageContext.request.contextPath}/three/张三/18.action">1/1值</a>
</body>
</html>
DataController.java
package com.itheima.controller;
import com.itheima.domain.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
/**
* Description:
* date: 2023/6/8 21:40
* 参数名与jsp传入的变量名要一致
* @since JDK 11
*/
@Controller
public class DataController {
@RequestMapping(value = "/one")
public String dataSubmit(String name, String pw){
System.out.printf(name+" "+pw);
return "login";
}
@RequestMapping(value = "/two")
public String dataSubmit2(User user){
System.out.printf(user.getName()+","+user.getAge());
return "login";
}
//3、动态占位符,1/1值,1/1{}
@RequestMapping(value = "/three/{name}/{age}")
public String dataSubmit3(@PathVariable String name, @PathVariable Integer age){
System.out.printf(name +","+age);
return "login";
}
@RequestMapping(value = "/three/{name2}/{age2}")
public String dataSubmit4(@PathVariable("name2") String name, @PathVariable("age2") Integer age){
System.out.printf(name +","+age);
return "login";
}
}
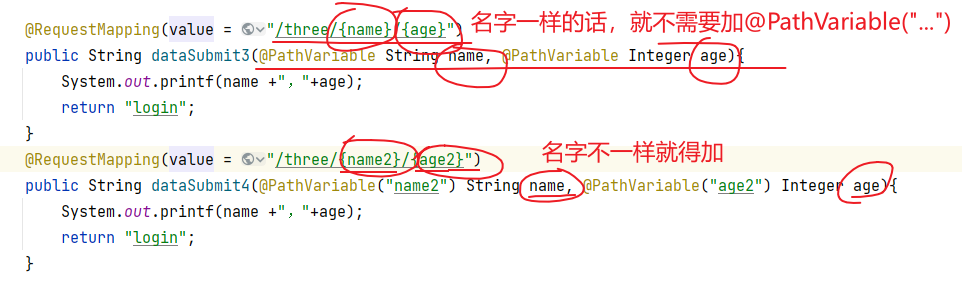
4、参数名称不一致
index.jsp
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<h3>4、参数名称不一致解决方案</h3>
<%--1个/1个值,1/一个{}--%>
<form action="${pageContext.request.contextPath}/four.action">
name:<input type="text" name="name"><br/>
age:<input type="text" name="age"><br/>
<input type="submit" value="提交">
</form>
</body>
</html>
controller.java
@RequestMapping(value = "/four")
public String dataSubmit4(@RequestParam("name") String name4,@RequestParam("age") Integer age4){
System.out.printf(name4 +","+age4);
return "login";
}
login.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/6/7
Time: 22:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h2>hello</h2>
</body>
</html>
5、原始方法,手动提取
index.jsp
<%@ page contentType="text/html; charset=UTF-8" pageEncoding="UTF-8" %>
<!DOCTYPE html>
<html>
<head>
<title>JSP - Hello World</title>
</head>
<body>
<h1><%= "Hello World!" %></h1>
<br/>
<h3>5、原始方法</h3>
<form action="${pageContext.request.contextPath}/five.action">
name:<input type="text" name="name"><br/>
age:<input type="text" name="age"><br/>
<input type="submit" value="提交">
</form>
</body>
</html>
DataController.java
package com.itheima.controller;
import com.itheima.domain.User;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpServletRequest;
@Controller
public class DataController {
@RequestMapping("/five")
public String dataSubmit5(HttpServletRequest httpServletRequest){
String name = httpServletRequest.getParameter("name");
Integer age = Integer.parseInt(httpServletRequest.getParameter("age"));
System.out.printf("name"+","+age);
return "login";
}
}
login.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/6/7
Time: 22:25
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>Title</title>
</head>
<body>
<h2>hello</h2>
</body>
</html>
六、中文编码设置
在发送post请求时,输入汉字,会发生乱码
配置过滤器
中文编码过滤器靠前放
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--中文乱码解决配置-->
<filter>
<filter-name>encode</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<!-- 源码中3个成员变量 ,默认false不编码
private String encoding;
private boolean forceRequestEncoding = false;
private boolean forceResponseEncoding = false;
-->
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceRequestEncoding</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encode</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--中文乱码解决配置-->
<!--springmvc注册-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping><!--根据请求的路由,查询是否有处理请求的方法(根据@RequestMapping)-->
<servlet-name>springmvc</servlet-name>
<url-pattern>*.action</url-pattern>
</servlet-mapping>
<!--springmvc注册-->
</web-app>
七、处理器controller返回值
1、String
客户端资源的地址,自动拼接前缀和后缀,还可以屏蔽自动拼接字符串,可以指定返回的路径
2、Object
返回json格式的对象,自动将对象或集合转为json。需要用到jackson工具进行转换,必须要添加jackson依赖,一般用于Ajax请求。
3、void
无返回值,一般用于ajax请求。
4、基本数据类型,用于ajax请求
5、ModelAndView
返回数据和视图对象,现在很少用了。
八、ajax请求
1、流程
完成ajax请求,访问服务器,返回学生集合。
1、添加jackson依赖
2、在webapp目录下新建js目录,添加jQuery函数库
3、在index.jsp页面上导入函数库
4、在action上添加注解@ResponseBody,用来处理ajax请求
5、在springmvc.xml文件中添加注解驱动<mvc:annotationdriven>,它用来解析@ResponseBody
2、配置
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.itheima</groupId>
<artifactId>springmvc_003_ajax</artifactId>
<version>1.0-SNAPSHOT</version>
<name>springmvc_003_ajax</name>
<packaging>war</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.source>1.8</maven.compiler.source>
<junit.version>5.9.1</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<!--servlet依赖-->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>3.1.0</version>
</dependency>
<!--springmvc依赖-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
<!--jackson,json转换工具-->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.9.8</version>
</dependency>
</dependencies>
<!--别忘了build-->
<build>
<resources>
<resource>
<directory>${basedir}/src/main/java</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
<resource>
<directory>${basedir}/src/main/resources</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
</build>
</project>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd http://www.springframework.org/schema/mvc https://www.springframework.org/schema/mvc/spring-mvc.xsd">
<!--包扫描-->
<context:component-scan base-package="com.itheima.controller"></context:component-scan>
<!--不需要视图解析器,因为处理的是ajax请求-->
<!-- <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/ajax/"/>
<property name="suffix" value=".jsp"/>
</bean>-->
<!--必须要添加注解驱动,使@RespondBody生效-->
<mvc:annotation-driven></mvc:annotation-driven>
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--添加中文编码解决方案-->
<filter>
<filter-name>encode</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<!--
private String encoding;
private boolean forceRequestEncoding = false;
private boolean forceResponseEncoding = false;
-->
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceRequestEncoding</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encode</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--添加中文编码解决方案-->
<!--注册springmvc框架-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>*.action</url-pattern>
</servlet-mapping>
<!--注册springmvc框架-->
</web-app>
九、ajax请求返回json数组功能实现
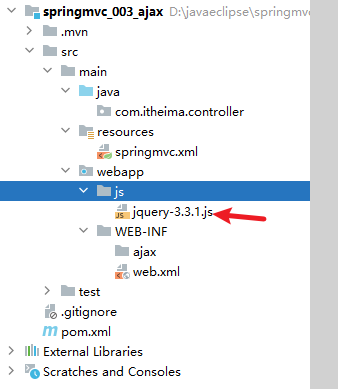
目录结构,导入jQuery文件
前面有许多内容没有记笔记,是在company的电脑上完成的。
P45登陆业务实现
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.powerpoint</groupId>
<artifactId>springmvc_005_webinf</artifactId>
<version>1.0-SNAPSHOT</version>
<name>springmvc_005_webinf</name>
<packaging>war</packaging>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.source>1.8</maven.compiler.source>
<junit.version>5.9.1</junit.version>
</properties>
<dependencies>
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>javax.servlet-api</artifactId>
<version>4.0.1</version>
<scope>provided</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-engine</artifactId>
<version>${junit.version}</version>
<scope>test</scope>
</dependency>
<!--配置springmvc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.5.RELEASE</version>
</dependency>
</dependencies>
<!--配置资源文件-->
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.xml</include>
<include>**/*.properties</include>
</includes>
</resource>
</resources>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.2</version>
</plugin>
</plugins>
</build>
</project>
springmvc.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context https://www.springframework.org/schema/context/spring-context.xsd">
<!--添加包扫描-->
<context:component-scan base-package="com.powerpoint.controller"></context:component-scan>
<!--添加视图解析器-->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<!--配置前缀-->
<property name="prefix" value="/WEB-INF/jsp/"/>
<!--配置后缀-->
<property name="suffix" value=".jsp"/>
</bean>
</beans>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
version="4.0">
<!--中文编码过滤器-->
<filter>
<filter-name>encode</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<!-- private String encoding;
private boolean forceRequestEncoding = false;
private boolean forceResponseEncoding = false;-->
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceRequestEncoding</param-name>
<param-value>true</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>encode</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<!--注册springmvc.xml-->
<servlet>
<servlet-name>springmvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
</servlet>
<servlet-mapping>
<servlet-name>springmvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
webapp/WEB-INF/jsp/login.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/7/4
Time: 21:41
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>登录</title>
</head>
<body>
<form action="${pageContext.request.contextPath}/loginShow" method="post">
姓名:<input type="text" name="name">
<br>
密码:<input type="password" name="pwd">
<br>
<input type="submit">
${msg}
</form>
</body>
</html>
webapp/WEB-INF/jsp/main.jsp
<%--
Created by IntelliJ IDEA.
User: xiaoshiguang
Date: 2023/7/4
Time: 21:57
To change this template use File | Settings | File Templates.
--%>
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>main</title>
</head>
<body>
用户名:${name}<br>
密码:${pwd}
</body>
</html>
controller/WebInfAction.java
package com.powerpoint.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpServletRequest;
/**
* Description:
* date: 2023/7/4 21:52
*
* @since JDK 11
*/
@Controller
public class WebInfAction {
@RequestMapping("/login")
public String login(){
System.out.println("请求转发到login页面");
return "login";
}
@RequestMapping("loginShow")
public String loginShow(String name,String pwd,HttpServletRequest request){
if("yyds".equalsIgnoreCase(name) && "yyds".equalsIgnoreCase(pwd)){
request.setAttribute("name",name);
request.setAttribute("pwd",pwd);
return "main";
}
String msg = "用户名或密码错误";
request.setAttribute("msg",msg);
return "login";
}
}
登陆业务的实现,隐藏了.jsp,安全性提高,只能访问webapp下的index.jsp,通过在springmvc.xml的视图解析器的修改以及在controller层的路径修改,实现在页面输出localhost:8080/login,就可以跳转到login.jsp页面