目录
- 一、commons-lang包下的StrSubstitutor
- 1、使用场景一
- 2、使用场景二
- 二、hutoo包下的StrUtil
- 1、使用场景一
- 2、使用场景二
- 3、使用场景三
- 三、原生的三种方式
一、commons-lang包下的StrSubstitutor
StrSubstitutor
是commons-lang
包提供的一个字符串替换类,整体使用体验还是非常不错的。commons-lang
包很多框架都有引用,很容易通过依赖传递到我们项目,所以有时候会出现我们根本没有引用这个包,但是却可以使用他包下的类。
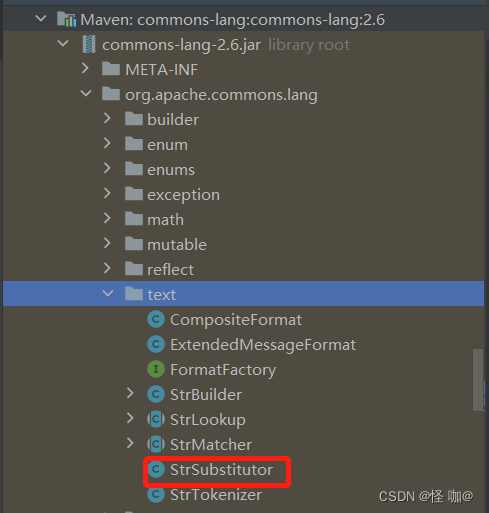
1、使用场景一
public static void main(String[] args) {
HashMap<String, String> objectObjectHashMap = new HashMap<>();
objectObjectHashMap.put("name","张三");
StrSubstitutor strSubstitutor = new StrSubstitutor(objectObjectHashMap);
// hello在map当中不存在,所以直接原样输出了
String replace = strSubstitutor.replace("${name}先生,${hello}");
System.out.println(replace);
}
运行结果:
2、使用场景二
默认是通过${}
来做字符串替换的,我们可以修改符号。
StrSubstitutor strSubstitutor = new StrSubstitutor(objectObjectHashMap, "%(", ")");
public static void main(String[] args) {
HashMap<String, String> objectObjectHashMap = new HashMap<>();
objectObjectHashMap.put("name", "张三");
StrSubstitutor strSubstitutor = new StrSubstitutor(objectObjectHashMap, "%(", ")");
// hello在map当中不存在,所以直接原样输出了
String replace = strSubstitutor.replace("%(name)先生,${hello:'我的 '}");
System.out.println(replace);
}
运行结果
二、hutoo包下的StrUtil
再有就是我们也可以通过hutoo
工具类当中的StrUtil
来完成字符串的替换,hutoo可以说是近期非常火的工具类了,基本上项目都会引用他。
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.20</version>
</dependency>
1、使用场景一
public static void main(String[] args) {
String str = "你好,{},我的大宝贝,{}";
String format = StrUtil.format(str, "张三", "哈哈");
System.out.println(format);
}
运行结果
2、使用场景二
这个跟上面的StrSubstitutor相比又简单了些,而且都是通过map来映射参数。
public static void main(String[] args) {
String str = "你好,{name},我的大宝贝,{aaa}";
HashMap<String, String> objectObjectHashMap = new HashMap<>();
objectObjectHashMap.put("name","张三");
String format = StrUtil.format(str, objectObjectHashMap);
System.out.println(format);
}
运行结果
3、使用场景三
假如key为null,会原样返回对应的变量
public static void main(String[] args) {
String str = "你好,{name},我的大宝贝,{aaa}";
HashMap<String, String> objectObjectHashMap = new HashMap<>();
objectObjectHashMap.put("name", "张三");
objectObjectHashMap.put("aaa", null);
// 默认ignoreNull这个参数就是为true
String format = StrUtil.format(str, objectObjectHashMap, true);
System.out.println(format);
}
运行结果:
设置ignoreNull为false就是忽略null。
public static void main(String[] args) {
String str = "你好,{name},我的大宝贝,{aaa}";
HashMap<String, String> objectObjectHashMap = new HashMap<>();
objectObjectHashMap.put("name", "张三");
objectObjectHashMap.put("aaa", null);
String format = StrUtil.format(str, objectObjectHashMap, false);
System.out.println(format);
}
运行结果
三、原生的三种方式
以上两种都是基于了第三方jar,下面这几种方式都是不需要任何依赖的,都是Java自带的。
public static void main(String[] args) {
// 方式一
String format = String.format("你好,%s,我的大宝贝,%s", "张三", "李四");
System.out.println(format);
// 方式二
String format1 = MessageFormat.format("你好,{0},我的大宝贝,{1}", "张三", "李四");
System.out.println(format1);
// 方式三
String replace = "你好,{0},我的大宝贝,{1}".replace("{0}", "张三").replace("{1}", "李四");
System.out.println(replace);
}
运行结果