目录
框架:
编程思维:
MVC架构:
前端——组件式开发
开发思路梳理:
后端——
前端——
效果图
信息列表:
修改用户编辑
新增用户
删除用户
数据清空
批量上传
框架:
后端:JAVA-SpringBoot2.6、包管理器Maven3.6、JDK1.8
数据:MySQL5.7、可视化工具Navicat
前端:Vue2.x、Element-ui3.x、jqurey、axios
编程思维:
后端——自底向上思想:
数据表——》实体层(pojo/entity):实体类——》映射层(dao/mapper):编写数据库连接接口和mapper.xml(使用Mybatis语句连接数据库)——》业务层(service):Service接口和ServiceImpl实现类——》控制层(Controller):Controller类
MVC架构:
M——模型/数据
V——视图/页面
C——控制/数据页面
前端——组件式开发
重要知识点
VUE的基本语法
(Components)组件式开发
(Router)路由转发
(Axios)网络请求
(VueX)状态管理
开发思路梳理:
后端——
创建数据库、数据表
后端连接数据库
进行数据映射
实体类
Mybatis——》数据库映射
Mapper接口(Dao/mapper)
业务层接口与实现
接口中定义抽象函数
实现基本上就是调用Dao层方法
控制层
控制类
前端——
vue支持组件式开发,每个组件基本上都有三大模块组成:<template/>、<scripts/>、<style/>
基本语法不过多说,重点在于Router(路由配置)和Axios(网络请求)。
路由配置时注意引入组件,
按格式设置子路由。
import Vue from 'vue'
import Router from 'vue-router'
Vue.use(Router)
/* Layout */
import Layout from '@/layout'
/**
* Note: sub-menu only appear when route children.length >= 1
* Detail see: https://panjiachen.github.io/vue-element-admin-site/guide/essentials/router-and-nav.html
*
* hidden: true if set true, item will not show in the sidebar(default is false)
* alwaysShow: true if set true, will always show the root menu
* if not set alwaysShow, when item has more than one children route,
* it will becomes nested mode, otherwise not show the root menu
* redirect: noRedirect if set noRedirect will no redirect in the breadcrumb
* name:'router-name' the name is used by <keep-alive> (must set!!!)
* meta : {
roles: ['admin','editor'] control the page roles (you can set multiple roles)
title: 'title' the name show in sidebar and breadcrumb (recommend set)
icon: 'svg-name'/'el-icon-x' the icon show in the sidebar
breadcrumb: false if set false, the item will hidden in breadcrumb(default is true)
activeMenu: '/example/list' if set path, the sidebar will highlight the path you set
}
*/
/**
* constantRoutes
* a base page that does not have permission requirements
* all roles can be accessed
*/
export const constantRoutes = [
{
path: '/login',
component: () => import('@/views/login/index'),
hidden: true
},
{
path: '/404',
component: () => import('@/views/404'),
hidden: true
},
{
path: '/',
component: Layout,
redirect: '/dashboard',
children: [{
path: 'dashboard',
name: 'Dashboard',
component: () => import('@/views/dashboard/index'),
meta: { title: 'Dashboard', icon: 'dashboard' }
}]
},
{
path: '/user',
component: Layout,
redirect: '/user',
name: 'User',
meta: { title: 'User', icon: 'el-icon-s-help' },
children: [
{
path: 'list',
name: 'List',
component: () => import('@/views/user/userlist'),
meta: { title: 'User', icon: 'table' }
}
]
},
{
path: '/userupload',
component: Layout,
redirect: '/userupload',
name: 'Upload',
meta: { title: 'Upload', icon: 'el-icon-s-help' },
children: [
{
path: 'userupload',
name: 'Upload',
component: () => import('@/views/upload/index'),
meta: { title: 'Upload', icon: 'table' }
}
]
},
{
path: '/example',
component: Layout,
redirect: '/example/table',
name: 'Example',
meta: { title: 'Example', icon: 'el-icon-s-help' },
children: [
{
path: 'table',
name: 'Table',
component: () => import('@/views/table/index'),
meta: { title: 'Table', icon: 'table' }
},
{
path: 'tree',
name: 'Tree',
component: () => import('@/views/tree/index'),
meta: { title: 'Tree', icon: 'tree' }
}
]
},
{
path: '/form',
component: Layout,
children: [
{
path: 'index',
name: 'Form',
component: () => import('@/views/form/index'),
meta: { title: 'Form', icon: 'form' }
}
]
},
{
path: '/nested',
component: Layout,
redirect: '/nested/menu1',
name: 'Nested',
meta: {
title: 'Nested',
icon: 'nested'
},
children: [
{
path: 'menu1',
component: () => import('@/views/nested/menu1/index'), // Parent router-view
name: 'Menu1',
meta: { title: 'Menu1' },
children: [
{
path: 'menu1-1',
component: () => import('@/views/nested/menu1/menu1-1'),
name: 'Menu1-1',
meta: { title: 'Menu1-1' }
},
{
path: 'menu1-2',
component: () => import('@/views/nested/menu1/menu1-2'),
name: 'Menu1-2',
meta: { title: 'Menu1-2' },
children: [
{
path: 'menu1-2-1',
component: () => import('@/views/nested/menu1/menu1-2/menu1-2-1'),
name: 'Menu1-2-1',
meta: { title: 'Menu1-2-1' }
},
{
path: 'menu1-2-2',
component: () => import('@/views/nested/menu1/menu1-2/menu1-2-2'),
name: 'Menu1-2-2',
meta: { title: 'Menu1-2-2' }
}
]
},
{
path: 'menu1-3',
component: () => import('@/views/nested/menu1/menu1-3'),
name: 'Menu1-3',
meta: { title: 'Menu1-3' }
}
]
},
{
path: 'menu2',
component: () => import('@/views/nested/menu2/index'),
name: 'Menu2',
meta: { title: 'menu2' }
}
]
},
{
path: 'external-link',
component: Layout,
children: [
{
path: 'https://panjiachen.github.io/vue-element-admin-site/#/',
meta: { title: 'External Link', icon: 'link' }
}
]
},
// 404 page must be placed at the end !!!
{ path: '*', redirect: '/404', hidden: true }
]
const createRouter = () => new Router({
// mode: 'history', // require service support
scrollBehavior: () => ({ y: 0 }),
routes: constantRoutes
})
const router = createRouter()
// Detail see: https://github.com/vuejs/vue-router/issues/1234#issuecomment-357941465
export function resetRouter() {
const newRouter = createRouter()
router.matcher = newRouter.matcher // reset router
}
export default router
网络请求时:
注意:数据绑定、双向绑定、获取一行的数据、网络请求传参……
<template>
<div>
<el-table :data="tableData" style="width: 100%" max-height="250">
<el-table-column prop="id" label="用户ID" width="300"> </el-table-column>
<el-table-column prop="account" label="用户账号" width="300">
</el-table-column>
<el-table-column fixed prop="username" label="用户姓名" width="100">
</el-table-column>
<el-table-column prop="password" label="用户密码" width="300">
</el-table-column>
<el-table-column fixed prop="address" label="用户住址" width="100">
</el-table-column>
<el-table-column fixed="right" label="操作" width="120">
<template slot-scope="scope">
<!-- {{scope.row.id}} -->
<el-button @click.native.prevent="update_(scope.row)" type="text" size="small">
修改
</el-button>
<el-button @click.native.prevent="delete_(scope.row.id)" type="text" size="small">
删除
</el-button>
</template>
</el-table-column>
</el-table>
<el-row style="float: right">
<el-button
@click.native.prevent="add()"
type="text"
>新增数据</el-button
>
<el-button
@click.native.prevent="removeAll()"
type="text"
>数据清空</el-button
>
</el-row>
<!-- 新增弹窗-->
<el-dialog
title="新增用户"
:visible.sync="dialogVisible_add"
width="30%">
<el-form ref="form" :model="user" label-width="80px">
<el-form-item label="用户账号">
<el-input v-model="user.account"></el-input>
</el-form-item>
<el-form-item label="用户姓名">
<el-input v-model="user.username"></el-input>
</el-form-item>
<el-form-item label="用户密码">
<el-input v-model="user.password"></el-input>
</el-form-item>
<el-form-item label="用户地址">
<el-input v-model="user.address"></el-input>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible_add = false">取 消</el-button>
<el-button type="primary" @click="save_add">确 定</el-button>
</span>
</el-dialog>
<!-- 修改弹窗-->
<el-dialog
title="修改用户"
:visible.sync="dialogVisible_up"
width="30%">
<el-form ref="form" :model="user" label-width="80px">
<el-form-item label="用户账号">
<el-input v-model="user.account"></el-input>
</el-form-item>
<el-form-item label="用户姓名">
<el-input v-model="user.username"></el-input>
</el-form-item>
<el-form-item label="用户密码">
<el-input v-model="user.password"></el-input>
</el-form-item>
<el-form-item label="用户地址">
<el-input v-model="user.address"></el-input>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible_up = false">取 消</el-button>
<el-button type="primary" @click="save_up">确 定</el-button>
</span>
</el-dialog>
</div>
</template>
<script>
import axios from 'axios'
export default {
data() {
return {
tableData: [], // 数据列表
dialogVisible_add: false, // 新增弹窗控制器
dialogVisible_up: false, // 新增弹窗控制器
user: { // 数据封装
account: '',
username: '',
password: '',
address: ''
}
}
},
created: function() {
this.loadTableData()
},
methods: {
loadTableData() {
axios.get('http://localhost:8088/queryAll').then((response) => {
this.tableData = response.data
})
},
update_(row) {
this.dialogVisible_up = true
this.user = row
},
delete_(id) {
// console.log(this.user)
const data = JSON.stringify(this.user)
console.log(data)
if (confirm('是否删除此数据?')) {
axios.get('http://localhost:8088/remove?id=' + id).then((response) => {
this.loadTableData()
location.reload(0)
})
} else {
location.reload(0)
}
},
add() {
this.user = {}
this.dialogVisible_add = true
},
save_add() {
// 新增
axios.post('http://localhost:8088/add', this.user).then((response) => {
this.dialogVisible_add = false
this.loadTableData()
})
},
save_up() {
axios.post('http://localhost:8088/update', this.user).then((response) => {
this.dialogVisible_up = false
this.loadTableData()
})
},
removeAll() {
if (confirm('是否全部删除?')) {
axios.get('http://localhost:8088/removeAll').then((response) => {
console.log('已全部删除')
})
}
location.reload(0)
}
}
}
</script>
特殊说明:要想在vue中使用JQuery需要下载安装第三方依赖。
更多参考:【Java-SpringBoot+Vue+MySql】项目开发杂记_代码骑士的博客-CSDN博客
效果图
1、信息列表:
2、修改用户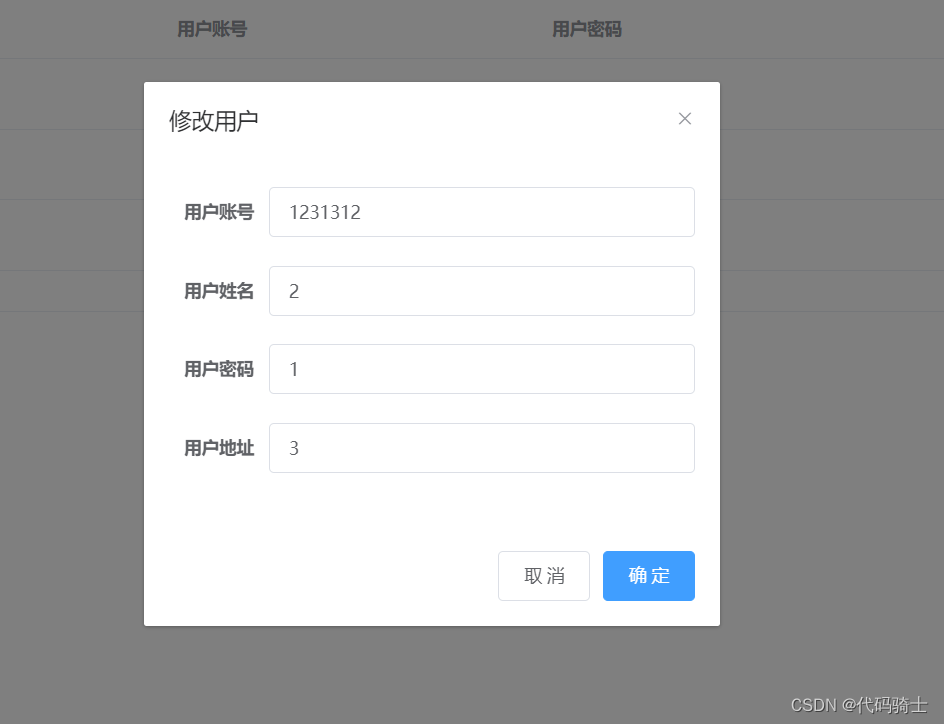
3、新增用户
4、删除用户
5、 数据清空
6、批量上传