CSS处理页面元素浮动的几个办法
- 不使用浮动的情况下,子盒子1和2分别在盒子1中占据一行的空间。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>未使用浮动</title>
<style>
.box1 {
height: 200px;
width: 600px;
background-color: pink;
}
.box2 {
width: 600px;
height: 240px;
background-color: purple;
}
.son1 {
width: 150px;
height: 100px;
background-color: skyblue;
}
.son2 {
width: 300px;
height: 100px;
background-color: hotpink;
}
</style>
</head>
<body>
<div class="box1">
<div class="son1"></div>
<div class="son2"></div>
</div>
<div class="box2"></div>
</body>
</html>
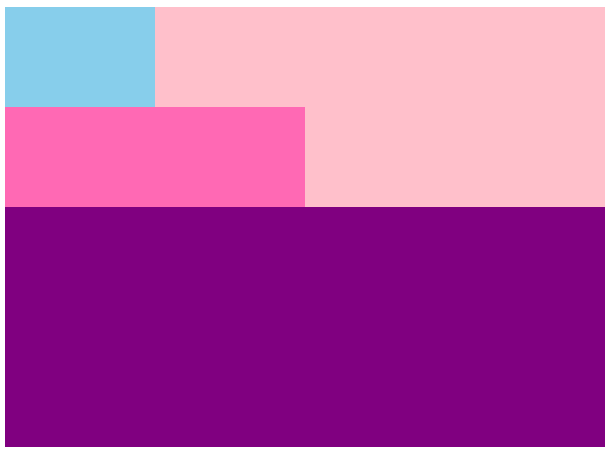
- 使用添加额外标签的方法清除浮动,子盒子1和2分别使用左浮动, 两个元素都不占用原来文档流的空间了,跑到了同一行,额外添加一个div标签,清除浮动,避免盒子2也随着向上占据盒子1的空间。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除浮动 - 额外标签</title>
<style>
.box1 {
width: 600px;
background-color: pink;
}
.box2 {
width: 600px;
height: 240px;
background-color: purple;
}
.son1 {
width: 150px;
height: 100px;
background-color: skyblue;
float: left;
}
.son2 {
width: 300px;
height: 100px;
background-color: hotpink;
float: left;
}
.clearfix {
clear: both;
}
</style>
</head>
<body>
<div class="box1">
<div class="son1"></div>
<div class="son2"></div>
<div class="clearfix"></div>
</div>
<div class="box2"></div>
</body>
</html>
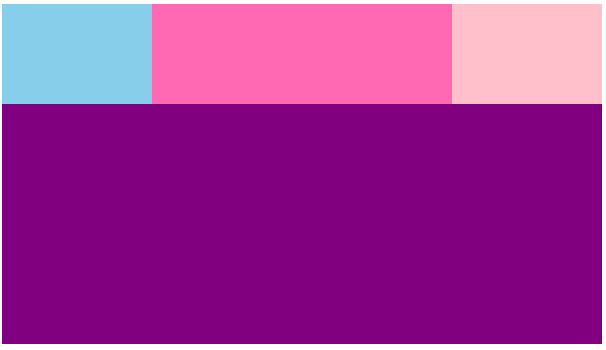
- 使用双伪元素的方式清除浮动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除浮动 - 双伪元素</title>
<style>
.box1 {
width: 600px;
background-color: pink;
}
.box2 {
width: 600px;
height: 240px;
background-color: purple;
}
.son1 {
width: 150px;
height: 100px;
background-color: skyblue;
float: left;
}
.son2 {
width: 300px;
height: 100px;
background-color: hotpink;
float: left;
}
.clearfix:before, .clearfix:after {
content: "";
display: table;
}
.clearfix:after {
clear: both;
}
</style>
</head>
<body>
<div class="box1 clearfix">
<div class="son1"></div>
<div class="son2"></div>
</div>
<div class="box2"></div>
</body>
</html>
- 使用after伪元素的方式清除浮动
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除浮动 - after伪元素</title>
<style>
.box1 {
width: 600px;
background-color: pink;
}
.box2 {
width: 600px;
height: 240px;
background-color: purple;
}
.son1 {
width: 150px;
height: 100px;
background-color: skyblue;
float: left;
}
.son2 {
width: 300px;
height: 100px;
background-color: hotpink;
float: left;
}
.clearfix:after {
content: ".";
display: block;
height: 0;
visibility: hidden;
clear: both;
}
.clearfix {
*zoom: 1;
}
</style>
</head>
<body>
<div class="box1 clearfix">
<div class="son1"></div>
<div class="son2"></div>
</div>
<div class="box2"></div>
</body>
</html>
- 使用父级元素的overflow属性清除浮动,盒子1设置overflow为hidden,触发BFC达到清除浮动的目的。
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清除浮动 - 父级overflow</title>
<style>
.box1 {
width: 600px;
background-color: pink;
overflow: hidden;
}
.box2 {
width: 600px;
height: 240px;
background-color: purple;
}
.son1 {
width: 150px;
height: 100px;
background-color: skyblue;
float: left;
}
.son2 {
width: 300px;
height: 100px;
background-color: hotpink;
float: left;
}
</style>
</head>
<body>
<div class="box1">
<div class="son1"></div>
<div class="son2"></div>
</div>
<div class="box2"></div>
</body>
</html>