目录
- 棋盘
- HTML+CSS版本
- HTML+CSS+JavaScript版本
- 电话拨号盘
- 红绿灯
棋盘
HTML+CSS版本
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>作业2591实现棋盘效果</title>
<style>
.container {
width: 600px;
height: 600px;
border: 5px solid black;
margin: auto;
display: flex;
}
.black {
background-color: black;
width: 200px;
height: 200px;
}
.white {
background-color: white;
width: 200px;
height: 200px;
}
</style>
</head>
<body>
<div class="container">
<div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
</div>
<div>
<div class="white"></div>
<div class="black"></div>
<div class="white"></div>
</div>
<div>
<div class="black"></div>
<div class="white"></div>
<div class="black"></div>
</div>
</div>
</body>
</html>
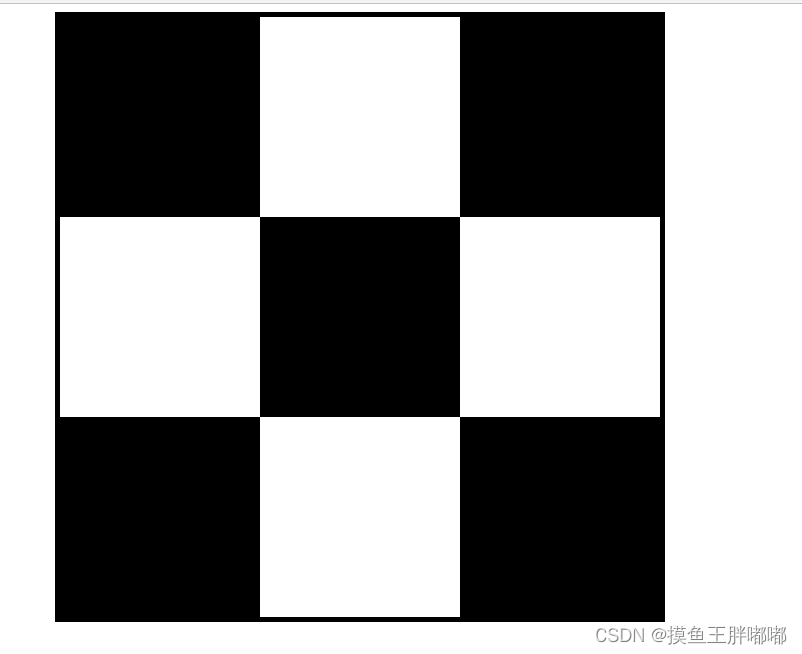
HTML+CSS+JavaScript版本
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>棋盘</title>
</head>
<body>
<style>
.parent-class {
border: 10px chocolate groove;
}
.containereven,
.containerodd {
display: flex;
background-color: dodgerblue;
}
.item {
background-color: #f1f1f1;
padding: 50px;
font-size: 30px;
flex: 1;
height: 50px;
text-align: center;
}
.containereven>div:nth-child(odd) {
background-color:black;
}
.containereven>div:nth-child(even) {
background-color: white;
}
.containerodd>div:nth-child(odd) {
background-color: white;
}
.containerodd>div:nth-child(even) {
background-color: black;
}
</style>
<div class="parent-class">
<section class="containereven" id="container1"></section>
<section class="containerodd" id="container2"></section>
<section class="containereven" id="container3"></section>
</div>
<!-- 使用 javascript 在相应的 id 的帮助下在这些部分标签内动态附加元素。 -->
<script>
let res1 = [],
res2 = [],
res3 = [];
for (let i = 1; i <= 3; i++) {
res1 += `<div class="item"></div>`;
res2 += `<div class="item"></div>`;
res3 += `<div class="item"></div>`;
}
document.getElementById('container1').innerHTML = res1;
document.getElementById('container2').innerHTML = res2;
document.getElementById('container3').innerHTML = res3;
</script>
</body>
</html>
在这里插入代码片
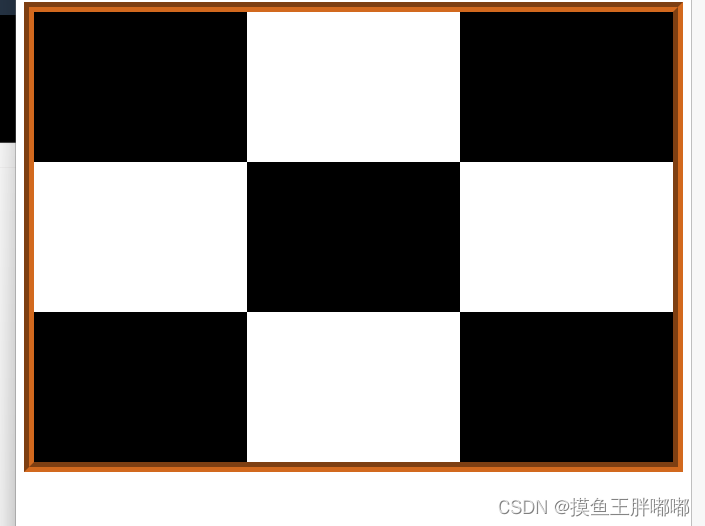
电话拨号盘
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>电话拨号盘</title>
</head>
<body>
<style>
#css1 {
text-decoration:underline;
color: red;
font-size: 17px;
}
#css2 {
text-decoration: line-through;
color: green;
font-size: 17px;
}
#css3 {
font-weight: bold;
color: blue;
font-size: 17px;
}
table {
border-collapse: collapse;
}
td {
width: 100px;
height: 40px;
border: 1px solid black;
font-size: 12px;
text-align: center;
}
</style>
<table>
<tr>
<td id="css1">1</td>
<td id="css1">2</td>
<td id="css1">3</td>
</tr>
<tr>
<td id="css2">4</td>
<td id="css2">5</td>
<td id="css2">6</td>
</tr>
<tr>
<td id="css3">7</td>
<td id="css3">8</td>
<td id="css3">9</td>
</tr>
</table>
</body>
</html>
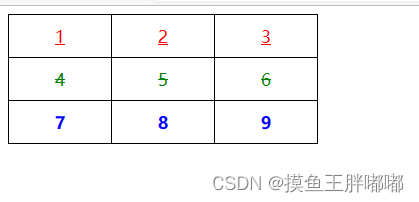
红绿灯
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>红绿灯</title>
</head>
<body>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
.parent {
width: 200px;
height: 600px;
margin: 0 auto;
background-color: gray;
padding: 25px;
}
.one,
.two,
.three {
width: 150px;
height: 150px;
border-radius: 50%;
}
.one {
background-color: red;
}
.two {
background-color: green;
margin-top: 50px;
}
.three {
background-color: yellow;
margin-top: 50px;
}
</style>
<div class="parent">
<div class="one"></div>
<div class="two"></div>
<div class="three"></div>
</div>
</body>
</html>
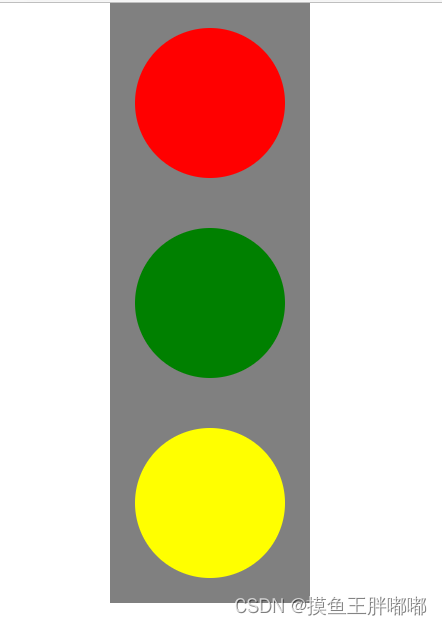