目录
一、引出异常
实例
运行结果
二、异常体系图
三、五大运行时异常
一、NullPointerException 空指针异常
二、ArithmeticException 数学运算异常
三、ArrayIndexOutOfBoundsException 数组下标越界异常
四、ClassCastException 类型转换异常
编辑五、 NumberFormatException 数字格式不正确异常
四、编译异常
三、练习
一、引出异常
实例
package com.hspedu.exception_;
/**
* @author GQQ
* @version 1.0
*/
public class Exception01 {
public static void main(String[] args) {
int num1 = 10;
int num2 = 0;
//1. num1 / num2 => 10 / 0
//2.当执行到 num1 / num2,因为 num2 = 0, 程序就会出现(抛出)异常,ArithmeticException
//3. 当抛出异常后,程序就退出,崩溃了,下面的代码就不在执行
//4.这样的程序好吗? 不好,不应该出现了一个不算致命的问题,就导致整个系统崩溃
//5. java 设计者提供了一个 异常处理机制 来解决该问题
//6.使用try-catch异常处理机制来结局可能出现的异常/问题
//7.选中可能出现异常的代码 ,ctrl + alt + t ,选中 6
try {
int res = num1 / num2;
} catch (Exception e) {
//throw new RuntimeException(e);//自动跳出的代码
e.printStackTrace();//老师的代码
}
System.out.println("程序继续运行....");
}
}
运行结果
也可以打印异常信息
二、异常体系图
Error是严重错误,不可挽回的,类似无法治疗的疾病
Exception是局部错误,还可以修改,即可以治疗康复的病
虚线是实现,实线是继承
编译异常
package com.hspedu.exception_;
import java.io.FileInputStream;
/**
* @author GQQ
* @version 1.0
*/
public class Exception02 {
public static void main(String[] args) {
//Throwable
FileInputStream fis;
fis = new FileInputStream("d:\\aa.jpg");
int len;
while ((len = fis.read()) != -1) {System.out.printin(len) :
fis.close();
}
}
是编译异常中的FileNotFoundException
可能是jdk版本不同,我的五大运行异常并没有归到RuntimeErrorException下面
三、五大运行时异常
一、NullPointerException 空指针异常
package com.hspedu.exception_;
/**
* @author GQQ
* @version 1.0
*/
public class NullPointerException_ {
public static void main(String[] args) {
String name = null;
System.out.println(name.length());
}
}
运行时提示空指针异常
二、ArithmeticException 数学运算异常
引出异常的案例就是数学运算异常,除数为0
三、ArrayIndexOutOfBoundsException 数组下标越界异常
package com.hspedu.exception_;
/**
* @author GQQ
* @version 1.0
*/
public class ArithmeticException_ {
public static void main(String[] args) {
int[] arr = {1,2,4};
//如果判断条件是i <= arr.length,当i=3时,输出arr[3],就会报下标越界
for (int i = 0; i <= arr.length; i++) {
System.out.println(arr[i]);
}
}
}
四、ClassCastException 类型转换异常
当试图将对象强制转换为不是实例的子类时, 抛出该异常。
package com.hspedu.exception_;
/**
* @author GQQ
* @version 1.0
*/
public class NumberFormatException_ {
public static void main(String[] args) {
String name = "java学习";
//数据类型转换,将String转换成int
//java学习 是无法转换成 数字的,运行是会抛出NumberFormatException
int num = Integer.parseInt(name);
System.out.println(num);
}
}
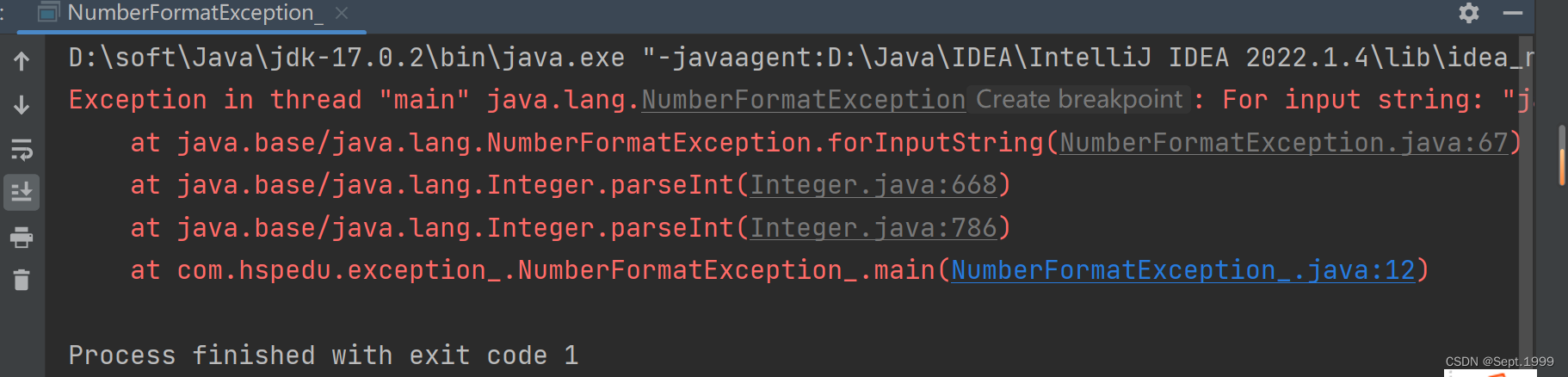
五、 NumberFormatException 数字格式不正确异常
当应用程序试图将字符串转换成一种数值类型, 但该字符串不能转换为适当格式时, 抛出该异常
package com.hspedu.exception_;
/**
* @author GQQ
* @version 1.0
*/
public class NumberFormatException_ {
public static void main(String[] args) {
String name = "java学习";
//数据类型转换,将String转换成int
//java学习 是无法转换成 数字的,运行是会抛出NumberFormatException
int num = Integer.parseInt(name);
System.out.println(num);
}
}
四、编译异常
编译异常指的是编译阶段出现的异常,是必须处理的异常
package com.hspedu.exception_;
import java.io.FileInputStream;
import java.io.IOException;
/**
* @author GQQ
* @version 1.0
*/
public class Exception02 {
public static void main(String[] args) {
//Throwable
try{
FileInputStream fis;
fis = new FileInputStream("d:\\aa.jpg");
int len;
while ((len = fis.read()) != -1) {
System.out.println(len);
}
fis.close();
}catch (IOException e) {
e.printStackTrace();
}
}
}
三、练习
分别是下标越界异常 ArrayIndexOutOfBoundsException 、
空指针异常 NullPointerException、
数学运算异常ArithmeticException(属性x为int型变量,其的默认值为0)、
类型转换异常 ClassCastException(Person类和Date类没有任何关系)