import sys
from PyQt5.QtWidgets import QApplication, QWidget,QVBoxLayout,QTextEdit,QPushButton,QHBoxLayout,QFileDialog,QLabel
class PromoteLabel(QLabel):
def __init__(self,parent = None):
super().__init__(parent)
self.setText("111111")
self.setStyleSheet("background-color:rgb(100,100,100);")
<?xml version="1.0" encoding="UTF-8"?>
<ui version="4.0">
<class>Form</class>
<widget class="QWidget" name="Form">
<property name="geometry">
<rect>
<x>0</x>
<y>0</y>
<width>520</width>
<height>431</height>
</rect>
</property>
<property name="windowTitle">
<string>Form</string>
</property>
<widget class="PromoteLabel" name="label">
<property name="geometry">
<rect>
<x>70</x>
<y>120</y>
<width>111</width>
<height>61</height>
</rect>
</property>
<property name="text">
<string/>
</property>
</widget>
<widget class="QPushButton" name="pushButton">
<property name="geometry">
<rect>
<x>260</x>
<y>200</y>
<width>75</width>
<height>23</height>
</rect>
</property>
<property name="text">
<string>PushButton</string>
</property>
</widget>
</widget>
<customwidgets>
<customwidget>
<class>PromoteLabel</class>
<extends>QLabel</extends>
<header>PromoteLabelTest</header>
</customwidget>
</customwidgets>
<resources/>
<connections/>
</ui>
# -*- coding: utf-8 -*-
# Form implementation generated from reading ui file 'c:\Users\481073\Desktop\new\test.ui'
#
# Created by: PyQt5 UI code generator 5.15.9
#
# WARNING: Any manual changes made to this file will be lost when pyuic5 is
# run again. Do not edit this file unless you know what you are doing.
from PyQt5 import QtCore, QtGui, QtWidgets
class Ui_Form(object):
def setupUi(self, Form):
Form.setObjectName("Form")
Form.resize(520, 431)
self.label = PromoteLabel(Form)
self.label.setGeometry(QtCore.QRect(70, 120, 111, 61))
self.label.setText("")
self.label.setObjectName("label")
self.pushButton = QtWidgets.QPushButton(Form)
self.pushButton.setGeometry(QtCore.QRect(260, 200, 75, 23))
self.pushButton.setObjectName("pushButton")
self.retranslateUi(Form)
QtCore.QMetaObject.connectSlotsByName(Form)
def retranslateUi(self, Form):
_translate = QtCore.QCoreApplication.translate
Form.setWindowTitle(_translate("Form", "Form"))
self.pushButton.setText(_translate("Form", "PushButton"))
from PromoteLabelTest import PromoteLabel
from Ui_T import *
from PyQt5.QtWidgets import QApplication, QWidget,QVBoxLayout,QTextEdit,QPushButton,QHBoxLayout
from PyQt5.QtWidgets import QLabel
class LogicalLabel(Ui_Form,QWidget):
def __init__(self,parent = None):
super().__init__(parent)
self.initUI()
def initUI(self):
self.setupUi(self)
self.label.setText("22222")
import sys
from PyQt5.QtWidgets import QApplication, QWidget,QVBoxLayout,QTextEdit,QPushButton,QHBoxLayout
from Public_Ui_T import *
class Demo(QWidget):
def __init__(self):
super(Demo, self).__init__()
self.initUI()
def initUI(self):
self.setWindowTitle("...")
self.resize(1200, 600)
Vlayout = QVBoxLayout()
MyLabel = LogicalLabel()
Vlayout.addWidget(MyLabel)
self.setLayout(Vlayout)
if __name__ == "__main__":
app = QApplication(sys.argv)
main = Demo()
main.show()
sys.exit(app.exec_())
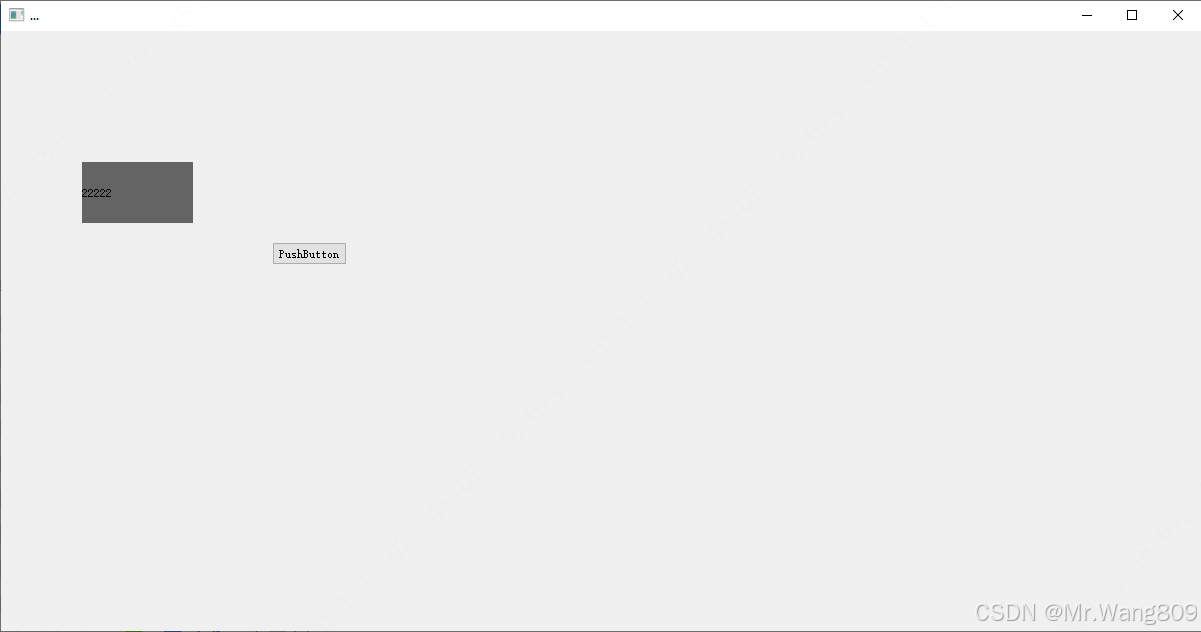