概念:
队列
:只允许在一端进行插入数据操作,在另一端进行删除数据操作的特殊线性表,队列具有先进先出
FIFO(First
In First Out)
入队列:进行插入操作的一端称为
队尾(
Tail/Rear
)
出队列:进行删除操作的一端称为
队头
(
Head/Front
)
队列的使用
在
Java
中,
Queue
是个接口,底层是通过链表实现
的。
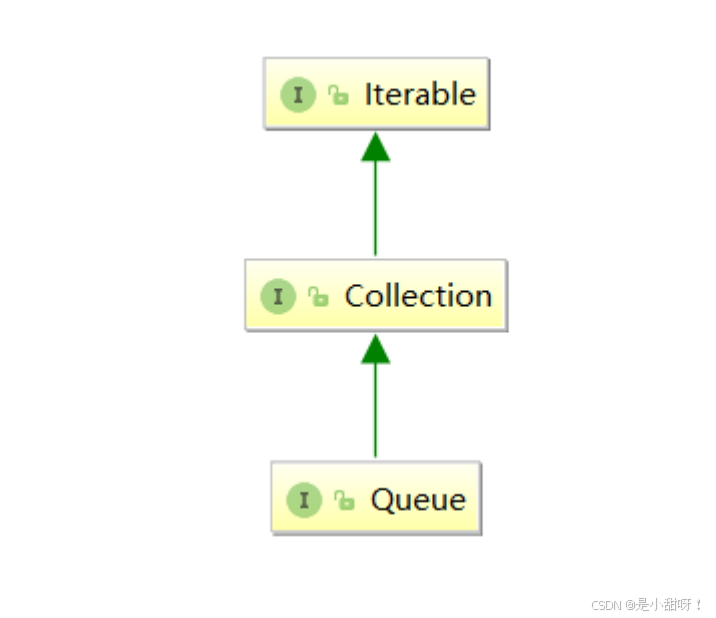
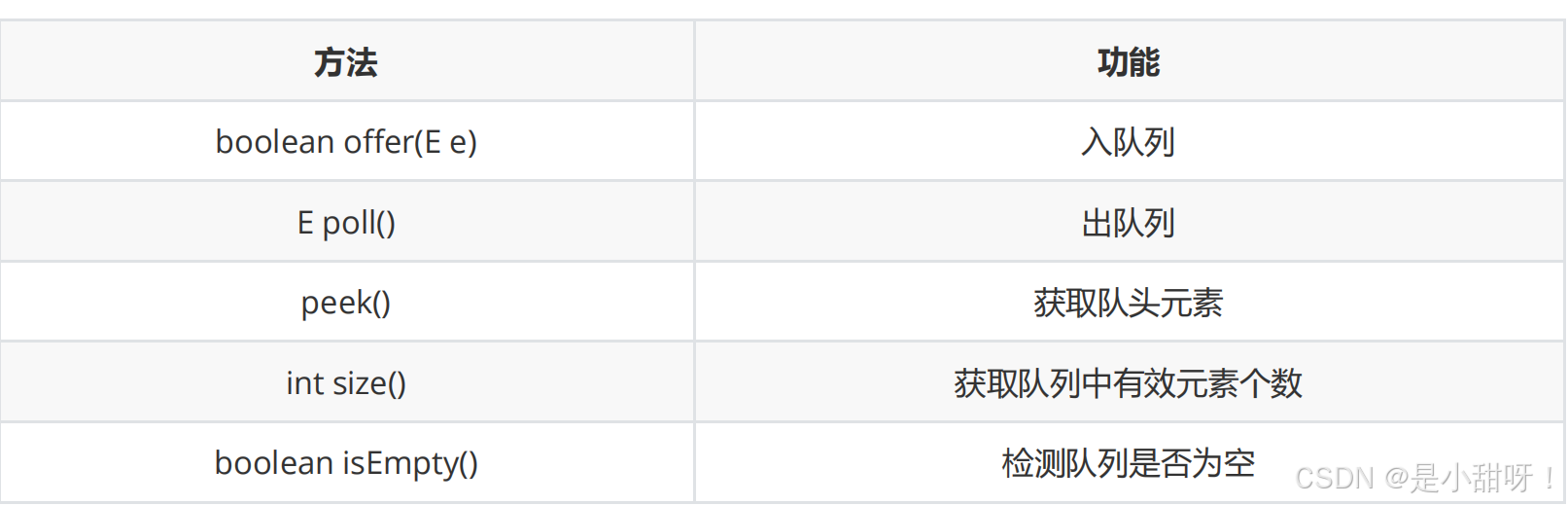
注意:
Queue
是个接口,在实例化时必须实例化
LinkedList
的对象,因为
LinkedList
实现了
Queue
接口。
Queue<Integer> q = new LinkedList<>();
队列模拟实现
队列中既然可以存储元素,那底层肯定要有能够保存元素的空间,所以队列的实现用链式结构好。
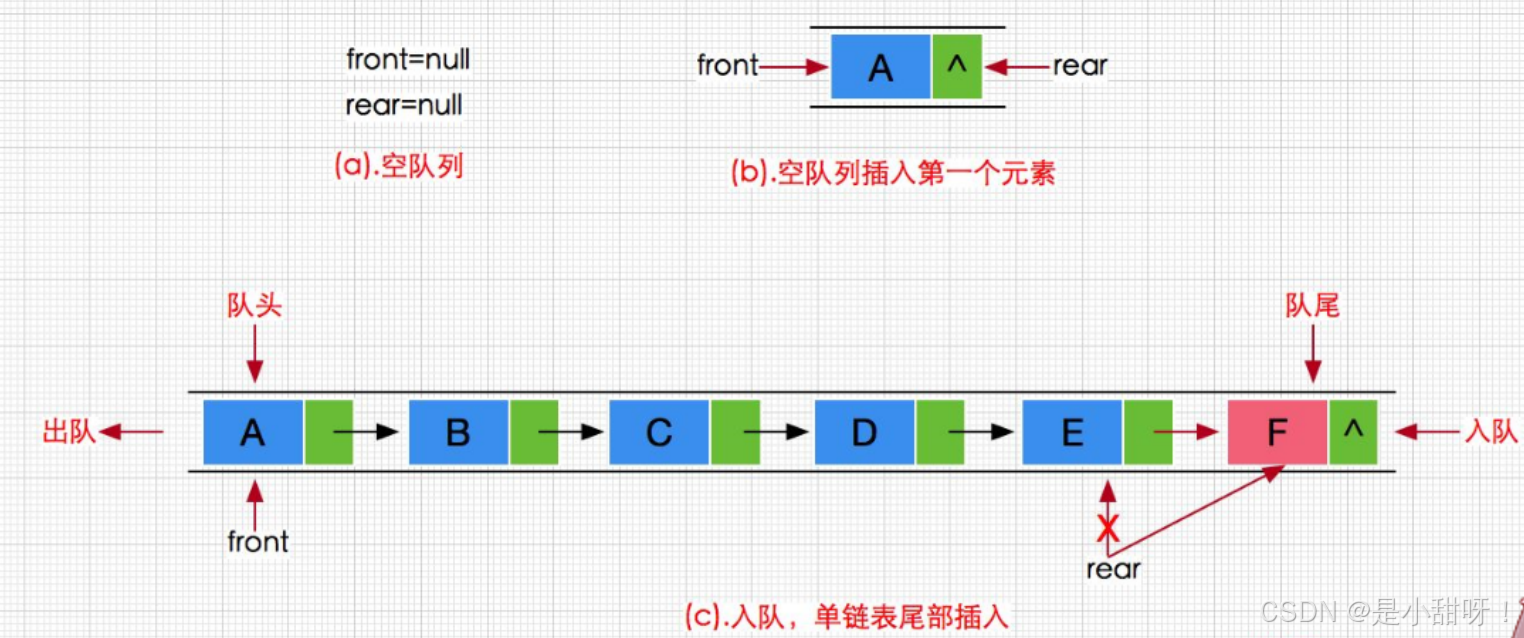
代码:
分为两部分:一个测试类一个实现类。
实现类:
public class MyQueue {
static class ListNode {
private int val;
private ListNode prev;
private ListNode next;
public ListNode(int val) {
this.val = val;
}
}
private ListNode front;//队头
private ListNode rear;//队尾
private int usedSize;
//队尾入队
public void offer(int x) {
ListNode node = new ListNode(x);
if (front == null) {
front = rear = node;
} else {
rear.next = node;
node.prev = rear;
rear = node;
}
usedSize++;
}
//队尾删除
public int poll() {
//队为空
if (front == null) {
return -1;
}
int ret = front.val;
//队里只有一个元素
if (front == rear) {
front = null;
rear = null;
usedSize--;
return -1;
}
front = front.next;
front.prev = null;
usedSize--;
return ret;
}
//获取队头的第一个节点的元素
public int peek() {
if (front == null) {
return -1;
}
return front.val;
}
//获取元素个数
public int getUsedSize() {
return usedSize;
}
//判空
public boolean isEmpty() {
if (usedSize == 0){
return true;
}
return false;
}
}
测试类:
public class Test {
public static void main(String[] args) {
MyQueue myQueue=new MyQueue();
myQueue.offer(1);
myQueue.offer(2);
myQueue.offer(3);
myQueue.offer(4);
System.out.println(myQueue.poll());
System.out.println(myQueue.poll());
System.out.println(myQueue.peek());
System.out.println(myQueue.getUsedSize());
System.out.println(myQueue.isEmpty());
}
}
结果: