目录
线程的创建与等待:
编辑 代码中tid是什么?
如何看待线程函数传参?
编辑
编辑创建多线程:编辑
终止多线程:
线程分离:
线程的创建与等待:
void *threadrun(void *args)
{
int cnt=10;
while(cnt)
{
std::cout<<"new thread run ...cnt:"<<cnt--<<std::endl;
sleep(1);
}
return nullptr;
}
int main()
{
pthread_t tid;
int n=pthread_create(&tid,nullptr,threadrun,(void*)"thread 1");//创建线程
if(n!=0)
{
std::cout<<"create fail"<<std::endl;
return 1;
}
std::cout<<"main thread join begin"<<std::endl;
n=pthread_join(tid,nullptr);//主线程等待
if(n==0)
{
std::cout<<"main thread wait sucess"<<std::endl;//主线程等待成功
}
return 0;
}
主线程需要等待其他线程结束后再退出,避免僵尸进程等情况的出现,线程运行的顺序并不确定,是随机切换的
代码中tid是什么?
tid是一个虚拟地址。
如何看待线程函数传参?
第三个参数不止可以传任意类型,也可以传类对象的地址。
- 当主线程正常运行,但其他线程出现异常,进程会直接终止。因此,线程函数只考虑正确的返回,不考虑异常的情况。
- 线程函数返回不止可以返回任意类型,也可以传递任意对象的地址
创建多线程:
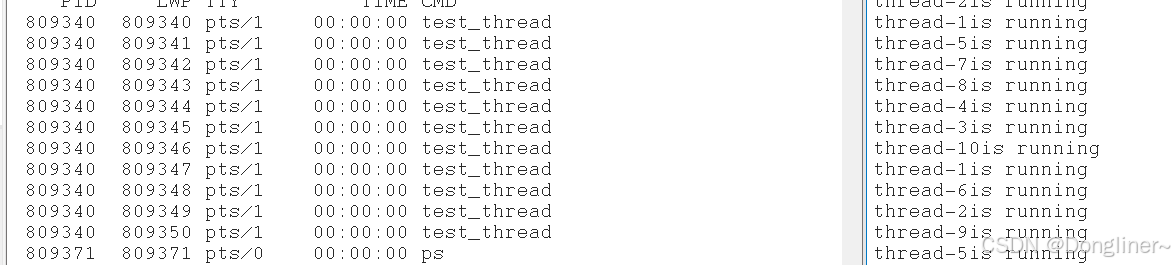
const int num=10;
void *threadrun(void *args)
{
std::string name=static_cast<const char*>(args);
while(true)
{
std::cout<<name<<"is running"<<std::endl;
sleep(1);
}
}
int main()
{
std::vector<pthread_t>tips;
//创建多线程
for(int i=0;i<num;i++)
{
//线程id
pthread_t tid;
char *name=new char[128];
snprintf(name,128,"thread-%d",i+1);
pthread_create(&tid,nullptr,threadrun,name);
}
sleep(100);
终止多线程:
主线程退出代表进程终止。
新线程退出方式:
- 函数return
- exit:在多线程中,任意一个线程调用exit ,进程都会终止,因为exit是终止进程的,线程使用的exit叫做pthread_exit函数
- main thread call pthread_cancel,新线程的退出结果是-1
线程分离:
一个线程被分离,线程的工作状态为分离状态,不需要被join 也不可以被join
C++11多线程
c++11多线程的本质就是对原生线程库接口 的封装