效果图
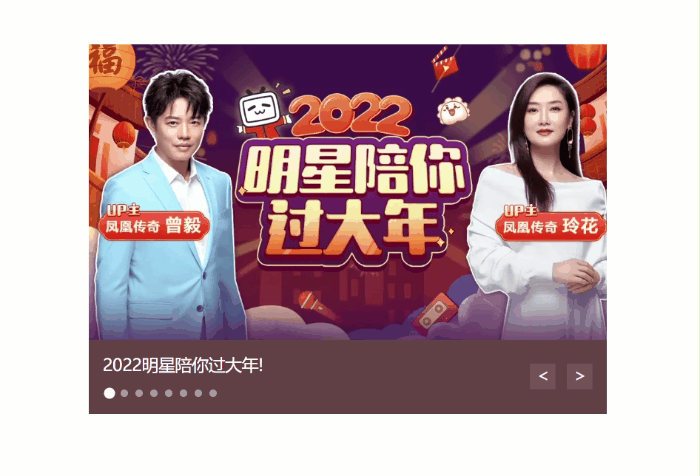
代码
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>练习:轮播图</title>
<style>
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
li {
list-style: none;
}
.box {
margin: 50px auto;
width: 560px;
height: 400px;
overflow: hidden;
}
.box>ul {
display: flex;
margin-left: -560px;
transition: all .5s;
}
.box ul li a {
display: block;
width: 560px;
height: 320px;
background: url(images/slider01.jpg) no-repeat left top/cover;
}
.box ul li:first-child a {
background-image: url(images/slider08.jpg);
}
.box .bottom {
position: relative;
width: 560px;
height: 80px;
background-color: rgb(100, 67, 68);
}
.box .bottom p {
padding: 15px;
font-size: 18px;
color: #fff;
}
.box .bottom ul {
display: flex;
margin-left: 14px;
}
.box .bottom ul li {
margin: 0 4px;
width: 8px;
height: 8px;
border-radius: 4px;
background-color: rgba(255, 255, 255, .5);
}
.box .bottom ul .active {
transform: scale(1.5);
border-radius: 6px;
background-color: #fff;
}
.box .bottom span {
position: absolute;
top: 26px;
width: 28px;
height: 28px;
background-color: rgba(255, 255, 255, .1);
font-size: 18px;
color: #fff;
text-align: center;
cursor: pointer;
}
.box .bottom .left {
right: 55px;
}
.box .bottom .right {
right: 15px;
}
</style>
</head>
<body>
<div class="box">
<ul>
<!-- 10个li,实际上只有8张轮播图,第1个和第10个li是为了无缝衔接轮播图
第1个li显示最后一张轮播图的图片,第10个li显示第一张轮播图的图片
轮播图是第2个li到第9个li -->
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
<li><a href="#"></a></li>
</ul>
<div class="bottom">
<p>2022明星陪你过大年!</p>
<ul>
<li class="active"></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
<li></li>
</ul>
<span class="left"><</span>
<span class="right">></span>
</div>
</div>
<script>
// 数据
const data = [
{ url: 'images/slider01.jpg', title: '2022明星陪你过大年!', color: 'rgb(100, 67, 68)' },
{ url: 'images/slider02.jpg', title: '开启剑与雪的黑暗传说!', color: 'rgb(43, 35, 26)' },
{ url: 'images/slider03.jpg', title: '八年游戏策划"缝"的游戏是啥样?', color: 'rgb(36, 31, 33)' },
{ url: 'images/slider04.jpg', title: '真正的jo厨出现了!', color: 'rgb(139, 98, 66)' },
{ url: 'images/slider05.jpg', title: '李玉刚:让世界通过B站看到东方大国文化', color: 'rgb(67, 90, 92)' },
{ url: 'images/slider06.jpg', title: '快来分享你的寒假日常吧~', color: 'rgb(166, 131, 143)' },
{ url: 'images/slider07.jpg', title: '哔哩哔哩小年YEAH!', color: 'rgb(53, 29, 25)' },
{ url: 'images/slider08.jpg', title: '一站式解决你的电脑配置问题!!!', color: 'rgb(99, 72, 114)' },
]
// 设置轮播图的图片,第一张和最后一张图片在CSS里面设置,这里不设置
for (let i = 0; i < data.length; i++) {
const a = document.querySelectorAll(".box ul li a")[i + 1]
a.style.backgroundImage = `url(${data[i].url})`
}
// 定义一个更换图片, 底部颜色, 文字, 小圆点的函数
const ul = document.querySelector(".box>ul")
const bottom = document.querySelector(".box .bottom")
const p = document.querySelector(".box .bottom p")
const lis = document.querySelectorAll(".box .bottom ul li")
function change(distance, num) {
ul.style.marginLeft = `${distance}px` //图片
bottom.style.backgroundColor = data[num].color //底部颜色
p.innerText = data[num].title //文字
document.querySelector(".box .bottom ul .active").classList.remove("active") //移除原来的高亮小圆点
lis[num].classList.add("active") //添加高亮小圆点
}
// 点击左按钮播放上一张
const left = document.querySelector(".box .bottom .left")
let num = 0
let distance = -560
left.addEventListener("click", () => {
if (num === 0) {
num = data.length - 1
} else {
num--
}
distance += 560
ul.style.transition = "all .5s"
change(distance, num)
// 如果当前图片为第一张图片(最后一张轮播图),则切换成倒数第二张图片(最后一张轮播图)
if (distance === 0) {
// 0.5秒后再切换,保证滑动效果
setTimeout(() => {
ul.style.transition = "all 0s" //转换前先取消过渡效果
distance = -(560 * (data.length))
ul.style.marginLeft = `${distance}px`
}, 500);
}
})
// 点击右按钮播放下一张
const right = document.querySelector(".box .bottom .right")
right.addEventListener("click", () => {
if (num === data.length - 1) {
num = 0
} else {
num++
}
distance -= 560
ul.style.transition = "all .5s"
change(distance, num)
// 如果当前图片为最后一张图片(第一张轮播图),则切换成第二张图片(第一张轮播图)
if (distance === -(560 * (data.length + 1))) {
// 0.5秒后再切换,保证滑动效果
setTimeout(() => {
ul.style.transition = "all 0s" //转换前先取消过渡效果
distance = -560
ul.style.marginLeft = `${distance}px`
}, 500);
}
})
// 每隔2秒自动播放
let autoplay = setInterval(() => {
right.click() //模拟点击右按钮
}, 2000)
//鼠标移入暂停播放,鼠标移出继续播放
const box = document.querySelector(".box")
box.addEventListener("mouseenter", () => {
clearInterval(autoplay)
})
box.addEventListener("mouseleave", () => {
autoplay = setInterval(() => {
right.click() //模拟点击右按钮
}, 2000)
})
</script>
</body>
</html>