目录
一,创建maven项目
1,左上角file > new > maven project
2,next 到 创建 Group id 和 Artifact id编辑编辑
二,配置springboot
1,配置pom文件,
2,创建启动类
3,配置 yml 文件
三,集成 Redis
1,yml追加配置
2,对 Redis 做些代码配置
3,spring 注解@Configuration类中编写代码访问
4,过滤器 ,获取Redis的代码 注:过滤路径在yml配置文件中配置
5,配置 Redis 数据
6,页面访问controller
四,集成 跨域
1,yml文件配置需要跨域的路径端口
2,过滤器,获取跨域代码
3,spring 注解@Configuration类中编写代码访问
五,集成 远程请求
1,封装工具类
2,通用工具类方法sendRequest
一,创建maven项目
1,左上角file > new > maven project
2,next 到 创建 Group id 和 Artifact id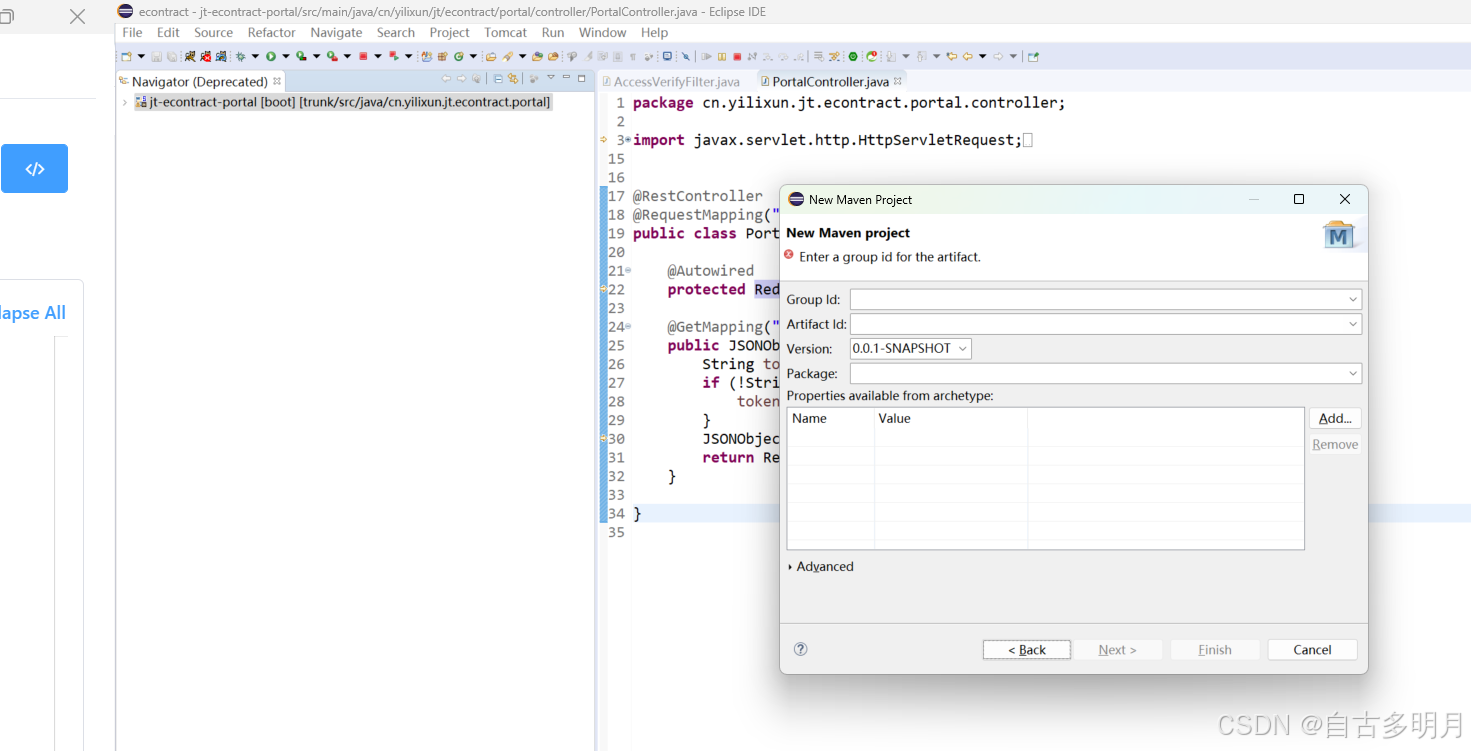
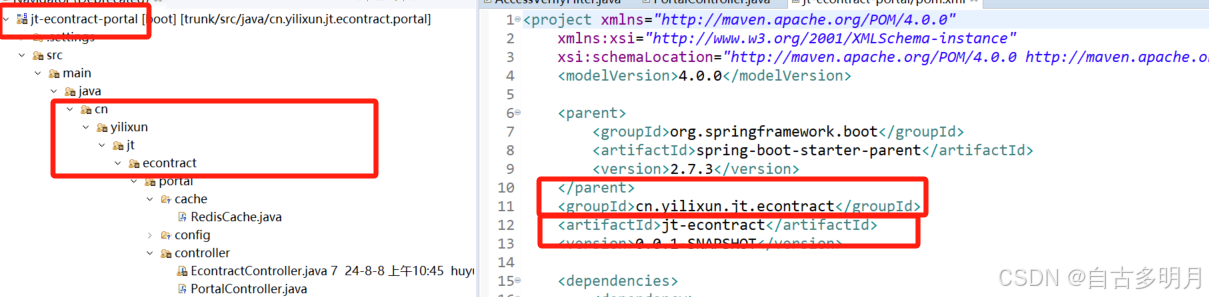
二,配置springboot
1,配置pom文件,
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.7.3</version>
</parent>
<groupId>cn.yilixun.jt.econtract</groupId>
<artifactId>jt-econtract</artifactId>
<version>0.0.1-SNAPSHOT</version>
<dependencies>
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>com.alibaba.fastjson2</groupId>
<artifactId>fastjson2</artifactId>
<version>2.0.12</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
</dependency>
<dependency>
<groupId>cn.hutool</groupId>
<artifactId>hutool-all</artifactId>
<version>5.8.12</version>
</dependency>
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-annotations</artifactId>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp-sse</artifactId>
</dependency>
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-boot-starter</artifactId>
<version>3.5.4.1</version>
</dependency>
<dependency>
<groupId>com.baomidou</groupId>
<artifactId>mybatis-plus-generator</artifactId>
<version>3.5.4.1</version>
</dependency>
<dependency>
<groupId>org.mybatis.scripting</groupId>
<artifactId>mybatis-velocity</artifactId>
<version>2.1.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>core</artifactId>
<version>3.3.0</version>
</dependency>
<dependency>
<groupId>com.google.zxing</groupId>
<artifactId>javase</artifactId>
<version>3.2.1</version>
</dependency>
<!-- 分页插件 -->
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.4.2</version>
</dependency>
<!-- easypoi的支持 -->
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>3.2.0</version>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<configuration>
<mainClass>cn.yilixun.jt.econtract.portal.EcontractPortalStartup</mainClass>
</configuration>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<configuration>
<source>1.8</source>
<target>1.8</target>
<encoding>UTF-8</encoding>
</configuration>
</plugin>
</plugins>
</build>
</project>
2,创建启动类
package cn.yilixun.jt.econtract.portal;
import org.mybatis.spring.annotation.MapperScan;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.context.properties.EnableConfigurationProperties;
import org.springframework.context.annotation.ComponentScan;
/**
* Hello world!
*
*/
@SpringBootApplication
@ComponentScan(basePackages = { "cn.yilixun.*" })
@MapperScan("cn.yilixun.jt.econtract.portal.mapper")
@EnableConfigurationProperties
public class EcontractPortalStartup {
public static void main(String[] args) {
SpringApplication.run(EcontractPortalStartup.class, args);
}
}
3,配置 yml 文件
application.yml
spring:
application:
name: portal
profiles:
active: dev
jackson:
default-property-inclusion: non_null
time-zone: GMT+8
main:
allow-bean-definition-overriding: true
mybatis-plus:
mapper-locations: classpath:/mybatis/*.xml
type-aliases-package: cn.yilixun.jt.econtract.portal.info
configuration:
log-impl: org.apache.ibatis.logging.stdout.StdOutImpl
#日志配置
logging:
config: classpath:logback-spring.xml
level:
org.springframework: warn
access:
ignore-pattern: /*|
control:
allow:
origin: http://localhost:8080
application-dev.yml
server:
port: 10000
servlet:
context-path: /econtract
logging:
file:
path: D:\logs
spring:
redis:
host: 172.16.1.205
port: 6379
database: 8
password: "Redis12#$56"
timeout: 10s
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://172.16.1.205:3306/jt_econtract?serverTimezone=GMT%2B8&remarks=true&useInformationSchema=true&tinyInt1isBit=true&allowMultiQueries=true
username: jt_econtract
password: JTecontract@2024.
至此,springboot项目生成完毕
三,集成 Redis
1,yml追加配置
2,对 Redis 做些代码配置
package cn.yilixun.jt.econtract.portal.cache;
import java.util.concurrent.TimeUnit;
import org.springframework.data.redis.core.HashOperations;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import com.alibaba.fastjson2.JSON;
import com.alibaba.fastjson2.JSONObject;
public class RedisCache {
public final static long REDIS_EXPIRE_TIME = 7200L;
public final static String REDIS_KEY_USER_TOKEN = "user-token:";
public final static String REDIS_KEY_BASE_DATA = "base-data:";
private RedisTemplate<String, String> redisTemplate;
public RedisCache(RedisTemplate<String, String> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void hset(final String key, final String hashKey, final Object value) {
this.redisTemplate.opsForHash().put(key, hashKey, JSON.toJSONString(value));
}
public String hget(final String key, final String hashKey) {
HashOperations<String,Object,Object> operation = this.redisTemplate.opsForHash();
if (operation != null && operation.get(key, hashKey) != null) {
return (String)operation.get(key, hashKey);
}
return null;
}
public void set(final String key, final Object value) {
this.redisTemplate.opsForValue().set(key, JSON.toJSONString(value));
}
public void set(final String key, final Object value, final long timeout, final TimeUnit timeUnit) {
this.redisTemplate.opsForValue().set(key, JSON.toJSONString(value), timeout, timeUnit);
}
public String get(final String key) {
ValueOperations<String, String> operation = this.redisTemplate.opsForValue();
return operation.get(key);
}
public boolean expire(final String key, final long timeout) {
return this.expire(key, timeout, TimeUnit.SECONDS);
}
public boolean expire(final String key, final long timeout, final TimeUnit unit) {
return this.redisTemplate.expire(key, timeout, unit);
}
public boolean delete(final String key) {
return this.redisTemplate.delete(key);
}
public void createUserToken(String token, Object user) {
if (user != null) {
this.set(RedisCache.REDIS_KEY_USER_TOKEN + token, user, RedisCache.REDIS_EXPIRE_TIME, TimeUnit.SECONDS);
}
}
public JSONObject getLoginUserInfo(String token) {
Object obj = this.get(RedisCache.REDIS_KEY_USER_TOKEN + token);
if (obj != null) {
this.expire(RedisCache.REDIS_KEY_USER_TOKEN + token, RedisCache.REDIS_EXPIRE_TIME, TimeUnit.SECONDS);
return JSONObject.parseObject(obj.toString());
}
return null;
}
}
3,spring 注解@Configuration类中编写代码访问
package cn.yilixun.jt.econtract.portal.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.core.RedisTemplate;
import cn.yilixun.jt.econtract.portal.cache.RedisCache;
import cn.yilixun.jt.econtract.portal.filter.SimpleCorsFilter;
@Configuration
public class PropertiesConfig {
@Autowired
private RedisTemplate<String, String> redisTemplate;
@Bean
public RedisCache redisCache() {
return new RedisCache(this.redisTemplate);
}
}
4,过滤器 ,获取Redis的代码 注:过滤路径在yml配置文件中配置
package cn.yilixun.jt.econtract.portal.filter;
import java.io.IOException;
import java.util.regex.Pattern;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.annotation.WebFilter;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.annotation.Order;
import org.springframework.stereotype.Component;
import org.springframework.util.StringUtils;
import com.alibaba.fastjson2.JSONObject;
import cn.yilixun.jt.econtract.portal.cache.RedisCache;
@Component
@WebFilter(urlPatterns = {"/*"})
@Order(1)
public class AccessVerifyFilter implements Filter {
@Value("${access.ignore-pattern:}")
private String ignoreLoginUri;
@Autowired
private RedisCache redisCache;
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest req = (HttpServletRequest) request;
String token = req.getHeader("User-Token");
if (!StringUtils.hasLength(token)) {
token = req.getParameter("userToken");
}
JSONObject user = redisCache.getLoginUserInfo(token);
String path = req.getServletPath();
if (!this.isIgnoreUri(this.ignoreLoginUri, path) && (user == null || user.isEmpty())) {
JSONObject erInfo = new JSONObject();
erInfo.put("state", 900);
erInfo.put("code", "302");
this.writeResponse(response, erInfo);
return;
}
chain.doFilter(request, response);
}
private void writeResponse(ServletResponse response, JSONObject msg) throws IOException {
response.setCharacterEncoding("UTF-8");
response.setContentType("application/json; charset=utf-8");
response.getWriter().write(msg.toJSONString());
response.getWriter().flush();
}
private boolean isIgnoreUri(String patternsStr, String path) {
if (StringUtils.hasLength(patternsStr)) {
String[] patterns = patternsStr.split("\\|");
if (patterns.length > 0) {
for (String p : patterns) {
if (StringUtils.hasLength(p) && Pattern.matches(p.replace("*", ".*"), path)) {
return true;
}
}
}
}
return false;
}
}
5,配置 Redis 数据
6,页面访问controller
package cn.yilixun.jt.econtract.portal.controller;
import javax.servlet.http.HttpServletRequest;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.StringUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import com.alibaba.fastjson2.JSONObject;
import cn.yilixun.jt.econtract.portal.cache.RedisCache;
import cn.yilixun.jt.econtract.portal.response.ResponseResult;
@RestController
@RequestMapping("/")
public class PortalController {
@Autowired
protected RedisCache redisCache;
@GetMapping("/getBaseInfo")
public JSONObject dictList(HttpServletRequest req) {
String token = req.getHeader("User-Token");
if (!StringUtils.hasLength(token)) {
token = req.getParameter("userToken");
}
JSONObject user = redisCache.getLoginUserInfo(token);
return ResponseResult.success(user);
}
}
至此,Redis 集成结束
四,集成 跨域
1,yml文件配置需要跨域的路径端口
2,过滤器,获取跨域代码
package cn.yilixun.jt.econtract.portal.filter;
import java.io.IOException;
import java.util.Arrays;
import javax.servlet.Filter;
import javax.servlet.FilterChain;
import javax.servlet.FilterConfig;
import javax.servlet.ServletException;
import javax.servlet.ServletRequest;
import javax.servlet.ServletResponse;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
/**
* SimpleCORSFilter.
* @Title:SimpleCORSFilter.java
* @Description SimpleCORSFilter.java
*/
public class SimpleCorsFilter implements Filter {
private String originUrl;
@Override
public void init(FilterConfig filterConfig) throws ServletException {
originUrl = filterConfig.getInitParameter("originUrl");
}
/**
* 请求过滤器.
*/
@Override
public void doFilter(ServletRequest req, ServletResponse res, FilterChain chain)
throws IOException, ServletException {
HttpServletResponse response = (HttpServletResponse) res;
HttpServletRequest re = (HttpServletRequest) req;
String url = re.getHeader("Origin");
String[] urlArray = originUrl.split(",");
if (Arrays.asList(urlArray).contains(url)) {
response.setHeader("Access-Control-Allow-Origin", url);
}
response.setHeader("Access-Control-Allow-Methods", "POST, GET, OPTIONS, DELETE");
response.setHeader("Access-Control-Max-Age", "3600");
response.setHeader("Access-Control-Allow-Headers", "x-requested-with,content-type,token,Access-Token");
response.setHeader("Access-Control-Allow-Credentials", "true");
chain.doFilter(req, res);
}
}
3,spring 注解@Configuration类中编写代码访问
package cn.yilixun.jt.econtract.portal.config;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.core.RedisTemplate;
import cn.yilixun.jt.econtract.portal.cache.RedisCache;
import cn.yilixun.jt.econtract.portal.filter.SimpleCorsFilter;
@Configuration
public class PropertiesConfig {
@Value("${access.control.allow.origin}")
private String originUrl;
/**
* 跨域过滤器.
*
* @return
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
@Bean
public FilterRegistrationBean filterRegist() {
FilterRegistrationBean frBean = new FilterRegistrationBean();
frBean.setFilter(new SimpleCorsFilter());
frBean.addUrlPatterns("/*");
frBean.addInitParameter("originUrl", originUrl);
return frBean;
}
}
至此,跨域集成结束
五,集成 远程请求
1,封装工具类
package cn.yilixun.jt.econtract.portal.util;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.http.HttpEntity;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.util.StringUtils;
import org.springframework.web.client.RestTemplate;
import com.alibaba.fastjson2.JSONObject;
import lombok.extern.log4j.Log4j2;
@Log4j2
public class RestTemplateUtil {
/**
* 发送请求.
*
* @param method not null
* @return
*/
public static JSONObject sendRequest(String url, HttpMethod method, Object bodyParams, JSONObject headerParams) throws Exception {
log.info("RestTemplateUtil.sendRequest start :: [url] = " + url + ", [method] = " + method);
RestTemplate rest = new RestTemplate();
rest.getMessageConverters().add(new WxMappingJackson2HttpMessageConverter());
HttpEntity<Object> request = RestTemplateUtil.getRequestEntity(bodyParams, headerParams);
ResponseEntity<JSONObject> res = rest.exchange(url, method, request, JSONObject.class);
if (res == null || res.getBody() == null) {
throw new Exception("获取api数据异常");
}
log.info("RestTemplateUtil.sendRequest end :: " + res.getBody().toString());
return res.getBody();
}
private static HttpEntity<Object> getRequestEntity(Object rbp, JSONObject rhp) {
MultiValueMap<String, String> header = new LinkedMultiValueMap<>();
if (rhp != null && !rhp.isEmpty()) {
for (String key : rhp.keySet()) {
header.add(key, rhp.getString(key));
}
}
return new HttpEntity<>(rbp, header);
}
/**
* 发送请求.
*
* @param method not null
* @return
*/
public static JSONObject sendRequestMap(String url, HttpMethod method, Map<String, Object> requestBodyParams, Map<String, String> requestHeaderParams) throws Exception {
log.info("RestTemplateUtil.sendRequest start :: [url] = " + url + ", [method] = " + method);
RestTemplate rest = new RestTemplate();
rest.getMessageConverters().add(new WxMappingJackson2HttpMessageConverter());
HttpEntity<Map<String, Object>> request = getRequest(requestBodyParams, requestHeaderParams);
if (method == HttpMethod.GET) {
url = convetUrl(url, requestBodyParams);
}
ResponseEntity<JSONObject> res = rest.exchange(url, method, request, JSONObject.class);
if (res == null || res.getBody() == null) {
throw new Exception("获取api数据异常");
}
log.info("RestTemplateUtil.sendRequest end :: " + res.getBody().toString());
return res.getBody();
}
/**
* 请求配置.
*
* @Param :authorization
* @Return :HttpEntity
*/
private static HttpEntity<Map<String, Object>> getRequest(Map<String, Object> rbp, Map<String, String> rhp) {
Map<String, Object> body = new HashMap<>();
HttpHeaders header = new HttpHeaders();
if (rbp != null && !rbp.isEmpty()) {
for (String key : rbp.keySet()) {
body.put(key, rbp.get(key));
}
}
if (rhp != null && !rhp.isEmpty()) {
for (String key : rhp.keySet()) {
header.add(key, rhp.get(key));
}
}
return new HttpEntity<>(body, header);
}
public static class WxMappingJackson2HttpMessageConverter extends MappingJackson2HttpMessageConverter {
/**
* RestTemplate 把数据从 HttpResponse 转换成 Object 的时候,找不到合适的 HttpMessageConverter 来转换
* 需要继承 MappingJackson2HttpMessageConverter 并在构造过程中设置其支持的 MediaType 类型.
*/
public WxMappingJackson2HttpMessageConverter() {
List<MediaType> mediaTypes = new ArrayList<>();
mediaTypes.add(MediaType.APPLICATION_OCTET_STREAM);
setSupportedMediaTypes(mediaTypes);
}
}
/**
* get请求url拼接参数.
* @Param :authorization
* @Return :HttpEntity
*/
public static String convetUrl(String url, Map<String, Object> param) {
String paramUrl = "";
if (param != null) {
for (Map.Entry<String, Object> e : param.entrySet()) {
paramUrl+=(e.getKey() + "=" + e.getValue() + "&");
}
}
if (StringUtils.hasLength(paramUrl)) {
return url + "?" + paramUrl.substring(0, paramUrl.length() - 1);
}
return url;
}
}
2,通用工具类方法sendRequest
private void executeAfterStartup() {
JSONObject jsonObject = null;
try {
jsonObject = RestTemplateUtil.sendRequest("http://localhost:8080/aaaaa", HttpMethod.POST,new JSONObject(), null);
} catch (Exception e) {
e.printStackTrace();
}
if ("10".equals(jsonObject.get("code").toString())) {
JSONObject jsonObject = jsonObject.get("data");
}
}
至此,远程请求集成结束