网页秒表小工具
效果展示
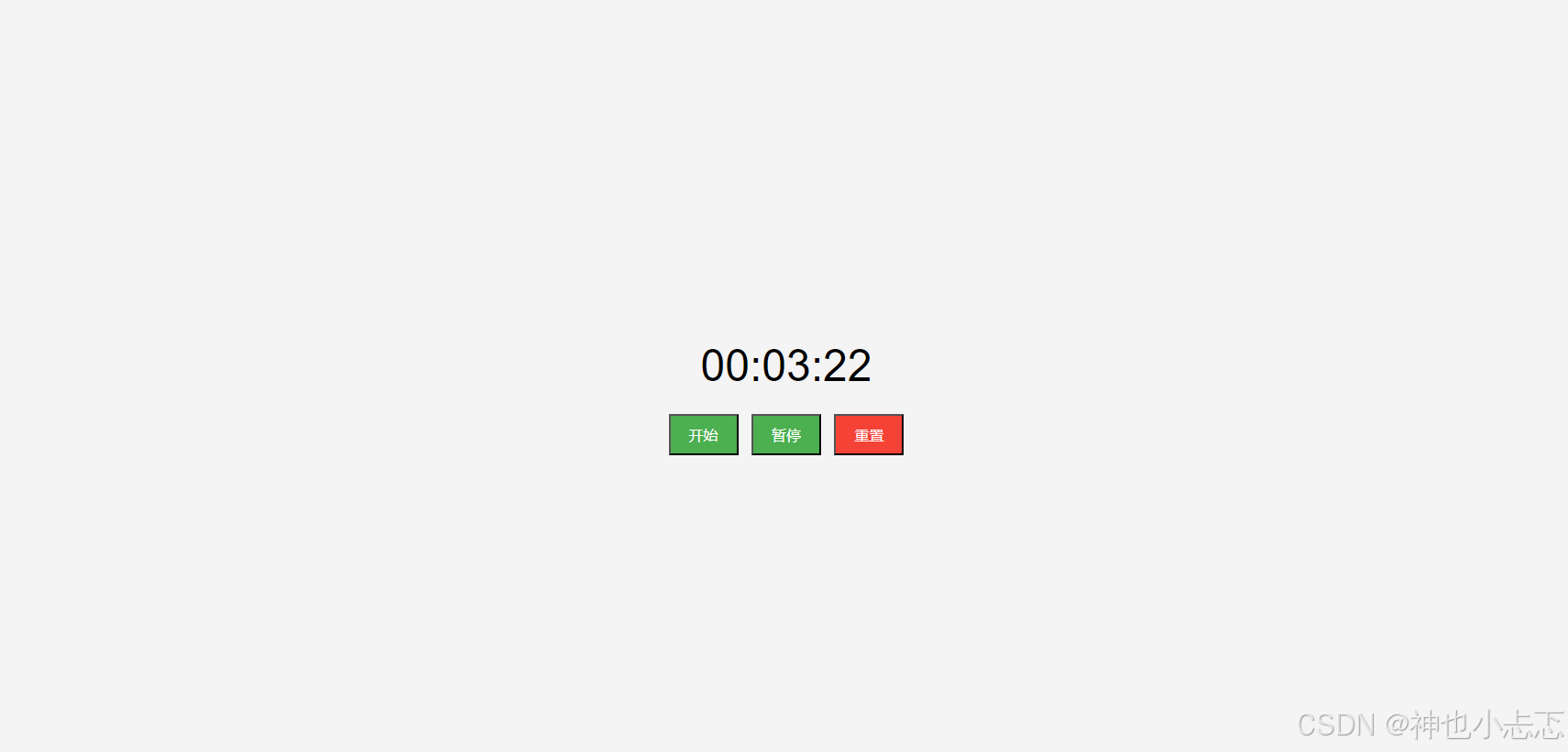
HTML代码
<!DOCTYPE html>
<html lang="zh-CN">
<head>
<meta charset="UTF-8">
<title>简洁秒表</title>
<style>
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
background-color: #f4f4f4;
}
.timer-container {
text-align: center;
}
.display {
font-size: 48px;
margin-bottom: 20px;
}
.button {
padding: 10px 20px;
margin: 5px;
font-size: 16px;
cursor: pointer;
}
#start, #pause {
background-color: #4CAF50;
color: white;
}
#reset {
background-color: #f44336;
color: white;
}
</style>
</head>
<body>
<div class="timer-container">
<div class="display" id="timerDisplay">00:00:00</div>
<button class="button" id="start">开始</button>
<button class="button" id="pause">暂停</button>
<button class="button" id="reset">重置</button>
</div>
<script>
let seconds = 0;
let interval = null;
function updateTimerDisplay() {
let hours = Math.floor(seconds / 3600);
let minutes = Math.floor((seconds - (hours * 3600)) / 60);
let secs = seconds % 60;
hours = hours < 10 ? '0' + hours : hours;
minutes = minutes < 10 ? '0' + minutes : minutes;
secs = secs < 10 ? '0' + secs : secs;
document.getElementById('timerDisplay').textContent =
hours + ':' + minutes + ':' + secs;
}
document.getElementById('start').addEventListener('click', function() {
if (interval) clearInterval(interval);
interval = setInterval(function() {
seconds++;
updateTimerDisplay();
}, 1000);
});
document.getElementById('pause').addEventListener('click', function() {
clearInterval(interval);
});
document.getElementById('reset').addEventListener('click', function() {
clearInterval(interval);
seconds = 0;
updateTimerDisplay();
});
</script>
</body>
</html>