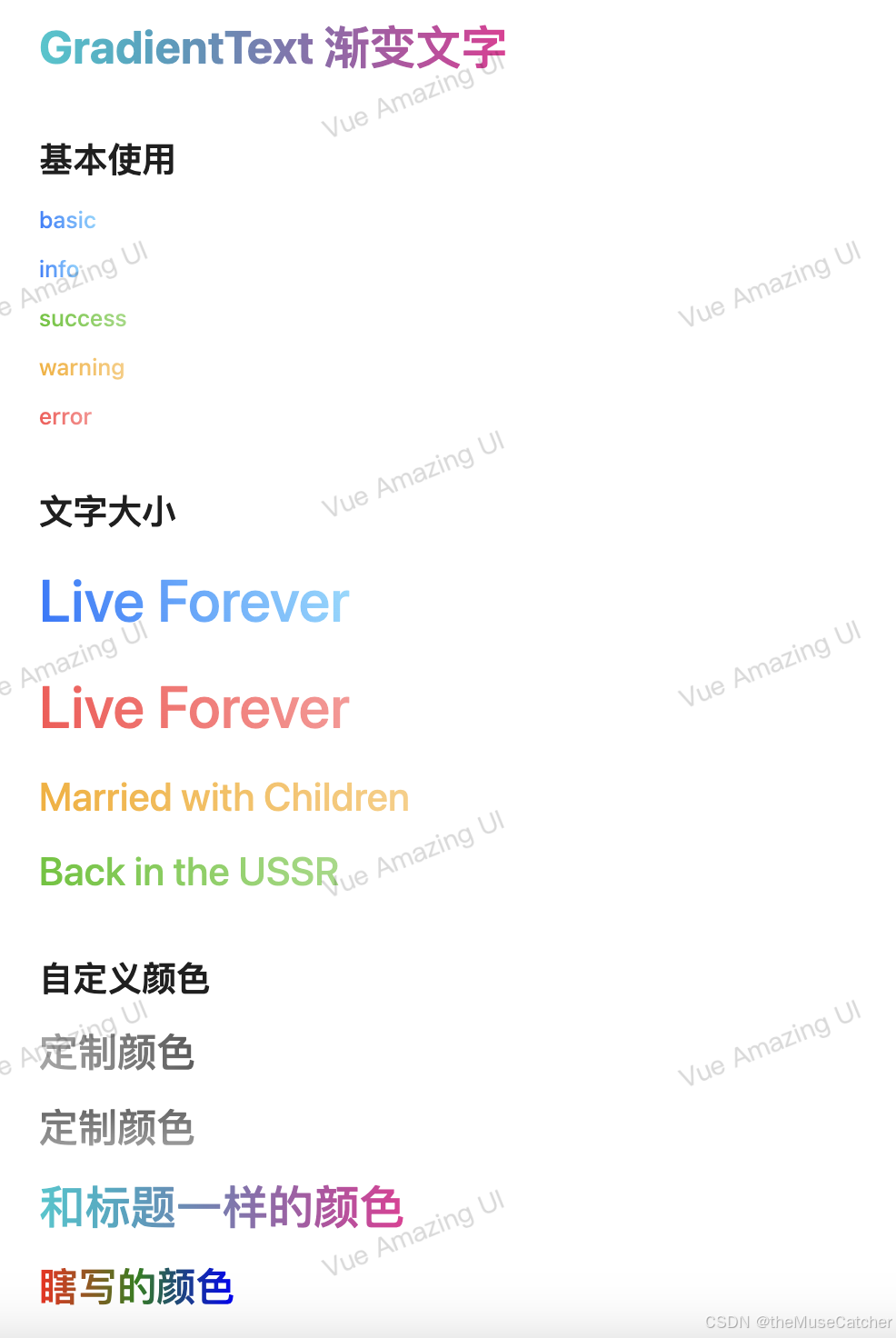
APIs
GradientText
参数 | 说明 | 类型 | 默认值 | 必传 |
---|
gradient | 文字渐变色参数 | string | Gradient | undefined | false |
size | 文字大小,不指定单位时,默认单位 px | number | string | 14 | false |
type | 渐变文字的类型 | ‘primary’ | ‘info’ | ‘success’ | ‘warning’ | ‘error’ | ‘primary’ | false |
Gradient Type
名称 | 说明 | 类型 | 必传 |
---|
from | 起始颜色 | string | true |
to | 终点颜色 | string | true |
deg | 渐变角度,单位 deg | number | string | false |
创建渐变文字组件GradientText.vue
<script setup lang="ts">
import { computed } from 'vue'
interface Gradient {
from: string
to: string
deg?: number | string
}
interface Props {
gradient?: string | Gradient
size?: number | string
type?: 'primary' | 'info' | 'success' | 'warning' | 'error'
}
const props = withDefaults(defineProps<Props>(), {
gradient: undefined,
size: 14,
type: 'primary'
})
enum TypeStartColor {
primary = 'rgba(22, 199, 255, 0.6)',
info = 'rgba(22, 199, 255, 0.6)',
success = 'rgba(82, 196, 26, 0.6)',
warning = 'rgba(250, 173, 20, 0.6)',
error = 'rgba(255, 77, 79, 0.6)'
}
enum TypeEndColor {
primary = '#1677FF',
info = '#1677FF',
success = '#52c41a',
warning = '#faad14',
error = '#ff4d4f'
}
const gradientText = computed(() => {
if (typeof props.gradient === 'string') {
return {
backgroundImage: props.gradient
}
}
return {}
})
const rotate = computed(() => {
if (typeof props.gradient === 'object' && props.gradient.deg) {
return isNumber(props.gradient.deg) ? props.gradient.deg + 'deg' : props.gradient.deg
}
return '252deg'
})
const colorStart = computed(() => {
if (typeof props.gradient === 'object') {
return props.gradient.from
} else {
return TypeStartColor[props.type]
}
})
const colorEnd = computed(() => {
if (typeof props.gradient === 'object') {
return props.gradient.to
} else {
return TypeEndColor[props.type]
}
})
const fontSize = computed(() => {
if (typeof props.size === 'number') {
return props.size + 'px'
}
if (typeof props.size === 'string') {
return props.size
}
})
function isNumber(value: string | number): boolean {
return typeof value === 'number'
}
</script>
<template>
<span
class="m-gradient-text"
:style="[
`--rotate: ${rotate}; --color-start: ${colorStart}; --color-end: ${colorEnd}; --font-size: ${fontSize};`,
gradientText
]"
>
<slot></slot>
</span>
</template>
<style lang="less" scoped>
.m-gradient-text {
display: inline-block;
font-size: var(--font-size);
font-weight: 500;
line-height: 1.5714285714285714;
-webkit-background-clip: text;
background-clip: text;
color: #0000;
white-space: nowrap;
background-image: linear-gradient(var(--rotate), var(--color-start) 0%, var(--color-end) 100%);
transition: all 0.3s cubic-bezier(0.4, 0, 0.2, 1);
}
</style>
在要使用的页面引入
<script setup lang="ts">
import GradientText from './GradientText.vue'
</script>
<template>
<div>
<h1>{{ $route.name }} {{ $route.meta.title }}</h1>
<h2 class="mt30 mb10">基本使用</h2>
<Space vertical>
<GradientText>basic</GradientText>
<GradientText type="info">info</GradientText>
<GradientText type="success">success</GradientText>
<GradientText type="warning">warning</GradientText>
<GradientText type="error">error</GradientText>
</Space>
<h2 class="mt30 mb10">文字大小</h2>
<Space vertical>
<GradientText :size="36" type="info">Live Forever</GradientText>
<GradientText :size="36" type="error">Live Forever</GradientText>
<GradientText :size="24" type="warning">Married with Children</GradientText>
<GradientText :size="24" type="success">Back in the USSR</GradientText>
</Space>
<h2 class="mt30 mb10">自定义颜色</h2>
<Space vertical>
<GradientText
:size="24"
:gradient="{
from: 'rgb(85, 85, 85)',
to: 'rgb(170, 170, 170)'
}"
>定制颜色</GradientText
>
<GradientText
:size="24"
:gradient="{
deg: 180,
from: 'rgb(85, 85, 85)',
to: 'rgb(170, 170, 170)'
}"
>定制颜色</GradientText
>
<GradientText
:size="28"
:gradient="{
deg: '90deg',
from: '#09c8ce',
to: '#eb2f96'
}"
>和标题一样的颜色</GradientText
>
<GradientText :size="24" gradient="linear-gradient(90deg, red 0%, green 50%, blue 100%)">瞎写的颜色</GradientText>
</Space>
</div>
</template>