目录
前言
1.第一个C++程序
2.命名空间
2.1概念理解
2.2namespace 的价值
2.3 namespace的定义
3.命名空间的使用
4.C++的输入输出
结束语
前言
本节我们将正式进入C++基础的学习,话不多说,直接上货!!!
1.第一个C++程序
// test.cpp
#include<stdio.h>
int main()
{
printf("hello world\n");
return 0;
}
#include <iostream>
using namespace std;
int main() {
cout << "hello world" << endl;
return 0;
}
2.命名空间
2.1概念理解
在计算机科学中,命名空间是一种将一组符号名称(如变量名、函数名、类名等)绑定到特定作用域的上下文的方法,以避免名称冲突。在不同的编程语言中,命名空间可能以不同的形式存在,但基本概念是相似的。
在C++中,命名空间是一种组织代码的结构,它允许你将一组具有唯一名称的实体(如类、函数和变量)封装在一起,从而避免了全局命名空间的名称冲突问题。命名空间对于大型项目和库尤其有用,因为它们可以减少名称碰撞的可能性,并使代码更易于管理。
在C++中定义命名空间非常简单,使用namespace关键字后跟命名空间的名字。
要访问命名空间中的成员,可以使用作用域解析运算符::
2.2namespace 的价值
#include <stdio.h>
#include <stdio.h>
#include <stdlib.h>
int rand = 10;
int main()
{
// 编译报错:error C2365: “rand”: 重定义;以前的定义是“函数”
printf("%d\n", rand);
return 0;
}
C++使用namespace后:
#include <iostream>
#include <stdio.h>
namespace hu{
int rand = 10;
}
int main()
{
printf("%d\n", hu::rand);
return 0;
}
2.3 namespace的定义
在C++中,namespace
(命名空间)是C++语言的一个核心特性,不属于任何特定的库。
1.定义命名空间,需要使用到namespace关键字,后面跟命名空间的名字,然后接⼀对{}即可,{}中即为命名空间的成员。命名空间中可以定义变量/函数/类型等。2.namespace本质是定义出⼀个域,这个域跟全局域各自独立,不同的域可以定义同名变量,所以rand不在冲突了。3.C++中域有函数局部域,全局域,命名空间域,类域;域影响的是编译时语法查找⼀个变量/函数/ 类型出处(声明或定义)的逻辑,所有有了域隔离,名字冲突就解决了。局部域和全局域除了会影响编译查找逻辑,还会影响变量的生命周期, 命名空间域和类域不影响变量生命周期。
#include <stdio.h>
//#include <stdio.h>
#include <stdlib.h>
// hu是命名空间的名字,⼀般开发中是⽤项⽬名字做命名空间名
namespace hu{
// 命名空间中可以定义变量/函数/类型
int rand = 10;
int add(int x, int y) {
return x + y;
}
//定义一个节点
struct Node
{
struct Node* next;
int val;
};
}
int main()
{
hu::Node* node = (hu::Node*)malloc(sizeof(hu::Node));
node->val = 1;
// 这⾥默认是访问的是全局的rand函数指针
printf("%p\n", rand);
// 这⾥指定hu命名空间中的rand
printf("%d\n", hu::rand);
printf("%d\n", hu::add(2, 3));
printf("%d\n", node->val);
return 0;
}
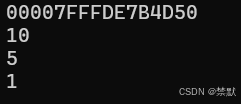
namespace HL{
namespace H{
int rand = 1;
int Add(int left, int right)
{
return left + right;
}
}
namespace L{
int rand = 2;
int Add(int left, int right)
{
return (left + right) * 10;
}
}
}
int main()
{
printf("%d\n", HL::H::rand);
printf("%d\n", HL::L::rand);
printf("%d\n", HL::H::Add(1, 2));
printf("%d\n", HL::L::Add(1, 2));
return 0;
}
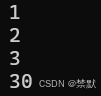
//头文件--function1.h
#include <stdio.h>
namespace MyNamespace {
void function1() {
printf("This is function1 in file1\n");
}
}
//头文件--function2.h
#include <stdio.h>
namespace MyNamespace {
void function2() {
printf("This is function2 in file2\n");
}
}
//测试文件
#include "function1.h"
#include "function2.h"
// 声明函数原型,以便在main函数中调用
int main() {
MyNamespace::function1();
MyNamespace::function2();
return 0;
}

3.命名空间的使用
#include<stdio.h>
namespace bite
{
int a = 0;
int b = 1;
}
int main()
{
// 编译报错:error C2065: “a”: 未声明的标识符
printf("%d\n", a);
return 0;
}
• 指定命名空间访问,项目中推荐这种方式。• using将命名空间中某个成员展开,项目中经常访问的不存在冲突的成员推荐这种方式。• 展开命名空间中全部成员,项目不推荐,冲突风险很大,日常小练习程序为了方便推荐使用。
#include <stdio.h>
namespace N {
int a = 520;
int b = 1314;
}
int main()
{
// 指定命名空间访问
printf("%d\n", N::a);
// using将命名空间中某个成员展开
using N::b;
printf("%d\n", b);
return 0;
}
C++标准库都放在⼀个叫std(standard)的命名空间中标准库命名空间: C++标准库中的所有功能都定义在std命名空间中。你可以使用std::前缀来访问标准库中的成员,或者使用using声明或指令来引入特定的成员。在使用命名空间时,应该考虑到代码的清晰性和可维护性。虽然using指令可以简化代码,但过度使用可能会导致名称冲突和代码的不可预测性。因此,最好是在局部作用域或函数内部使用using声明,而不是在全局作用域或头文件中使用using namespace指令。
4.C++的输入输出
代码展示
#include <iostream>
using namespace std;
int main() {
int a = 10;
double b = 5.2;
char c = 'd';
cout << a << " " << b << " " << c << endl;
std::cout << a << " " << b << " " << c << std::endl;
scanf("%d %lf", &a, &b);
printf("%d %lf", a, b);
// 可以⾃动识别变量的类型
cin >> a;
cin >> b >> c;
cout << a << endl;
cout << b << " " << c << endl;
return 0;
}
扩展了解部分(提高效率)
#include<iostream>
using namespace std;
int main()
{
// 在io需求⽐较⾼的地⽅,如部分⼤量输⼊的竞赛题中,加上以下3⾏代码
// 可以提⾼C++IO效率
ios_base::sync_with_stdio(false);
cin.tie(nullptr);
cout.tie(nullptr);
return 0;
}
结束语
本节内容新知识不是很多,着重讲了namespace的用法,下节内容将带来更加有趣的C++知识,欢迎大家的捧场!!!
最后感谢各位友友的支持,友友们动个手指点个赞,留个评论吧!!!