桥接模式
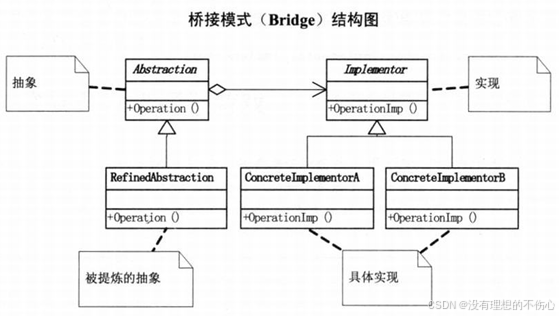
C++
#include <iostream>
using namespace std;
class HandsetSoft
{
public:
virtual ~HandsetSoft() = default;
virtual void Run() = 0;
};
class HandsetGame : public HandsetSoft
{
public:
void Run() override
{
cout << "运行手机游戏!" << endl;
}
};
class HandsetAddressList : public HandsetSoft
{
public:
void Run() override
{
cout << "运行手机通讯录!" << endl;
}
};
class HandsetBrand
{
protected:
HandsetSoft *m_soft;
public:
virtual ~HandsetBrand() = default;
void SetHandsetSoft(HandsetSoft *soft)
{
m_soft = soft;
}
virtual void Run() = 0;
};
class HandsetBrandN : public HandsetBrand
{
public:
void Run() override
{
m_soft->Run();
}
};
class HandsetBrandM : public HandsetBrand
{
public:
void Run() override
{
m_soft->Run();
}
};
int main()
{
cout << "手机品牌N:" << endl;
HandsetBrand *abn = new HandsetBrandN();
HandsetGame *hgn = new HandsetGame();
abn->SetHandsetSoft(hgn);
abn->Run();
HandsetAddressList *haln = new HandsetAddressList();
abn->SetHandsetSoft(haln);
abn->Run();
cout << endl
<< "手机品牌M:" << endl;
HandsetBrand *abm = new HandsetBrandM();
HandsetGame *hgm = new HandsetGame();
abm->SetHandsetSoft(hgm);
abm->Run();
HandsetAddressList *halm = new HandsetAddressList();
abm->SetHandsetSoft(halm);
abm->Run();
delete abn;
delete hgn;
delete haln;
delete abm;
delete hgm;
delete halm;
return 0;
}
C
#include <stdio.h>
typedef struct HandsetSoft
{
void (*run)(struct HandsetSoft *self);
} HandsetSoft;
typedef struct HandsetGame
{
HandsetSoft base;
} HandsetGame;
void run_game(HandsetSoft *self)
{
printf("运行手机游戏!\n");
}
typedef struct HandsetAddressList
{
HandsetSoft base;
} HandsetAddressList;
void run_address_list(HandsetSoft *self)
{
printf("运行手机通讯录!\n");
}
typedef struct HandsetBrand
{
HandsetSoft *m_soft;
} HandsetBrand;
void set_handset_soft(HandsetBrand *self, HandsetSoft *soft)
{
self->m_soft = soft;
}
typedef struct HandsetBrandN
{
HandsetBrand base;
} HandsetBrandN;
void run_brandN(HandsetBrand *self)
{
self->m_soft->run(self->m_soft);
}
typedef struct HandsetM
{
HandsetBrand base;
} HandsetM;
void run_brandM(HandsetBrand *self)
{
self->m_soft->run(self->m_soft);
}
int main()
{
HandsetSoft game_software = {.run = run_game};
HandsetSoft address_list_software = {.run = run_address_list};
HandsetGame game = {.base = game_software};
HandsetAddressList address_list = {.base = address_list_software};
HandsetBrandN brandN = {};
set_handset_soft((HandsetBrand *)&brandN, (HandsetSoft *)&game);
printf("手机品牌N:\n");
run_brandN((HandsetBrand *)&brandN);
set_handset_soft((HandsetBrand *)&brandN, (HandsetSoft *)&address_list);
run_brandN((HandsetBrand *)&brandN);
HandsetM brandM = {};
set_handset_soft((HandsetBrand *)&brandM, (HandsetSoft *)&game);
printf("\n手机品牌M:\n");
run_brandM((HandsetBrand *)&brandM);
set_handset_soft((HandsetBrand *)&brandM, (HandsetSoft *)&address_list);
run_brandM((HandsetBrand *)&brandM);
return 0;
}