M螺旋矩阵 ||(leetcode59)
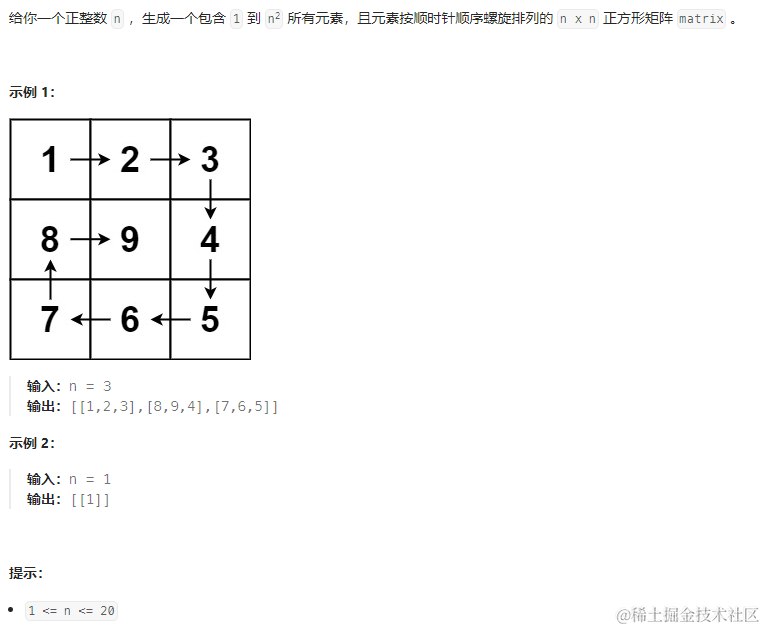
var generateMatrix = function(n) {
const maxNum = n * n;
let curNum = 1;
const matrix = new Array(n).fill(0).map(() => new Array(n).fill(0));
let row = 0,column = 0;
const directions = [[0, 1], [1, 0], [0, -1], [-1, 0]];
let directionIndex = 0;
while (curNum <= maxNum) {
matrix[row][column] = curNum;
curNum++;
const nextRow = row + directions[directionIndex][0], nextColumn = column + directions[directionIndex][1];
if (nextRow < 0 || nextRow >= n || nextColumn < 0 || nextColumn >= n || matrix[nextRow][nextColumn] !== 0) {
directionIndex = (directionIndex + 1) % 4;
}
row = row + directions[directionIndex][0];
column = column + directions[directionIndex][1];
}
return matrix;
};
M螺旋矩阵(leetcode54)
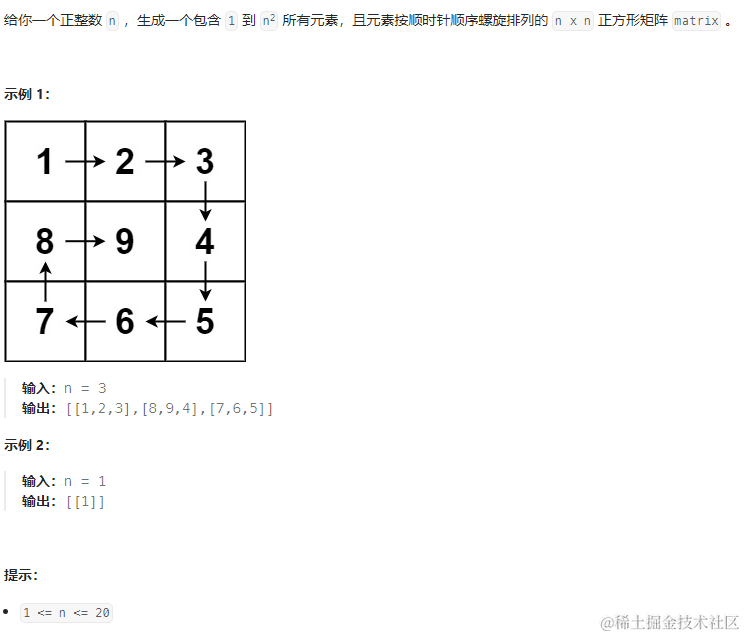
var spiralOrder = function(matrix) {
let left = 0;
let right = matrix[0].length - 1;
let top = 0;
let bottom = matrix.length - 1;
let res = [];
while(true) {
for(let i = left; i <= right; i++) {
res.push(matrix[top][i]);
}
top++;
if (top > bottom) break;
for (let i = top; i <= bottom; i++) {
res.push(matrix[i][right]);
}
right--;
if (right < left) break;
for (let i = right; i >= left; i--) {
res.push(matrix[bottom][i]);
}
bottom--;
if(bottom < top) break;
for(let i = bottom; i >= top;i--) {
res.push(matrix[i][left]);
}
left++;
if(left > right) break;
}
return res;
};
E螺旋遍历二维数组(leetcode146)
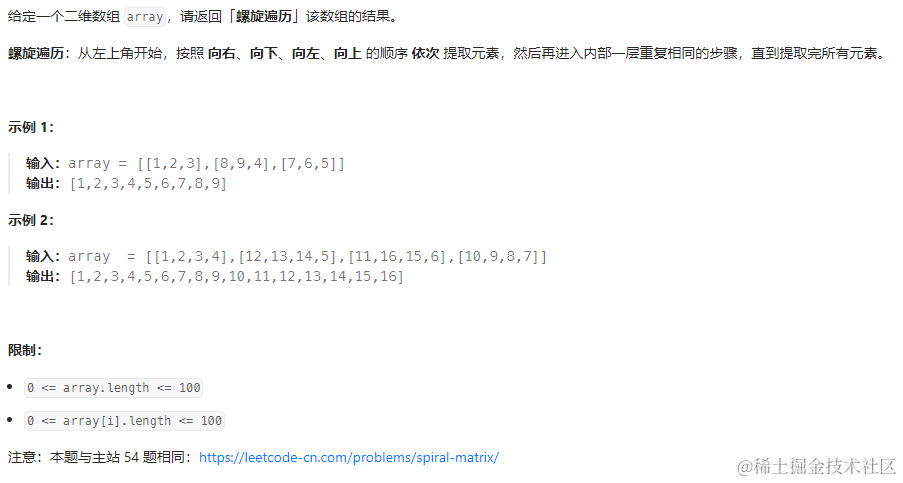
var spiralArray = function(array) {
if (!array.length || !array[0].length) {
return [];
}
const rows = array.length, columns = array[0].length;
const visited = new Array(rows).fill(0).map(() => new Array(columns).fill(false));
const total = rows * columns;
const order = new Array(total).fill(0);
let directionIndex = 0, row = 0, column = 0;
const directions = [[0, 1], [1, 0], [0, -1], [-1, 0]];
for (let i = 0; i < total; i++) {
order[i] = array[row][column];
visited[row][column] = true;
const nextRow = row + directions[directionIndex][0], nextColumn = column + directions[directionIndex][1];
if (!(0 <= nextRow && nextRow < rows && 0 <= nextColumn && nextColumn < columns && !(visited[nextRow][nextColumn]))) {
directionIndex = (directionIndex + 1) % 4;
}
row += directions[directionIndex][0];
column += directions[directionIndex][1];
}
return order;
};