elasticsearch我已经装了ik,中文分词器。已经使用容器搭建了集群。之前在我的博客-elasticsearch入门中,已经介绍了http请求操纵es的基本功能,java API功能和他一样,只是从http请求换成了javaApi操作。
springBoot里继承了elasticsearch,他是spring-data的一个子模块,里面的主要核心就是ElasticsearchRepository。只要你写一个interface继承他,就可以用基本的CRUD操作es。
如果你想要http那样灵活的操作es,他提供了elasticsearchRestTemplate,你可以把他看成一个小型的http,你要通过代码的形式将http中的内容表示出来,在通过这个template发送。
导入依赖
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-elasticsearch</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.12</version>
</dependency>
</dependencies>
填写es地址
在你的springBoot项目里,我的是application.properties,加一个自己的地址,这样启动springBoot的时候就会自动识别了。
spring.elasticsearch.uris=http://192.168.9.102:9200

做一个数据类来测试
最好创建一个与es数据相对应的实体类
我的es:

我的实体类:
package com.example.elaticsearchtest2.entity;
import lombok.Data;
import org.springframework.data.annotation.Id;
import org.springframework.data.elasticsearch.annotations.DateFormat;
import org.springframework.data.elasticsearch.annotations.Document;
import org.springframework.data.elasticsearch.annotations.Field;
import org.springframework.data.elasticsearch.annotations.FieldType;
//lombok自动加get,set以及构造
@Data
//指定索引
@Document(indexName = "game")
public class GameEntity {
@Id
private String id;
/**
* 游戏名
*/
@Field(type = FieldType.Text, analyzer = "ik_max_word")
private String name;
/**
* 创建日期
*/
@Field(type = FieldType.Date, format = DateFormat.date, pattern = "yyyy-MM-dd HH:mm:ss")
private String date;
/**
* 游戏介绍
*/
@Field(type = FieldType.Text, analyzer = "ik_max_word", searchAnalyzer = "ik_smart")
private String introduction;
/**
* 主页面地址
*/
@Field(type = FieldType.Text)
private String url;
}
其中analyzer代表的是分词方式,ik_max_word是ik中文分词器的细粒度模式,关于ik具体请看我的博客 elatiscsearch入门教程中有写到
最基本的ElasticsearchRepository
他的标准用法是用【数据实体类的interface-repository】去继承它
首先写一个,关于game索引的数据实体类的interface
package com.example.elaticsearchtest2.controller;
import com.example.elaticsearchtest2.entity.GameEntity;
import org.springframework.data.elasticsearch.repository.ElasticsearchRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface GameRepository extends ElasticsearchRepository<GameEntity,Long> {
}
其中,继承参数的那里GameEntity就是我之前写的数据实体,Long代表的是行数类型
一定要加一个repository注解,这样才能被springBoot识别
测试类,一定要用@SpringBootTest注解,这样才能模拟springBoot的运行
然后,自动注入刚刚的接口gameRepository,然后在写一个test方法,调用查询做测试
package com.example.elaticsearchtest2;
import com.example.elaticsearchtest2.controller.GameRepository;
import com.example.elaticsearchtest2.entity.GameEntity;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class ConnectTest {
@Autowired
GameRepository gameRepository ;
@Test
public void test1(){
Iterable<GameEntity> all = gameRepository.findAll();
for(GameEntity one:all){
System.out.println(one);
}
}
}
右键运行,成功显示出es的数据
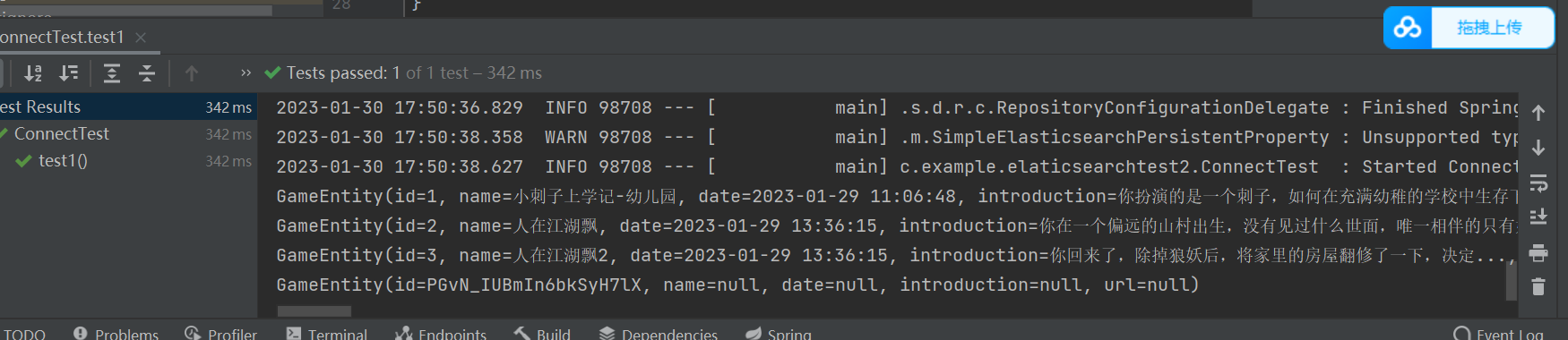
灵活的elasticsearchRestTemplate
参考文章:
https://www.cnblogs.com/tanghaorong/p/16365684.html#_label0