以下记录的是,我在学习中的一些学习笔记,这篇笔记是自己学习的学习大杂烩,主要用于记录,方便查找
1、相关学习链接
https://www.rust-lang.org/zh-CN/governance/ RUST 官网博客
https://kaisery.github.io/trpl-zh-cn/(最好的入门书)Rust 程序设计语言简体中文版
https://doc.rust-lang.org/1.30.0/book/second-edition/ch00-00-introduction.html
https://play.rust-lang.org/ 在线rust平台
https://doc.rust-lang.org/stable/rust-by-example rust-by-example
第三方库:https://crates.io/
库的使用https://docs.rs/
https://poly000.github.io/perf-book-zh/introduction.html rust性能优化
Rust编写的开源代码编辑器:https://docs.lapce.dev/
https://os.phil-opp.com/zh-CN/ Rust编写了一个小操作系统
https://github.com/LukeMathWalker/zero-to-production “在 Rust 中零到生产”的代码,一本关于使用 Rust 进行 API 开发的书
https://github.com/SeaDve/Kooha Rust写的优雅录屏工具
2、Rust语言环境搭建
在 Linux 或 macOS 上安装 rustup
安装:curl --proto '=https' --tlsv1.3 https://sh.rustup.rs -sSf | sh
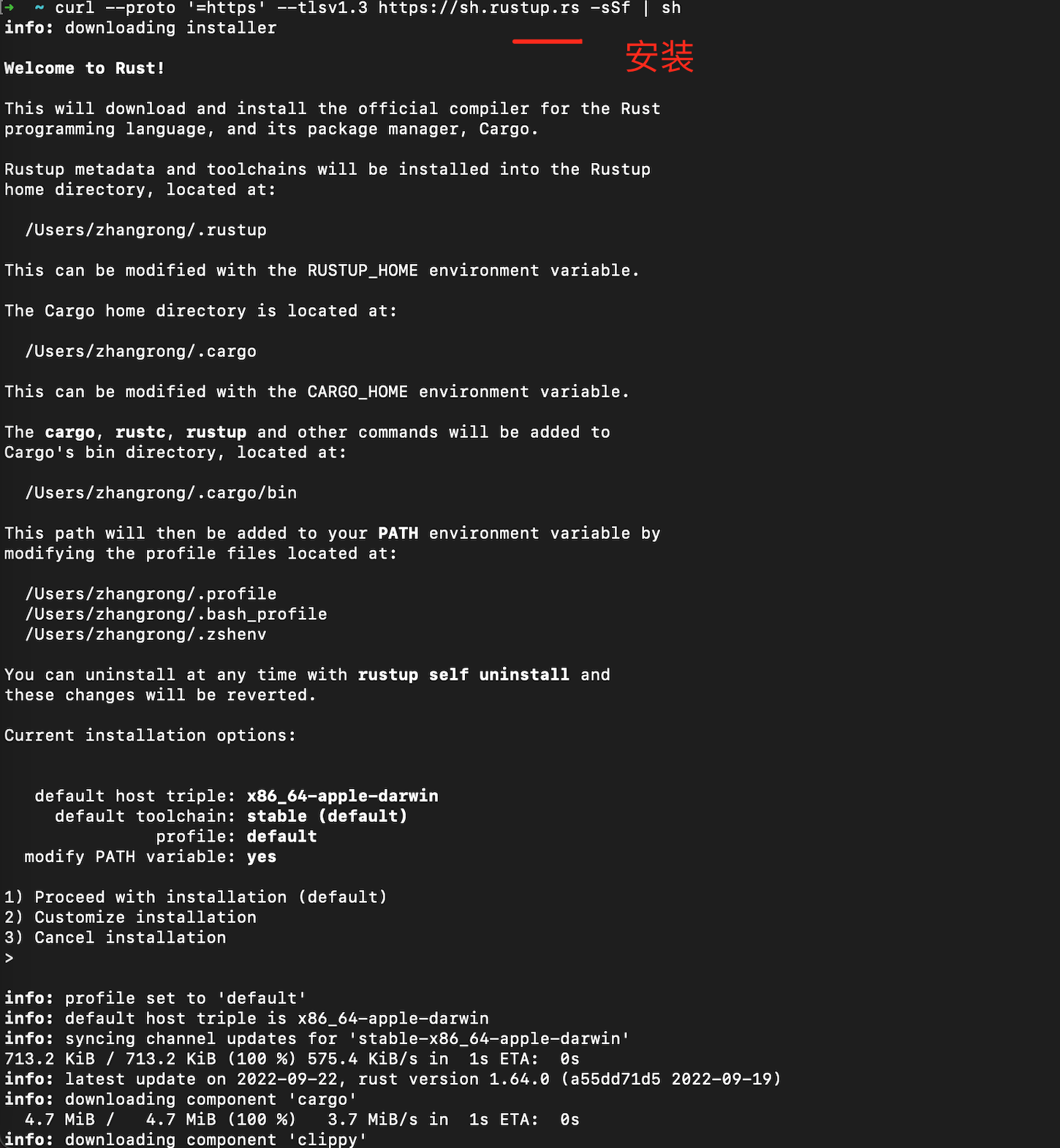
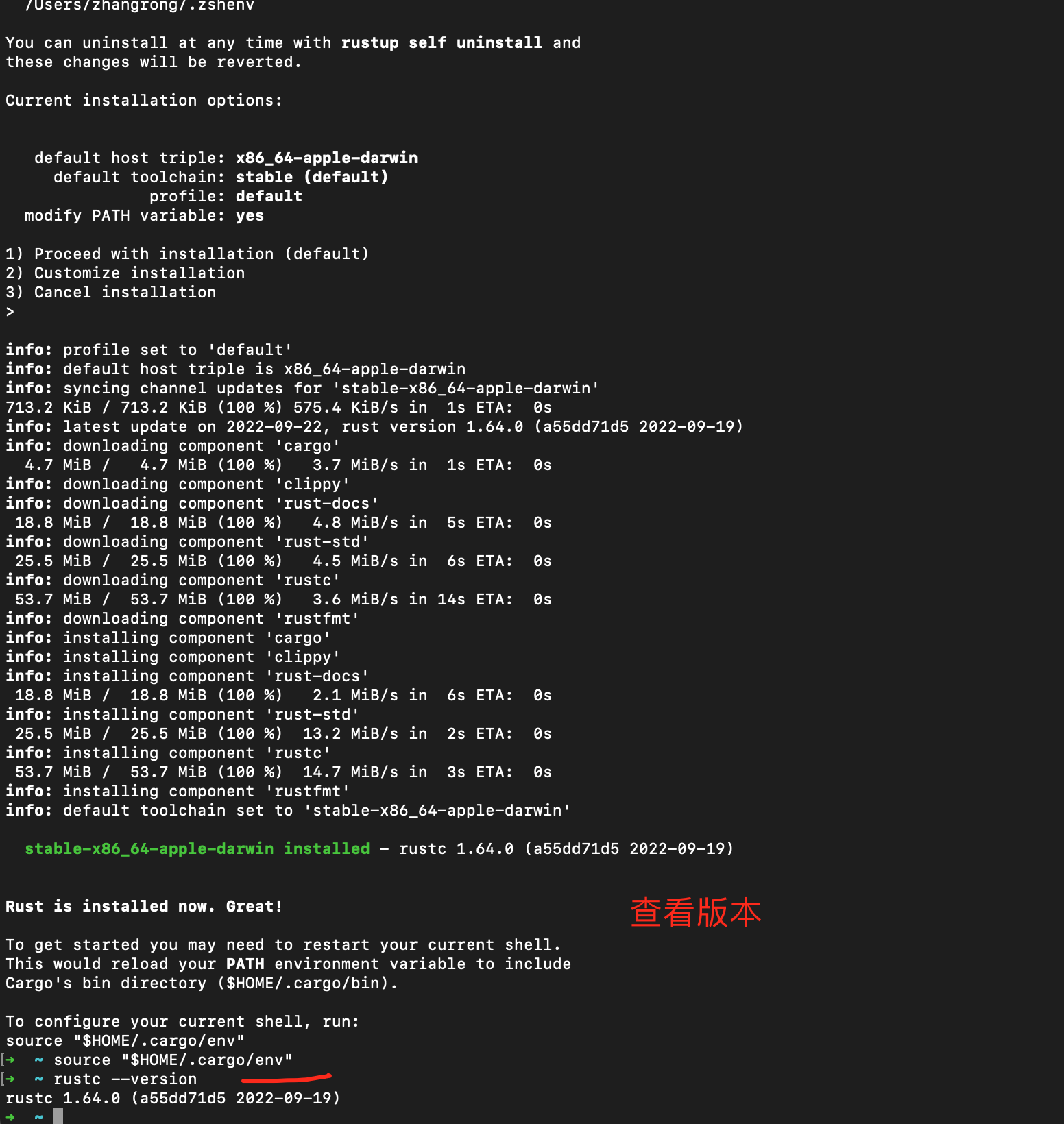
更新:rustup update
卸载: rustup self uninstall
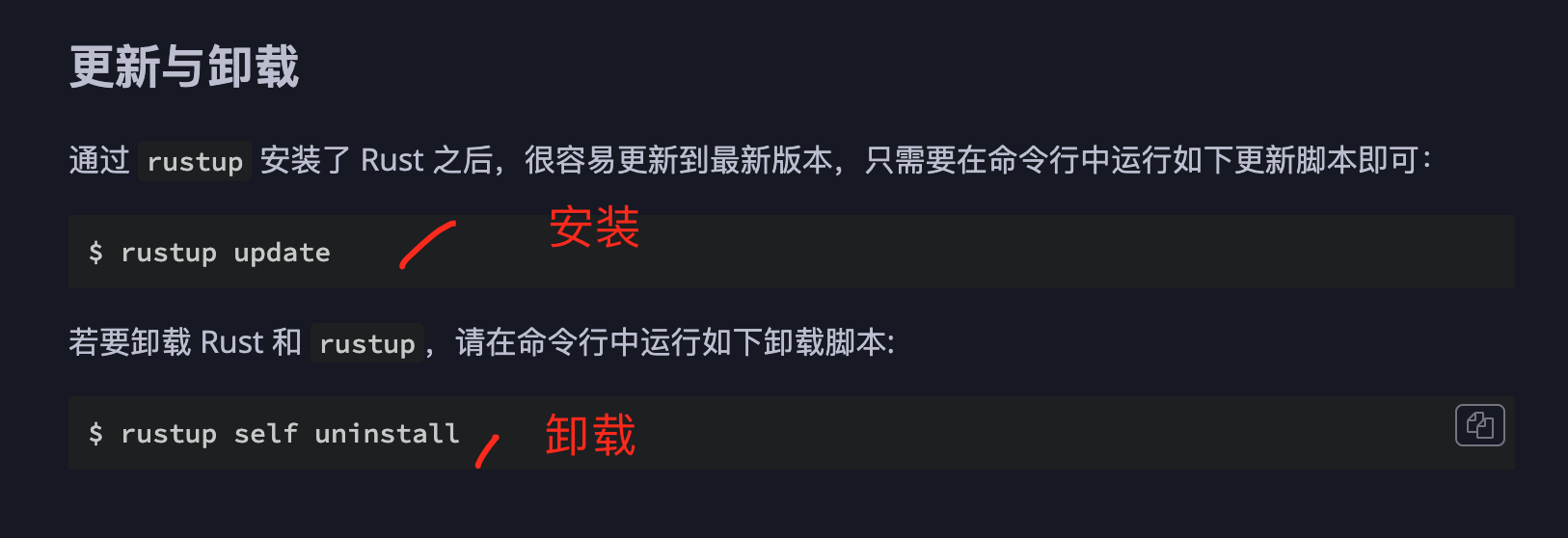
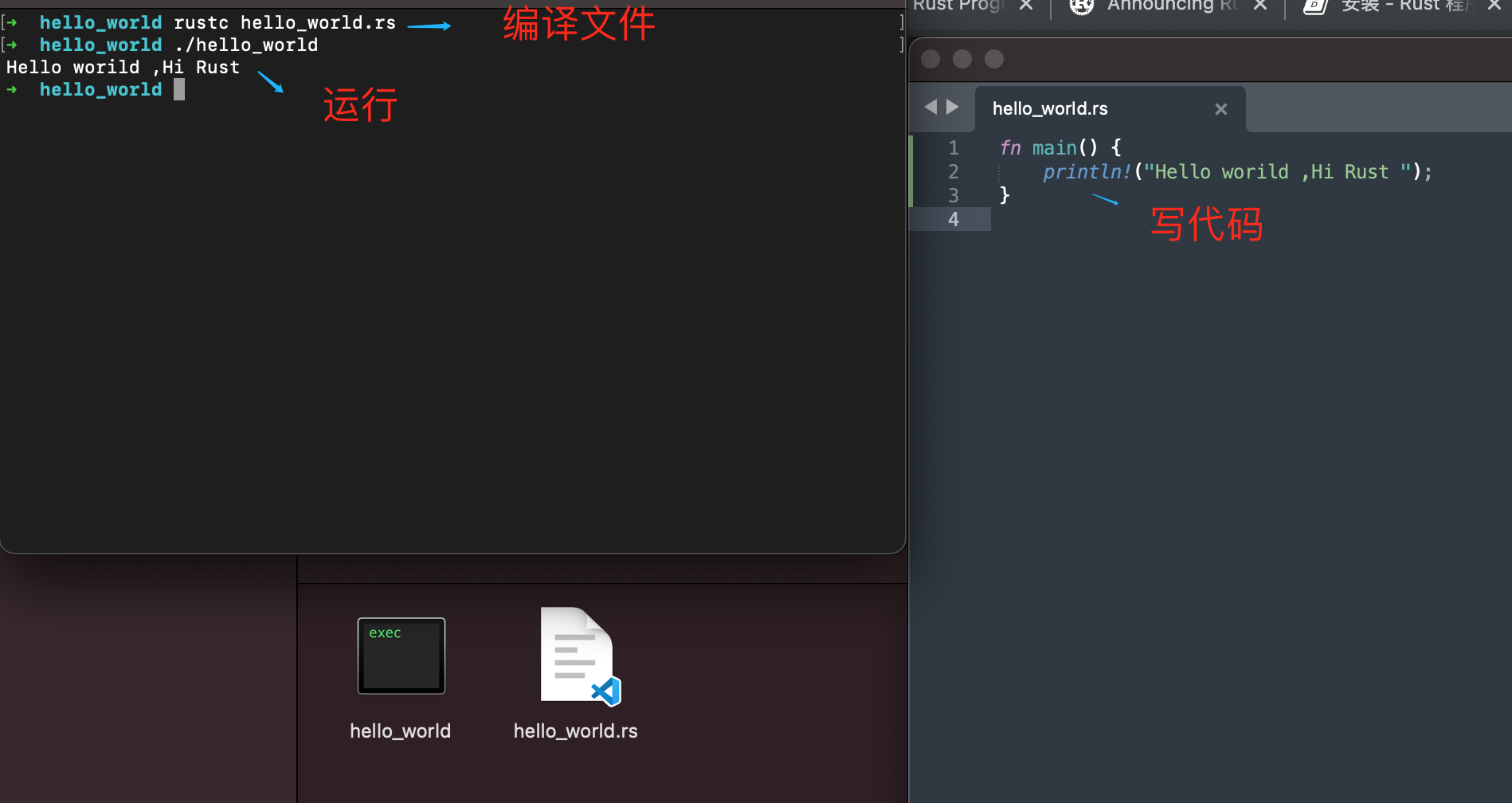

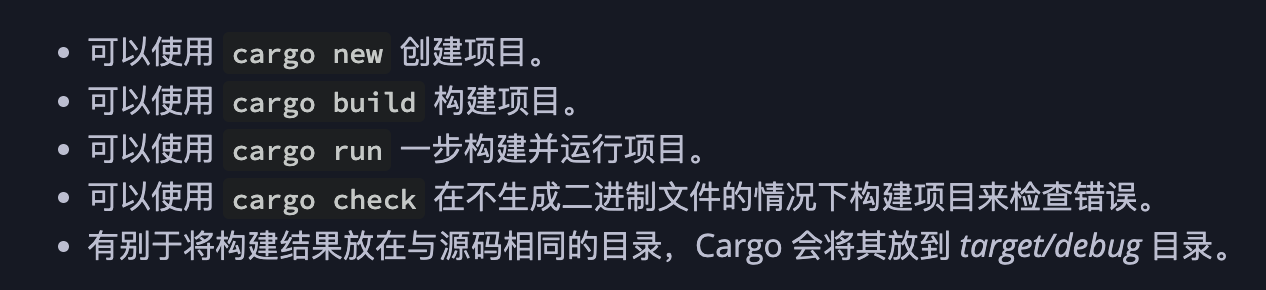
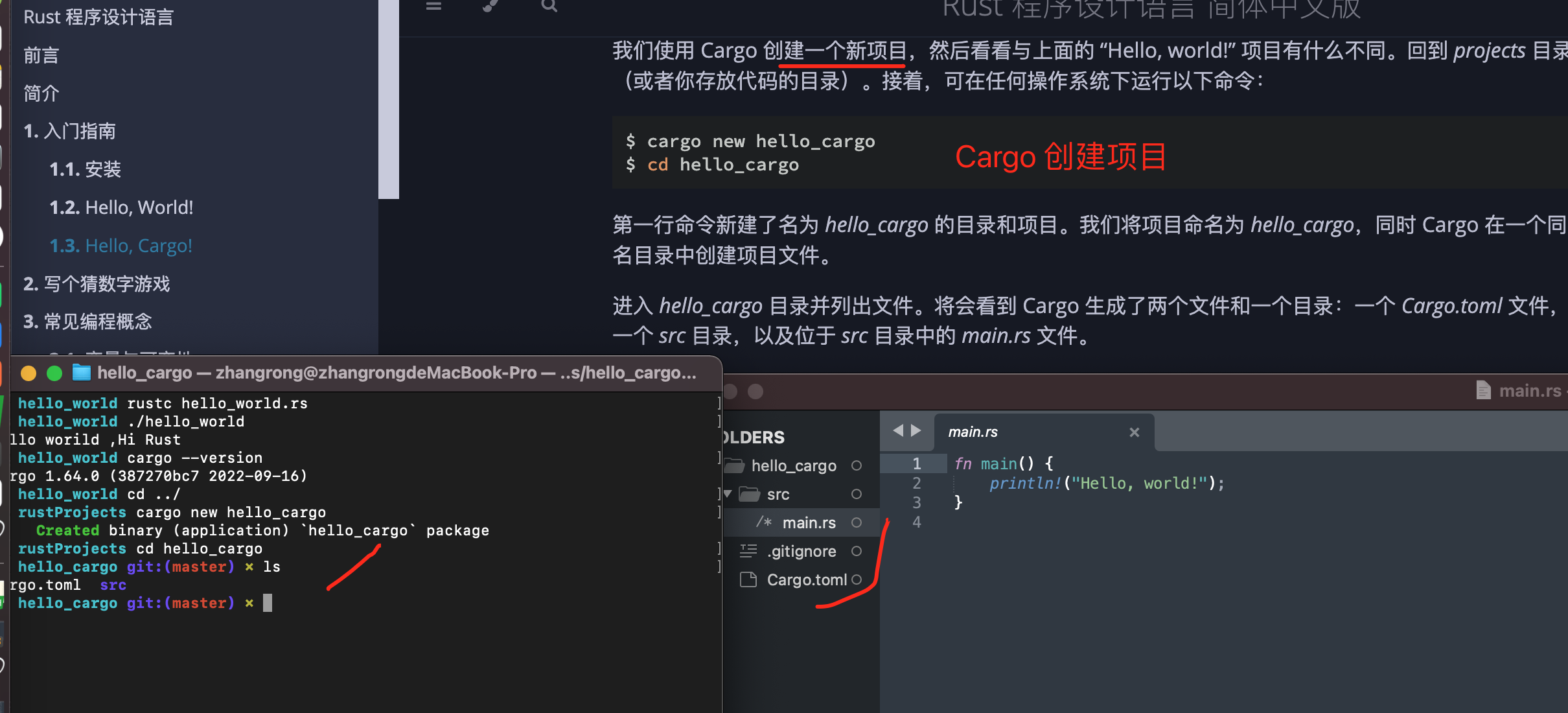
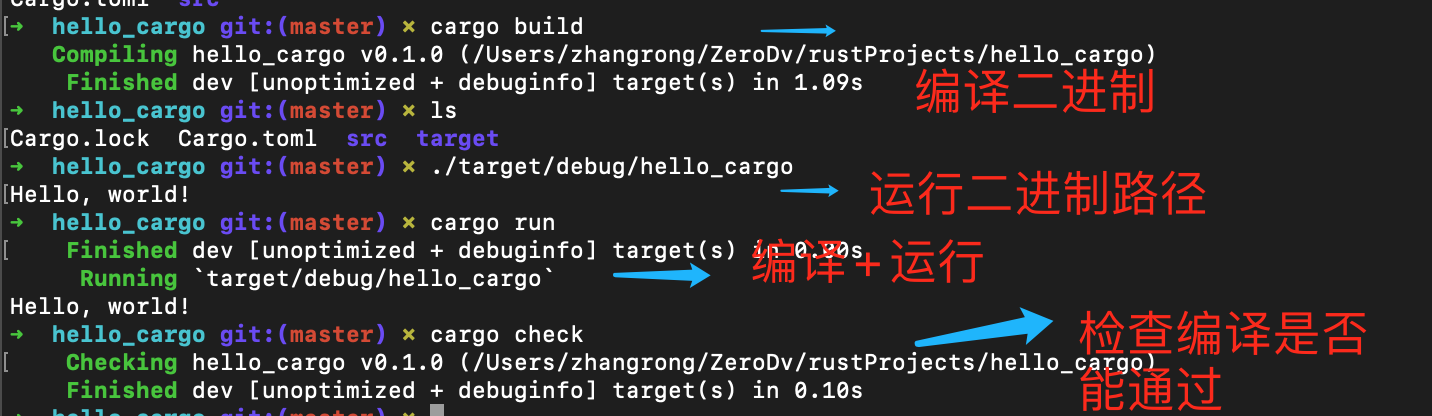
cargo build --release 并使用 target/release 下的可执行文件进行测试
cargo doc 可生成注释文档
Rust IDE 环境:
https://blog.csdn.net/weixin_41195426/article/details/108896151
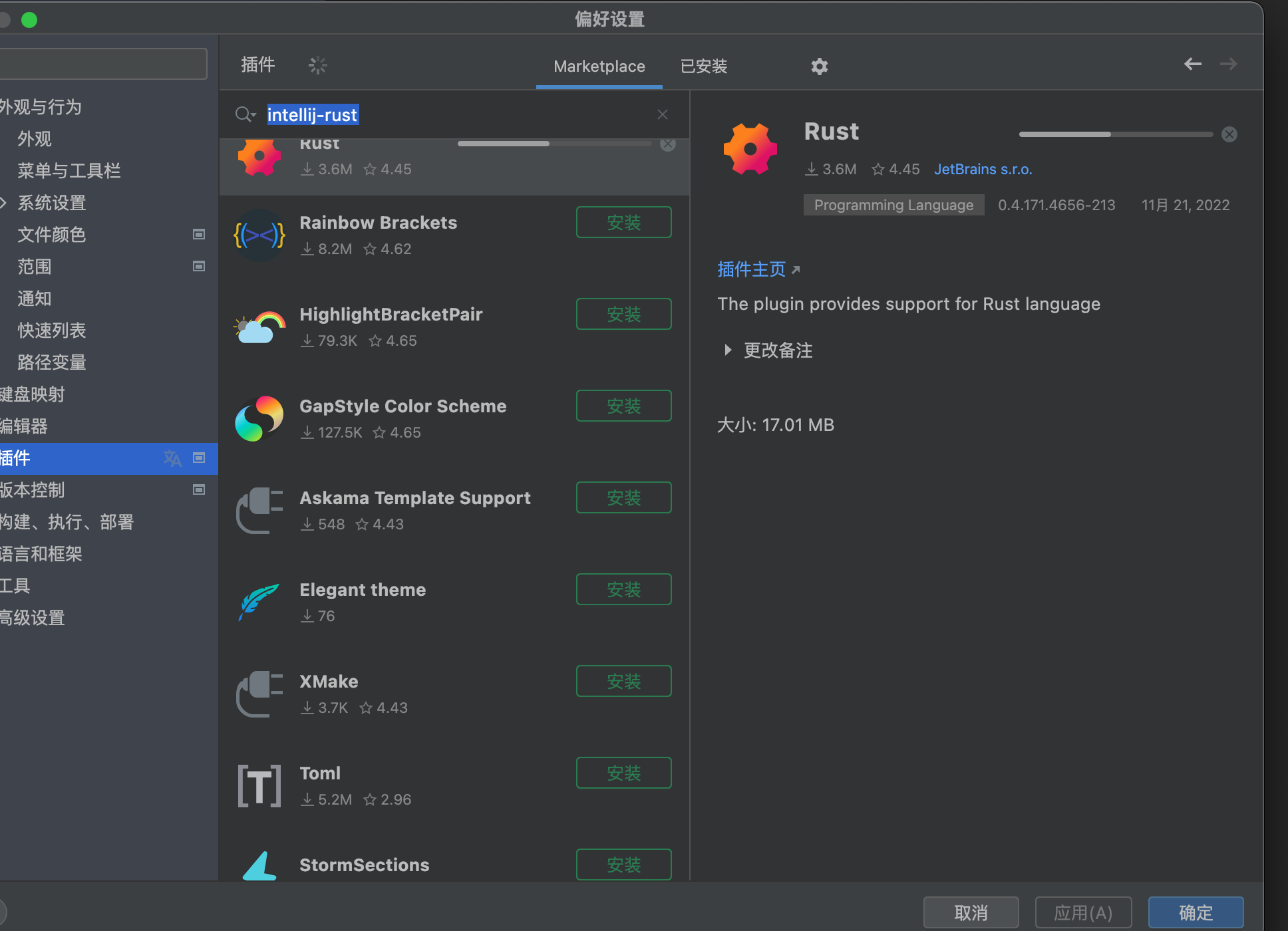

Rust下载速度慢:https://zhuanlan.zhihu.com/p/468840038
3、Rust语言知识
变量:只有 let 定义的变量不能变 ,let 和 mut 定义的变量 可变。
fn main() {
let y=6;
let mut x = 5;
println!("The value of x is: {x}");
x = 6;
println!("The value of x is: {x}");
}
常量: const THREE_HOURS_IN_SECONDS: u32 = 60 * 60 * 3;
数据类型:
1、标量类型:整型、浮点型、布尔类型和字符类型。
let x = 2.0; let y: f32 = 3.0; let sum = 5 + 10; let f: bool = false; let z: char = 'ℤ';
2、复合类型: 元组(tuple)和数组(array)
元祖: let tup: (i32, f64, u8) = (500, 6.4, 1);
let tup = (500, 6.4, 1); let (x, y, z) = tup;
println!("The value of y is: {y}");
let x: (i32, f64, u8) = (500, 6.4, 1); let five_hundred = x.0;
数组: let a = [1, 2, 3, 4, 5]; let first = a[0];
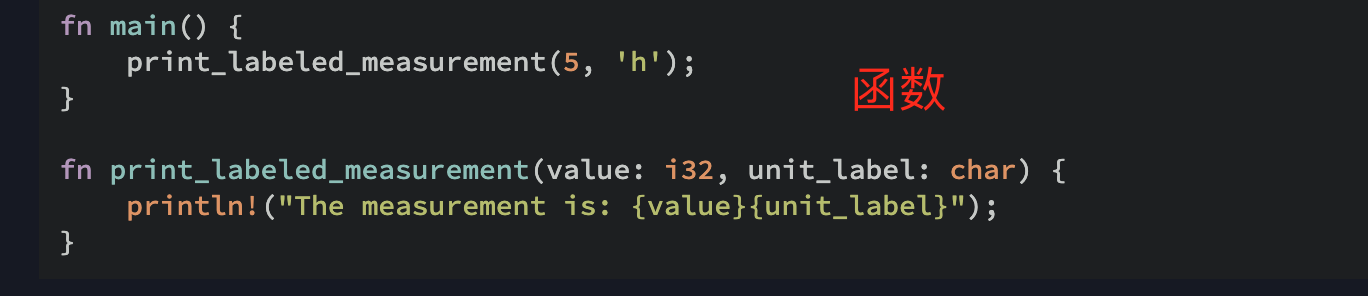
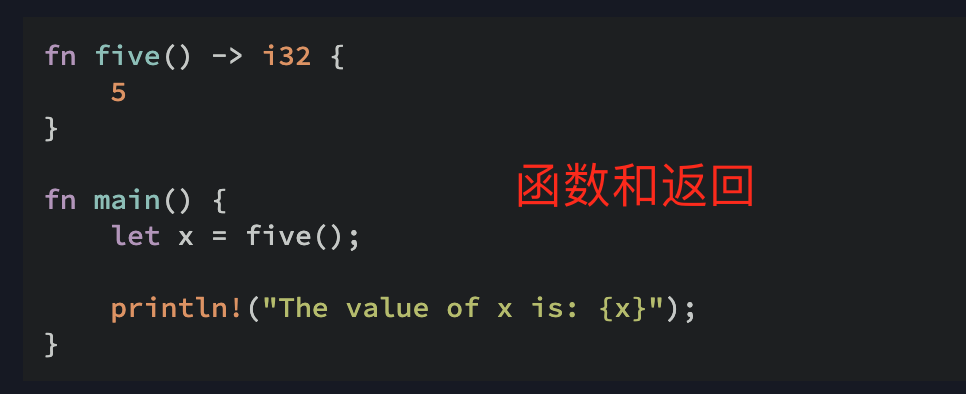
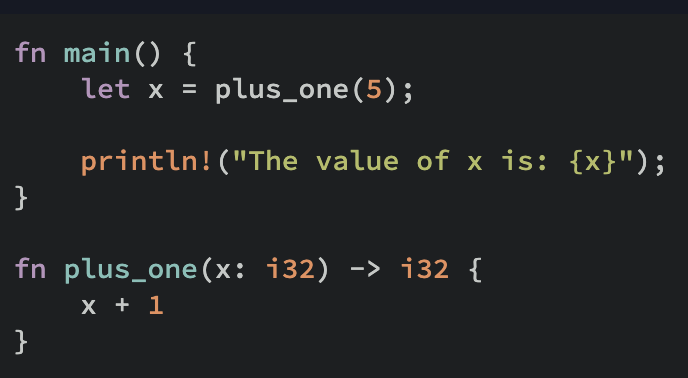
单行注释: // hello, world 文档注释:
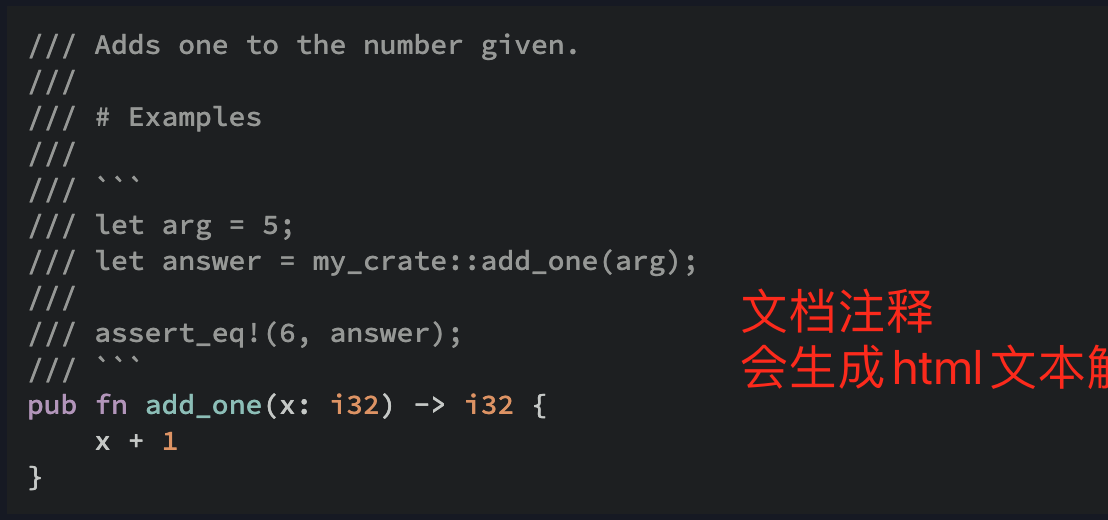
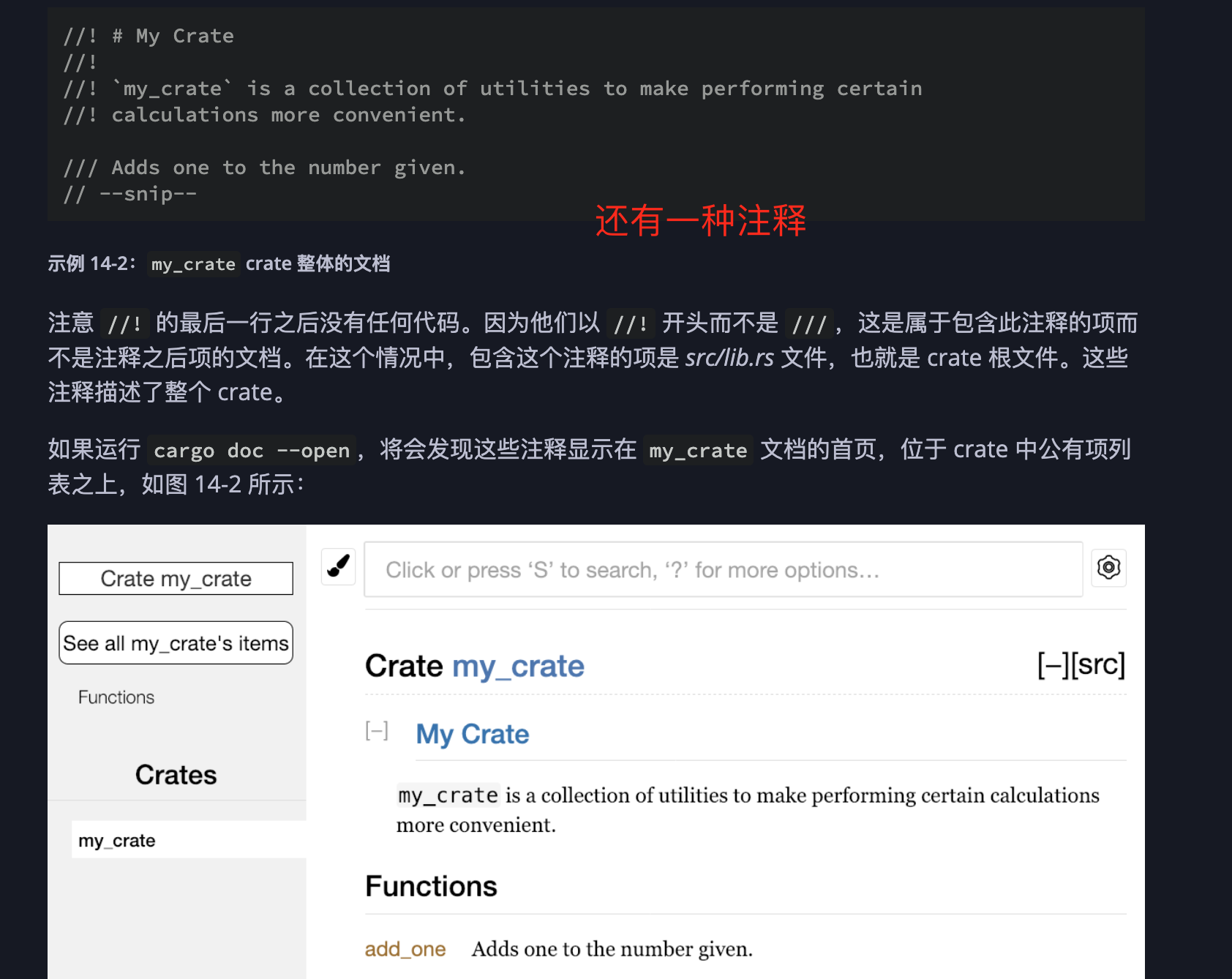
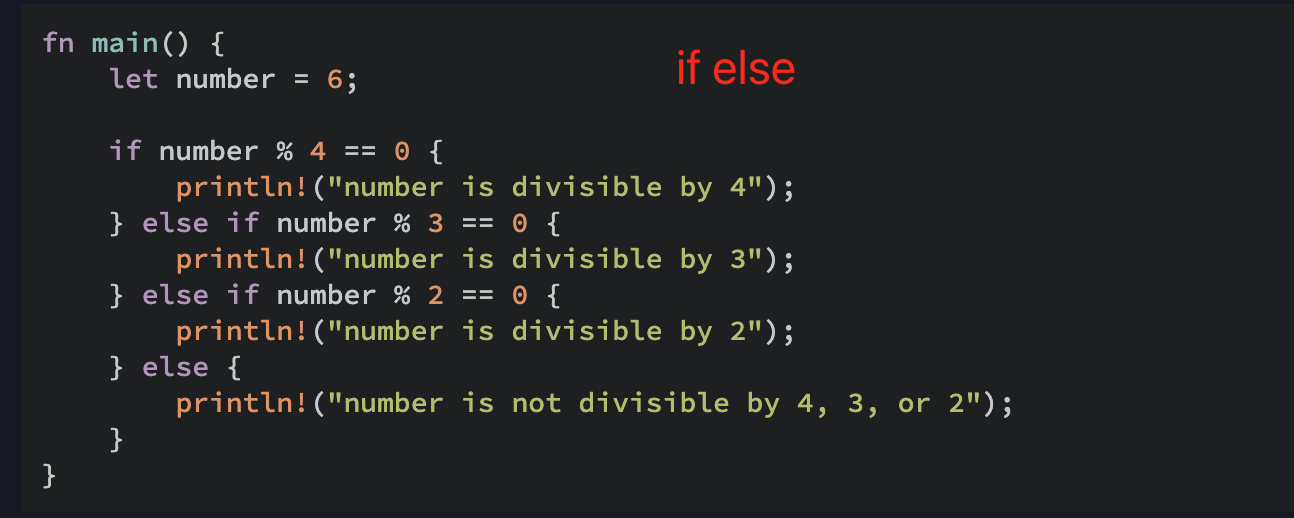
Rust 有三种循环:loop、while 和 for
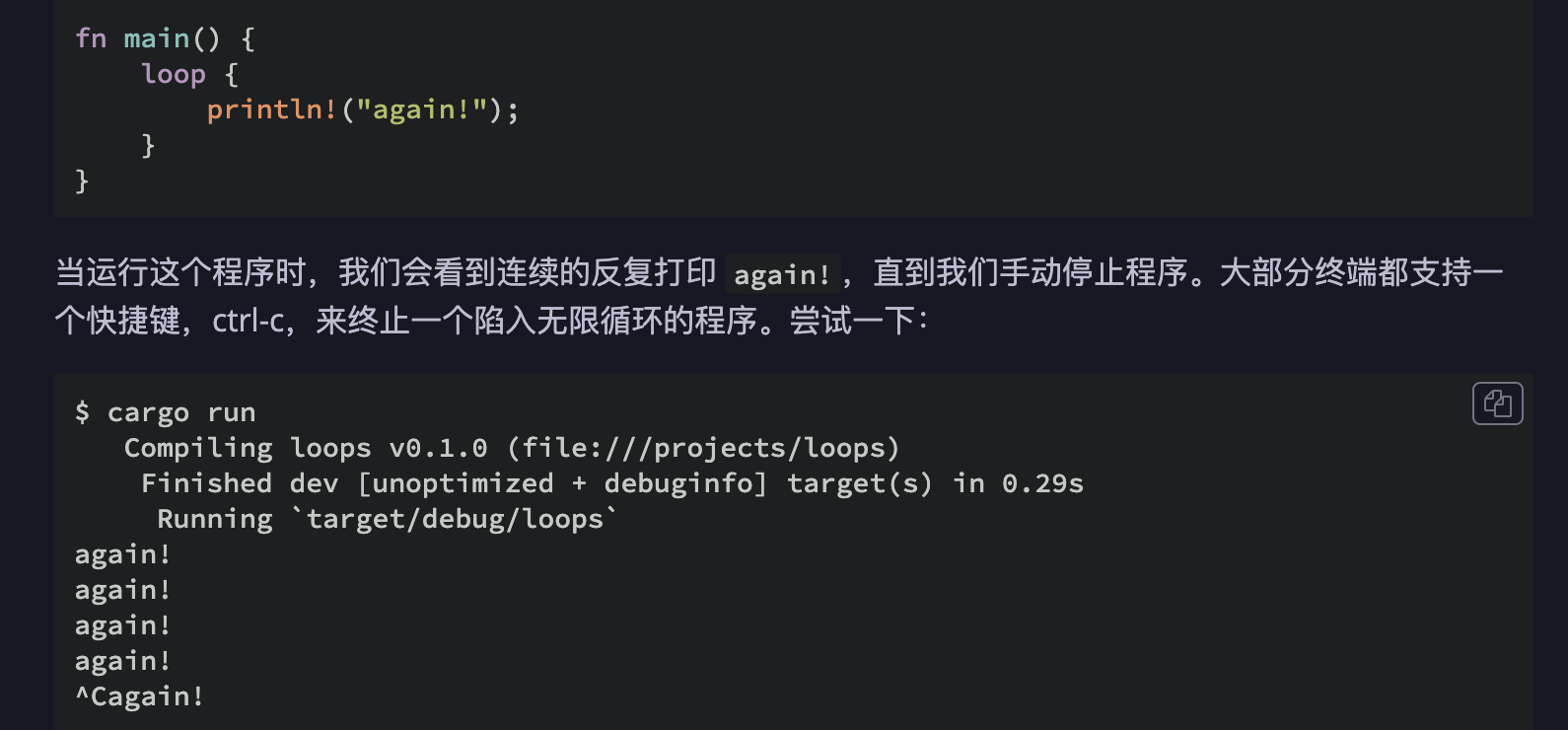
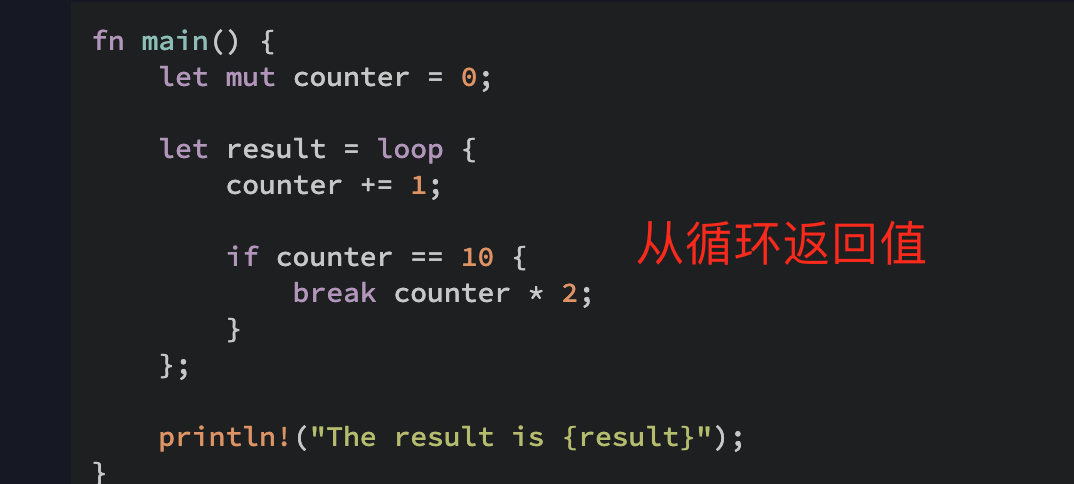
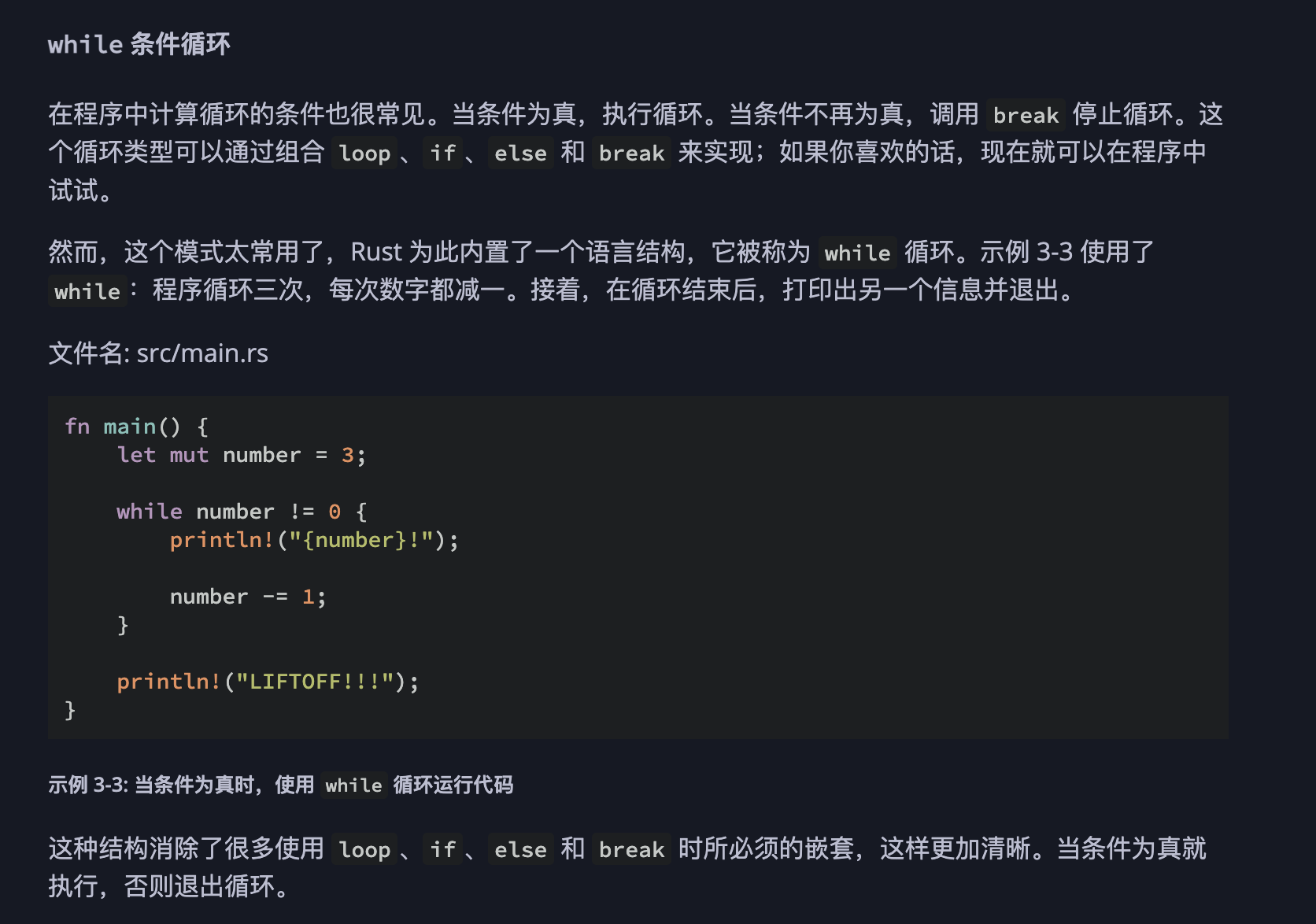
fn main() {
let a = [10, 20, 30, 40, 50];
let mut index = 0;
while index < 5 {
println!("the value is: {}", a[index]);
index += 1;
}
}
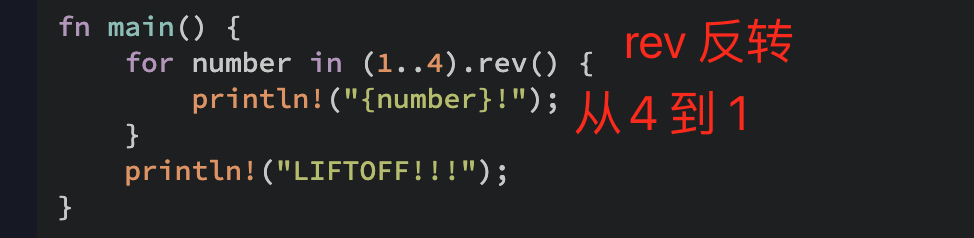
let a = [10, 20, 30, 40, 50];
for element in a {
println!("the value is: {element}");
}
结构体:
struct User {
active: bool,
username: String,
email: String,
sign_in_count: u64,
}
fn main() {
let user1 = User {
email: String::from("someone@example.com"),
username: String::from("someusername123"),
active: true,
sign_in_count: 1,
};
}
fn main() {
let mut user1 = User {
email: String::from("someone@example.com"),
username: String::from("someusername123"),
active: true,
sign_in_count: 1,
};
user1.email = String::from("anotheremail@example.com");
}
fn build_user(email: String, username: String) -> User {
User {
email: email,
username: username,
active: true,
sign_in_count: 1,
}
}
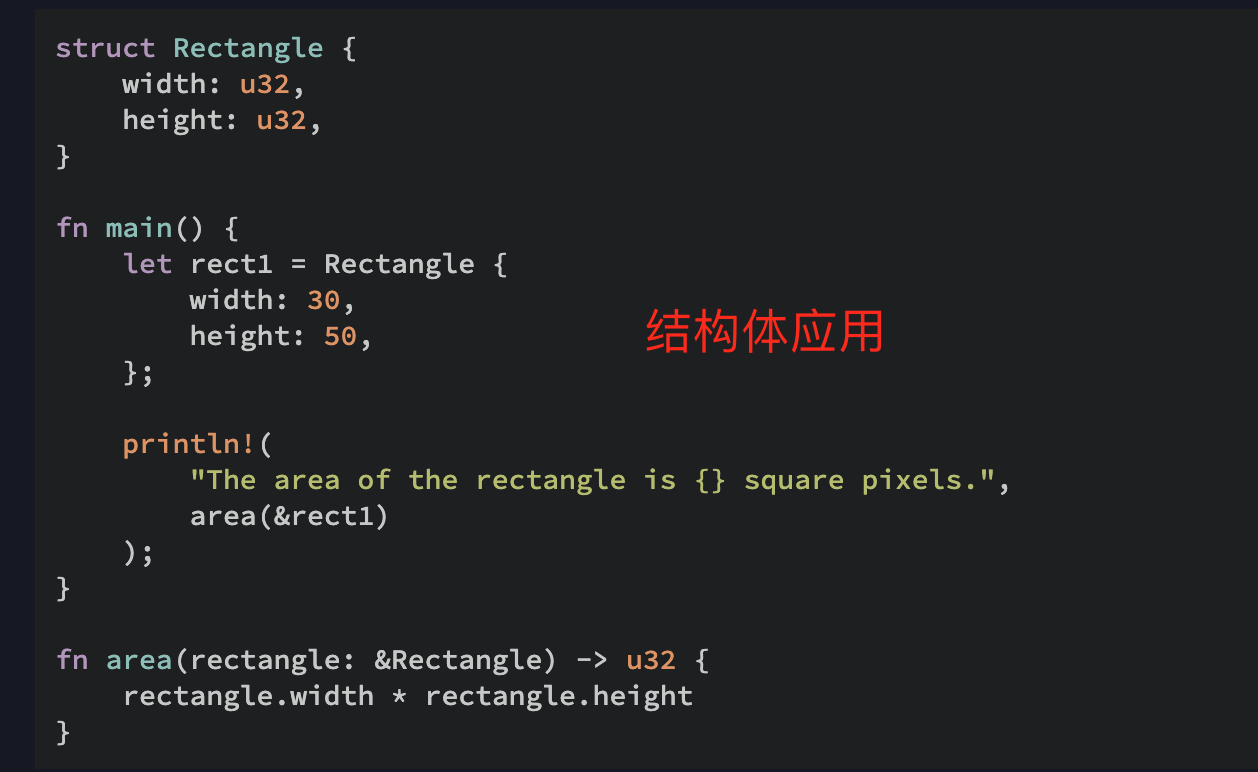
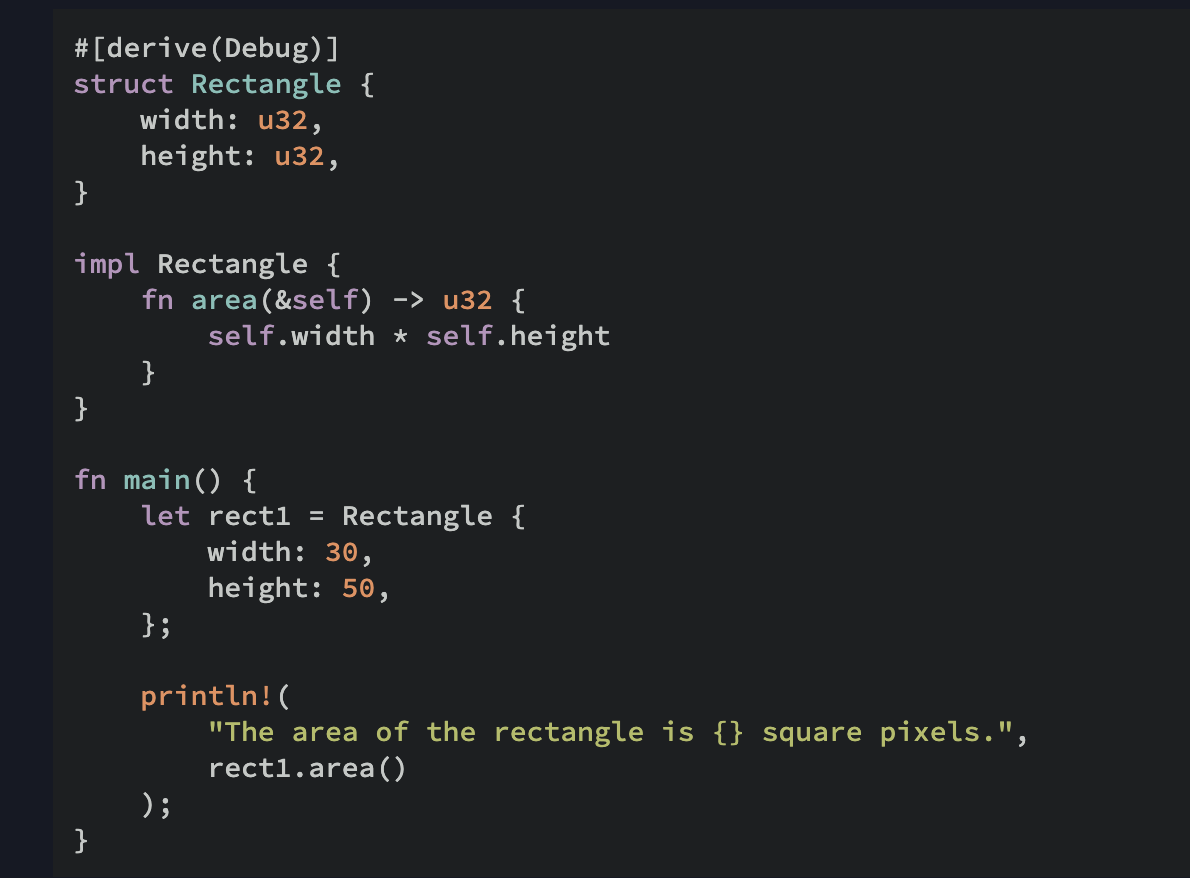
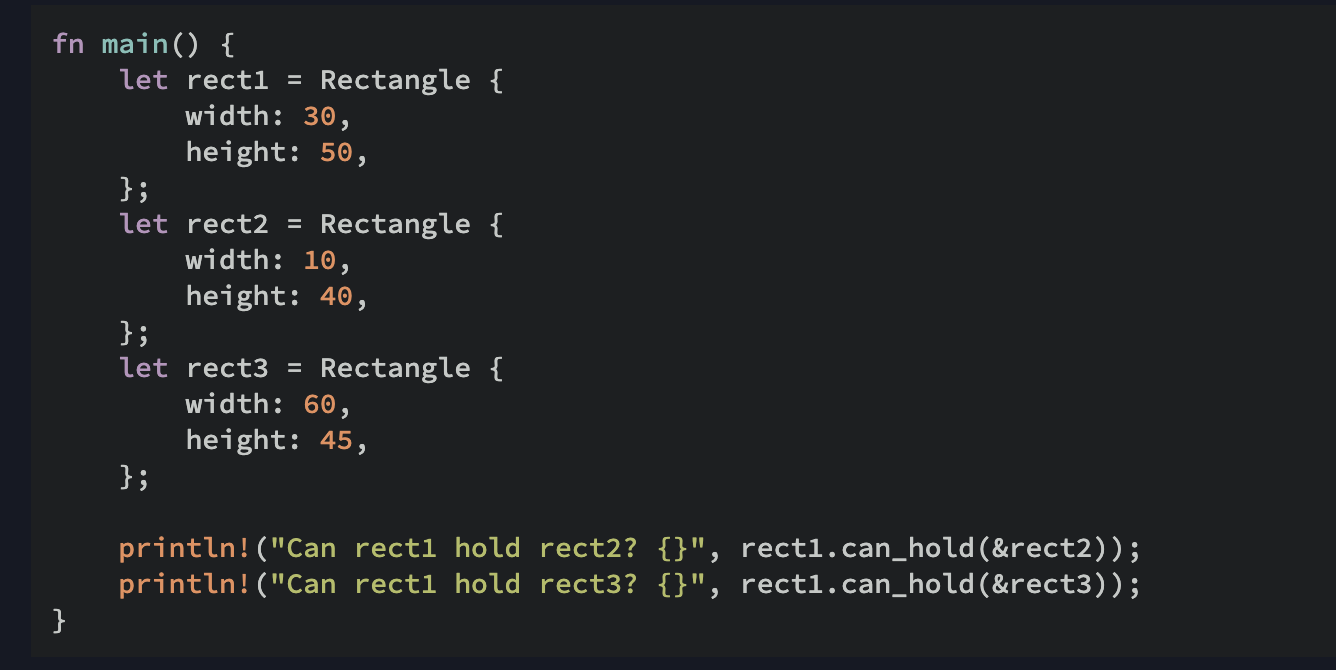
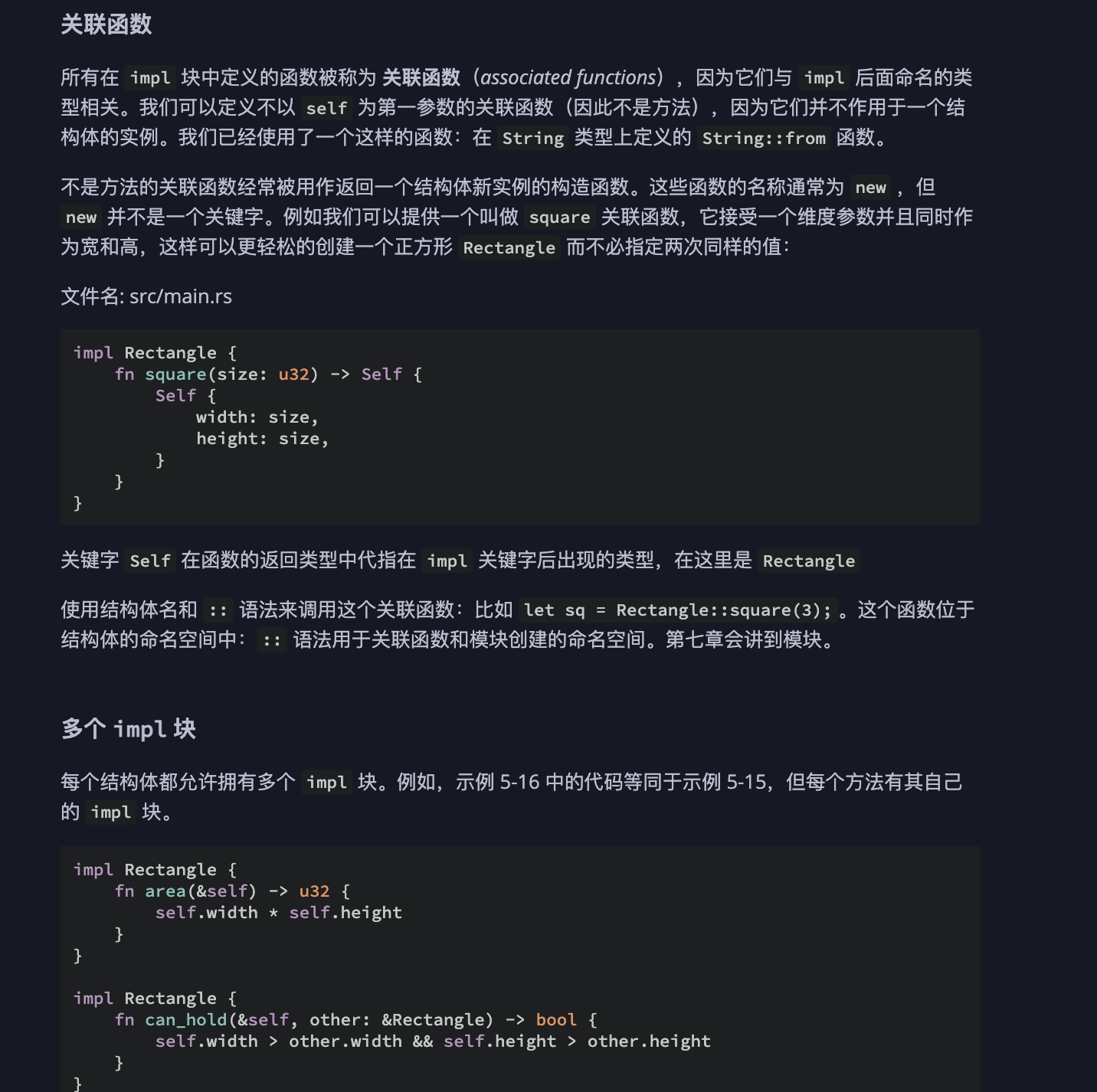
枚举:
enum IpAddrKind {
V4,
V6,
}
let four = IpAddrKind::V4;
let six = IpAddrKind::V6;
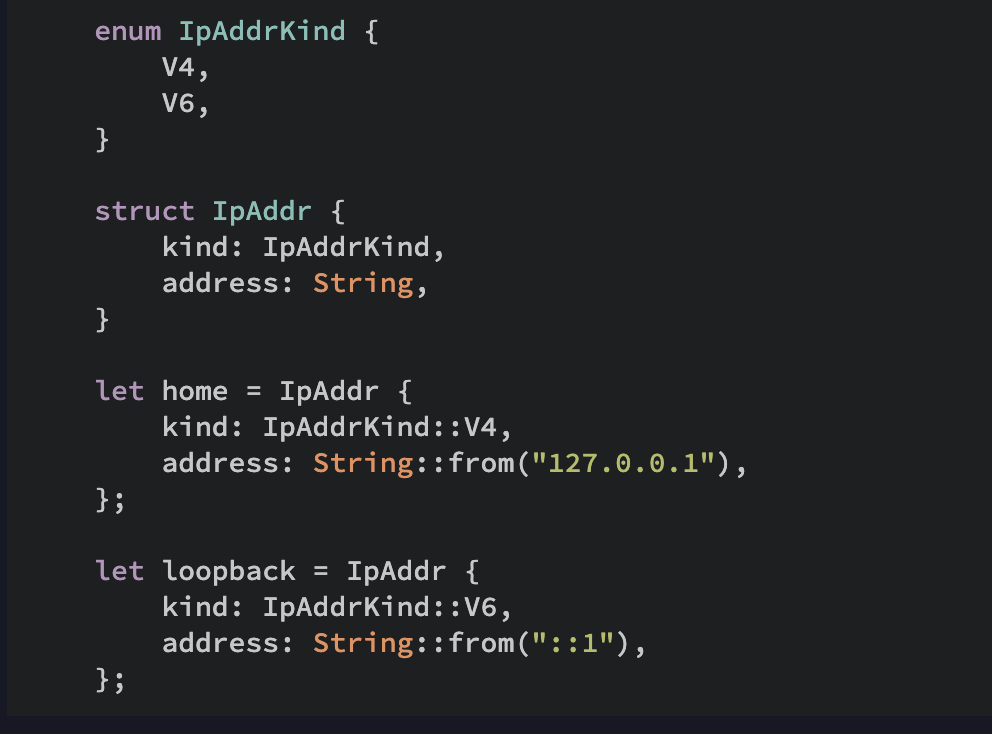
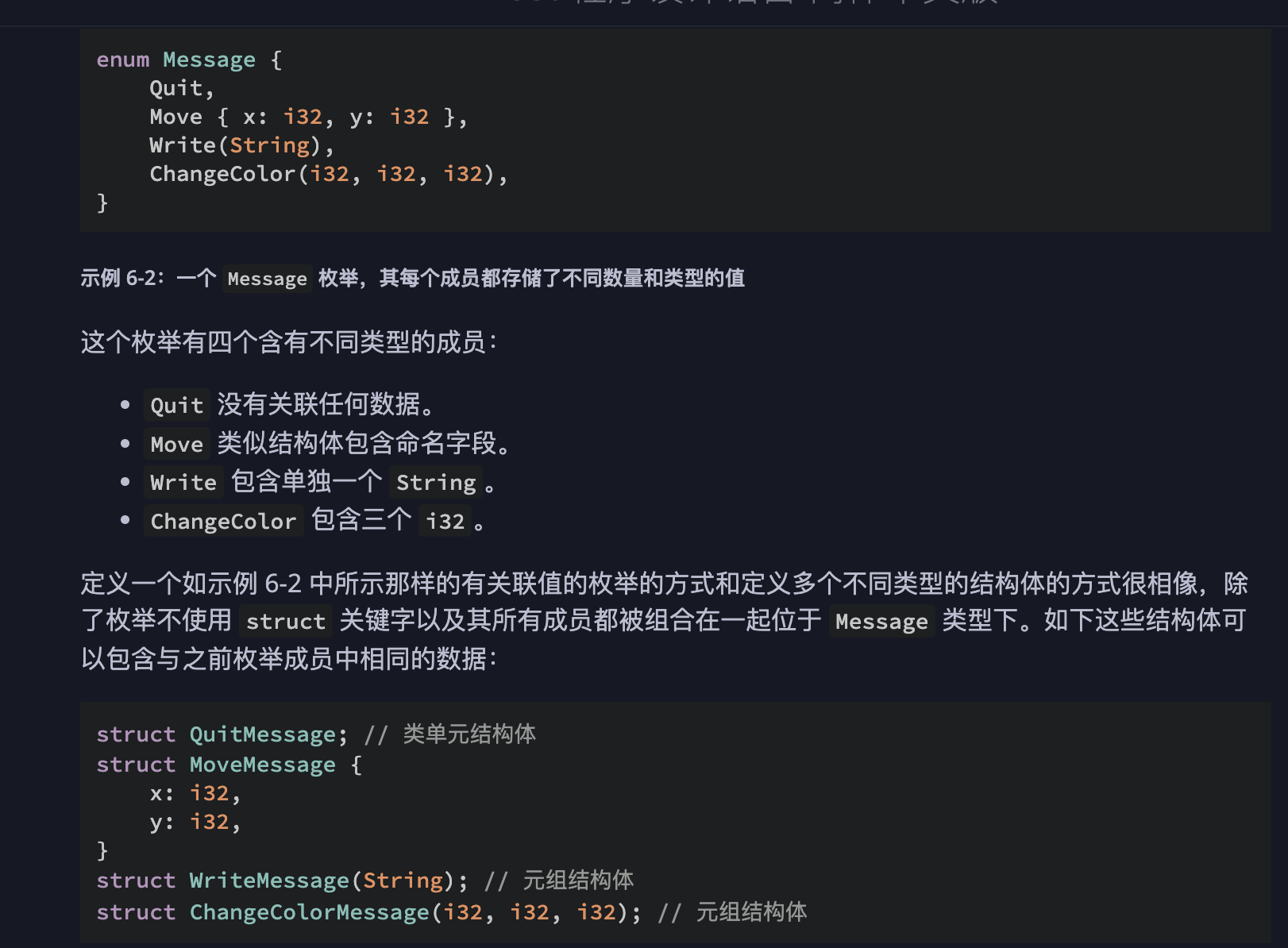
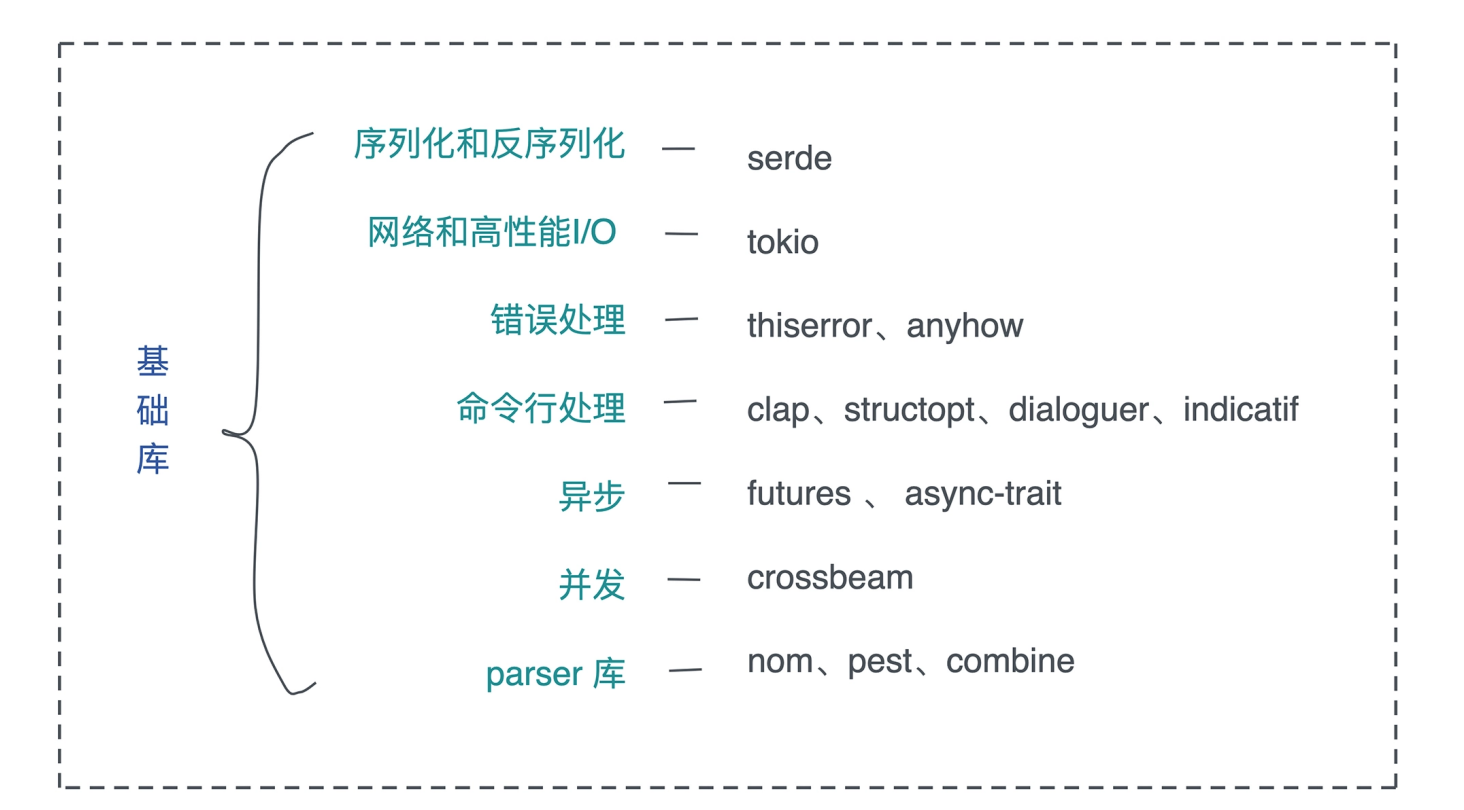
异步框架:Rocket 和 tide ,actix-web ,gotham-and-thruster 和tower ,hyper ,warp 和 http库 ,hyper-tower-http-http-body 层
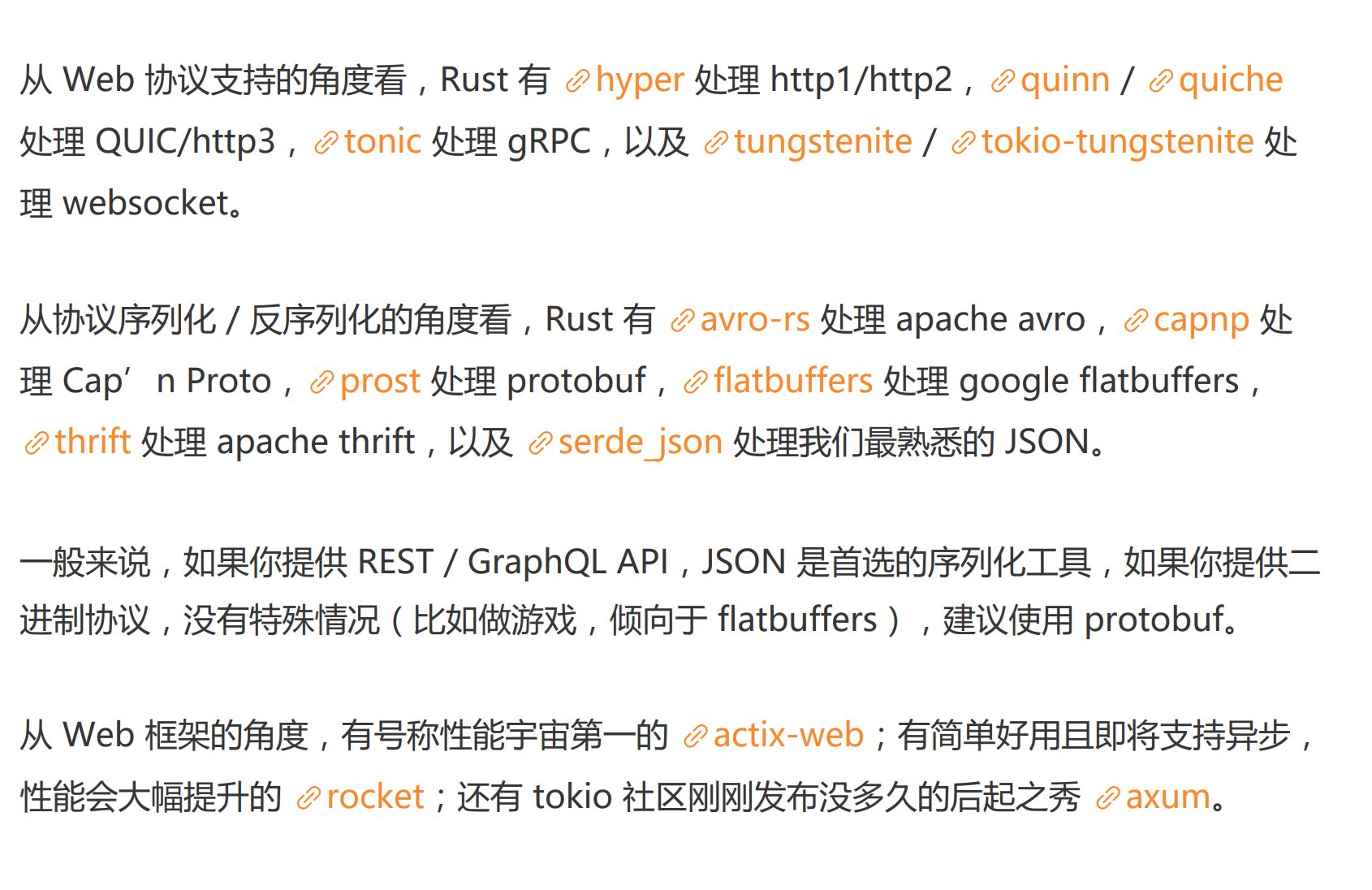
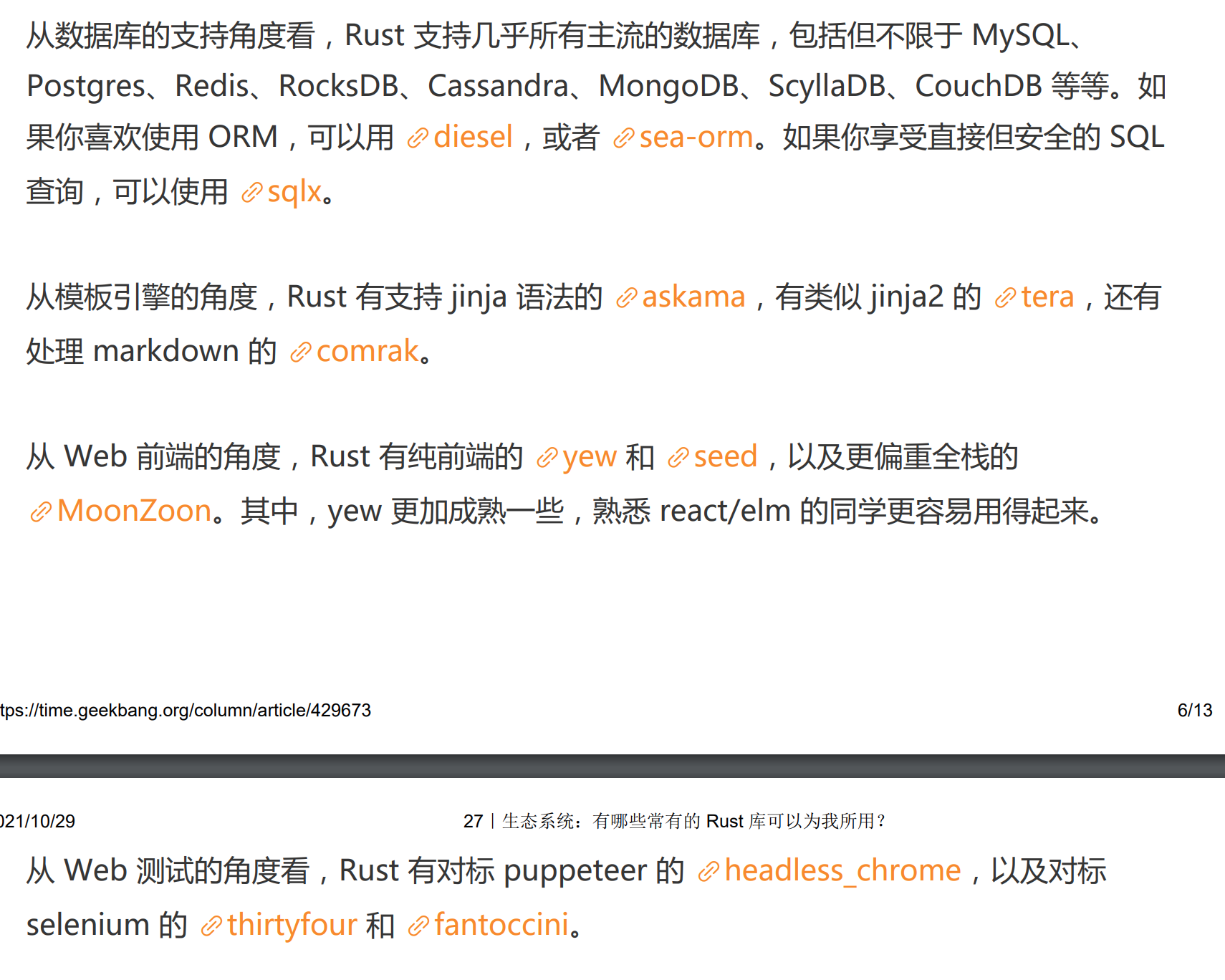
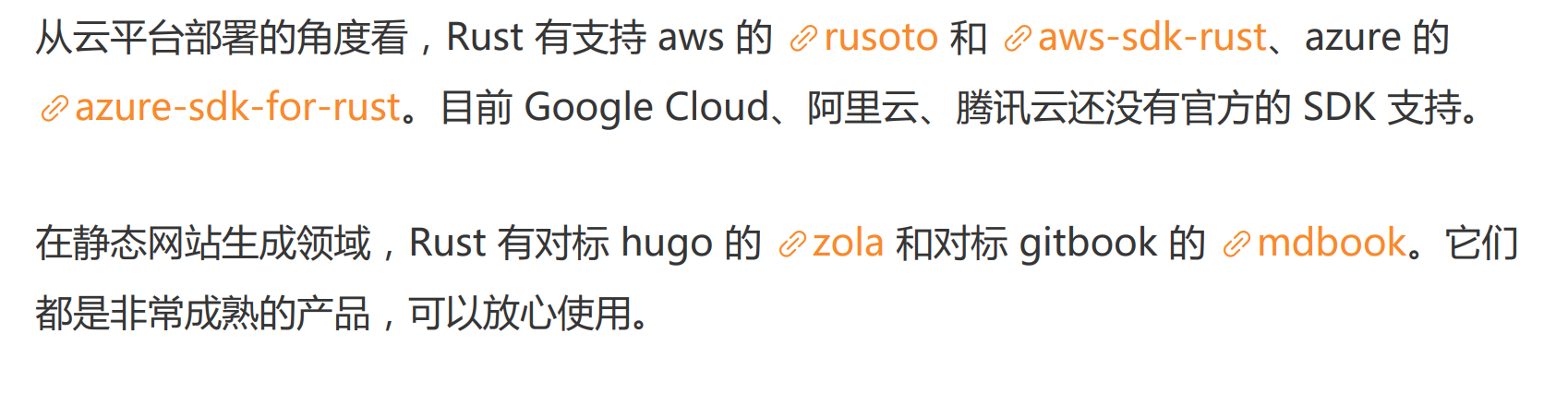
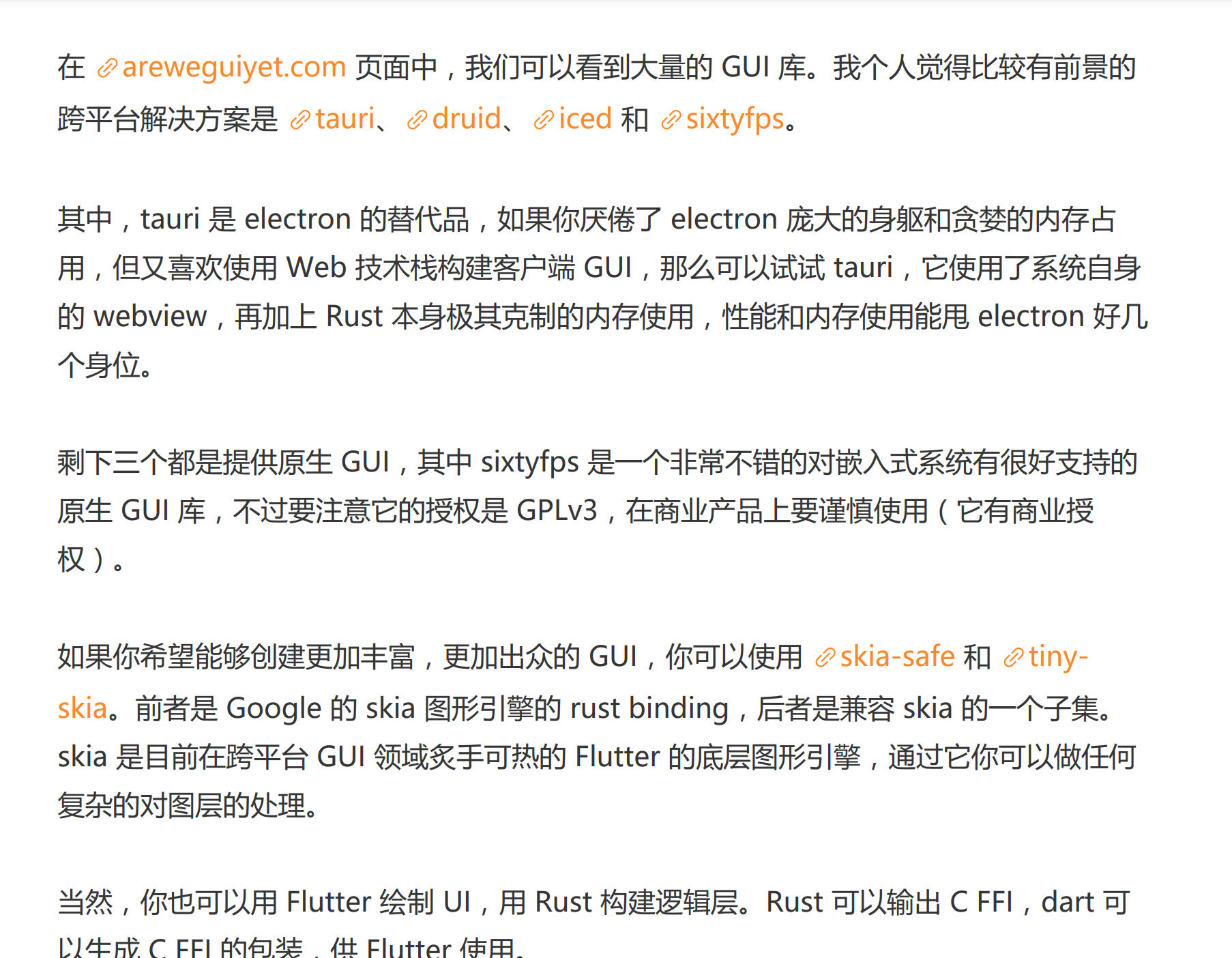
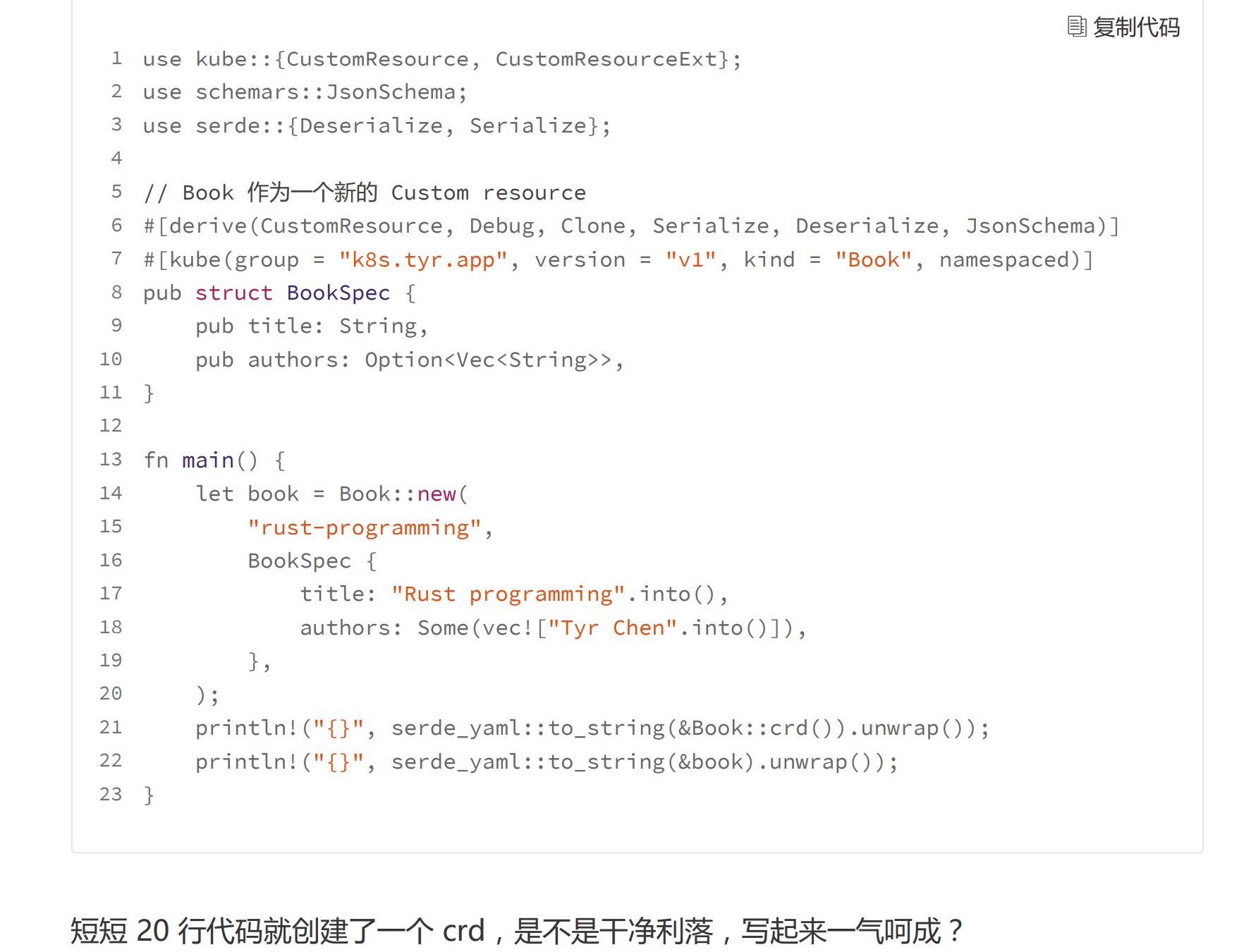
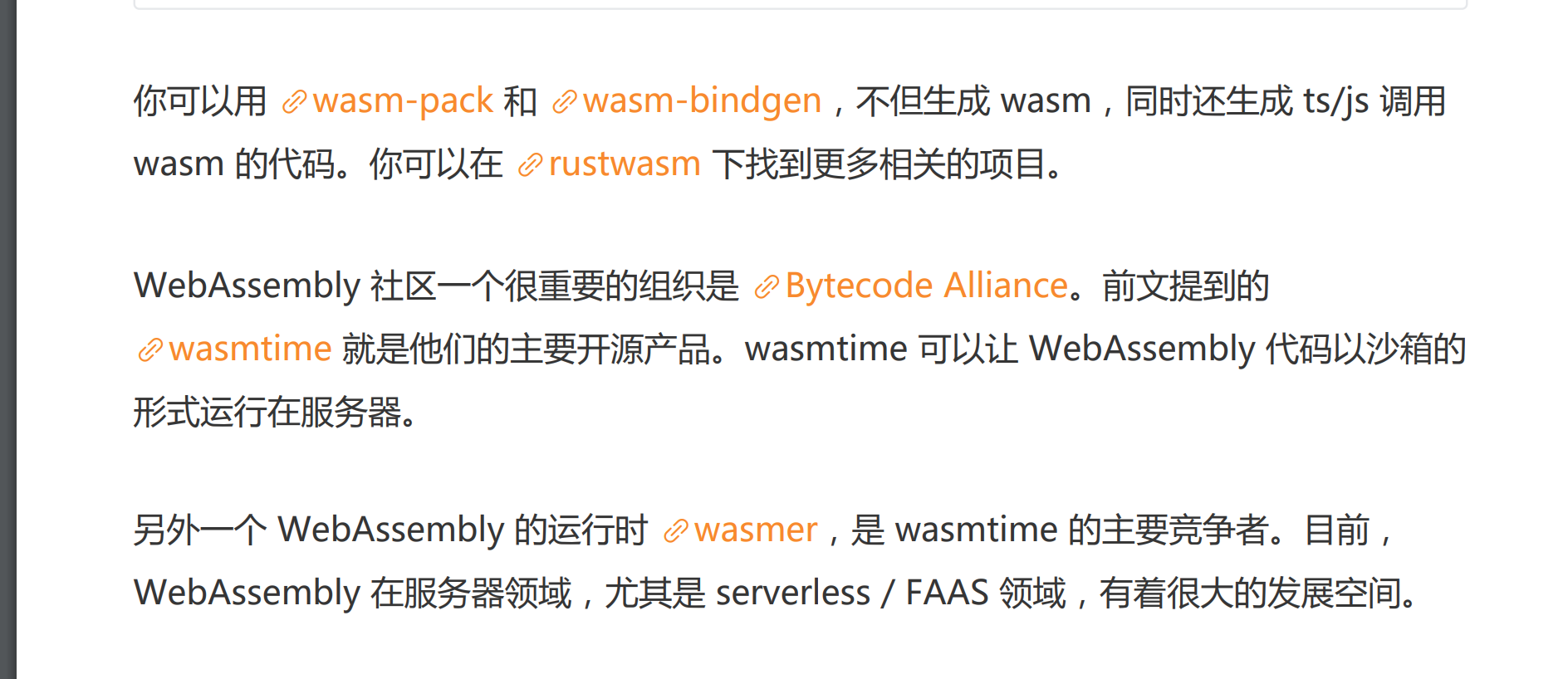
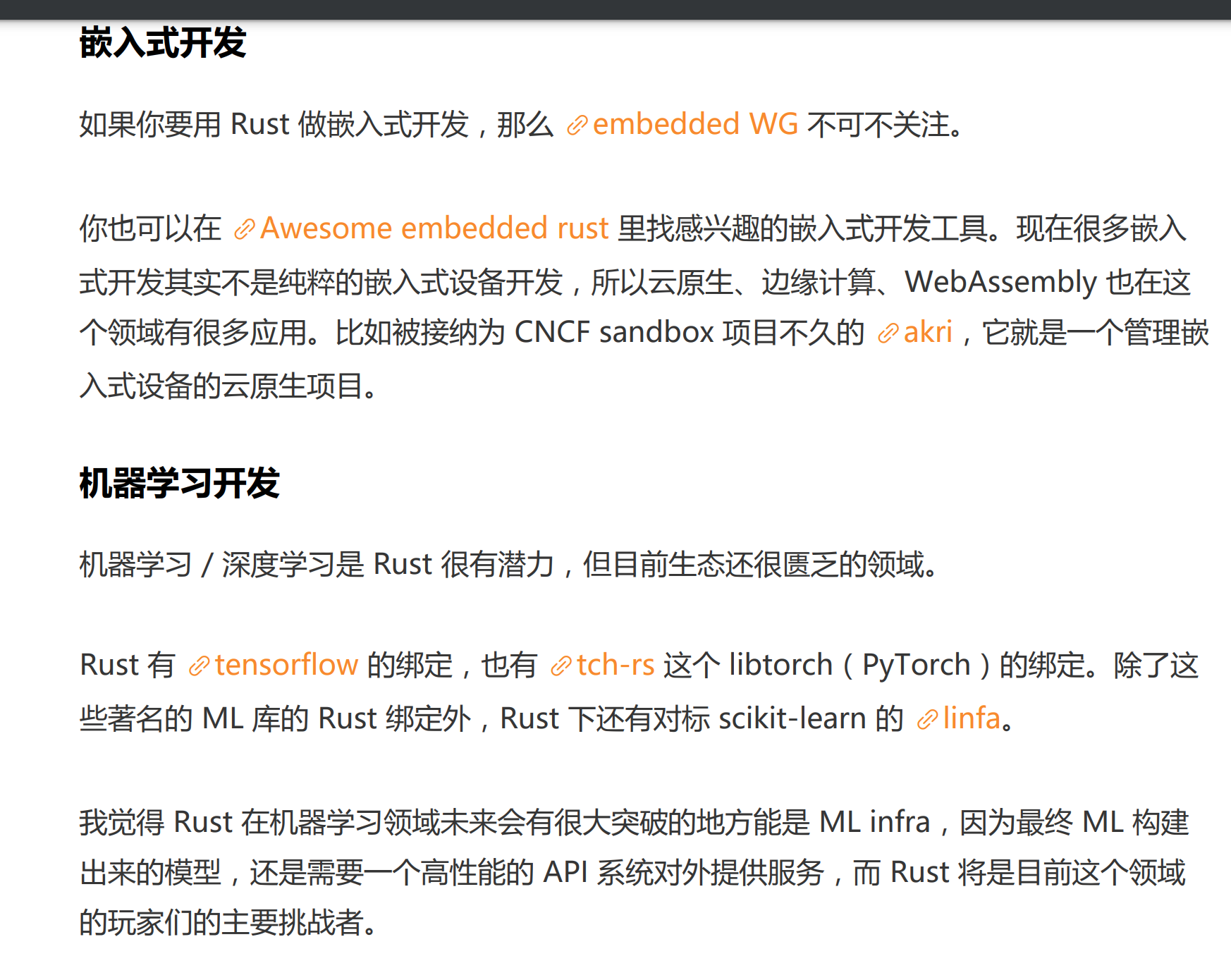
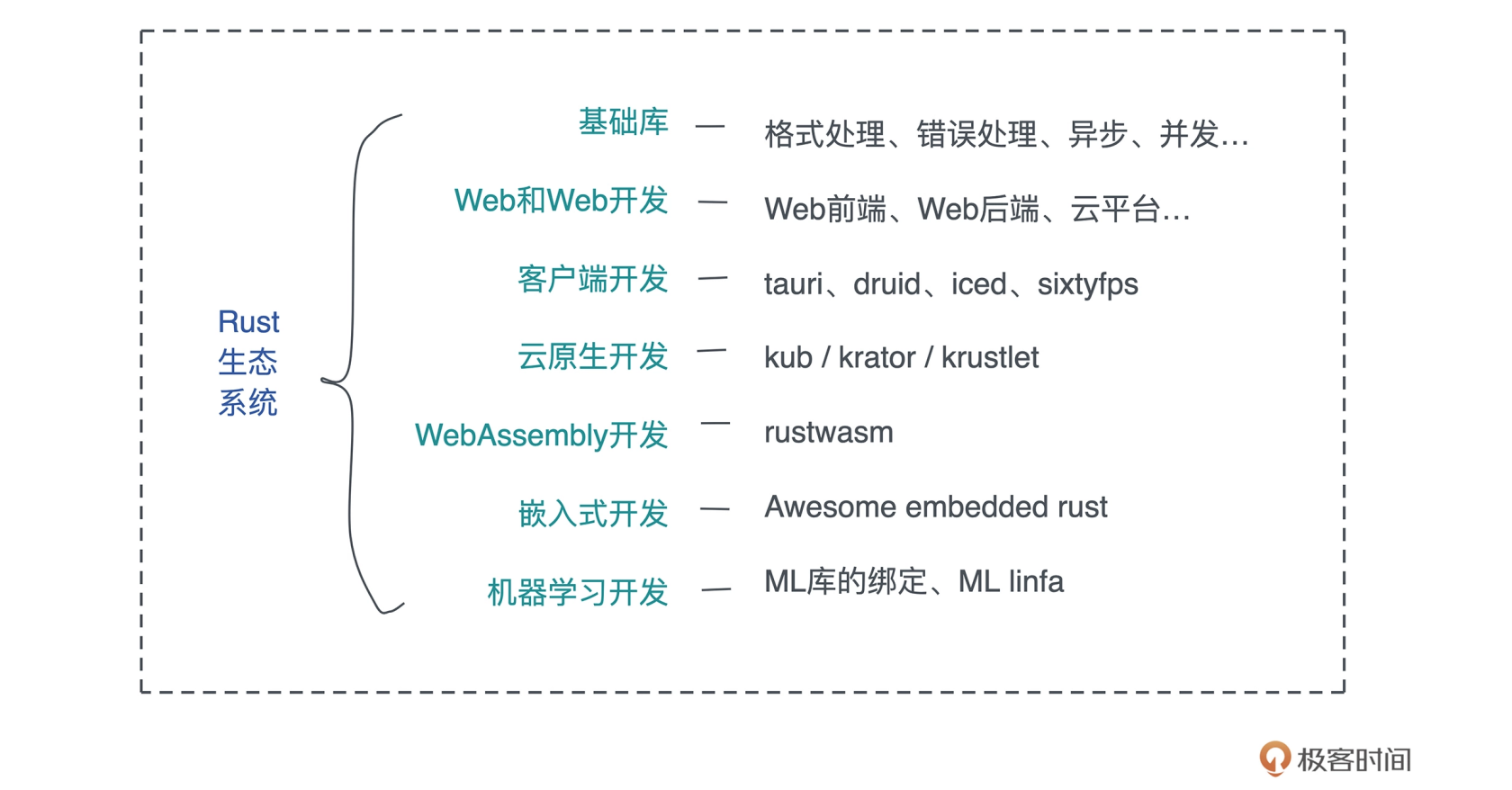
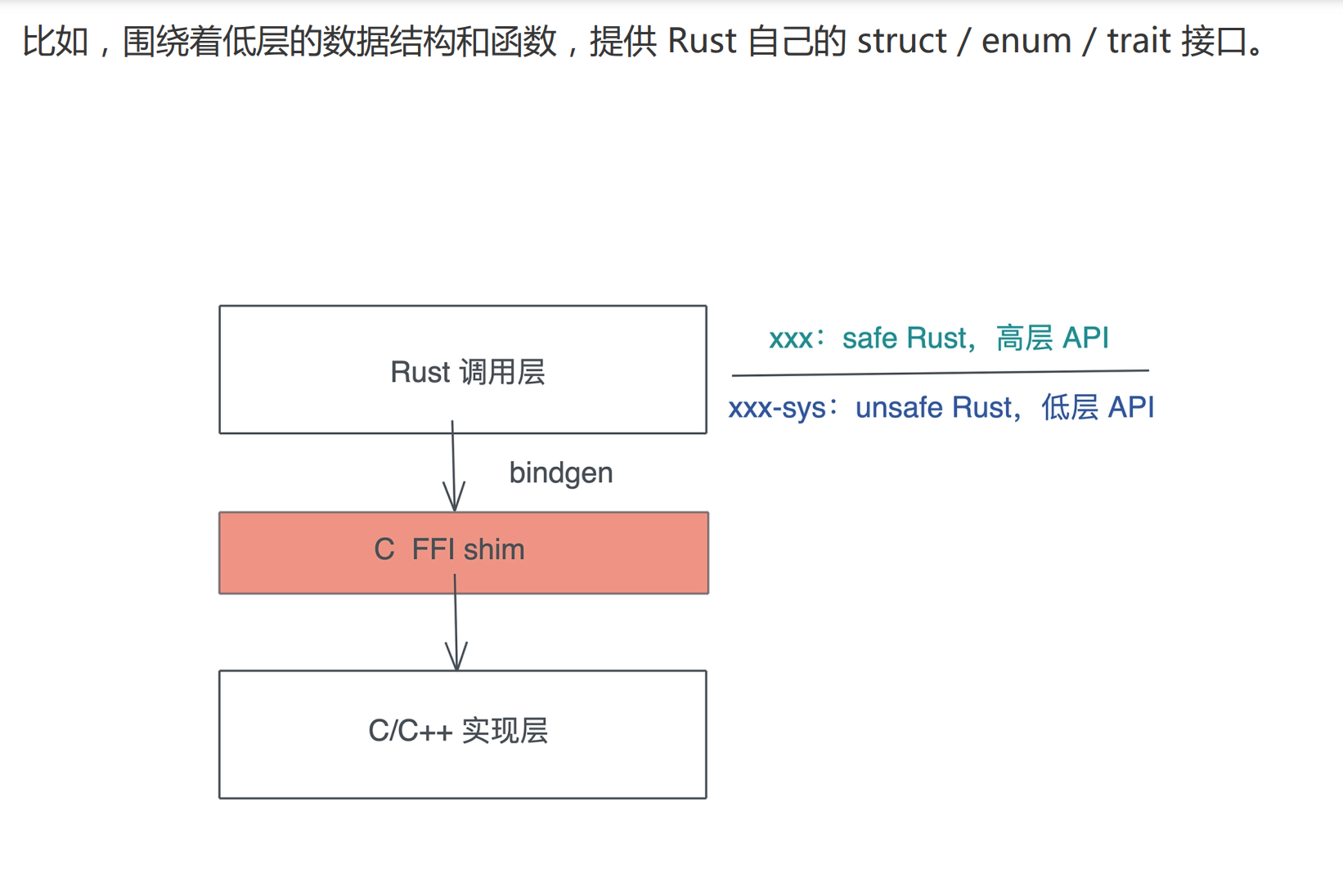
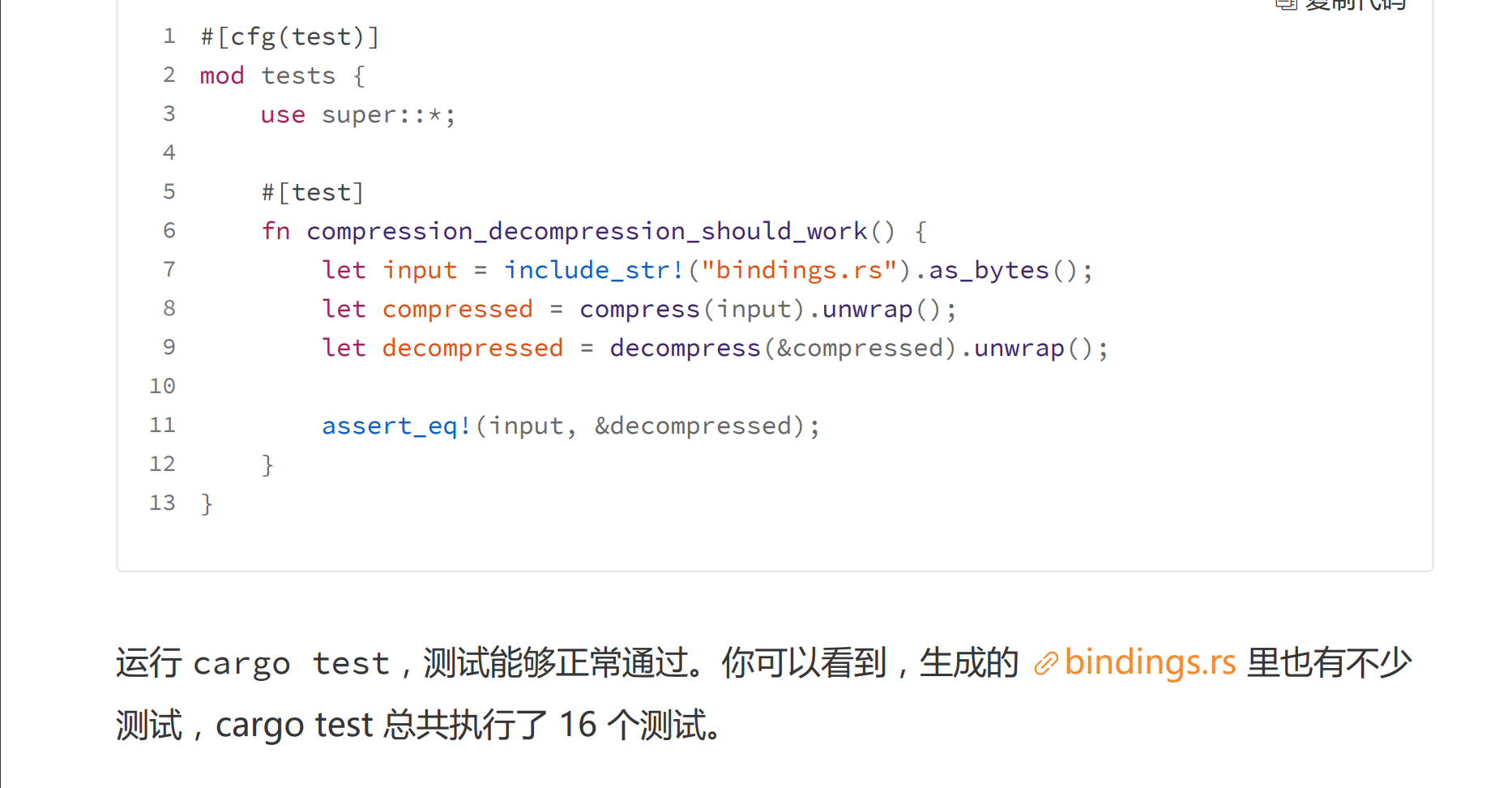
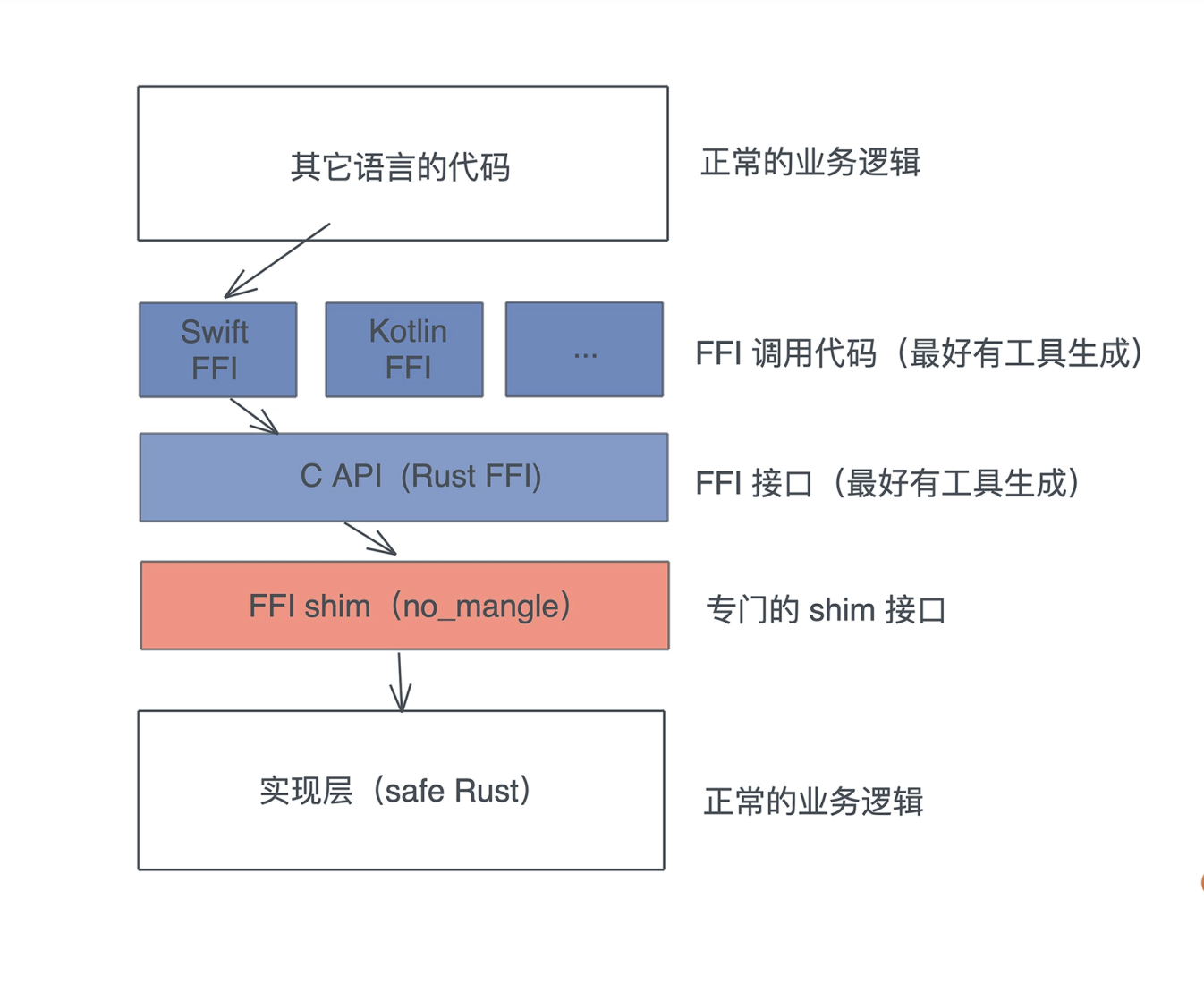
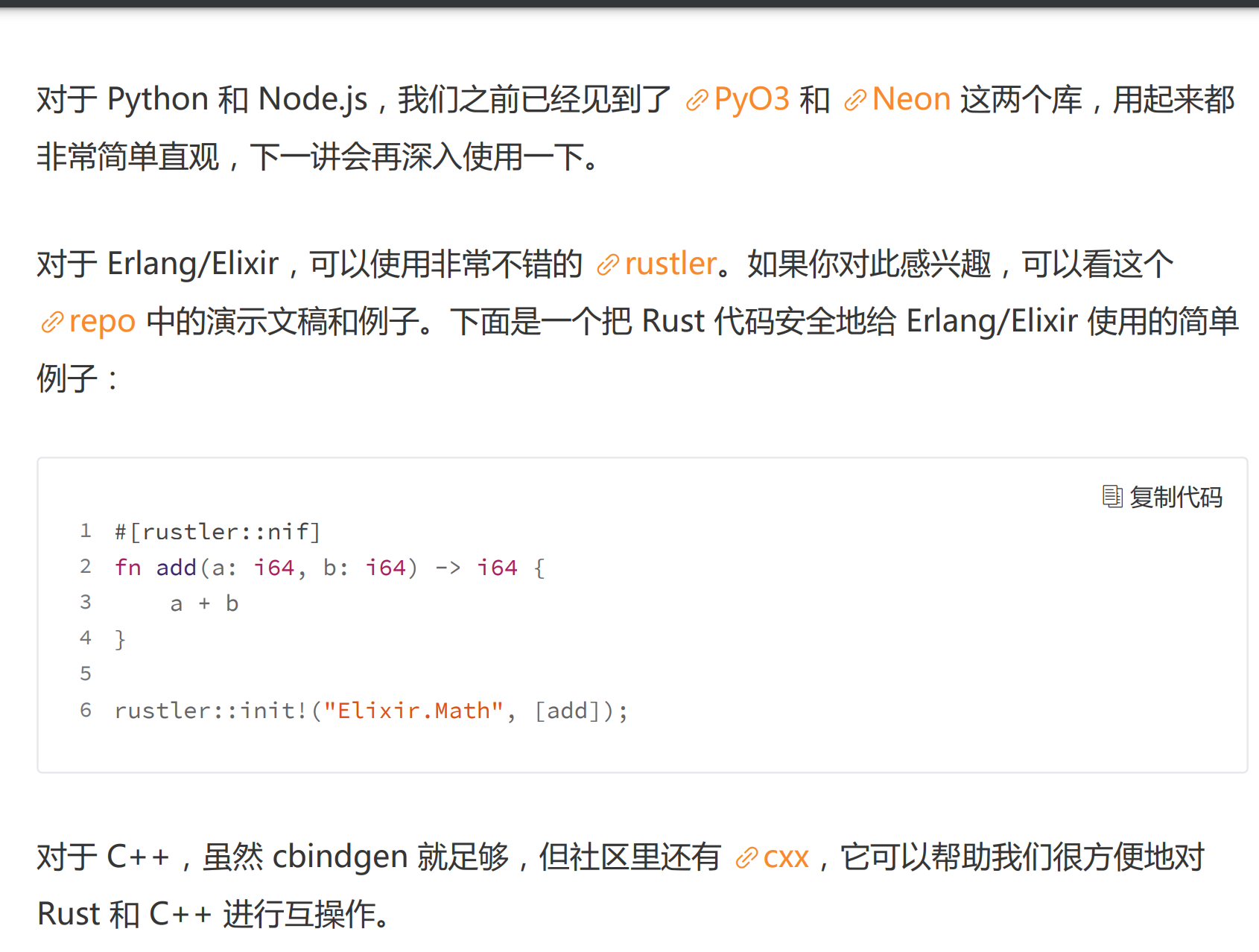
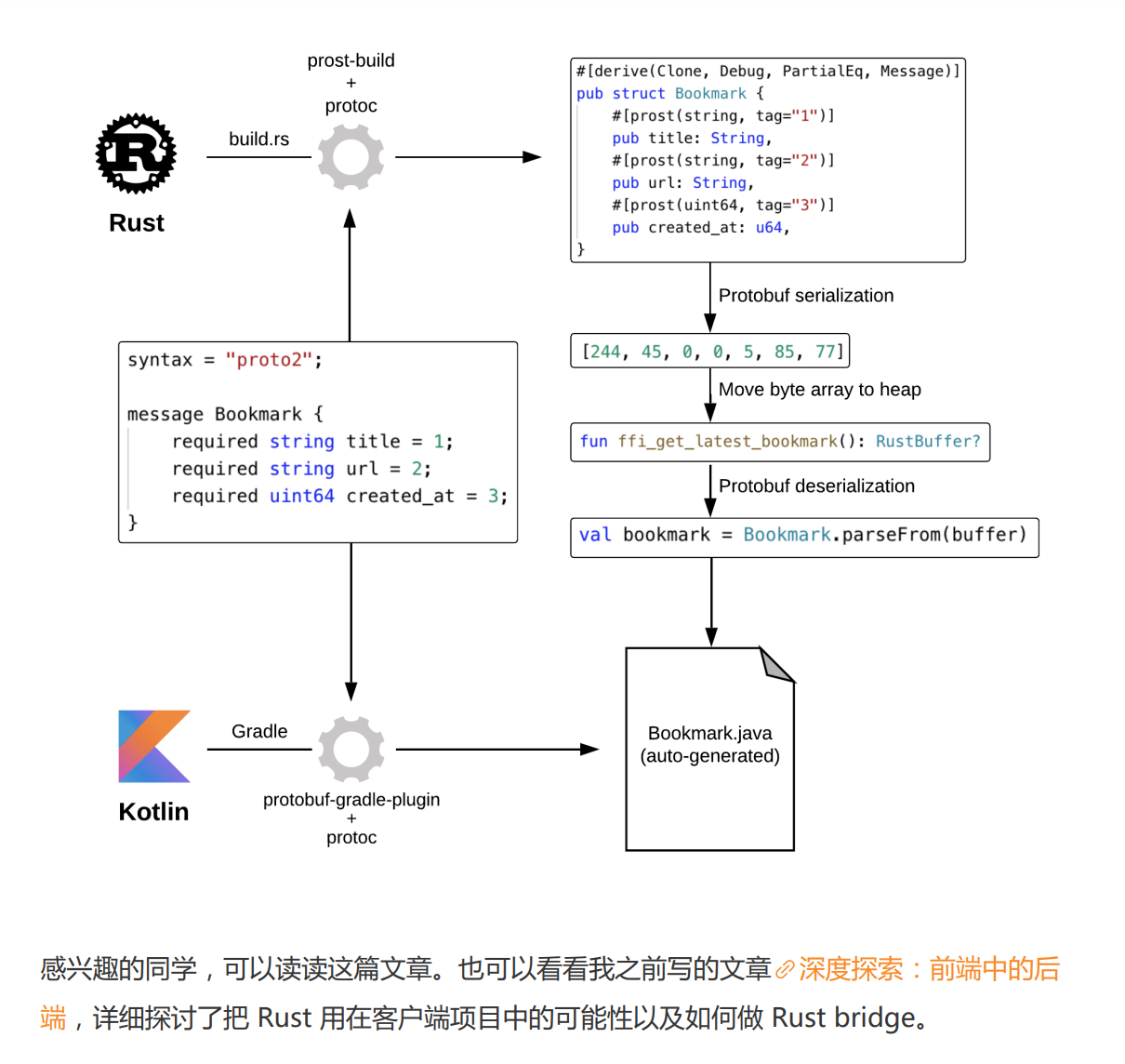
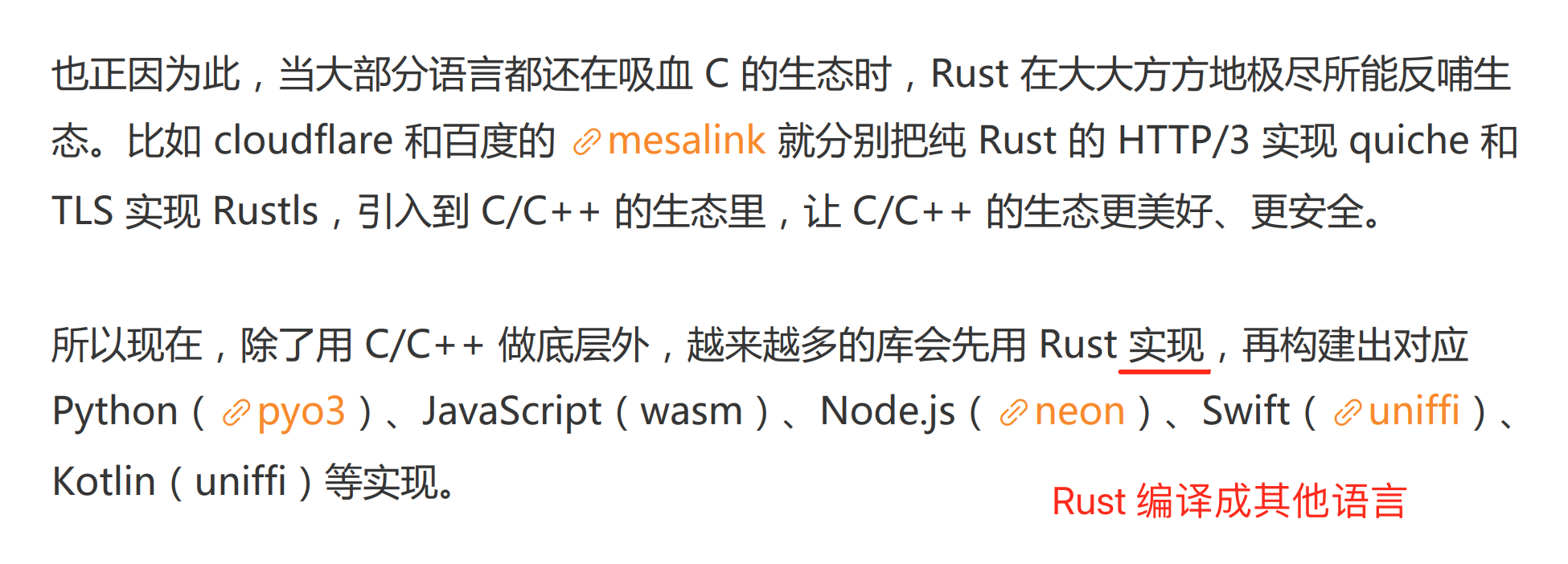
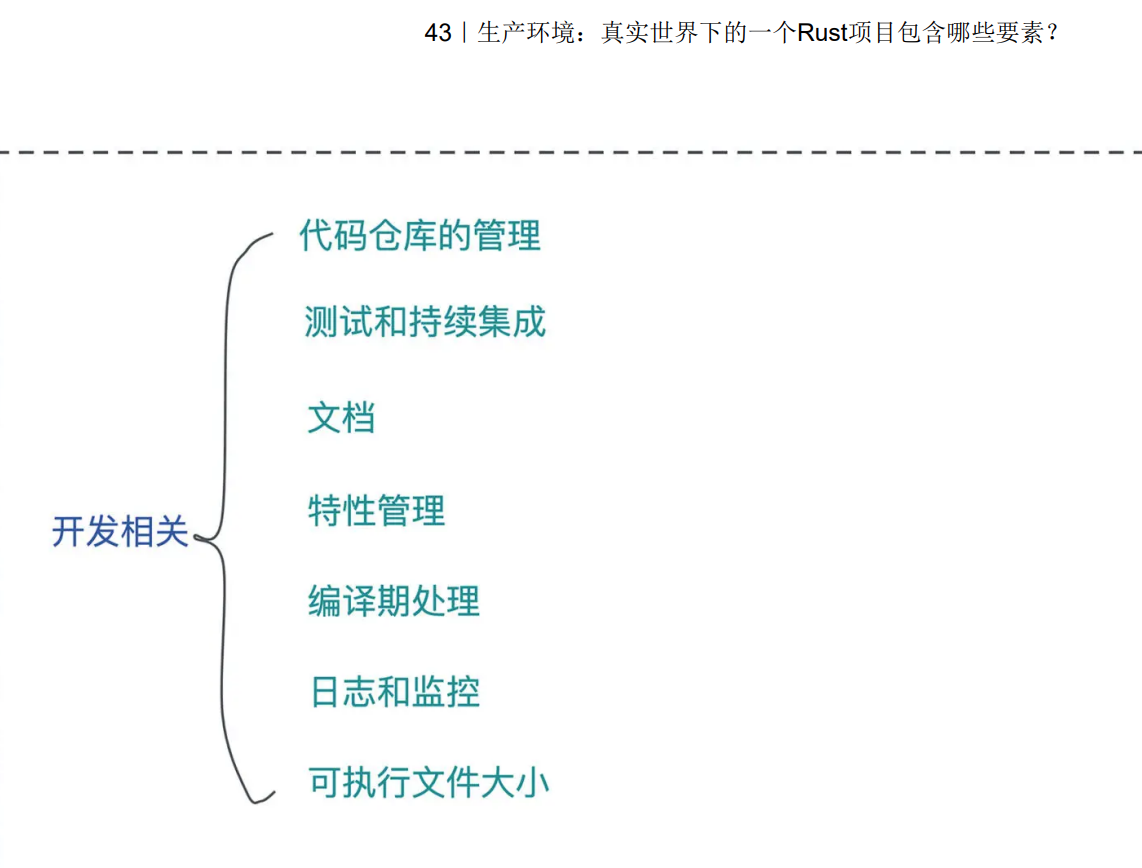
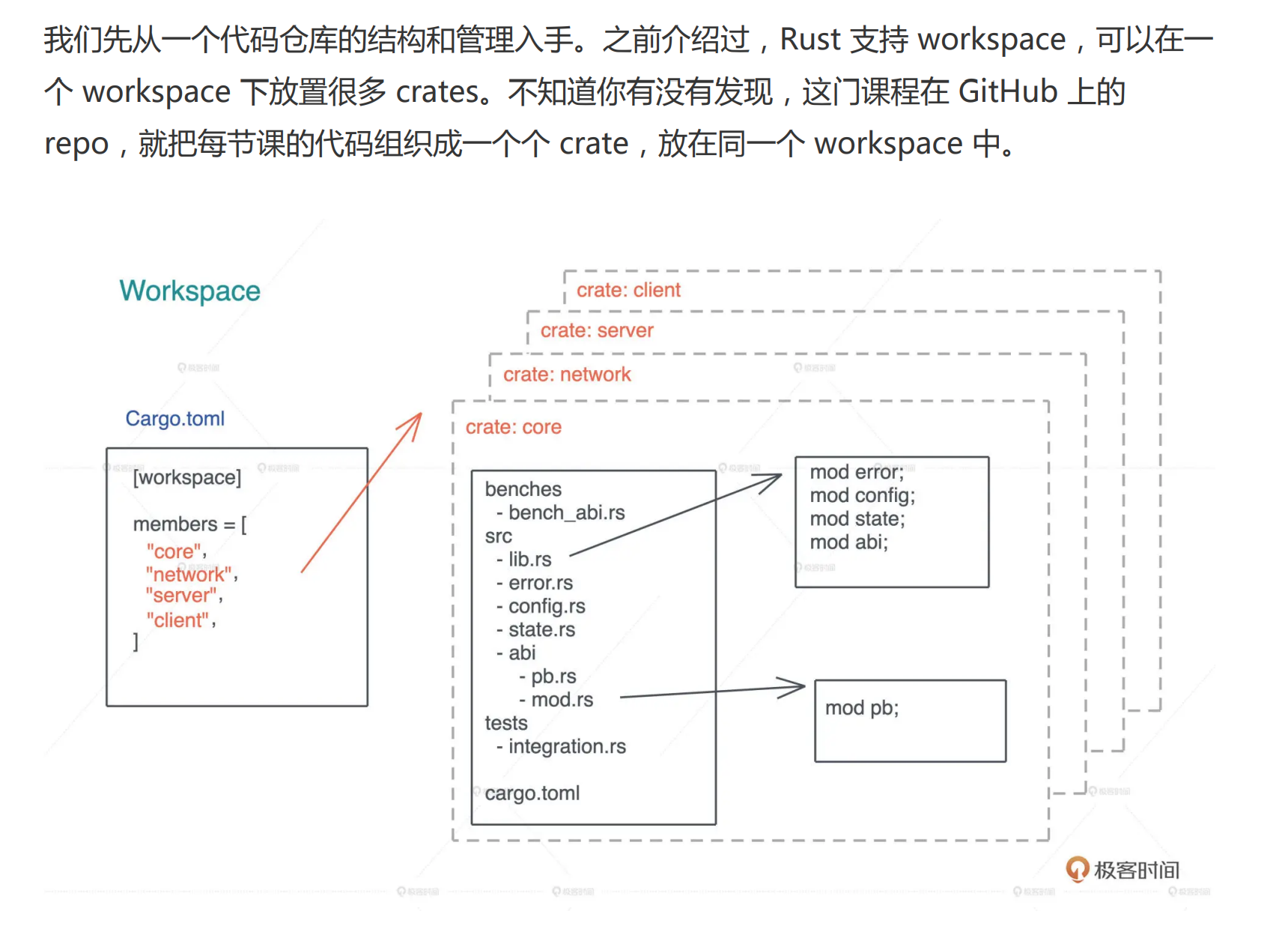
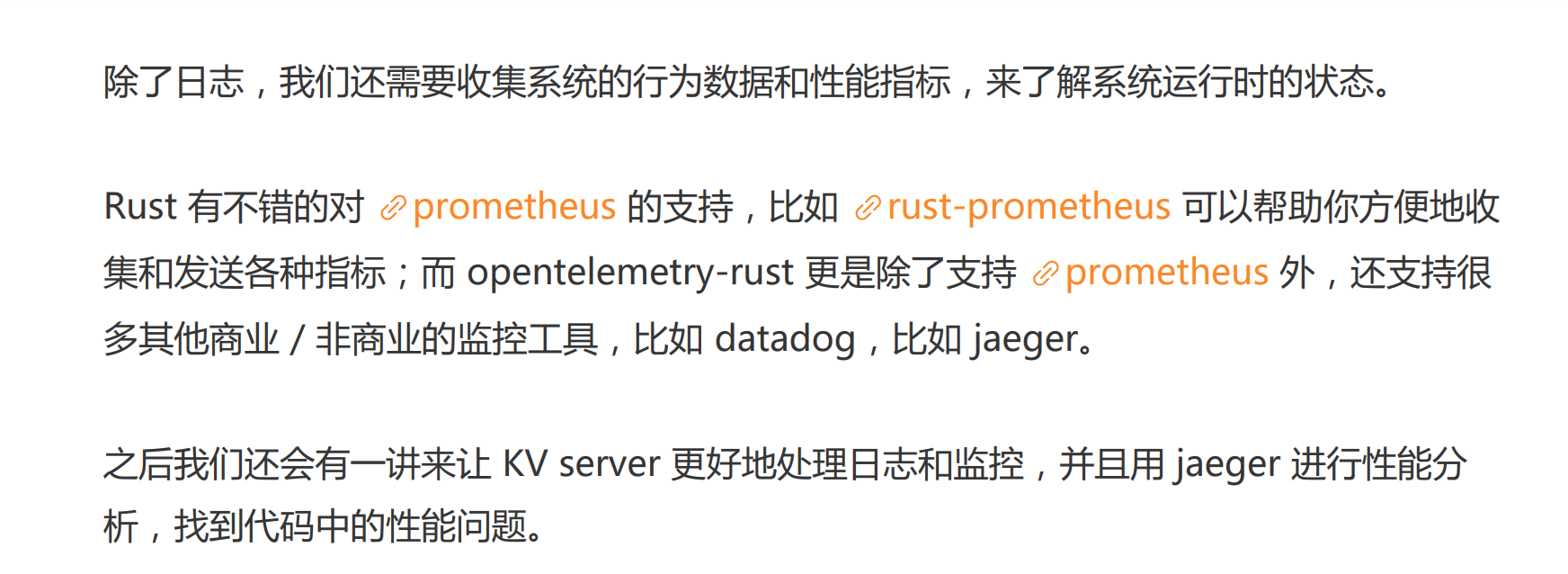
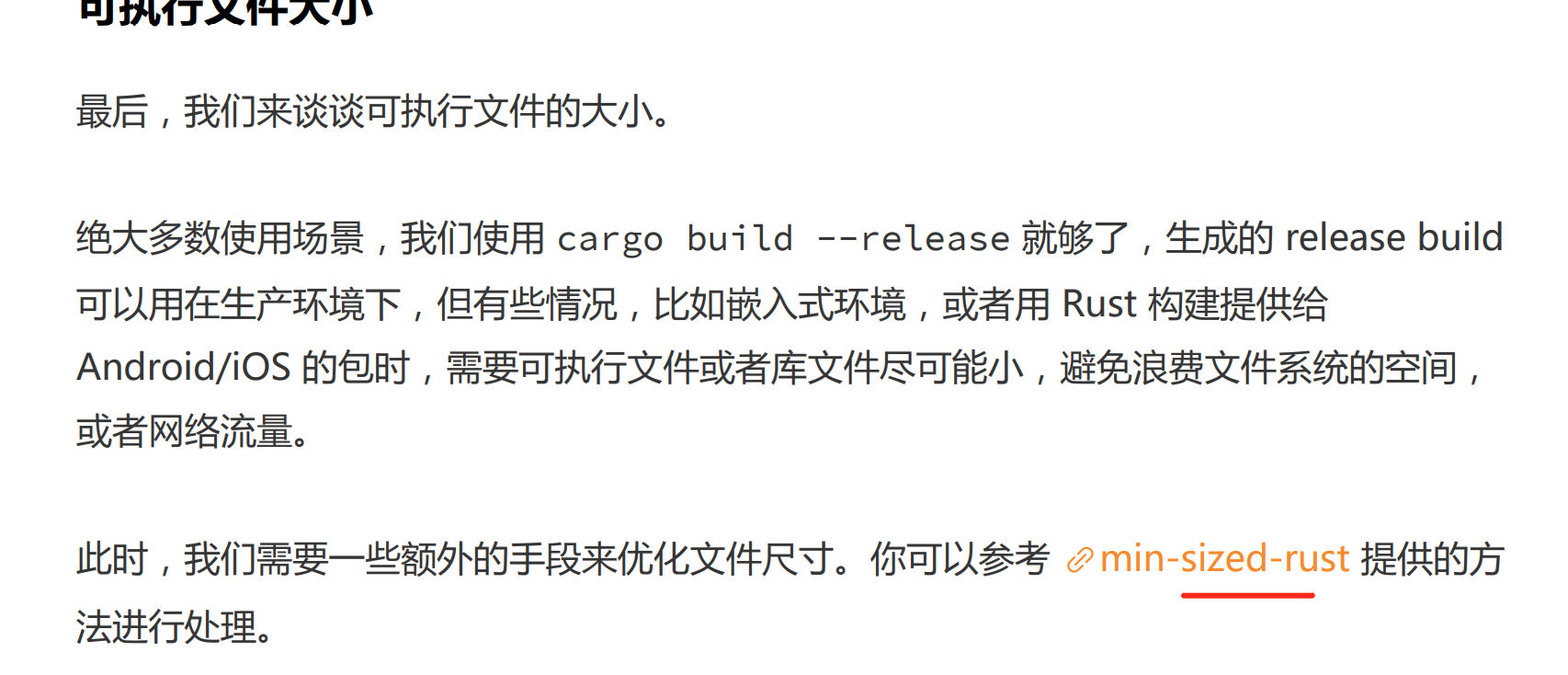
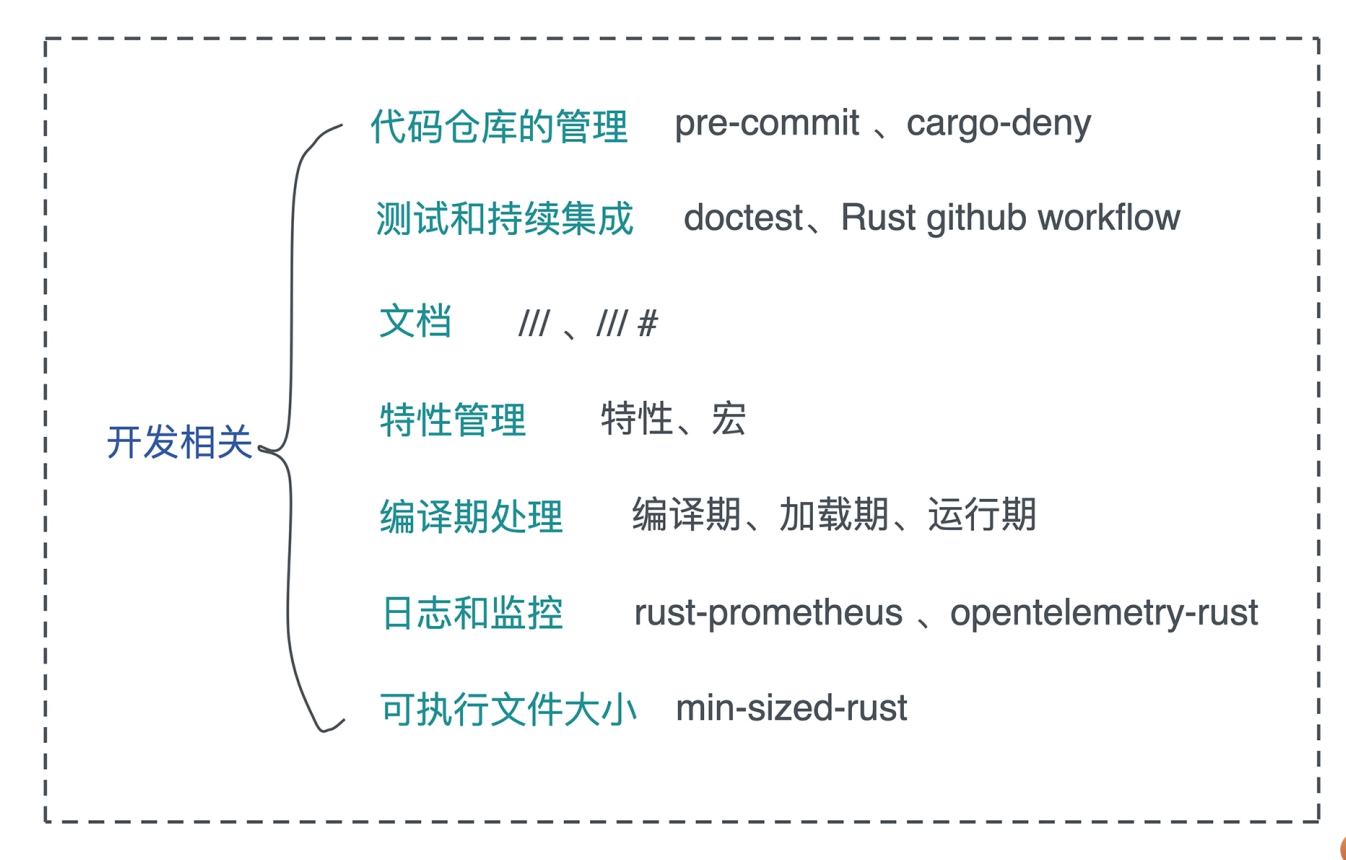
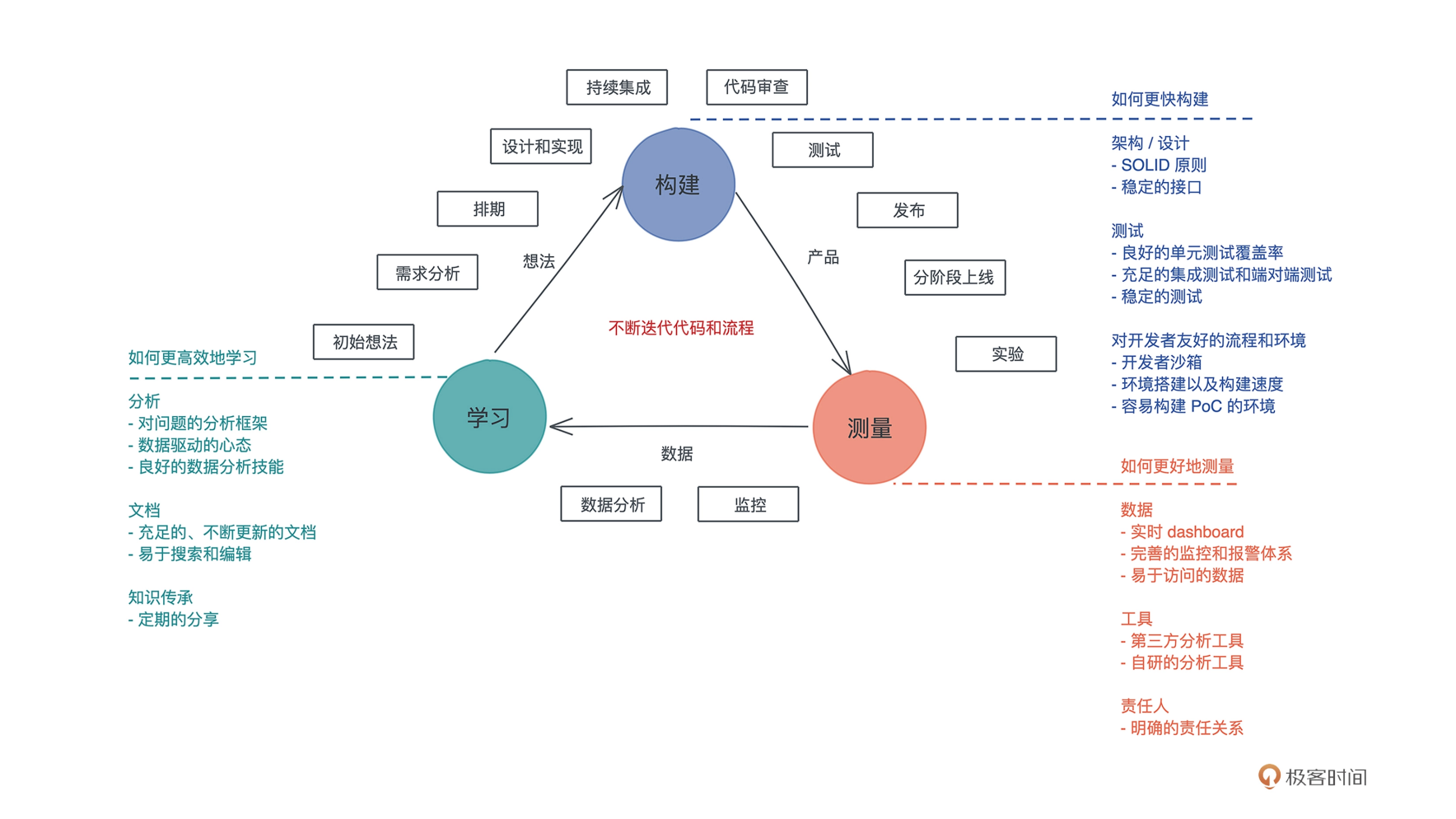
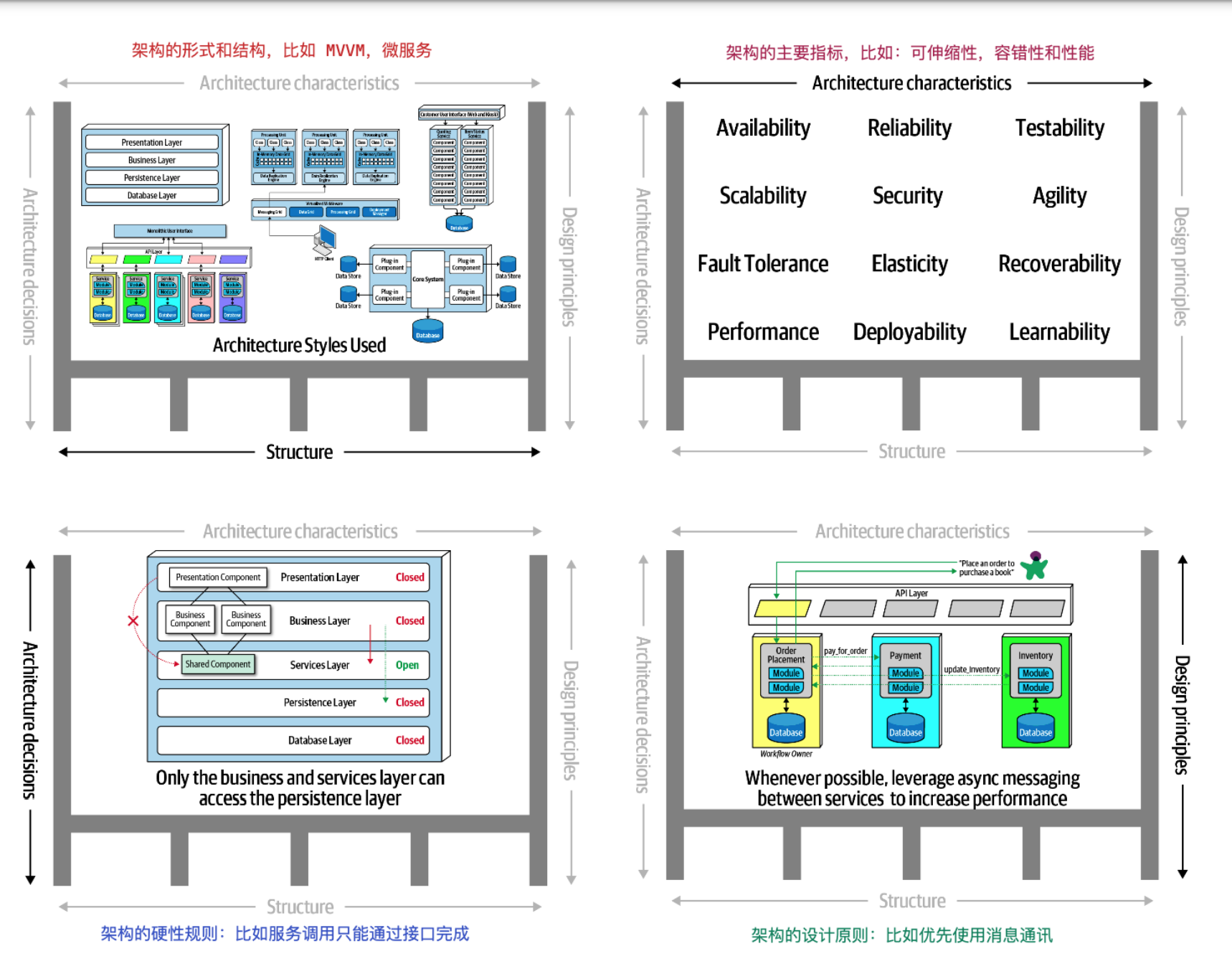
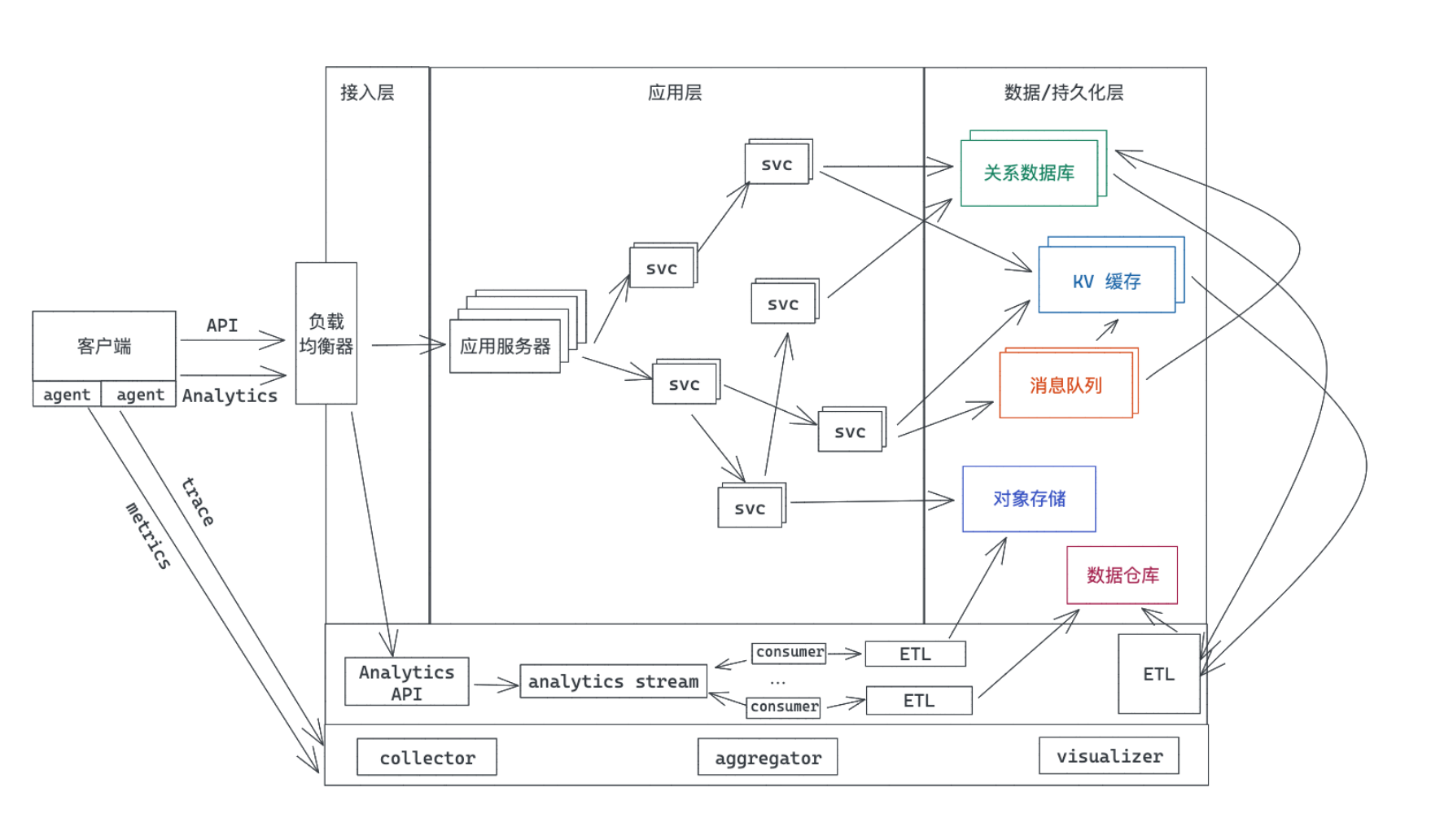
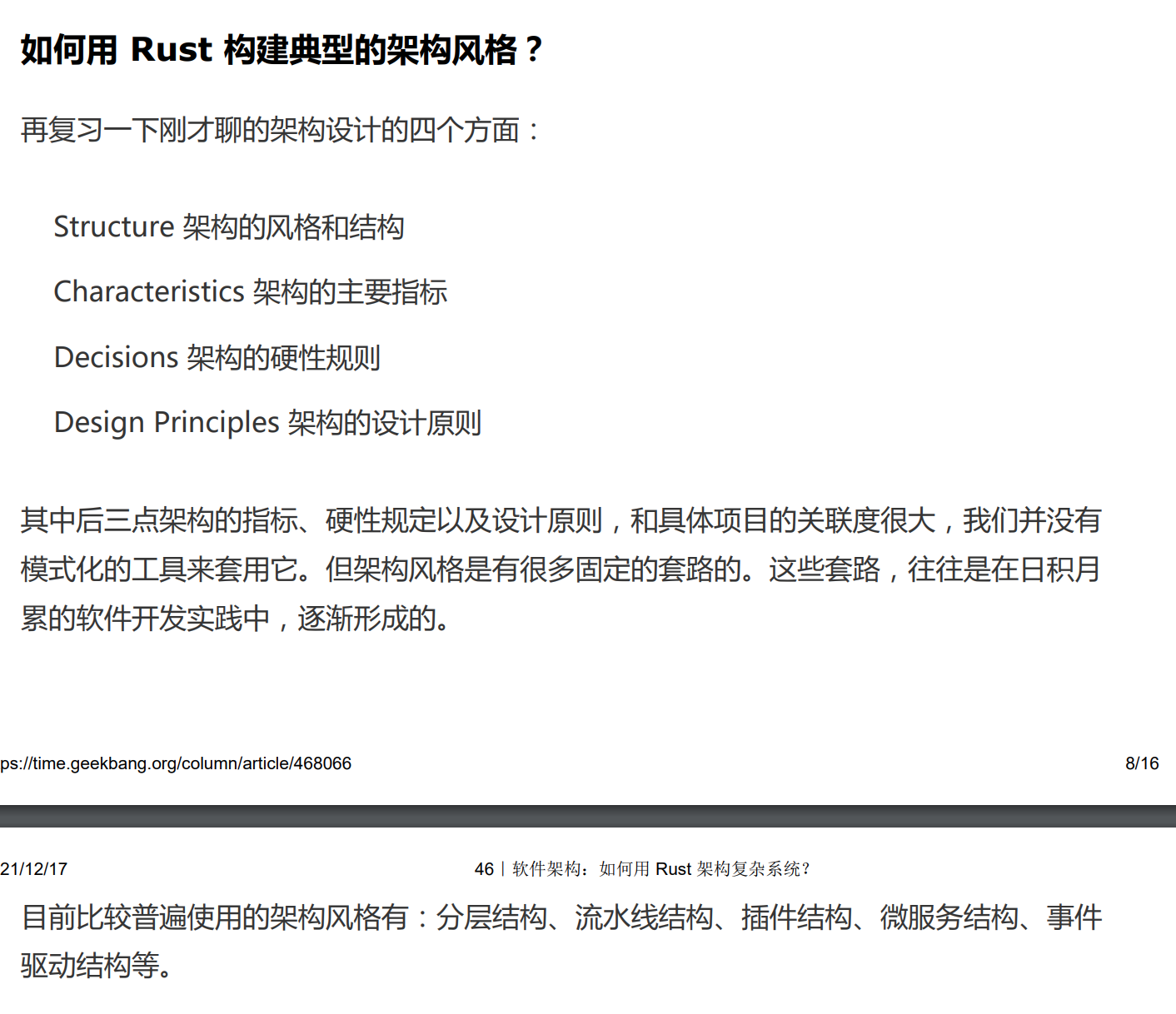
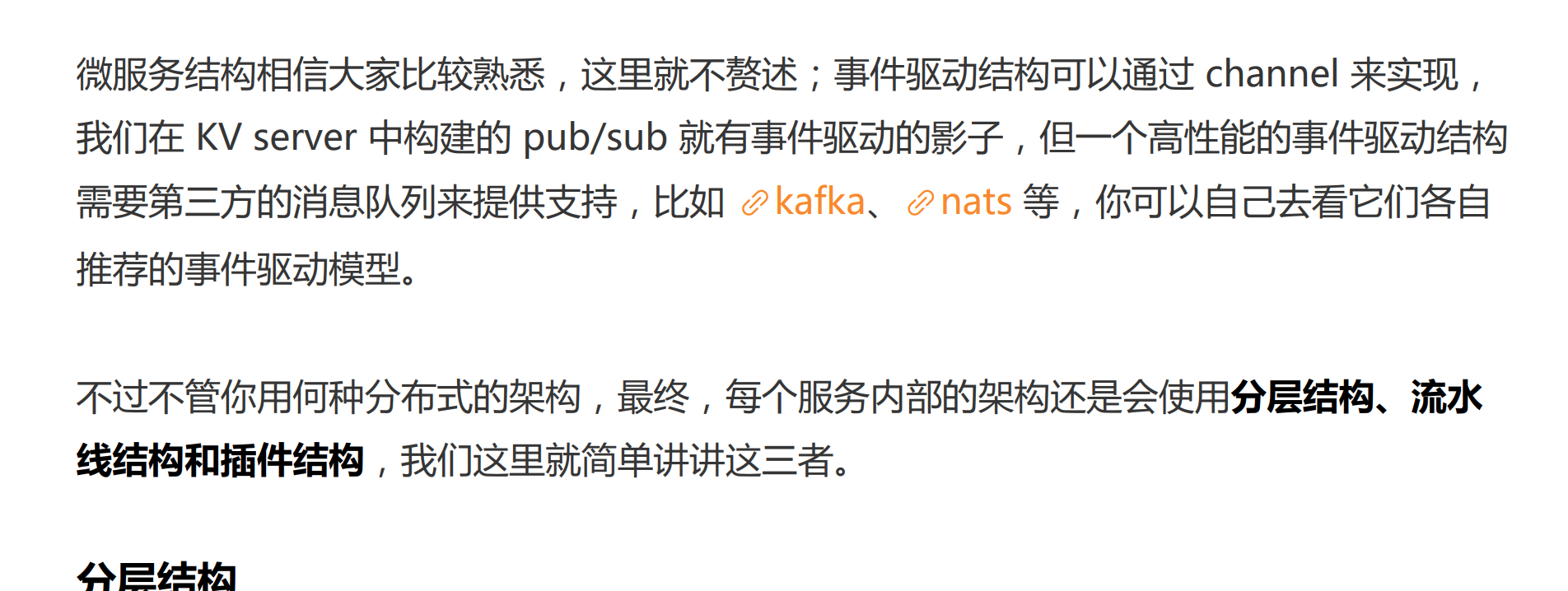
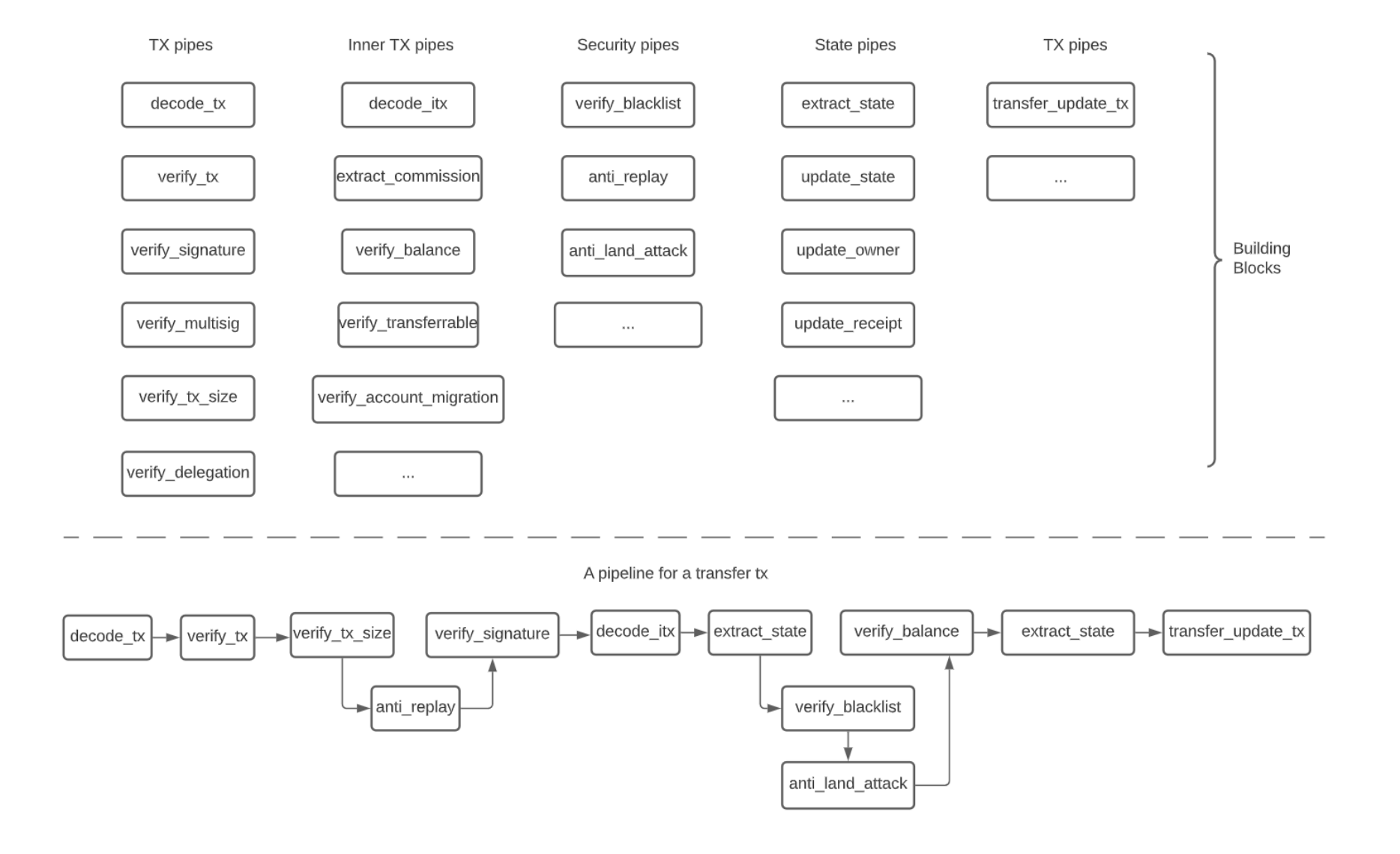
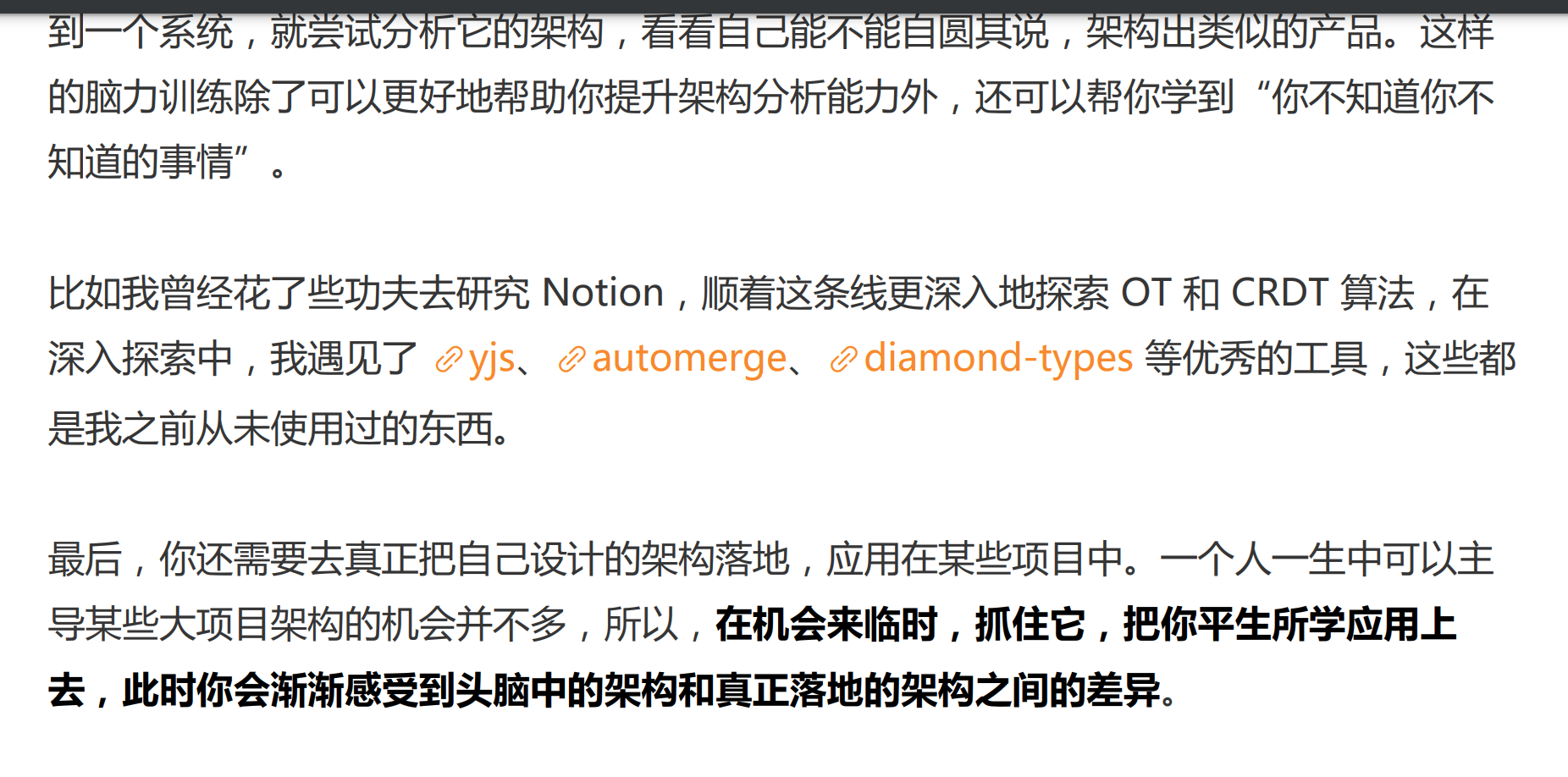
4、Rust学习路线和好的学习园地
张汉东的Rust课,重个基础,逐一展开, 最后实践是 一个web框架。
Rust第一课: 重整个项目和实战,创建了各方面综合项目。
Rust写的操作系统:https://github.com/redox-os/redox
氧化还原-os/氧化还原:https://gitlab.redox-os.org/redox-os/redox 的镜像 (github.com)
https://github.com/pop-os Pop!_OS 系统
https://github.com/orgs/pop-os/repositories?page=5 Pop!_OS rust 学习
Pop!_OS将被证明是Linux领域的苹果,因为Pop!_OS是为他们的硬件量身定制的,不像Ubuntu。
https://pop.system76.com/ Pop!_OS by System76
https://manjaro.org曼扎罗山
https://archlinux.org/Arch Linux
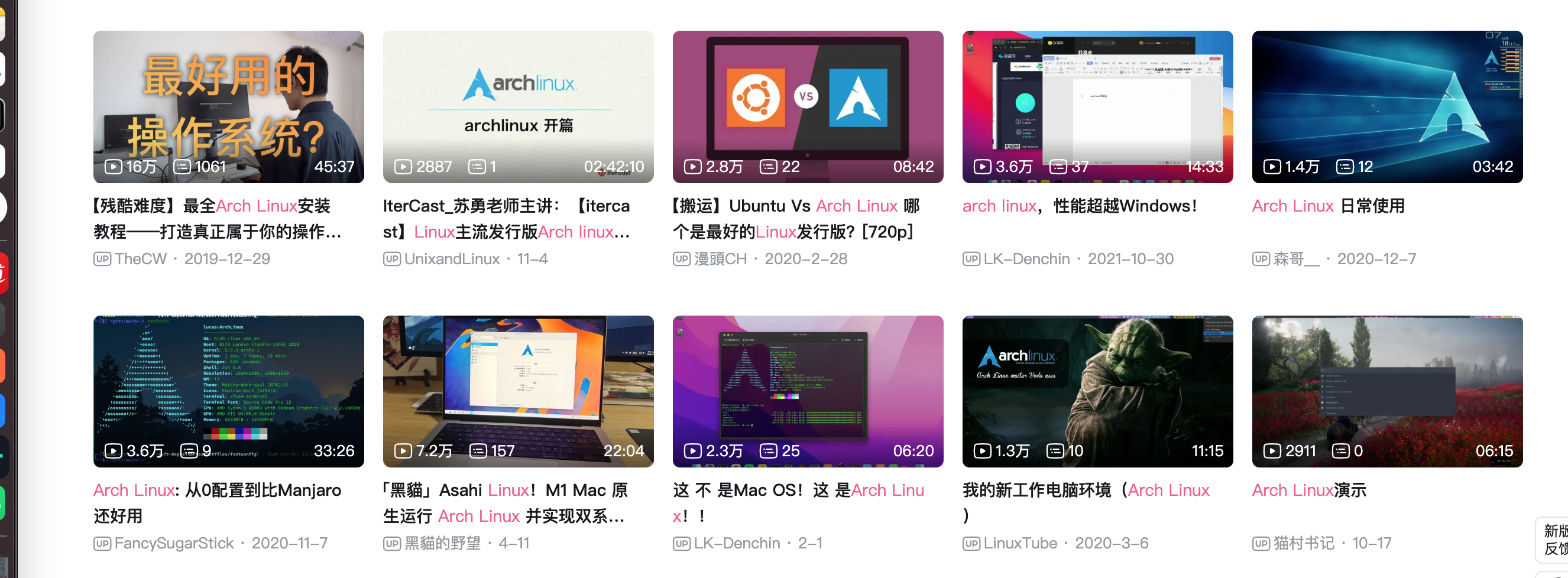
rust项目:
https://github.com/bilelmoussaoui/process-viewer 跨平台进程查看器
https://github.com/bilelmoussaoui/wallabag-api api客户端
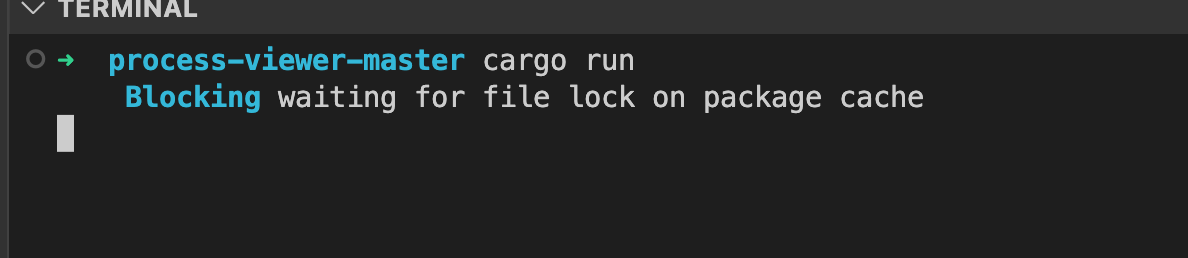
rm -rf ~/.cargo/.package-cache