文章目录
- 引言
- 第一章 RESTful API基础知识
- 1.1 什么是RESTful API
- 1.2 RESTful API的优势
- 第二章 Spring Boot基础知识
- 2.1 什么是Spring Boot
- 2.2 Spring Boot的主要特性
- 第三章 使用Spring Boot构建RESTful API
- 3.1 项目初始化
- 3.2 构建基础结构
- 3.3 定义实体类
- 3.4 创建Repository接口
- 3.5 实现Service类
- 3.6 创建Controller类
- 第四章 Spring Boot高级特性
- 4.1 Spring Boot Actuator
- 4.2 Spring Boot与消息队列
- 第五章 部署与监控
- 5.1 部署Spring Boot应用
- 5.2 使用Docker部署Spring Boot应用
- 5.3 监控Spring Boot应用
- 第六章 实践案例:构建一个简单的博客平台
- 6.1 项目结构
- 6.2 文章管理
- 结论
.
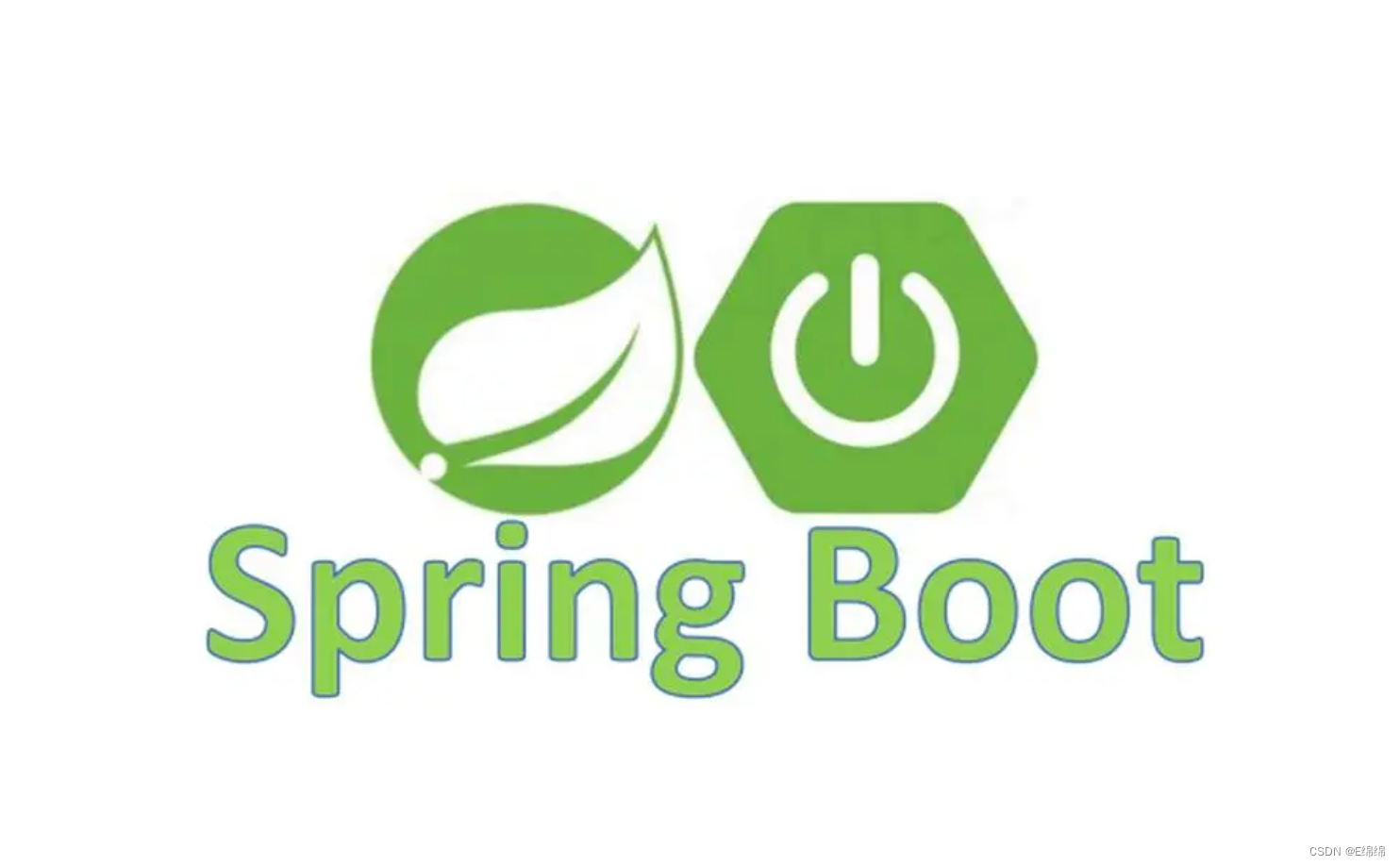
引言
RESTful API是Web服务开发中常用的一种架构风格,通过HTTP协议提供与资源交互的方式。Spring Boot作为一个流行的Java框架,通过简化配置和快速开发,成为构建RESTful API的理想选择。本文将深入探讨如何使用Spring Boot构建RESTful API,包括基础知识、核心功能、最佳实践和实际应用,并提供具体的代码示例和应用案例。
第一章 RESTful API基础知识
1.1 什么是RESTful API
RESTful API是一种基于REST(Representational State Transfer)架构风格的Web服务接口,通过使用HTTP协议进行资源的创建、读取、更新和删除(CRUD)操作。RESTful API具有以下特点:
- 资源(Resource):API中的每个实体都是一个资源,通过URI(统一资源标识符)来标识。
- HTTP动词:使用HTTP动词(GET、POST、PUT、DELETE)进行操作,表示对资源的不同操作。
- 状态表示:资源的状态通过表述(Representation)来传递,可以是JSON、XML等格式。
- 无状态:每个请求都是独立的,不依赖于之前的请求状态。
1.2 RESTful API的优势
- 简单易用:通过HTTP协议和标准化的动词操作,简单易用。
- 灵活性:支持多种数据格式和通信方式,灵活性高。
- 可扩展性:支持分布式系统的扩展和集成,适合大规模应用。
第二章 Spring Boot基础知识
2.1 什么是Spring Boot
Spring Boot是一个基于Spring框架的开源项目,提供了一种快速构建生产级Spring应用的方法。通过简化配置、提供自动化配置(Auto Configuration)和内嵌服务器等特性,Spring Boot使得开发者能够更加专注于业务逻辑,而不需要花费大量时间在配置和部署上。
2.2 Spring Boot的主要特性
- 自动化配置:通过自动化配置减少了大量的手动配置工作。
- 内嵌服务器:提供内嵌的Tomcat、Jetty和Undertow服务器,方便开发者在开发和测试阶段快速启动和运行应用。
- 独立运行:应用可以打包成一个可执行的JAR文件,包含所有依赖项,可以独立运行,不需要外部的应用服务器。
- 生产级功能:提供了监控、度量、健康检查等生产级功能,方便开发者管理和监控应用的运行状态。
- 多样化的配置:支持多种配置方式,包括YAML、Properties文件和环境变量,满足不同开发和部署环境的需求。
第三章 使用Spring Boot构建RESTful API
3.1 项目初始化
使用Spring Initializr生成一个Spring Boot项目,并添加所需依赖。
<!-- 示例:通过Spring Initializr生成的pom.xml配置文件 -->
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>rest-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
<packaging>jar</packaging>
<name>rest-api</name>
<description>Demo project for Spring Boot RESTful API</description>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.4</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
3.2 构建基础结构
在项目中创建必要的包结构,包括controller、service、repository和model。
rest-api
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com
│ │ │ └── example
│ │ │ └── restapi
│ │ │ ├── RestApiApplication.java
│ │ │ ├── controller
│ │ │ │ └── UserController.java
│ │ │ ├── service
│ │ │ │ └── UserService.java
│ │ │ ├── repository
│ │ │ │ └── UserRepository.java
│ │ │ └── model
│ │ │ └── User.java
│ │ └── resources
│ │ ├── application.yml
│ │ └── data.sql
└── pom.xml
3.3 定义实体类
定义一个简单的用户实体类,并配置JPA注解。
// 示例:用户实体类
package com.example.restapi.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String name;
private String email;
// Getters and Setters
}
3.4 创建Repository接口
创建一个JPA Repository接口,用于数据访问操作。
// 示例:用户Repository接口
package com.example.restapi.repository;
import com.example.restapi.model.User;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
}
3.5 实现Service类
创建一个Service类,封装业务逻辑和数据访问操作。
// 示例:用户服务类
package com.example.restapi.service;
import com.example.restapi.model.User;
import com.example.restapi.repository.UserRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> findAll() {
return userRepository.findAll();
}
public Optional<User> findById(Long id) {
return userRepository.findById(id);
}
public User save(User user) {
return userRepository.save(user);
}
public void deleteById(Long id) {
userRepository.deleteById(id);
}
}
3.6 创建Controller类
创建一个Controller类,定义RESTful API的端点,并通过Service类处理请求。
// 示例:用户控制器
package com.example.restapi.controller;
import com.example.restapi.model.User;
import com.example.restapi.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping
public List<User> getAllUsers() {
return userService.findAll();
}
@GetMapping("/{id}")
public Optional<User> getUserById(@PathVariable Long id) {
return userService.findById(id);
}
@PostMapping
public User createUser(@RequestBody User user) {
return userService.save(user);
}
@PutMapping("/{id}")
public User updateUser(@PathVariable Long id, @RequestBody User userDetails) {
User user = userService.findById(id).orElseThrow(() -> new ResourceNotFoundException("User not found with id " + id));
user.setName(userDetails.getName());
user.setEmail(userDetails.getEmail());
return userService.save(user);
}
@DeleteMapping("/{id}")
public void deleteUser(@PathVariable Long id) {
userService.deleteById(id);
}
}
第四章 Spring Boot高级特性
4.1 Spring Boot Actuator
Spring Boot Actuator提供了一组用于监控和管理Spring Boot应用的功能,通过一组内置的端点,开发者可以方便地获取应用的运行状态、健康检查、度量指标等信息。
# 示例:启用Spring Boot Actuator
management:
endpoints:
web:
exposure:
include: "*"
endpoint:
health:
show-details: always
// 示例:自定义健康检查
package com.example.restapi.actuator;
import org.springframework.boot.actuate.health.Health;
import org.springframework.boot.actuate.health.HealthIndicator;
import org.springframework.stereotype.Component;
@Component
public class CustomHealthIndicator implements HealthIndicator {
@Override
public Health health() {
// 自定义健康检查逻辑
return Health.up().withDetail("status", "All systems operational").build();
}
}
4.2 Spring Boot与消息队列
Spring Boot与消息队列(如RabbitMQ、Kafka)结合,可以实现异步处理和事件驱动的架构。
<!-- 示例:集成RabbitMQ的pom.xml配置文件 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
// 示例:RabbitMQ配置类
package com.example.restapi.config;
import org.springframework.amqp.core.Queue;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class RabbitMQConfig {
@Bean
public Queue myQueue() {
return new Queue("myQueue", false);
}
}
// 示例:消息发送服务
package com.example.restapi.service;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class MessageSender {
@Autowired
private RabbitTemplate rabbitTemplate;
public void sendMessage(String message) {
rabbitTemplate.convertAndSend("myQueue", message);
}
}
// 示例:消息接收服务
package com.example.restapi.service;
import org.springframework.amqp.rabbit.annotation.RabbitListener;
import org.springframework.stereotype.Service;
@Service
public class MessageReceiver {
@RabbitListener(queues = "myQueue")
public void receiveMessage(String message) {
System.out.println("Received message: " + message);
}
}
第五章 部署与监控
5.1 部署Spring Boot应用
Spring Boot应用可以通过多种方式进行部署,包括打包成JAR文件、Docker容器、Kubernetes集群等。
# 打包Spring Boot应用
mvn clean package
# 运行Spring Boot应用
java -jar target/rest-api-0.0.1-SNAPSHOT.jar
5.2 使用Docker部署Spring Boot应用
Docker是一个开源的容器化平台,可以帮助开发者将Spring Boot应用打包成容器镜像,并在任何环境中运行。
# 示例:Dockerfile文件
FROM openjdk:11-jre-slim
VOLUME /tmp
COPY target/rest-api-0.0.1-SNAPSHOT.jar app.jar
ENTRYPOINT ["java","-jar","/app.jar"]
# 构建Docker镜像
docker build -t spring-boot-rest-api .
# 运行Docker容器
docker run -p 8080:8080 spring-boot-rest-api
5.3 监控Spring Boot应用
Spring Boot Actuator提供了丰富的监控功能,通过Prometheus和Grafana,可以实现对Spring Boot应用的监控和可视化。
<!-- 示例:集成Prometheus的pom.xml配置文件 -->
<dependency>
<groupId>io.micrometer</groupId>
<artifactId>micrometer-registry-prometheus</artifactId>
</dependency>
# 示例:Prometheus配置文件
management:
endpoints:
web:
exposure:
include: "*"
endpoint:
prometheus:
enabled: true
第六章 实践案例:构建一个简单的博客平台
6.1 项目结构
本节将通过一个简单的博客平台案例,展示Spring Boot在实际应用中的使用,包括文章管理、用户管理和评论管理等功能。
blog-platform
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com
│ │ │ └── example
│ │ │ └── blog
│ │ │ ├── BlogApplication.java
│ │ │ ├── controller
│ │ │ │ ├── ArticleController.java
│ │ │ │ ├── UserController.java
│ │ │ │ └── CommentController.java
│ │ │ ├── service
│ │ │ │ ├── ArticleService.java
│ │ │ │ ├── UserService.java
│ │ │ │ └── CommentService.java
│ │ │ ├── repository
│ │ │ │ ├── ArticleRepository.java
│ │ │ │ ├── UserRepository.java
│ │ │ │ └── CommentRepository.java
│ │ │ └── model
│ │ │ ├── Article.java
│ │ │ ├── User.java
│ │ │ └── Comment.java
│ │ └── resources
│ │ ├── application.yml
│ │ └── data.sql
└── pom.xml
6.2 文章管理
文章管理包括文章的创建、更新、删除和查询。
// 示例:文章实体类
package com.example.blog.model;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
@Entity
public class Article {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String title;
private String content;
// Getters and Setters
}
// 示例:文章Repository接口
package com.example.blog.repository;
import com.example.blog.model.Article;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface ArticleRepository extends JpaRepository<Article, Long> {
}
// 示例:文章服务类
package com.example.blog.service;
import com.example.blog.model.Article;
import com.example.blog.repository.ArticleRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class ArticleService {
@Autowired
private ArticleRepository articleRepository;
public List<Article> findAll() {
return articleRepository.findAll();
}
public Optional<Article> findById(Long id) {
return articleRepository.findById(id);
}
public Article save(Article article) {
return articleRepository.save(article);
}
public void deleteById(Long id) {
articleRepository.deleteById(id);
}
}
// 示例:文章控制器
package com.example.blog.controller;
import com.example.blog.model.Article;
import com.example.blog.service.ArticleService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.Optional;
@RestController
@RequestMapping("/api/articles")
public class ArticleController {
@Autowired
private ArticleService articleService;
@GetMapping
public List<Article> getAllArticles() {
return articleService.findAll();
}
@GetMapping("/{id}")
public Optional<Article> getArticleById(@PathVariable Long id) {
return articleService.findById(id);
}
@PostMapping
public Article createArticle(@RequestBody Article article) {
return articleService.save(article);
}
@PutMapping("/{id}")
public Article updateArticle(@PathVariable Long id, @RequestBody Article articleDetails) {
Article article = articleService.findById(id).orElseThrow(() -> new ResourceNotFoundException("Article not found with id " + id));
article.setTitle(articleDetails.getTitle());
article.setContent(articleDetails.getContent());
return articleService.save(article);
}
@DeleteMapping("/{id}")
public void deleteArticle(@PathVariable Long id) {
articleService.deleteById(id);
}
}
结论
通过Spring Boot,开发者可以高效地构建一个RESTful API,涵盖用户管理、文章管理等关键功能。本文详细介绍了RESTful API的基础知识、Spring Boot的核心功能、高级特性以及实践案例,帮助读者深入理解和掌握Spring Boot在RESTful API开发中的应用。希望本文能够为您进一步探索和应用Spring Boot提供有价值的参考。