1 前言
前文我们已经介绍了AOP概念Day11 AOP介绍,并将其总结如下:
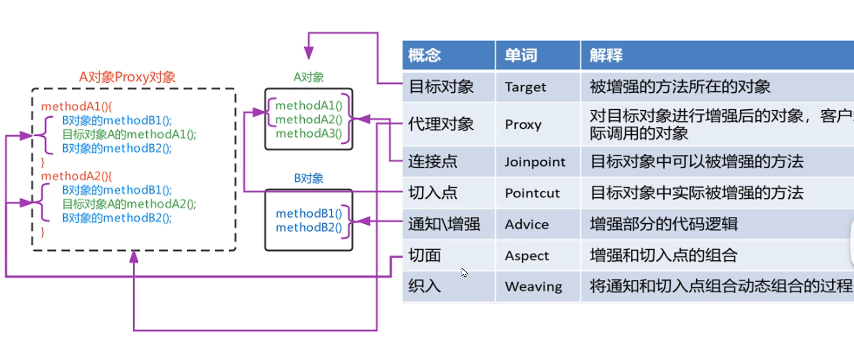
2 AOP 标签和expression表达式学习
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
">
<!--配置目标对象-->
<bean id="userService" class="org.example.service.impl.UserServiceImpl"/>
<!--配置增强对象-->
<bean id="myAdvice" class="org.example.advice.MyAdvice"/>
<!--增强逻辑-->
<bean class="org.example.processor.AopBeanPostProcessor"/>
<!----><context:property-placeholder location="classpath:jdbc.properties"/>
<!--AOP配置-->
<aop:config>
<!--切点配置-->
<aop:pointcut id="myPointcut" expression="execution(void org.example.service.impl.UserServiceImpl.show1())"/>
<!--配置织入-->
<aop:aspect ref="myAdvice">
<aop:before method="beforeAdvice" pointcut-ref="myPointcut"/>
</aop:aspect>
</aop:config>
<!--注解组件扫描:扫描指定的基本包及其子包下的类,识别使用@Component注解-->
<!-- <context:component-scan base-package="org.example"/>-->
</beans>
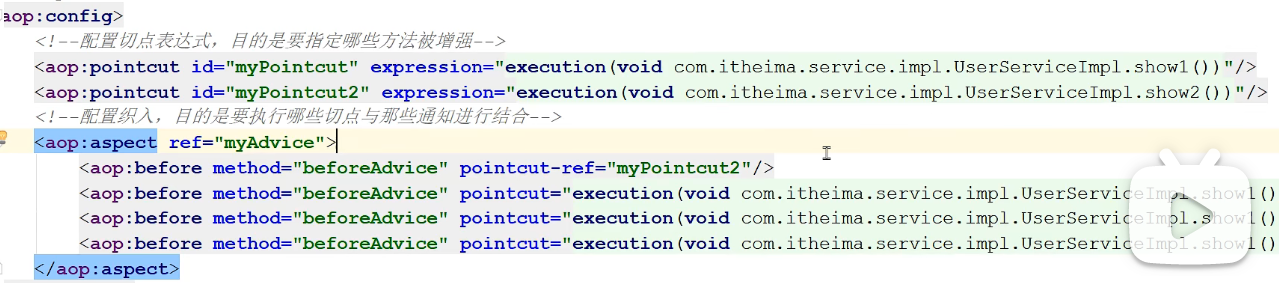
测试结果
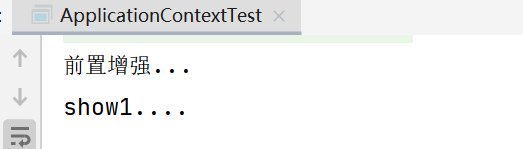
2.1 切面表达式配置语法

其中
访问修饰符可以省略不写
返回值类型、某一级包名、类名、方法名 可以使用*表示任意
包名与类名之间使用单点.表示该包下的类,使用双点..表示该包及其子包下的类
参数列表可以使用两个点..表示任意参数
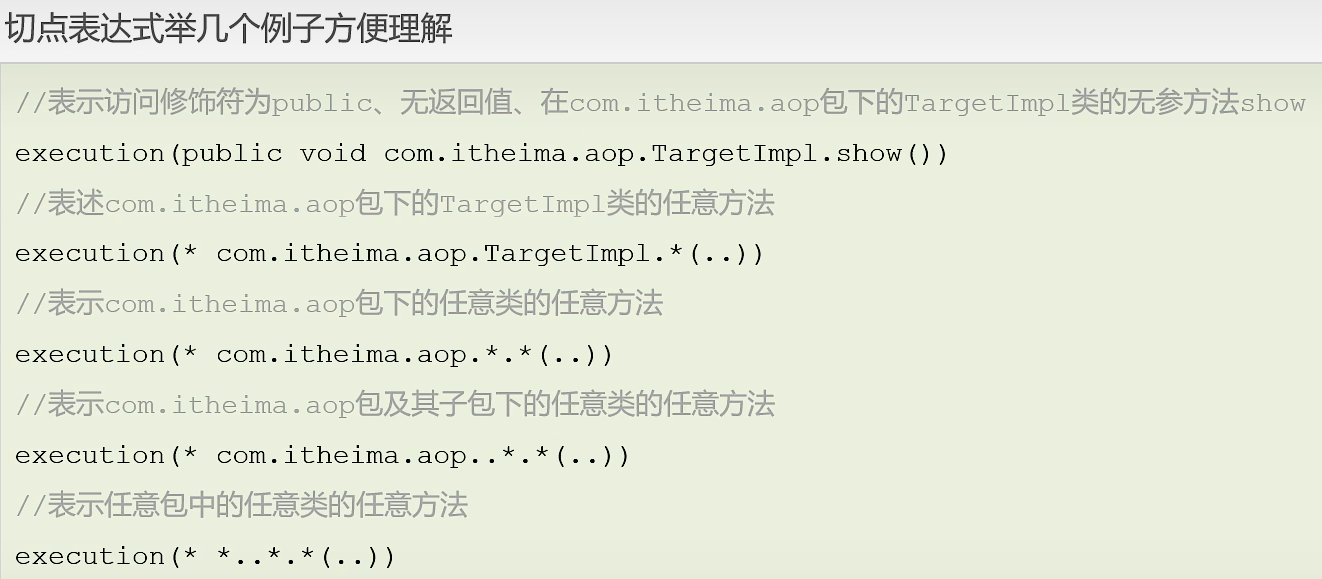
2.2 切面表达式的五种通知类型
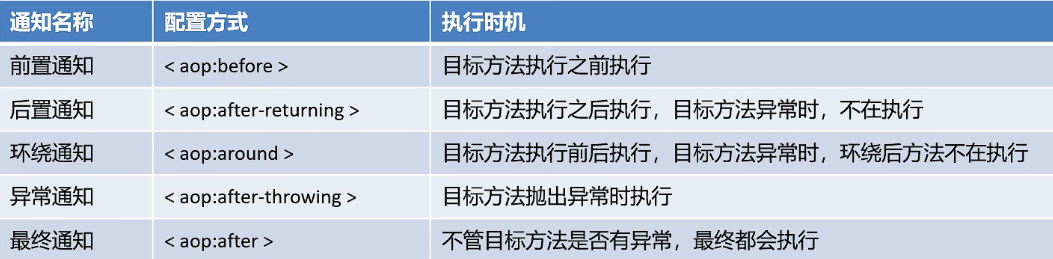
通知可以传入的参数有哪些呢?
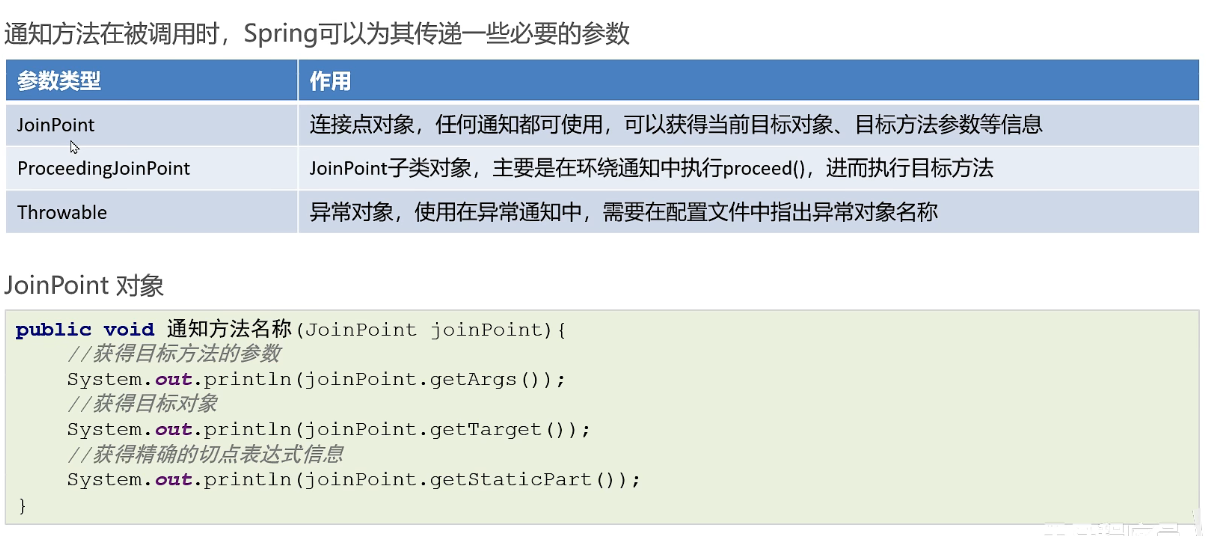
3 AOP配置的两种语法形式
采用<aspect> 配置切面:参考前文
采用<advice> 配置切面:实现Advice接口

<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="
http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd
">
<!--配置目标对象-->
<bean id="userService" class="org.example.service.impl.UserServiceImpl"/>
<!--配置增强对象-->
<bean id="myAdvice" class="org.example.advice.MyAdvice2"/>
<!----><context:property-placeholder location="classpath:jdbc.properties"/>
<!--AOP配置-->
<aop:config>
<!--切点配置-->
<aop:pointcut id="myPointcut" expression="execution(void org.example.service.impl.UserServiceImpl.show1())"/>
<!--配置织入-->
<aop:advisor advice-ref="myAdvice" pointcut-ref="myPointcut"/>
</aop:config>
</beans>
增强类
/**
* @author : msf
* @date : 2023/1/27
*/
public class MyAdvice2 implements MethodBeforeAdvice, AfterReturningAdvice {
@Override
public void before(Method method, Object[] args, Object target) throws Throwable {
System.out.println("前置通知....");
}
@Override
public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable {
System.out.println("后置通知....");
}
}
结果
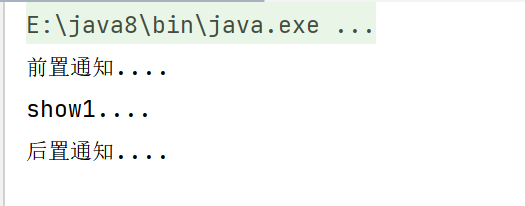
<aspect> 和 <advisor>区别
语法
advisor是通过实现接口来确认通知的类型
aspect是通过配置确认通知的类型,更加灵活
可配置的切面数量不同
一个advisor只能配置一个固定通知和一个切点表达式
一个aspect可以配置多个通知和多个切点表达式任意组合
使用场景不同:
允许随意搭配情况下可以使用aspect进行配置
如果通知类型单一、切面单一的情况下可以使用advisor进行配置
在通知类型已经固定,不用人为指定通知类型时,可以使用advisor进行配置,例如事务控制的配置