1、List的介绍
在集合框架中,List是一个接口,继承自Collection(也是一个接口)。
Collection也是一个接口,该接口中规范了后序容器中常用的一些方法,Iterable也是一个接口,表示实现该接口的类是可以逐个元素进行遍历的。
站在数据结构的角度来看,List就是一个线性表,即n个具有相同类型元素的有限序列,在该序列上可以执行增删改查以及变量等操作。
常用方法
下表是实现它的基础功能。
// 其中 E 是数据类型
注意:List是个接口,并不能直接用来实例化。
如果要使用,必须去实例化List的实现类。在集合框架中,ArrayList和LinkedList都实现了List接口。
2、顺序表
MyArrayList类
打印顺序表
其中 this.usedSize 代表获取顺序表的有效长度。
判断是否包含某个元素
查找该元素对应的下标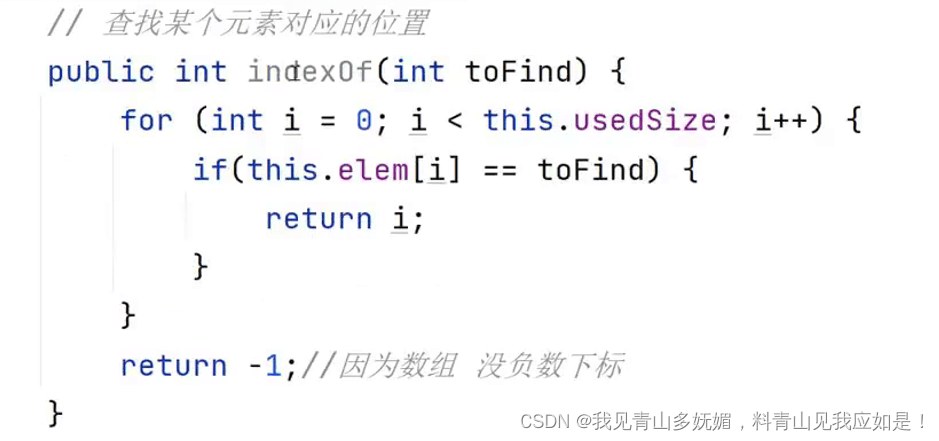
新增元素Add后插法
在pos位置新增元素
获取pos位置的元素
位置pos更新为新值value
删除第一次出现的关键字key
特殊情况
清空顺序表
3、顺序表–代码
package demo2;
import java.util.Arrays;
public class MyArrayList {
public int[] elem;
public int usedSize;//存储了多少个有效的数据
public static final int DEFAULT_SIZE = 5;
public MyArrayList() {
this.elem = new int[DEFAULT_SIZE];
}
// 打印顺序表,注意:该方法并不是顺序表中的方法,为了方便看测试结果给出的
public void display() {
for (int i = 0; i < this.usedSize; i++) {
System.out.print(this.elem[i] +" ");
}
System.out.println();
}
// 获取顺序表长度
public int size() {
return this.usedSize;
}
// 判定是否包含某个元素
public boolean contains(int toFind) {
for (int i = 0; i < this.usedSize; i++) {
if(this.elem[i] == toFind) {
return true;
}
}
return false;
}
// 查找某个元素对应的位置
public int indexOf(int toFind) {
for (int i = 0; i < this.usedSize; i++) {
if(this.elem[i] == toFind) {
return i;
}
}
return -1;//因为数组 没负数下标
}
// 新增元素,默认在数组最后新增
public void add(int data) {
if(this.isFull()) {
resize();
}
this.elem[this.usedSize] = data;
this.usedSize++;
}
/**
* 扩容
*/
private void resize() {
this.elem = Arrays.copyOf(this.elem,
2*this.elem.length);
}
/**
* 判断是否为满
* @return
*/
public boolean isFull() {
/*if(this.usedSize == this.elem.length) {
return true;
}
return false;*/
return this.usedSize == this.elem.length;
}
// 在 pos 位置新增元素 O(N)
public void add(int pos, int data) {
checkIndex(pos);
if(isFull()) {
resize();
}
for (int i = usedSize-1; i >= pos ; i--) {
elem[i+1] = elem[i];
}
elem[pos] = data;
usedSize++;
}
/**
* 检查add数据的时候,pos是否是合法的
* @param pos
*/
private void checkIndex(int pos) {
if(pos < 0 || pos > usedSize) {
throw new IndexOutOfException
("位置不合法,请检查位置的合法性!");
}
}
// 获取 pos 位置的元素
public int get(int pos) {
checkGetIndex(pos);
return elem[pos];
}
private void checkGetIndex(int pos) {
if(pos < 0 || pos >= usedSize) {
throw new IndexOutOfException
("get获取元素的时候,位置不合法,请检查位置的合法性!");
}
}
// 给 pos 位置的元素设为 value 1
public void set(int pos, int value) {
checkIndex(pos);
elem[pos] = value;
}
//删除第一次出现的关键字key O(n)
public boolean remove(int toRemove) {
int index = indexOf(toRemove);
if(index == -1) {
System.out.println("没有这个数据");
return false;
}
for (int i = index;i < usedSize-1;i++) {
elem[i] = elem[i+1];
}
usedSize --;
//elem[usedSize] = null;
// 如果里面是引用类型 那么此时就需要手动置空
elem[usedSize] = 0;
return true;
}
// 清空顺序表
public void clear() {
/*
for (int i = 0; i < usedSize; i++) {
elem[i] = null;
}
usedSize = 0;
*/
usedSize = 0;
}
}
package demo2;
public class Test {
public static void main(String[] args) {
MyArrayList myArrayList = new MyArrayList();
myArrayList.add(1);
myArrayList.add(2);
myArrayList.add(3);
myArrayList.display();
myArrayList.clear();
System.out.println("fdsfsa");
myArrayList.display();
/*myArrayList.remove(3);
myArrayList.display();
try {
myArrayList.add(1,99);
}catch (IndexOutOfException e) {
e.printStackTrace();
}
myArrayList.display();
System.out.println("============");
try {
System.out.println(myArrayList.get(4));
}catch (IndexOutOfException e) {
e.printStackTrace();
}*/
}
}