背景
- 函数式接口很简单,但是不是每一个函数式接口都需要我们自己来写
- jdk 根据
有无参数,有无返回值,参数的个数和类型,返回值的类型
提前定义了一些通用
的函数式接口
IntPredicate
- 参数:有一个,类型是int类型
- 返回值:返回值是boolean类型 所以是Predicate
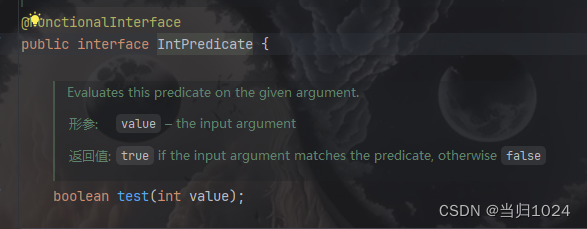
IntPredicate type1_1 = (int a) -> (a & 1) == 0;
@FunctionalInterface
interface MyInterface1{
boolean op(int arg1);
}
IntBinaryOperator
- 参数:两个int 所以是Binary
- 返回值:和参数一样是int 所以是Operator
IntBinaryOperator type4_1 = (int a, int b) -> a + b;
IntBinaryOperator type5_1 = (int a, int b) -> a * b;
@FunctionalInterface
interface MyInterface3{
int op(int num1, int num2);
}
Supplier
- 参数:无
- 返回值:没有参数但是有返回值,所以是Supplier
Supplier<Student> type6_2 = () -> new Student("张三", 18);
Supplier<List<Student>> type7_2 = () -> {
List<Student> list = new ArrayList<>();
list.add(new Student("张三", 18));
list.add(new Student("李四", 19));
return list;
};
@FunctionalInterface
interface MyInterface5_1<T>{
T op() ;
}
Function
- 有参数,也有返回值
- 但是参数类型和返回值类型不一样,所以是Function
Function<Student,String> type8_2 = (Student student) -> student.getName();
Function<Student,Integer> type9_2 = (Student student) -> student.getage();
@FunctionalInterface
interface MyInterface8<I,O>{
O op(I inObj) ;
}
常见的函数式接口
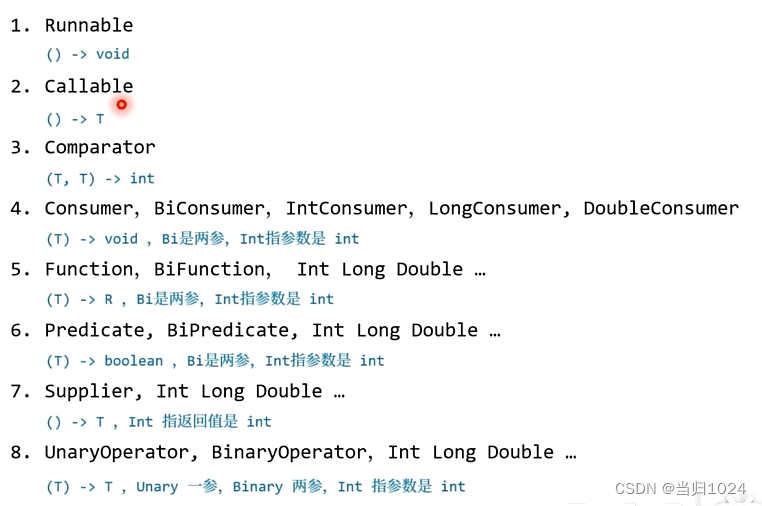
命名规则
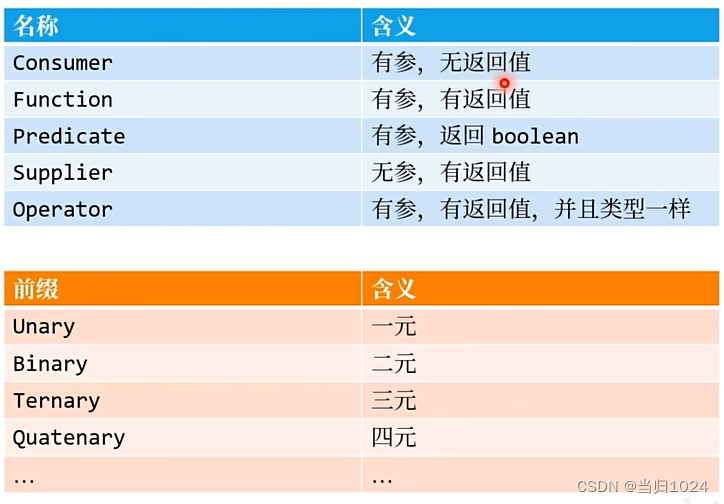