文章目录
- 1. Pinia 是什么
- 2. Pinia 功能作用
- 3. 手动添加Pinia到Vue项目
- 4. Pinia基础使用
- 5. getters实现
- 6. action异步实现
- 7. storeToRefs工具函数
- 8. Pinia的调试
- 9. 总结
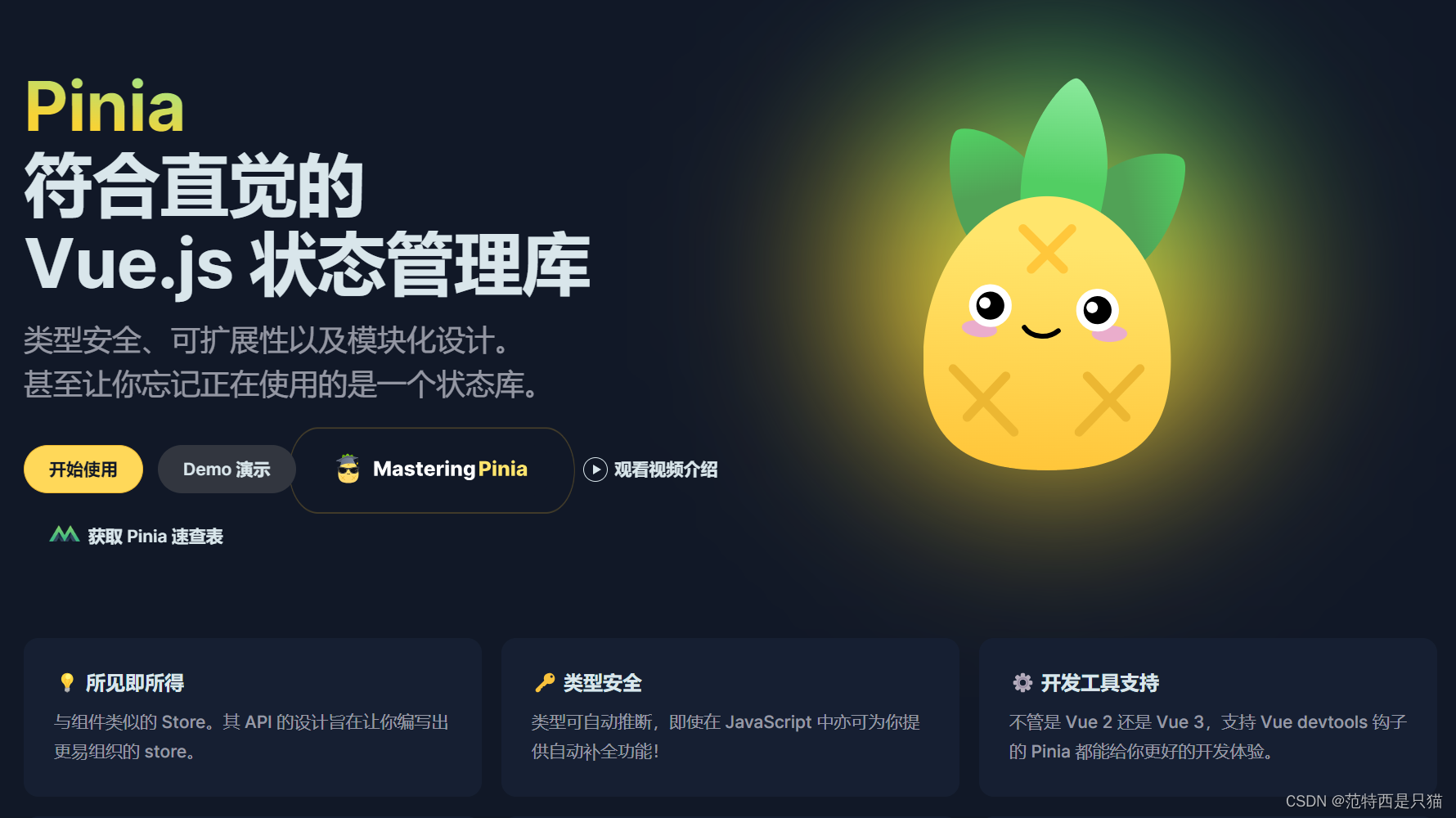
1. Pinia 是什么
- Pinia 是
Vue
的专属的最新状态管理库
- 是
Vuex
状态管理工具的替代品和 Vuex 一样为 Vue 项目提供共享状态管理工具 - 并且支持vue2和vue3,主要是为
vue3提供状态管理
,支持typescript
- Pinia可以创建多个全局仓库,不用像 Vuex 一个仓库嵌套模块,结构复杂。管
理数据简单,提供数据和修改数据的逻辑即可,不像Vuex需要记忆太多的API
2. Pinia 功能作用
- 提供更加简单的API (去掉了 mutation )
- 提供符合组合式风格的API (和 Vue3 新语法统一)
- 去掉了
modules
的概念,每一个 store 都是一个独立的模块 - 配合
TypeScript
更加友好,提供可靠的类型推断
3. 手动添加Pinia到Vue项目
Pinia可以在项目创建时自动添加,现在我们初次学习,从零开始: 2.1 使用 Vite 创建一个空的TS + Vue3项目
npm create vite@latest vue-pinia-ts -- --template vue-ts
按照官方文档安装 pinia 到项目中
cnpm i pinia
4. Pinia基础使用
- 在 src 中定义一个 stores 文件夹,新建 counter.ts 文件
- counter.ts 组件使用 store
import { defineStore } from 'pinia'
import { ref } from 'vue'
export const useCounterStore = defineStore('counter', () => {
// 数据(state)
const count = ref(0)
// 修改数据的方法(action)
const increment = () => {
count.value++
}
// 已对象的形式返回
return {
count,
increment
}
})
- main.js 中引入 pinia
...
import { createApp } from 'vue'
import App from './App.vue'
import { createPinia } from 'pinia'
const pinia = createPinia();
createApp(App).use(pinia).mount('#app')
- App.vue 页面中使用
<script setup>
// 1. 导入 useCounterStore 方法
import { useCounterStore } from './stores/counter'
// 2. 执行方法得到counterStore对象
const counterStore = useCounterStore();
</script>
<template>
<div>{{counterStore.count}}</div>
</template>
5. getters实现
- Pinia中的
getters
直接使用computed
函数 进行模拟, 组件中需要使用需要把 getters return出去
const doubleCount = computed(() => count.value * 2)
6. action异步实现
方式:异步action
函数的写法和组件中获取异步数据的写法完全一致
const getList = async ()=>{
const res = await axios.request<接口数据类型>({})
}
7. storeToRefs工具函数
使用 storeToRefs
函数可以辅助保持数据(state + getter)的响应式解构
<script setup>
import { storeToRefs } from 'pinia'
import { useCounterStore } from './stores/counter'
// 使用storeToRefs保持数据响应式
const { count } = storeToRefs(useCounterStore());
function add() {
counterStore.increment()
}
</script>
<template>
<p class="mt-4">
number:<strong class="text-green-500"> {{ count }} </strong>
</p>
<button class="btn" @click="add">
Add
</button>
</template>
8. Pinia的调试
Vue官方的 dev-tools
调试工具 对 Pinia直接支持,可以直接进行调试
9. 总结
- 通过
const useCounterStore = defineStore('counter', () => {})
创建数据仓库 - vuex 中的 state 在 pinia 中可以引用
ref
和reactive
创建响应式数据 - vuex 中的
getters
在 pinia 中可以引用computed
创建计算属性 - vuex 中的
mutations
和actions
在 pinia 中就是普通函数
, 同步异步都可以